text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_adaptive_scaffold/flutter_adaptive_scaffold.dart';
import 'package:flutter_test/flutter_test.dart';
import 'test_breakpoints.dart';
AnimatedWidget leftOutIn(Widget child, Animation<double> animation) {
return SlideTransition(
key: Key('in-${child.key}'),
position: Tween<Offset>(
begin: const Offset(-1, 0),
end: Offset.zero,
).animate(animation),
child: child,
);
}
AnimatedWidget leftInOut(Widget child, Animation<double> animation) {
return SlideTransition(
key: Key('out-${child.key}'),
position: Tween<Offset>(
begin: Offset.zero,
end: const Offset(-1, 0),
).animate(animation),
child: child,
);
}
class TestScaffold extends StatefulWidget {
const TestScaffold({
super.key,
this.initialIndex = 0,
this.isAnimated = true,
this.appBarBreakpoint,
});
final int? initialIndex;
final bool isAnimated;
final Breakpoint? appBarBreakpoint;
static const List<NavigationDestination> destinations =
<NavigationDestination>[
NavigationDestination(
key: Key('Inbox'),
icon: Icon(Icons.inbox),
label: 'Inbox',
),
NavigationDestination(
key: Key('Articles'),
icon: Icon(Icons.article),
label: 'Articles',
),
NavigationDestination(
key: Key('Chat'),
icon: Icon(Icons.chat),
label: 'Chat',
),
];
@override
State<TestScaffold> createState() => TestScaffoldState();
}
class TestScaffoldState extends State<TestScaffold> {
late int? index = widget.initialIndex;
@override
Widget build(BuildContext context) {
return AdaptiveScaffold(
selectedIndex: index,
onSelectedIndexChange: (int index) {
setState(() {
this.index = index;
});
},
drawerBreakpoint: NeverOnBreakpoint(),
appBarBreakpoint: widget.appBarBreakpoint,
internalAnimations: widget.isAnimated,
smallBreakpoint: TestBreakpoint0(),
mediumBreakpoint: TestBreakpoint800(),
largeBreakpoint: TestBreakpoint1000(),
destinations: TestScaffold.destinations,
smallBody: (_) => Container(color: Colors.red),
body: (_) => Container(color: Colors.green),
largeBody: (_) => Container(color: Colors.blue),
smallSecondaryBody: (_) => Container(color: Colors.red),
secondaryBody: (_) => Container(color: Colors.green),
largeSecondaryBody: (_) => Container(color: Colors.blue),
leadingExtendedNavRail: const Text('leading_extended'),
leadingUnextendedNavRail: const Text('leading_unextended'),
trailingNavRail: const Text('trailing'),
);
}
}
enum SimulatedLayout {
small(width: 400, navSlotKey: 'bottomNavigation'),
medium(width: 800, navSlotKey: 'primaryNavigation'),
large(width: 1100, navSlotKey: 'primaryNavigation1');
const SimulatedLayout({
required double width,
required this.navSlotKey,
}) : _width = width;
final double _width;
final double _height = 800;
final String navSlotKey;
static const Color navigationRailThemeBgColor = Colors.white;
static const IconThemeData selectedIconThemeData = IconThemeData(
color: Colors.red,
size: 32.0,
);
static const IconThemeData unSelectedIconThemeData = IconThemeData(
color: Colors.black,
size: 24.0,
);
Size get size => Size(_width, _height);
MaterialApp app({
int? initialIndex,
bool animations = true,
Breakpoint? appBarBreakpoint,
}) {
return MaterialApp(
theme: ThemeData.light().copyWith(
navigationRailTheme: const NavigationRailThemeData(
backgroundColor: navigationRailThemeBgColor,
selectedIconTheme: selectedIconThemeData,
unselectedIconTheme: unSelectedIconThemeData,
),
),
home: MediaQuery(
data: MediaQueryData(
size: size,
padding: const EdgeInsets.only(top: 30),
),
child: TestScaffold(
initialIndex: initialIndex,
isAnimated: animations,
appBarBreakpoint: appBarBreakpoint,
),
),
);
}
MediaQuery slot(WidgetTester tester) {
return MediaQuery(
data: MediaQueryData.fromView(tester.view)
.copyWith(size: Size(_width, _height)),
child: Theme(
data: ThemeData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: SlotLayout(
config: <Breakpoint, SlotLayoutConfig>{
Breakpoints.small: SlotLayout.from(
key: const Key('Breakpoints.small'),
builder: (BuildContext context) => Container(),
),
Breakpoints.smallMobile: SlotLayout.from(
key: const Key('Breakpoints.smallMobile'),
builder: (BuildContext context) => Container(),
),
Breakpoints.smallDesktop: SlotLayout.from(
key: const Key('Breakpoints.smallDesktop'),
builder: (BuildContext context) => Container(),
),
Breakpoints.medium: SlotLayout.from(
key: const Key('Breakpoints.medium'),
builder: (BuildContext context) => Container(),
),
Breakpoints.mediumMobile: SlotLayout.from(
key: const Key('Breakpoints.mediumMobile'),
builder: (BuildContext context) => Container(),
),
Breakpoints.mediumDesktop: SlotLayout.from(
key: const Key('Breakpoints.mediumDesktop'),
builder: (BuildContext context) => Container(),
),
Breakpoints.large: SlotLayout.from(
key: const Key('Breakpoints.large'),
builder: (BuildContext context) => Container(),
),
Breakpoints.largeMobile: SlotLayout.from(
key: const Key('Breakpoints.largeMobile'),
builder: (BuildContext context) => Container(),
),
Breakpoints.largeDesktop: SlotLayout.from(
key: const Key('Breakpoints.largeDesktop'),
builder: (BuildContext context) => Container(),
),
},
),
),
),
);
}
}
| packages/packages/flutter_adaptive_scaffold/test/simulated_layout.dart/0 | {
"file_path": "packages/packages/flutter_adaptive_scaffold/test/simulated_layout.dart",
"repo_id": "packages",
"token_count": 2645
} | 1,022 |
name: example
description: A project that showcases how to enable the recommended lints for Flutter apps, packages, and plugins.
publish_to: none
environment:
sdk: ^3.1.0
# Add the latest version of `package:flutter_lints` as a dev_dependency. The
# lint set provided by this package is activated in the `analysis_options.yaml`
# file located next to this `pubspec.yaml` file.
dev_dependencies:
flutter_lints: ^1.0.0 # Check https://pub.dev/packages/flutter_lints for latest version number.
| packages/packages/flutter_lints/example/pubspec.yaml/0 | {
"file_path": "packages/packages/flutter_lints/example/pubspec.yaml",
"repo_id": "packages",
"token_count": 154
} | 1,023 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import 'package:markdown/markdown.dart' as md;
import '../shared/dropdown_menu.dart' as dropdown;
import '../shared/markdown_demo_widget.dart';
import '../shared/markdown_extensions.dart';
// ignore_for_file: public_member_api_docs
const String _notes = '''
# Wrap Alignment Demo
---
The Wrap Alignment Demo shows the effect of defining a wrap alignment for
various Markdown elements. Wrap alignments for the block elements text
paragraphs, headers, ordered and unordered lists, blockquotes, and code blocks
are set in the **MarkdownStyleSheet**. This demo shows the effect of setting
this parameter universally on these block elements for illustration purposes,
but they are independent settings.
This demo also shows the effect of setting the **MarkdownStyleSheet** block
spacing parameter. The Markdown widget lays out block elements in a column using
**SizedBox** widgets to separate widgets with formatted output. The block
spacing parameter sets the height of the **SizedBox**.
''';
// TODO(goderbauer): Restructure the examples to avoid this ignore, https://github.com/flutter/flutter/issues/110208.
// ignore: avoid_implementing_value_types
class WrapAlignmentDemo extends StatefulWidget implements MarkdownDemoWidget {
const WrapAlignmentDemo({super.key});
static const String _title = 'Wrap Alignment Demo';
@override
String get title => WrapAlignmentDemo._title;
@override
String get description => 'Shows the effect the wrap alignment and block '
'spacing parameters have on various Markdown tagged elements.';
@override
Future<String> get data =>
rootBundle.loadString('assets/markdown_test_page.md');
@override
Future<String> get notes => Future<String>.value(_notes);
@override
State<WrapAlignmentDemo> createState() => _WrapAlignmentDemoState();
}
class _WrapAlignmentDemoState extends State<WrapAlignmentDemo> {
double _blockSpacing = 8.0;
WrapAlignment _wrapAlignment = WrapAlignment.start;
final Map<String, WrapAlignment> _wrapAlignmentMenuItems =
Map<String, WrapAlignment>.fromIterables(
WrapAlignment.values.map((WrapAlignment e) => e.displayTitle),
WrapAlignment.values,
);
static const List<double> _spacing = <double>[4.0, 8.0, 16.0, 24.0, 32.0];
final Map<String, double> _blockSpacingMenuItems =
Map<String, double>.fromIterables(
_spacing.map((double e) => e.toString()),
_spacing,
);
@override
Widget build(BuildContext context) {
return FutureBuilder<String>(
future: widget.data,
builder: (BuildContext context, AsyncSnapshot<String> snapshot) {
if (snapshot.connectionState == ConnectionState.done) {
return Column(
children: <Widget>[
dropdown.DropdownMenu<WrapAlignment>(
items: _wrapAlignmentMenuItems,
label: 'Wrap Alignment:',
initialValue: _wrapAlignment,
onChanged: (WrapAlignment? value) {
if (value != _wrapAlignment) {
setState(() {
_wrapAlignment = value!;
});
}
},
),
dropdown.DropdownMenu<double>(
items: _blockSpacingMenuItems,
label: 'Block Spacing:',
initialValue: _blockSpacing,
onChanged: (double? value) {
if (value != _blockSpacing) {
setState(() {
_blockSpacing = value!;
});
}
},
),
Expanded(
child: Markdown(
key: Key(_wrapAlignment.toString()),
data: snapshot.data!,
imageDirectory: 'https://raw.githubusercontent.com',
styleSheet:
MarkdownStyleSheet.fromTheme(Theme.of(context)).copyWith(
blockSpacing: _blockSpacing,
textAlign: _wrapAlignment,
pPadding: const EdgeInsets.only(bottom: 4.0),
h1Align: _wrapAlignment,
h1Padding: const EdgeInsets.only(left: 4.0),
h2Align: _wrapAlignment,
h2Padding: const EdgeInsets.only(left: 8.0),
h3Align: _wrapAlignment,
h3Padding: const EdgeInsets.only(left: 12.0),
h4Align: _wrapAlignment,
h4Padding: const EdgeInsets.only(left: 16.0),
h5Align: _wrapAlignment,
h5Padding: const EdgeInsets.only(left: 20.0),
h6Align: _wrapAlignment,
h6Padding: const EdgeInsets.only(left: 24.0),
unorderedListAlign: _wrapAlignment,
orderedListAlign: _wrapAlignment,
blockquoteAlign: _wrapAlignment,
codeblockAlign: _wrapAlignment,
),
paddingBuilders: <String, MarkdownPaddingBuilder>{
'p': CustomPaddingBuilder()
},
),
),
],
);
} else {
return const CircularProgressIndicator();
}
},
);
}
}
class CustomPaddingBuilder extends MarkdownPaddingBuilder {
final EdgeInsets _padding = const EdgeInsets.only(left: 10.0);
bool paddingUse = true;
@override
void visitElementBefore(md.Element element) {
if (element.children!.length == 1 && element.children![0] is md.Element) {
final md.Element child = element.children![0] as md.Element;
paddingUse = child.tag != 'img';
} else {
paddingUse = true;
}
}
@override
EdgeInsets getPadding() {
if (paddingUse) {
return _padding;
} else {
return EdgeInsets.zero;
}
}
}
| packages/packages/flutter_markdown/example/lib/demos/wrap_alignment_demo.dart/0 | {
"file_path": "packages/packages/flutter_markdown/example/lib/demos/wrap_alignment_demo.dart",
"repo_id": "packages",
"token_count": 2724
} | 1,024 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import 'package:flutter_test/flutter_test.dart';
import 'utils.dart';
void main() => defineTests();
void defineTests() {
group(
'structure',
() {
testWidgets(
'footnote is detected and handle correctly',
(WidgetTester tester) async {
const String data = 'Foo[^a]\n[^a]: Bar';
await tester.pumpWidget(
boilerplate(
const MarkdownBody(
data: data,
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
expectTextStrings(widgets, <String>[
'Foo1',
'1.',
'Bar ↩',
]);
},
);
testWidgets(
'footnote is detected and handle correctly for selectable markdown',
(WidgetTester tester) async {
const String data = 'Foo[^a]\n[^a]: Bar';
await tester.pumpWidget(
boilerplate(
const MarkdownBody(
data: data,
selectable: true,
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
expectTextStrings(widgets, <String>[
'Foo1',
'1.',
'Bar ↩',
]);
},
);
testWidgets(
'ignore footnotes without description',
(WidgetTester tester) async {
const String data = 'Foo[^1] Bar[^2]\n[^1]: Bar';
await tester.pumpWidget(
boilerplate(
const MarkdownBody(
data: data,
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
expectTextStrings(widgets, <String>[
'Foo1 Bar[^2]',
'1.',
'Bar ↩',
]);
},
);
testWidgets(
'ignore superscripts and footnotes order',
(WidgetTester tester) async {
const String data = '[^2]: Bar \n [^1]: Foo \n Foo[^f] Bar[^b]';
await tester.pumpWidget(
boilerplate(
const MarkdownBody(
data: data,
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
expectTextStrings(widgets, <String>[
'Foo1 Bar2',
'1.',
'Foo ↩',
'2.',
'Bar ↩',
]);
},
);
testWidgets(
'handle two digits superscript',
(WidgetTester tester) async {
const String data = '''
1[^1] 2[^2] 3[^3] 4[^4] 5[^5] 6[^6] 7[^7] 8[^8] 9[^9] 10[^10]
[^1]:1
[^2]:2
[^3]:3
[^4]:4
[^5]:5
[^6]:6
[^7]:7
[^8]:8
[^9]:9
[^10]:10
''';
await tester.pumpWidget(
boilerplate(
const MarkdownBody(
data: data,
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
expectTextStrings(widgets, <String>[
'11 22 33 44 55 66 77 88 99 1010',
'1.',
'1 ↩',
'2.',
'2 ↩',
'3.',
'3 ↩',
'4.',
'4 ↩',
'5.',
'5 ↩',
'6.',
'6 ↩',
'7.',
'7 ↩',
'8.',
'8 ↩',
'9.',
'9 ↩',
'10.',
'10 ↩',
]);
},
);
},
);
group(
'superscript textstyle replacing',
() {
testWidgets(
'superscript has correct fontfeature',
(WidgetTester tester) async {
const String data = 'Foo[^a]\n[^a]: Bar';
await tester.pumpWidget(
boilerplate(
const MarkdownBody(
data: data,
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
final Text text =
widgets.firstWhere((Widget widget) => widget is Text) as Text;
final TextSpan span = text.textSpan! as TextSpan;
final List<InlineSpan>? children = span.children;
expect(children, isNotNull);
expect(children!.length, 2);
expect(children[1].style, isNotNull);
expect(children[1].style!.fontFeatures?.length, 1);
expect(children[1].style!.fontFeatures?.first.feature, 'sups');
},
);
testWidgets(
'superscript index has the same font style like text',
(WidgetTester tester) async {
const String data = '# Foo[^a]\n[^a]: Bar';
await tester.pumpWidget(
boilerplate(
const MarkdownBody(
data: data,
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
final Text text =
widgets.firstWhere((Widget widget) => widget is Text) as Text;
final TextSpan span = text.textSpan! as TextSpan;
final List<InlineSpan>? children = span.children;
expect(children![0].style, isNotNull);
expect(children[1].style!.fontSize, children[0].style!.fontSize);
expect(children[1].style!.fontFamily, children[0].style!.fontFamily);
expect(children[1].style!.fontStyle, children[0].style!.fontStyle);
expect(children[1].style!.fontSize, children[0].style!.fontSize);
},
);
testWidgets(
'link is correctly copied to new superscript index',
(WidgetTester tester) async {
final List<MarkdownLink> linkTapResults = <MarkdownLink>[];
const String data = 'Foo[^a]\n[^a]: Bar';
await tester.pumpWidget(
boilerplate(
MarkdownBody(
data: data,
onTapLink: (String text, String? href, String title) =>
linkTapResults.add(MarkdownLink(text, href, title)),
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
final Text text =
widgets.firstWhere((Widget widget) => widget is Text) as Text;
final TextSpan span = text.textSpan! as TextSpan;
final List<Type> gestureRecognizerTypes = <Type>[];
span.visitChildren((InlineSpan inlineSpan) {
if (inlineSpan is TextSpan) {
final TapGestureRecognizer? recognizer =
inlineSpan.recognizer as TapGestureRecognizer?;
gestureRecognizerTypes.add(recognizer?.runtimeType ?? Null);
if (recognizer != null) {
recognizer.onTap!();
}
}
return true;
});
expect(span.children!.length, 2);
expect(
gestureRecognizerTypes,
orderedEquals(<Type>[Null, TapGestureRecognizer]),
);
expectLinkTap(linkTapResults[0], const MarkdownLink('1', '#fn-a'));
},
);
},
);
}
| packages/packages/flutter_markdown/test/footnote_test.dart/0 | {
"file_path": "packages/packages/flutter_markdown/test/footnote_test.dart",
"repo_id": "packages",
"token_count": 3918
} | 1,025 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import 'package:flutter_test/flutter_test.dart';
import 'utils.dart';
void main() => defineTests();
void defineTests() {
group('Text Alignment', () {
testWidgets(
'apply text alignments from stylesheet',
(WidgetTester tester) async {
final ThemeData theme =
ThemeData.light().copyWith(textTheme: textTheme);
final MarkdownStyleSheet style1 =
MarkdownStyleSheet.fromTheme(theme).copyWith(
h1Align: WrapAlignment.center,
h3Align: WrapAlignment.end,
);
const String data = '# h1\n ## h2';
await tester.pumpWidget(
boilerplate(
MarkdownBody(
data: data,
styleSheet: style1,
),
),
);
final Iterable<Widget> widgets = selfAndDescendantWidgetsOf(
find.byType(MarkdownBody),
tester,
);
expectWidgetTypes(widgets, <Type>[
MarkdownBody,
Column,
Column,
Wrap,
Text,
RichText,
SizedBox,
Column,
Wrap,
Text,
RichText,
]);
expect(
(widgets.firstWhere((Widget w) => w is RichText) as RichText)
.textAlign,
TextAlign.center);
expect((widgets.last as RichText).textAlign, TextAlign.start,
reason: 'default alignment if none is set in stylesheet');
},
);
testWidgets(
'should align formatted text',
(WidgetTester tester) async {
final ThemeData theme =
ThemeData.light().copyWith(textTheme: textTheme);
final MarkdownStyleSheet style =
MarkdownStyleSheet.fromTheme(theme).copyWith(
textAlign: WrapAlignment.spaceBetween,
);
const String data = 'hello __my formatted text__';
await tester.pumpWidget(
boilerplate(
MarkdownBody(
data: data,
styleSheet: style,
),
),
);
final RichText text =
tester.widgetList(find.byType(RichText)).single as RichText;
expect(text.textAlign, TextAlign.justify);
},
);
testWidgets(
'should align selectable text',
(WidgetTester tester) async {
final ThemeData theme =
ThemeData.light().copyWith(textTheme: textTheme);
final MarkdownStyleSheet style =
MarkdownStyleSheet.fromTheme(theme).copyWith(
textAlign: WrapAlignment.spaceBetween,
);
const String data = 'hello __my formatted text__';
await tester.pumpWidget(
boilerplate(
MediaQuery(
data: const MediaQueryData(),
child: MarkdownBody(
data: data,
styleSheet: style,
selectable: true,
),
),
),
);
final SelectableText text = tester
.widgetList(find.byType(SelectableText))
.single as SelectableText;
expect(text.textAlign, TextAlign.justify);
},
);
});
}
| packages/packages/flutter_markdown/test/text_alignment_test.dart/0 | {
"file_path": "packages/packages/flutter_markdown/test/text_alignment_test.dart",
"repo_id": "packages",
"token_count": 1644
} | 1,026 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:collection';
import 'package:meta/meta.dart';
/// Generates an [AppContext] value.
///
/// Generators are allowed to return `null`, in which case the context will
/// store the `null` value as the value for that type.
typedef Generator = dynamic Function();
/// An exception thrown by [AppContext] when you try to get a [Type] value from
/// the context, and the instantiation of the value results in a dependency
/// cycle.
class ContextDependencyCycleException implements Exception {
ContextDependencyCycleException._(this.cycle);
/// The dependency cycle (last item depends on first item).
final List<Type> cycle;
@override
String toString() => 'Dependency cycle detected: ${cycle.join(' -> ')}';
}
/// The Zone key used to look up the [AppContext].
@visibleForTesting
const Object contextKey = _Key.key;
/// The current [AppContext], as determined by the [Zone] hierarchy.
///
/// This will be the first context found as we scan up the zone hierarchy, or
/// the "root" context if a context cannot be found in the hierarchy. The root
/// context will not have any values associated with it.
///
/// This is guaranteed to never return `null`.
AppContext get context =>
Zone.current[contextKey] as AppContext? ?? AppContext._root;
/// A lookup table (mapping types to values) and an implied scope, in which
/// code is run.
///
/// [AppContext] is used to define a singleton injection context for code that
/// is run within it. Each time you call [run], a child context (and a new
/// scope) is created.
///
/// Child contexts are created and run using zones. To read more about how
/// zones work, see https://api.dart.dev/stable/dart-async/Zone-class.html.
class AppContext {
AppContext._(
this._parent,
this.name, [
this._overrides = const <Type, Generator>{},
this._fallbacks = const <Type, Generator>{},
]);
final String? name;
final AppContext? _parent;
final Map<Type, Generator> _overrides;
final Map<Type, Generator> _fallbacks;
final Map<Type, dynamic> _values = <Type, dynamic>{};
List<Type>? _reentrantChecks;
/// Bootstrap context.
static final AppContext _root = AppContext._(null, 'ROOT');
dynamic _boxNull(dynamic value) => value ?? _BoxedNull.instance;
dynamic _unboxNull(dynamic value) =>
value == _BoxedNull.instance ? null : value;
/// Returns the generated value for [type] if such a generator exists.
///
/// If [generators] does not contain a mapping for the specified [type], this
/// returns `null`.
///
/// If a generator existed and generated a `null` value, this will return a
/// boxed value indicating null.
///
/// If a value for [type] has already been generated by this context, the
/// existing value will be returned, and the generator will not be invoked.
///
/// If the generator ends up triggering a reentrant call, it signals a
/// dependency cycle, and a [ContextDependencyCycleException] will be thrown.
dynamic _generateIfNecessary(Type type, Map<Type, Generator> generators) {
if (!generators.containsKey(type)) {
return null;
}
return _values.putIfAbsent(type, () {
_reentrantChecks ??= <Type>[];
final int index = _reentrantChecks!.indexOf(type);
if (index >= 0) {
// We're already in the process of trying to generate this type.
throw ContextDependencyCycleException._(
UnmodifiableListView<Type>(_reentrantChecks!.sublist(index)));
}
_reentrantChecks!.add(type);
try {
return _boxNull(generators[type]!());
} finally {
_reentrantChecks!.removeLast();
if (_reentrantChecks!.isEmpty) {
_reentrantChecks = null;
}
}
});
}
/// Gets the value associated with the specified [type], or `null` if no
/// such value has been associated.
T? get<T>() {
dynamic value = _generateIfNecessary(T, _overrides);
if (value == null && _parent != null) {
value = _parent!.get<T>();
}
return _unboxNull(value ?? _generateIfNecessary(T, _fallbacks)) as T?;
}
/// Runs [body] in a child context and returns the value returned by [body].
///
/// If [overrides] is specified, the child context will return corresponding
/// values when consulted via [operator[]].
///
/// If [fallbacks] is specified, the child context will return corresponding
/// values when consulted via [operator[]] only if its parent context didn't
/// return such a value.
///
/// If [name] is specified, the child context will be assigned the given
/// name. This is useful for debugging purposes and is analogous to naming a
/// thread in Java.
Future<V> run<V>({
required FutureOr<V> Function() body,
String? name,
Map<Type, Generator>? overrides,
Map<Type, Generator>? fallbacks,
ZoneSpecification? zoneSpecification,
}) async {
final AppContext child = AppContext._(
this,
name,
Map<Type, Generator>.unmodifiable(overrides ?? const <Type, Generator>{}),
Map<Type, Generator>.unmodifiable(fallbacks ?? const <Type, Generator>{}),
);
return runZoned<Future<V>>(
() async => await body(),
zoneValues: <_Key, AppContext>{_Key.key: child},
zoneSpecification: zoneSpecification,
);
}
@override
String toString() {
final StringBuffer buf = StringBuffer();
String indent = '';
AppContext? ctx = this;
while (ctx != null) {
buf.write('AppContext');
if (ctx.name != null) {
buf.write('[${ctx.name}]');
}
if (ctx._overrides.isNotEmpty) {
buf.write('\n$indent overrides: [${ctx._overrides.keys.join(', ')}]');
}
if (ctx._fallbacks.isNotEmpty) {
buf.write('\n$indent fallbacks: [${ctx._fallbacks.keys.join(', ')}]');
}
if (ctx._parent != null) {
buf.write('\n$indent parent: ');
}
ctx = ctx._parent;
indent += ' ';
}
return buf.toString();
}
}
/// Private key used to store the [AppContext] in the [Zone].
class _Key {
const _Key();
static const _Key key = _Key();
@override
String toString() => 'context';
}
/// Private object that denotes a generated `null` value.
class _BoxedNull {
const _BoxedNull();
static const _BoxedNull instance = _BoxedNull();
}
| packages/packages/flutter_migrate/lib/src/base/context.dart/0 | {
"file_path": "packages/packages/flutter_migrate/lib/src/base/context.dart",
"repo_id": "packages",
"token_count": 2175
} | 1,027 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:yaml/yaml.dart';
import 'base/file_system.dart';
import 'base/logger.dart';
import 'base/terminal.dart';
import 'result.dart';
import 'utils.dart';
const String _kMergedFilesKey = 'merged_files';
const String _kConflictFilesKey = 'conflict_files';
const String _kAddedFilesKey = 'added_files';
const String _kDeletedFilesKey = 'deleted_files';
/// Represents the manifest file that tracks the contents of the current
/// migration working directory.
///
/// This manifest file is created with the MigrateResult of a computeMigration run.
class MigrateManifest {
/// Creates a new manifest from a MigrateResult.
MigrateManifest({
required this.migrateRootDir,
required this.migrateResult,
});
/// Parses an existing migrate manifest.
MigrateManifest.fromFile(File manifestFile)
: migrateResult = MigrateResult.empty(),
migrateRootDir = manifestFile.parent {
final Object? yamlContents = loadYaml(manifestFile.readAsStringSync());
if (yamlContents is! YamlMap) {
throw Exception(
'Invalid .migrate_manifest file in the migrate working directory. File is not a Yaml map.');
}
final YamlMap map = yamlContents;
bool valid = map.containsKey(_kMergedFilesKey) &&
map.containsKey(_kConflictFilesKey) &&
map.containsKey(_kAddedFilesKey) &&
map.containsKey(_kDeletedFilesKey);
if (!valid) {
throw Exception(
'Invalid .migrate_manifest file in the migrate working directory. File is missing an entry.');
}
final Object? mergedFilesYaml = map[_kMergedFilesKey];
final Object? conflictFilesYaml = map[_kConflictFilesKey];
final Object? addedFilesYaml = map[_kAddedFilesKey];
final Object? deletedFilesYaml = map[_kDeletedFilesKey];
valid = valid && (mergedFilesYaml is YamlList || mergedFilesYaml == null);
valid =
valid && (conflictFilesYaml is YamlList || conflictFilesYaml == null);
valid = valid && (addedFilesYaml is YamlList || addedFilesYaml == null);
valid = valid && (deletedFilesYaml is YamlList || deletedFilesYaml == null);
if (!valid) {
throw Exception(
'Invalid .migrate_manifest file in the migrate working directory. Entry is not a Yaml list.');
}
if (mergedFilesYaml != null) {
for (final Object? localPath in mergedFilesYaml as YamlList) {
if (localPath is String) {
// We can fill the maps with partially dummy data as not all properties are used by the manifest.
migrateResult.mergeResults.add(StringMergeResult.explicit(
mergedString: '',
hasConflict: false,
exitCode: 0,
localPath: localPath));
}
}
}
if (conflictFilesYaml != null) {
for (final Object? localPath in conflictFilesYaml as YamlList) {
if (localPath is String) {
migrateResult.mergeResults.add(StringMergeResult.explicit(
mergedString: '',
hasConflict: true,
exitCode: 1,
localPath: localPath));
}
}
}
if (addedFilesYaml != null) {
for (final Object? localPath in addedFilesYaml as YamlList) {
if (localPath is String) {
migrateResult.addedFiles.add(FilePendingMigration(
localPath, migrateRootDir.childFile(localPath)));
}
}
}
if (deletedFilesYaml != null) {
for (final Object? localPath in deletedFilesYaml as YamlList) {
if (localPath is String) {
migrateResult.deletedFiles.add(FilePendingMigration(
localPath, migrateRootDir.childFile(localPath)));
}
}
}
}
final Directory migrateRootDir;
final MigrateResult migrateResult;
/// A list of local paths of files that require conflict resolution.
List<String> get conflictFiles {
final List<String> output = <String>[];
for (final MergeResult result in migrateResult.mergeResults) {
if (result.hasConflict) {
output.add(result.localPath);
}
}
return output;
}
/// A list of local paths of files that require conflict resolution.
List<String> remainingConflictFiles(Directory workingDir) {
final List<String> output = <String>[];
for (final String localPath in conflictFiles) {
if (!_conflictsResolved(
workingDir.childFile(localPath).readAsStringSync())) {
output.add(localPath);
}
}
return output;
}
// A list of local paths of files that had conflicts and are now fully resolved.
List<String> resolvedConflictFiles(Directory workingDir) {
final List<String> output = <String>[];
for (final String localPath in conflictFiles) {
if (_conflictsResolved(
workingDir.childFile(localPath).readAsStringSync())) {
output.add(localPath);
}
}
return output;
}
/// A list of local paths of files that were automatically merged.
List<String> get mergedFiles {
final List<String> output = <String>[];
for (final MergeResult result in migrateResult.mergeResults) {
if (!result.hasConflict) {
output.add(result.localPath);
}
}
return output;
}
/// A list of local paths of files that were newly added.
List<String> get addedFiles {
final List<String> output = <String>[];
for (final FilePendingMigration file in migrateResult.addedFiles) {
output.add(file.localPath);
}
return output;
}
/// A list of local paths of files that are marked for deletion.
List<String> get deletedFiles {
final List<String> output = <String>[];
for (final FilePendingMigration file in migrateResult.deletedFiles) {
output.add(file.localPath);
}
return output;
}
/// Returns the manifest file given a migration workind directory.
static File getManifestFileFromDirectory(Directory workingDir) {
return workingDir.childFile('.migrate_manifest');
}
/// Writes the manifest yaml file in the working directory.
void writeFile() {
final StringBuffer mergedFileManifestContents = StringBuffer();
final StringBuffer conflictFilesManifestContents = StringBuffer();
for (final MergeResult result in migrateResult.mergeResults) {
if (result.hasConflict) {
conflictFilesManifestContents.write(' - ${result.localPath}\n');
} else {
mergedFileManifestContents.write(' - ${result.localPath}\n');
}
}
final StringBuffer newFileManifestContents = StringBuffer();
for (final String localPath in addedFiles) {
newFileManifestContents.write(' - $localPath\n');
}
final StringBuffer deletedFileManifestContents = StringBuffer();
for (final String localPath in deletedFiles) {
deletedFileManifestContents.write(' - $localPath\n');
}
final String migrateManifestContents =
'merged_files:\n${mergedFileManifestContents}conflict_files:\n${conflictFilesManifestContents}added_files:\n${newFileManifestContents}deleted_files:\n$deletedFileManifestContents';
final File migrateManifest = getManifestFileFromDirectory(migrateRootDir);
migrateManifest.createSync(recursive: true);
migrateManifest.writeAsStringSync(migrateManifestContents, flush: true);
}
}
/// Returns true if the file does not contain any git conflict markers.
bool _conflictsResolved(String contents) {
if (contents.contains('>>>>>>>') &&
contents.contains('=======') &&
contents.contains('<<<<<<<')) {
return false;
}
return true;
}
/// Returns true if the migration working directory has all conflicts resolved and prints the migration status.
///
/// The migration status printout lists all added, deleted, merged, and conflicted files.
bool checkAndPrintMigrateStatus(MigrateManifest manifest, Directory workingDir,
{bool warnConflict = false, Logger? logger}) {
final StringBuffer printout = StringBuffer();
final StringBuffer redPrintout = StringBuffer();
bool result = true;
final List<String> remainingConflicts = <String>[];
final List<String> mergedFiles = <String>[];
for (final String localPath in manifest.conflictFiles) {
if (!_conflictsResolved(
workingDir.childFile(localPath).readAsStringSync())) {
remainingConflicts.add(localPath);
} else {
mergedFiles.add(localPath);
}
}
mergedFiles.addAll(manifest.mergedFiles);
if (manifest.addedFiles.isNotEmpty) {
printout.write('Added files:\n');
for (final String localPath in manifest.addedFiles) {
printout.write(' - $localPath\n');
}
}
if (manifest.deletedFiles.isNotEmpty) {
printout.write('Deleted files:\n');
for (final String localPath in manifest.deletedFiles) {
printout.write(' - $localPath\n');
}
}
if (mergedFiles.isNotEmpty) {
printout.write('Modified files:\n');
for (final String localPath in mergedFiles) {
printout.write(' - $localPath\n');
}
}
if (remainingConflicts.isNotEmpty) {
if (warnConflict) {
printout.write(
'Unable to apply migration. The following files in the migration working directory still have unresolved conflicts:');
} else {
printout.write('Merge conflicted files:');
}
for (final String localPath in remainingConflicts) {
redPrintout.write(' - $localPath\n');
}
result = false;
}
if (logger != null) {
logger.printStatus(printout.toString());
logger.printStatus(redPrintout.toString(),
color: TerminalColor.red, newline: false);
}
return result;
}
| packages/packages/flutter_migrate/lib/src/manifest.dart/0 | {
"file_path": "packages/packages/flutter_migrate/lib/src/manifest.dart",
"repo_id": "packages",
"token_count": 3412
} | 1,028 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'package:flutter_migrate/src/base/common.dart';
import 'package:flutter_migrate/src/base/context.dart';
import 'package:flutter_migrate/src/base/file_system.dart';
import 'package:flutter_migrate/src/base/io.dart';
import 'package:flutter_migrate/src/base/logger.dart';
import 'package:flutter_migrate/src/base/signals.dart';
import 'package:flutter_migrate/src/environment.dart';
import 'package:process/process.dart';
import 'src/common.dart';
import 'src/context.dart';
void main() {
late FileSystem fileSystem;
late BufferLogger logger;
late ProcessManager processManager;
late Directory appDir;
late String separator;
setUp(() {
fileSystem = LocalFileSystem.test(signals: LocalSignals.instance);
appDir = fileSystem.systemTempDirectory.createTempSync('apptestdir');
logger = BufferLogger.test();
separator = isWindows ? r'\\' : '/';
processManager = FakeProcessManager('''
{
"FlutterProject.directory": "/Users/test/flutter",
"FlutterProject.metadataFile": "/Users/test/flutter/.metadata",
"FlutterProject.android.exists": false,
"FlutterProject.ios.exists": false,
"FlutterProject.web.exists": false,
"FlutterProject.macos.exists": false,
"FlutterProject.linux.exists": false,
"FlutterProject.windows.exists": false,
"FlutterProject.fuchsia.exists": false,
"FlutterProject.android.isKotlin": false,
"FlutterProject.ios.isSwift": false,
"FlutterProject.isModule": false,
"FlutterProject.isPlugin": false,
"FlutterProject.manifest.appname": "test_app_name",
"FlutterVersion.frameworkRevision": "4e181f012c717777681862e4771af5a941774bb9",
"Platform.operatingSystem": "macos",
"Platform.isAndroid": true,
"Platform.isIOS": false,
"Platform.isWindows": ${isWindows ? 'true' : 'false'},
"Platform.isMacOS": ${isMacOS ? 'true' : 'false'},
"Platform.isFuchsia": false,
"Platform.pathSeparator": "$separator",
"Cache.flutterRoot": "/Users/test/flutter"
}
''');
});
tearDown(() async {
tryToDelete(appDir);
});
testUsingContext('Environment initialization', () async {
final FlutterToolsEnvironment env =
await FlutterToolsEnvironment.initializeFlutterToolsEnvironment(
processManager, logger);
expect(env.getString('invalid key') == null, true);
expect(env.getBool('invalid key') == null, true);
expect(env.getString('FlutterProject.directory'), '/Users/test/flutter');
expect(env.getString('FlutterProject.metadataFile'),
'/Users/test/flutter/.metadata');
expect(env.getBool('FlutterProject.android.exists'), false);
expect(env.getBool('FlutterProject.ios.exists'), false);
expect(env.getBool('FlutterProject.web.exists'), false);
expect(env.getBool('FlutterProject.macos.exists'), false);
expect(env.getBool('FlutterProject.linux.exists'), false);
expect(env.getBool('FlutterProject.windows.exists'), false);
expect(env.getBool('FlutterProject.fuchsia.exists'), false);
expect(env.getBool('FlutterProject.android.isKotlin'), false);
expect(env.getBool('FlutterProject.ios.isSwift'), false);
expect(env.getBool('FlutterProject.isModule'), false);
expect(env.getBool('FlutterProject.isPlugin'), false);
expect(env.getString('FlutterProject.manifest.appname'), 'test_app_name');
expect(env.getString('FlutterVersion.frameworkRevision'),
'4e181f012c717777681862e4771af5a941774bb9');
expect(env.getString('Platform.operatingSystem'), 'macos');
expect(env.getBool('Platform.isAndroid'), true);
expect(env.getBool('Platform.isIOS'), false);
expect(env.getBool('Platform.isWindows'), isWindows);
expect(env.getBool('Platform.isMacOS'), isMacOS);
expect(env.getBool('Platform.isFuchsia'), false);
expect(env.getString('Platform.pathSeparator'), separator);
expect(env.getString('Cache.flutterRoot'), '/Users/test/flutter');
}, overrides: <Type, Generator>{
FileSystem: () => fileSystem,
ProcessManager: () => processManager,
});
}
class FakeProcessManager extends LocalProcessManager {
FakeProcessManager(this.runResult);
final String runResult;
@override
Future<ProcessResult> run(List<Object> command,
{String? workingDirectory,
Map<String, String>? environment,
bool includeParentEnvironment = true,
bool runInShell = false,
covariant Encoding? stdoutEncoding = systemEncoding,
covariant Encoding? stderrEncoding = systemEncoding}) async {
return ProcessResult(0, 0, runResult, '');
}
}
| packages/packages/flutter_migrate/test/environment_test.dart/0 | {
"file_path": "packages/packages/flutter_migrate/test/environment_test.dart",
"repo_id": "packages",
"token_count": 1639
} | 1,029 |
buildFlags:
_pluginToolsConfigGlobalKey:
- "--no-tree-shake-icons"
- "--dart-define=buildmode=testing"
| packages/packages/flutter_plugin_android_lifecycle/example/.pluginToolsConfig.yaml/0 | {
"file_path": "packages/packages/flutter_plugin_android_lifecycle/example/.pluginToolsConfig.yaml",
"repo_id": "packages",
"token_count": 45
} | 1,030 |
You can upgrade an existing app to go_router gradually, by starting with the
home screen and creating a GoRoute for each screen you would like to be
deep-linkable.
# Upgrade an app that uses Navigator
To upgrade an app that is already using the Navigator for routing, start with
a single route for the home screen:
```dart
import 'package:go_router/go_router.dart';
final _router = GoRouter(
routes: [
GoRoute(
path: '/',
builder: (context, state) => const HomeScreen(),
),
],
);
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp.router(
routerConfig: _router,
);
}
}
```
GoRouter leverages the Router API to provide backward compatibility with the
Navigator, so any calls to `Navigator.of(context).push()` or
`Navigator.of(context).pop()` will continue to work, but these destinations
aren't deep-linkable. You can gradually add more routes to the GoRouter
configuration.
# Upgrade an app that uses named routes
An app that uses named routes can be migrated to go_router by changing each
entry in the map to a GoRoute object and changing any calls to
`Navigator.of(context).pushNamed` to `context.go()`.
For example, if you are starting with an app like this:
```dart
MaterialApp(
initialRoute: '/details',
routes: {
'/': (context) => HomeScreen(),
'/details': (context) => DetailsScreen(),
},
);
```
Then the GoRouter configuration would look like this:
```dart
GoRouter(
initialRoute: '/details',
routes: [
GoRoute(
path: '/',
builder: (context, state) => const HomeScreen(),
),
GoRoute(
path: '/details',
builder: (context, state) => const DetailsScreen(),
),
],
);
```
| packages/packages/go_router/doc/upgrading.md/0 | {
"file_path": "packages/packages/go_router/doc/upgrading.md",
"repo_id": "packages",
"token_count": 575
} | 1,031 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
// This scenario demonstrates how to use redirect to handle a asynchronous
// sign-in flow.
//
// The `StreamAuth` is a mock of google_sign_in. This example wraps it with an
// InheritedNotifier, StreamAuthScope, and relies on
// `dependOnInheritedWidgetOfExactType` to create a dependency between the
// notifier and go_router's parsing pipeline. When StreamAuth broadcasts new
// event, the dependency will cause the go_router to reparse the current url
// which will also trigger the redirect.
void main() => runApp(StreamAuthScope(child: App()));
/// The main app.
class App extends StatelessWidget {
/// Creates an [App].
App({super.key});
/// The title of the app.
static const String title = 'GoRouter Example: Redirection';
// add the login info into the tree as app state that can change over time
@override
Widget build(BuildContext context) => MaterialApp.router(
routerConfig: _router,
title: title,
debugShowCheckedModeBanner: false,
);
late final GoRouter _router = GoRouter(
routes: <GoRoute>[
GoRoute(
path: '/',
builder: (BuildContext context, GoRouterState state) =>
const HomeScreen(),
),
GoRoute(
path: '/login',
builder: (BuildContext context, GoRouterState state) =>
const LoginScreen(),
),
],
// redirect to the login page if the user is not logged in
redirect: (BuildContext context, GoRouterState state) async {
// Using `of` method creates a dependency of StreamAuthScope. It will
// cause go_router to reparse current route if StreamAuth has new sign-in
// information.
final bool loggedIn = await StreamAuthScope.of(context).isSignedIn();
final bool loggingIn = state.matchedLocation == '/login';
if (!loggedIn) {
return '/login';
}
// if the user is logged in but still on the login page, send them to
// the home page
if (loggingIn) {
return '/';
}
// no need to redirect at all
return null;
},
);
}
/// The login screen.
class LoginScreen extends StatefulWidget {
/// Creates a [LoginScreen].
const LoginScreen({super.key});
@override
State<LoginScreen> createState() => _LoginScreenState();
}
class _LoginScreenState extends State<LoginScreen>
with TickerProviderStateMixin {
bool loggingIn = false;
late final AnimationController controller;
@override
void initState() {
super.initState();
controller = AnimationController(
vsync: this,
duration: const Duration(seconds: 1),
)..addListener(() {
setState(() {});
});
controller.repeat();
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(title: const Text(App.title)),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
if (loggingIn) CircularProgressIndicator(value: controller.value),
if (!loggingIn)
ElevatedButton(
onPressed: () {
StreamAuthScope.of(context).signIn('test-user');
setState(() {
loggingIn = true;
});
},
child: const Text('Login'),
),
],
),
),
);
}
/// The home screen.
class HomeScreen extends StatelessWidget {
/// Creates a [HomeScreen].
const HomeScreen({super.key});
@override
Widget build(BuildContext context) {
final StreamAuth info = StreamAuthScope.of(context);
return Scaffold(
appBar: AppBar(
title: const Text(App.title),
actions: <Widget>[
IconButton(
onPressed: () => info.signOut(),
tooltip: 'Logout: ${info.currentUser}',
icon: const Icon(Icons.logout),
)
],
),
body: const Center(
child: Text('HomeScreen'),
),
);
}
}
/// A scope that provides [StreamAuth] for the subtree.
class StreamAuthScope extends InheritedNotifier<StreamAuthNotifier> {
/// Creates a [StreamAuthScope] sign in scope.
StreamAuthScope({
super.key,
required super.child,
}) : super(
notifier: StreamAuthNotifier(),
);
/// Gets the [StreamAuth].
static StreamAuth of(BuildContext context) {
return context
.dependOnInheritedWidgetOfExactType<StreamAuthScope>()!
.notifier!
.streamAuth;
}
}
/// A class that converts [StreamAuth] into a [ChangeNotifier].
class StreamAuthNotifier extends ChangeNotifier {
/// Creates a [StreamAuthNotifier].
StreamAuthNotifier() : streamAuth = StreamAuth() {
streamAuth.onCurrentUserChanged.listen((String? string) {
notifyListeners();
});
}
/// The stream auth client.
final StreamAuth streamAuth;
}
/// An asynchronous log in services mock with stream similar to google_sign_in.
///
/// This class adds an artificial delay of 3 second when logging in an user, and
/// will automatically clear the login session after [refreshInterval].
class StreamAuth {
/// Creates an [StreamAuth] that clear the current user session in
/// [refeshInterval] second.
StreamAuth({this.refreshInterval = 20})
: _userStreamController = StreamController<String?>.broadcast() {
_userStreamController.stream.listen((String? currentUser) {
_currentUser = currentUser;
});
}
/// The current user.
String? get currentUser => _currentUser;
String? _currentUser;
/// Checks whether current user is signed in with an artificial delay to mimic
/// async operation.
Future<bool> isSignedIn() async {
await Future<void>.delayed(const Duration(seconds: 1));
return _currentUser != null;
}
/// A stream that notifies when current user has changed.
Stream<String?> get onCurrentUserChanged => _userStreamController.stream;
final StreamController<String?> _userStreamController;
/// The interval that automatically signs out the user.
final int refreshInterval;
Timer? _timer;
Timer _createRefreshTimer() {
return Timer(Duration(seconds: refreshInterval), () {
_userStreamController.add(null);
_timer = null;
});
}
/// Signs in a user with an artificial delay to mimic async operation.
Future<void> signIn(String newUserName) async {
await Future<void>.delayed(const Duration(seconds: 3));
_userStreamController.add(newUserName);
_timer?.cancel();
_timer = _createRefreshTimer();
}
/// Signs out the current user.
Future<void> signOut() async {
_timer?.cancel();
_timer = null;
_userStreamController.add(null);
}
}
| packages/packages/go_router/example/lib/async_redirection.dart/0 | {
"file_path": "packages/packages/go_router/example/lib/async_redirection.dart",
"repo_id": "packages",
"token_count": 2574
} | 1,032 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
/// This sample app shows how to use `GoRouter.onException` to redirect on
/// exception.
///
/// The first route '/' is mapped to [HomeScreen], and the second route
/// '/404' is mapped to [NotFoundScreen].
///
/// Any other unknown route or exception is redirected to `/404`.
void main() => runApp(const MyApp());
/// The route configuration.
final GoRouter _router = GoRouter(
onException: (_, GoRouterState state, GoRouter router) {
router.go('/404', extra: state.uri.toString());
},
routes: <RouteBase>[
GoRoute(
path: '/',
builder: (BuildContext context, GoRouterState state) {
return const HomeScreen();
},
),
GoRoute(
path: '/404',
builder: (BuildContext context, GoRouterState state) {
return NotFoundScreen(uri: state.extra as String? ?? '');
},
),
],
);
/// The main app.
class MyApp extends StatelessWidget {
/// Constructs a [MyApp]
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp.router(
routerConfig: _router,
);
}
}
/// The home screen
class HomeScreen extends StatelessWidget {
/// Constructs a [HomeScreen]
const HomeScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Home Screen')),
body: Center(
child: ElevatedButton(
onPressed: () => context.go('/some-unknown-route'),
child: const Text('Simulates user entering unknown url'),
),
),
);
}
}
/// The not found screen
class NotFoundScreen extends StatelessWidget {
/// Constructs a [HomeScreen]
const NotFoundScreen({super.key, required this.uri});
/// The uri that can not be found.
final String uri;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Page Not Found')),
body: Center(
child: Text("Can't find a page for: $uri"),
),
);
}
}
| packages/packages/go_router/example/lib/exception_handling.dart/0 | {
"file_path": "packages/packages/go_router/example/lib/exception_handling.dart",
"repo_id": "packages",
"token_count": 795
} | 1,033 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
// This scenario demonstrates the behavior when pushing ShellRoute in various
// scenario.
//
// This example have three routes, /shell1, /shell2, and /regular-route. The
// /shell1 and /shell2 are nested in different ShellRoutes. The /regular-route
// is a simple GoRoute.
void main() {
runApp(PushWithShellRouteExampleApp());
}
/// An example demonstrating how to use [ShellRoute]
class PushWithShellRouteExampleApp extends StatelessWidget {
/// Creates a [PushWithShellRouteExampleApp]
PushWithShellRouteExampleApp({super.key});
final GoRouter _router = GoRouter(
initialLocation: '/home',
debugLogDiagnostics: true,
routes: <RouteBase>[
ShellRoute(
builder: (BuildContext context, GoRouterState state, Widget child) {
return ScaffoldForShell1(child: child);
},
routes: <RouteBase>[
GoRoute(
path: '/home',
builder: (BuildContext context, GoRouterState state) {
return const Home();
},
),
GoRoute(
path: '/shell1',
pageBuilder: (_, __) => const NoTransitionPage<void>(
child: Center(
child: Text('shell1 body'),
),
),
),
],
),
ShellRoute(
builder: (BuildContext context, GoRouterState state, Widget child) {
return ScaffoldForShell2(child: child);
},
routes: <RouteBase>[
GoRoute(
path: '/shell2',
builder: (BuildContext context, GoRouterState state) {
return const Center(child: Text('shell2 body'));
},
),
],
),
GoRoute(
path: '/regular-route',
builder: (BuildContext context, GoRouterState state) {
return const Scaffold(
body: Center(child: Text('regular route')),
);
},
),
],
);
@override
Widget build(BuildContext context) {
return MaterialApp.router(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
routerConfig: _router,
);
}
}
/// Builds the "shell" for /shell1
class ScaffoldForShell1 extends StatelessWidget {
/// Constructs an [ScaffoldForShell1].
const ScaffoldForShell1({
required this.child,
super.key,
});
/// The widget to display in the body of the Scaffold.
/// In this sample, it is a Navigator.
final Widget child;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('shell1')),
body: child,
);
}
}
/// Builds the "shell" for /shell1
class ScaffoldForShell2 extends StatelessWidget {
/// Constructs an [ScaffoldForShell1].
const ScaffoldForShell2({
required this.child,
super.key,
});
/// The widget to display in the body of the Scaffold.
/// In this sample, it is a Navigator.
final Widget child;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('shell2')),
body: child,
);
}
}
/// The screen for /home
class Home extends StatelessWidget {
/// Constructs a [Home] widget.
const Home({super.key});
@override
Widget build(BuildContext context) {
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
TextButton(
onPressed: () {
GoRouter.of(context).push('/shell1');
},
child: const Text('push the same shell route /shell1'),
),
TextButton(
onPressed: () {
GoRouter.of(context).push('/shell2');
},
child: const Text('push the different shell route /shell2'),
),
TextButton(
onPressed: () {
GoRouter.of(context).push('/regular-route');
},
child: const Text('push the regular route /regular-route'),
),
],
),
);
}
}
| packages/packages/go_router/example/lib/push_with_shell_route.dart/0 | {
"file_path": "packages/packages/go_router/example/lib/push_with_shell_route.dart",
"repo_id": "packages",
"token_count": 1822
} | 1,034 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: diagnostic_describe_all_properties
import 'package:flutter/material.dart';
import '../misc/extensions.dart';
/// Checks for MaterialApp in the widget tree.
bool isMaterialApp(BuildContext context) =>
context.findAncestorWidgetOfExactType<MaterialApp>() != null;
/// Creates a Material HeroController.
HeroController createMaterialHeroController() =>
MaterialApp.createMaterialHeroController();
/// Builds a Material page.
MaterialPage<void> pageBuilderForMaterialApp({
required LocalKey key,
required String? name,
required Object? arguments,
required String restorationId,
required Widget child,
}) =>
MaterialPage<void>(
name: name,
arguments: arguments,
key: key,
restorationId: restorationId,
child: child,
);
/// Default error page implementation for Material.
class MaterialErrorScreen extends StatelessWidget {
/// Provide an exception to this page for it to be displayed.
const MaterialErrorScreen(this.error, {super.key});
/// The exception to be displayed.
final Exception? error;
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(title: const Text('Page Not Found')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SelectableText(error?.toString() ?? 'page not found'),
TextButton(
onPressed: () => context.go('/'),
child: const Text('Home'),
),
],
),
),
);
}
| packages/packages/go_router/lib/src/pages/material.dart/0 | {
"file_path": "packages/packages/go_router/lib/src/pages/material.dart",
"repo_id": "packages",
"token_count": 622
} | 1,035 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router/go_router.dart';
void main() {
group('replaceNamed', () {
Future<GoRouter> createGoRouter(
WidgetTester tester, {
Listenable? refreshListenable,
}) async {
final GoRouter router = GoRouter(
initialLocation: '/',
routes: <GoRoute>[
GoRoute(
path: '/',
name: 'home',
builder: (_, __) => const _MyWidget(),
),
GoRoute(
path: '/page-0/:tab',
name: 'page-0',
builder: (_, __) => const SizedBox())
],
);
addTearDown(router.dispose);
await tester.pumpWidget(MaterialApp.router(
routerConfig: router,
));
return router;
}
testWidgets('Passes GoRouter parameters through context call.',
(WidgetTester tester) async {
final GoRouter router = await createGoRouter(tester);
await tester.tap(find.text('Settings'));
await tester.pumpAndSettle();
final ImperativeRouteMatch routeMatch = router
.routerDelegate.currentConfiguration.last as ImperativeRouteMatch;
expect(routeMatch.matches.uri.toString(),
'/page-0/settings?search=notification');
});
});
}
class _MyWidget extends StatelessWidget {
const _MyWidget();
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: () => context.replaceNamed('page-0',
pathParameters: <String, String>{'tab': 'settings'},
queryParameters: <String, String>{'search': 'notification'}),
child: const Text('Settings'));
}
}
| packages/packages/go_router/test/extension_test.dart/0 | {
"file_path": "packages/packages/go_router/test/extension_test.dart",
"repo_id": "packages",
"token_count": 782
} | 1,036 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router/src/path_utils.dart';
void main() {
test('patternToRegExp without path parameter', () async {
const String pattern = '/settings/detail';
final List<String> pathParameter = <String>[];
final RegExp regex = patternToRegExp(pattern, pathParameter);
expect(pathParameter.isEmpty, isTrue);
expect(regex.hasMatch('/settings/detail'), isTrue);
expect(regex.hasMatch('/settings/'), isFalse);
expect(regex.hasMatch('/settings'), isFalse);
expect(regex.hasMatch('/'), isFalse);
expect(regex.hasMatch('/settings/details'), isFalse);
expect(regex.hasMatch('/setting/detail'), isFalse);
});
test('patternToRegExp with path parameter', () async {
const String pattern = '/user/:id/book/:bookId';
final List<String> pathParameter = <String>[];
final RegExp regex = patternToRegExp(pattern, pathParameter);
expect(pathParameter.length, 2);
expect(pathParameter[0], 'id');
expect(pathParameter[1], 'bookId');
final RegExpMatch? match = regex.firstMatch('/user/123/book/456/');
expect(match, isNotNull);
final Map<String, String> parameterValues =
extractPathParameters(pathParameter, match!);
expect(parameterValues.length, 2);
expect(parameterValues[pathParameter[0]], '123');
expect(parameterValues[pathParameter[1]], '456');
expect(regex.hasMatch('/user/123/book/'), isFalse);
expect(regex.hasMatch('/user/123'), isFalse);
expect(regex.hasMatch('/user/'), isFalse);
expect(regex.hasMatch('/'), isFalse);
});
test('patternToPath without path parameter', () async {
const String pattern = '/settings/detail';
final List<String> pathParameter = <String>[];
final RegExp regex = patternToRegExp(pattern, pathParameter);
const String url = '/settings/detail';
final RegExpMatch? match = regex.firstMatch(url);
expect(match, isNotNull);
final Map<String, String> parameterValues =
extractPathParameters(pathParameter, match!);
final String restoredUrl = patternToPath(pattern, parameterValues);
expect(url, restoredUrl);
});
test('patternToPath with path parameter', () async {
const String pattern = '/user/:id/book/:bookId';
final List<String> pathParameter = <String>[];
final RegExp regex = patternToRegExp(pattern, pathParameter);
const String url = '/user/123/book/456';
final RegExpMatch? match = regex.firstMatch(url);
expect(match, isNotNull);
final Map<String, String> parameterValues =
extractPathParameters(pathParameter, match!);
final String restoredUrl = patternToPath(pattern, parameterValues);
expect(url, restoredUrl);
});
test('concatenatePaths', () {
void verify(String pathA, String pathB, String expected) {
final String result = concatenatePaths(pathA, pathB);
expect(result, expected);
}
void verifyThrows(String pathA, String pathB) {
expect(
() => concatenatePaths(pathA, pathB), throwsA(isA<AssertionError>()));
}
verify('/a', 'b/c', '/a/b/c');
verify('/', 'b', '/b');
verifyThrows('/a', '/b');
verifyThrows('/a', '/');
verifyThrows('/', '/');
verifyThrows('/', '');
verifyThrows('', '');
});
test('canonicalUri', () {
void verify(String path, String expected) =>
expect(canonicalUri(path), expected);
verify('/a', '/a');
verify('/a/', '/a');
verify('/', '/');
verify('/a/b/', '/a/b');
expect(() => canonicalUri('::::'), throwsA(isA<FormatException>()));
expect(() => canonicalUri(''), throwsA(anything));
});
}
| packages/packages/go_router/test/path_utils_test.dart/0 | {
"file_path": "packages/packages/go_router/test/path_utils_test.dart",
"repo_id": "packages",
"token_count": 1329
} | 1,037 |
# Read about `build.yaml` at https://pub.dev/packages/build_config
builders:
go_router_builder:
import: "package:go_router_builder/go_router_builder.dart"
builder_factories: ["goRouterBuilder"]
build_extensions: {".dart": ["go_router.g.part"]}
auto_apply: dependents
build_to: cache
applies_builders: ["source_gen|combining_builder"]
| packages/packages/go_router_builder/build.yaml/0 | {
"file_path": "packages/packages/go_router_builder/build.yaml",
"repo_id": "packages",
"token_count": 137
} | 1,038 |
// GENERATED CODE - DO NOT MODIFY BY HAND
// ignore_for_file: always_specify_types, public_member_api_docs
part of 'shell_route_with_keys_example.dart';
// **************************************************************************
// GoRouterGenerator
// **************************************************************************
List<RouteBase> get $appRoutes => [
$myShellRouteData,
];
RouteBase get $myShellRouteData => ShellRouteData.$route(
navigatorKey: MyShellRouteData.$navigatorKey,
factory: $MyShellRouteDataExtension._fromState,
routes: [
GoRouteData.$route(
path: '/home',
factory: $HomeRouteDataExtension._fromState,
),
GoRouteData.$route(
path: '/users',
factory: $UsersRouteDataExtension._fromState,
routes: [
GoRouteData.$route(
path: ':id',
parentNavigatorKey: UserRouteData.$parentNavigatorKey,
factory: $UserRouteDataExtension._fromState,
),
],
),
],
);
extension $MyShellRouteDataExtension on MyShellRouteData {
static MyShellRouteData _fromState(GoRouterState state) =>
const MyShellRouteData();
}
extension $HomeRouteDataExtension on HomeRouteData {
static HomeRouteData _fromState(GoRouterState state) => const HomeRouteData();
String get location => GoRouteData.$location(
'/home',
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
extension $UsersRouteDataExtension on UsersRouteData {
static UsersRouteData _fromState(GoRouterState state) =>
const UsersRouteData();
String get location => GoRouteData.$location(
'/users',
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
extension $UserRouteDataExtension on UserRouteData {
static UserRouteData _fromState(GoRouterState state) => UserRouteData(
id: int.parse(state.pathParameters['id']!),
);
String get location => GoRouteData.$location(
'/users/${Uri.encodeComponent(id.toString())}',
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
| packages/packages/go_router_builder/example/lib/shell_route_with_keys_example.g.dart/0 | {
"file_path": "packages/packages/go_router_builder/example/lib/shell_route_with_keys_example.g.dart",
"repo_id": "packages",
"token_count": 984
} | 1,039 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router_builder_example/stateful_shell_route_example.dart';
void main() {
testWidgets('Navigate between section A and section B',
(WidgetTester tester) async {
await tester.pumpWidget(App());
expect(find.text('Details for A - Counter: 0'), findsOneWidget);
await tester.tap(find.text('Increment counter'));
await tester.pumpAndSettle();
expect(find.text('Details for A - Counter: 1'), findsOneWidget);
await tester.tap(find.text('Section B'));
await tester.pumpAndSettle();
expect(find.text('Details for B - Counter: 0'), findsOneWidget);
await tester.tap(find.text('Section A'));
await tester.pumpAndSettle();
expect(find.text('Details for A - Counter: 1'), findsOneWidget);
});
}
| packages/packages/go_router_builder/example/test/stateful_shell_route_test.dart/0 | {
"file_path": "packages/packages/go_router_builder/example/test/stateful_shell_route_test.dart",
"repo_id": "packages",
"token_count": 327
} | 1,040 |
RouteBase get $extraValueRoute => GoRouteData.$route(
path: '/default-value-route',
factory: $ExtraValueRouteExtension._fromState,
);
extension $ExtraValueRouteExtension on ExtraValueRoute {
static ExtraValueRoute _fromState(GoRouterState state) => ExtraValueRoute(
param:
_$convertMapValue('param', state.uri.queryParameters, int.parse) ??
0,
$extra: state.extra as int?,
);
String get location => GoRouteData.$location(
'/default-value-route',
queryParams: {
if (param != 0) 'param': param.toString(),
},
);
void go(BuildContext context) => context.go(location, extra: $extra);
Future<T?> push<T>(BuildContext context) =>
context.push<T>(location, extra: $extra);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location, extra: $extra);
void replace(BuildContext context) =>
context.replace(location, extra: $extra);
}
T? _$convertMapValue<T>(
String key,
Map<String, String> map,
T Function(String) converter,
) {
final value = map[key];
return value == null ? null : converter(value);
}
| packages/packages/go_router_builder/test_inputs/extra_value.dart.expect/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/extra_value.dart.expect",
"repo_id": "packages",
"token_count": 434
} | 1,041 |
RouteBase get $requiredNullableTypeArgumentsExtraValueRoute =>
GoRouteData.$route(
path: '/default-value-route',
factory:
$RequiredNullableTypeArgumentsExtraValueRouteExtension._fromState,
);
extension $RequiredNullableTypeArgumentsExtraValueRouteExtension
on RequiredNullableTypeArgumentsExtraValueRoute {
static RequiredNullableTypeArgumentsExtraValueRoute _fromState(
GoRouterState state) =>
RequiredNullableTypeArgumentsExtraValueRoute(
$extra: state.extra as List<int?>,
);
String get location => GoRouteData.$location(
'/default-value-route',
);
void go(BuildContext context) => context.go(location, extra: $extra);
Future<T?> push<T>(BuildContext context) =>
context.push<T>(location, extra: $extra);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location, extra: $extra);
void replace(BuildContext context) =>
context.replace(location, extra: $extra);
}
| packages/packages/go_router_builder/test_inputs/required_nullable_type_arguments_extra_value.dart.expect/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/required_nullable_type_arguments_extra_value.dart.expect",
"repo_id": "packages",
"token_count": 336
} | 1,042 |
The @TypedGoRoute annotation can only be applied to classes that extend or implement `GoRouteData`.
| packages/packages/go_router_builder/test_inputs/wrong_class_type.dart.expect/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/wrong_class_type.dart.expect",
"repo_id": "packages",
"token_count": 23
} | 1,043 |
name: google_identity_services_web_example
description: An example for the google_identity_services_web package, OneTap.
publish_to: 'none'
environment:
sdk: ^3.3.0
flutter: ">=3.19.0"
dependencies:
flutter:
sdk: flutter
google_identity_services_web:
path: ../
http: ">=0.13.0 <2.0.0"
web: ^0.5.1
dev_dependencies:
build_runner: ^2.1.10 # To extract README excerpts only.
flutter_test:
sdk: flutter
integration_test:
sdk: flutter
flutter:
uses-material-design: true
| packages/packages/google_identity_services_web/example/pubspec.yaml/0 | {
"file_path": "packages/packages/google_identity_services_web/example/pubspec.yaml",
"repo_id": "packages",
"token_count": 210
} | 1,044 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Authorization. API reference:
// https://developers.google.com/identity/oauth2/web/reference/js-reference
// ignore_for_file: non_constant_identifier_names
// * non_constant_identifier_names required to be able to use the same parameter
// names as the underlying library.
@JS()
library google_accounts_oauth2;
import 'dart:js_interop';
import 'shared.dart';
/// Binding to the `google.accounts.oauth2` JS global.
///
/// See: https://developers.google.com/identity/oauth2/web/reference/js-reference
@JS('google.accounts.oauth2')
external GoogleAccountsOauth2 get oauth2;
/// The Dart definition of the `google.accounts.oauth2` global.
@JS()
@staticInterop
abstract class GoogleAccountsOauth2 {}
/// The `google.accounts.oauth2` methods
extension GoogleAccountsOauth2Extension on GoogleAccountsOauth2 {
/// Initializes and returns a code client, with the passed-in [config].
///
/// Method: google.accounts.oauth2.initCodeClient
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#google.accounts.oauth2.initCodeClient
external CodeClient initCodeClient(CodeClientConfig config);
/// Initializes and returns a token client, with the passed-in [config].
///
/// Method: google.accounts.oauth2.initTokenClient
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#google.accounts.oauth2.initTokenClient
external TokenClient initTokenClient(TokenClientConfig config);
// Method: google.accounts.oauth2.hasGrantedAllScopes
// https://developers.google.com/identity/oauth2/web/reference/js-reference#google.accounts.oauth2.hasGrantedAllScopes
@JS('hasGrantedAllScopes')
external bool _hasGrantedScope(TokenResponse token, JSString scope);
/// Checks if hte user has granted **all** the specified [scopes].
///
/// [scopes] is a space-separated list of scope names.
///
/// Method: google.accounts.oauth2.hasGrantedAllScopes
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#google.accounts.oauth2.hasGrantedAllScopes
bool hasGrantedAllScopes(TokenResponse tokenResponse, List<String> scopes) {
return scopes
.every((String scope) => _hasGrantedScope(tokenResponse, scope.toJS));
}
/// Checks if hte user has granted **all** the specified [scopes].
///
/// [scopes] is a space-separated list of scope names.
///
/// Method: google.accounts.oauth2.hasGrantedAllScopes
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#google.accounts.oauth2.hasGrantedAllScopes
bool hasGrantedAnyScopes(TokenResponse tokenResponse, List<String> scopes) {
return scopes
.any((String scope) => _hasGrantedScope(tokenResponse, scope.toJS));
}
/// Revokes all of the scopes that the user granted to the app.
///
/// A valid [accessToken] is required to revoke permissions.
///
/// The [done] callback is called once the revoke action is done. It must be
/// manually wrapped in [allowInterop] before being passed to this method.
///
/// Method: google.accounts.oauth2.revoke
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#google.accounts.oauth2.revoke
void revoke(
String accessToken, [
RevokeTokenDoneFn? done,
]) {
if (done == null) {
return _revoke(accessToken.toJS);
}
return _revokeWithDone(accessToken.toJS, done.toJS);
}
@JS('revoke')
external void _revoke(JSString accessToken);
@JS('revoke')
external void _revokeWithDone(JSString accessToken, JSFunction done);
}
/// The configuration object for the [initCodeClient] method.
///
/// Data type: CodeClientConfig
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#CodeClientConfig
@JS()
@anonymous
@staticInterop
abstract class CodeClientConfig {
/// Constructs a CodeClientConfig object in JavaScript.
///
/// The [callback] property must be wrapped in [allowInterop] before it's
/// passed to this constructor.
factory CodeClientConfig({
required String client_id,
required List<String> scope,
bool? include_granted_scopes,
Uri? redirect_uri,
CodeClientCallbackFn? callback,
String? state,
bool? enable_granular_consent,
@Deprecated('Use `enable_granular_consent` instead.')
bool? enable_serial_consent,
String? login_hint,
String? hd,
UxMode? ux_mode,
bool? select_account,
ErrorCallbackFn? error_callback,
}) {
assert(scope.isNotEmpty);
return CodeClientConfig._toJS(
client_id: client_id.toJS,
scope: scope.join(' ').toJS,
include_granted_scopes: include_granted_scopes?.toJS,
redirect_uri: redirect_uri?.toString().toJS,
callback: callback?.toJS,
state: state?.toJS,
enable_granular_consent: enable_granular_consent?.toJS,
enable_serial_consent: enable_serial_consent?.toJS,
login_hint: login_hint?.toJS,
hd: hd?.toJS,
ux_mode: ux_mode.toString().toJS,
select_account: select_account?.toJS,
error_callback: error_callback?.toJS,
);
}
external factory CodeClientConfig._toJS({
JSString? client_id,
JSString? scope,
JSBoolean? include_granted_scopes,
JSString? redirect_uri,
JSFunction? callback,
JSString? state,
JSBoolean? enable_granular_consent,
JSBoolean? enable_serial_consent,
JSString? login_hint,
JSString? hd,
JSString? ux_mode,
JSBoolean? select_account,
JSFunction? error_callback,
});
}
/// A client that can start the OAuth 2.0 Code UX flow.
///
/// See: https://developers.google.com/identity/oauth2/web/guides/use-code-model
///
/// Data type: CodeClient
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#CodeClient
@JS()
@staticInterop
abstract class CodeClient {}
/// The methods available on the [CodeClient].
extension CodeClientExtension on CodeClient {
/// Starts the OAuth 2.0 Code UX flow.
external void requestCode();
}
/// The object passed as the parameter of your [CodeClientCallbackFn].
///
/// Data type: CodeResponse
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#CodeResponse
@JS()
@staticInterop
abstract class CodeResponse {}
/// The fields that are contained in the code response object.
extension CodeResponseExtension on CodeResponse {
/// The authorization code of a successful token response.
String? get code => _code?.toDart;
@JS('code')
external JSString? get _code;
/// A list of scopes that are approved by the user.
List<String> get scope => _scope?.toDart.split(' ') ?? List<String>.empty();
@JS('scope')
external JSString? get _scope;
/// The string value that your application uses to maintain state between your
/// authorization request and the response.
String? get state => _state?.toDart;
@JS('state')
external JSString? get _state;
/// A single ASCII error code.
String? get error => _error?.toDart;
@JS('error')
external JSString? get _error;
/// Human-readable ASCII text providing additional information, used to assist
/// the client developer in understanding the error that occurred.
String? get error_description => _error_description?.toDart;
@JS('error_description')
external JSString? get _error_description;
/// A URI identifying a human-readable web page with information about the
/// error, used to provide the client developer with additional information
/// about the error.
String? get error_uri => _error_uri?.toDart;
@JS('error_uri')
external JSString? get _error_uri;
}
/// The type of the `callback` function passed to [CodeClientConfig].
typedef CodeClientCallbackFn = void Function(CodeResponse response);
/// The configuration object for the [initTokenClient] method.
///
/// Data type: TokenClientConfig
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#TokenClientConfig
@JS()
@anonymous
@staticInterop
abstract class TokenClientConfig {
/// Constructs a TokenClientConfig object in JavaScript.
///
/// The [callback] property must be wrapped in [allowInterop] before it's
/// passed to this constructor.
factory TokenClientConfig({
required String client_id,
required TokenClientCallbackFn callback,
required List<String> scope,
bool? include_granted_scopes,
String? prompt,
bool? enable_granular_consent,
@Deprecated('Use `enable_granular_consent` instead.')
bool? enable_serial_consent,
String? login_hint,
String? hd,
String? state,
ErrorCallbackFn? error_callback,
}) {
assert(scope.isNotEmpty);
return TokenClientConfig._toJS(
client_id: client_id.toJS,
callback: callback.toJS,
scope: scope.join(' ').toJS,
include_granted_scopes: include_granted_scopes?.toJS,
prompt: prompt?.toJS,
enable_granular_consent: enable_granular_consent?.toJS,
enable_serial_consent: enable_serial_consent?.toJS,
login_hint: login_hint?.toJS,
hd: hd?.toJS,
state: state?.toJS,
error_callback: error_callback?.toJS,
);
}
external factory TokenClientConfig._toJS({
JSString? client_id,
JSFunction? callback,
JSString? scope,
JSBoolean? include_granted_scopes,
JSString? prompt,
JSBoolean? enable_granular_consent,
JSBoolean? enable_serial_consent,
JSString? login_hint,
JSString? hd,
JSString? state,
JSFunction? error_callback,
});
}
/// A client that can start the OAuth 2.0 Token UX flow.
///
/// See: https://developers.google.com/identity/oauth2/web/guides/use-token-model
///
/// Data type: TokenClient
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#TokenClient
@JS()
@staticInterop
abstract class TokenClient {}
/// The methods available on the [TokenClient].
extension TokenClientExtension on TokenClient {
/// Starts the OAuth 2.0 Code UX flow.
void requestAccessToken([
OverridableTokenClientConfig? overrideConfig,
]) {
if (overrideConfig == null) {
return _requestAccessToken();
}
return _requestAccessTokenWithConfig(overrideConfig);
}
@JS('requestAccessToken')
external void _requestAccessToken();
@JS('requestAccessToken')
external void _requestAccessTokenWithConfig(
OverridableTokenClientConfig config);
}
/// The overridable configuration object for the [TokenClientExtension.requestAccessToken] method.
///
/// Data type: OverridableTokenClientConfig
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#OverridableTokenClientConfig
@JS()
@anonymous
@staticInterop
abstract class OverridableTokenClientConfig {
/// Constructs an OverridableTokenClientConfig object in JavaScript.
///
/// The [callback] property must be wrapped in [allowInterop] before it's
/// passed to this constructor.
factory OverridableTokenClientConfig({
/// A list of scopes that identify the resources that your application could
/// access on the user's behalf. These values inform the consent screen that
/// Google displays to the user.
// b/251971390
List<String>? scope,
/// Enables applications to use incremental authorization to request access
/// to additional scopes in context. If you set this parameter's value to
/// `false` and the authorization request is granted, then the new access
/// token will only cover any scopes to which the `scope` requested in this
/// [OverridableTokenClientConfig].
bool? include_granted_scopes,
/// A space-delimited, case-sensitive list of prompts to present the user.
///
/// See `prompt` in [TokenClientConfig].
String? prompt,
/// If set to false, "more granular Google Account permissions" would be
/// disabled for OAuth client IDs created before 2019. If both
/// `enable_granular_consent` and `enable_serial_consent` are set, only
/// `enable_granular_consent` value would take effect and
/// `enable_serial_consent` value would be ignored.
///
/// No effect for newer OAuth client IDs, since more granular permissions is
/// always enabled for them.
bool? enable_granular_consent,
/// This has the same effect as `enable_granular_consent`. Existing
/// applications that use `enable_serial_consent` can continue to do so, but
/// you are encouraged to update your code to use `enable_granular_consent`
/// in your next application update.
///
/// See: https://developers.googleblog.com/2018/10/more-granular-google-account.html
@Deprecated('Use `enable_granular_consent` instead.')
bool? enable_serial_consent,
/// When your app knows which user it is trying to authenticate, it can
/// provide this parameter as a hint to the authentication server. Passing
/// this hint suppresses the account chooser and either pre-fills the email
/// box on the sign-in form, or selects the proper session (if the user is
/// using multiple sign-in), which can help you avoid problems that occur if
/// your app logs in the wrong user account.
///
/// The value can be either an email address or the `sub` string, which is
/// equivalent to the user's Google ID.
///
/// About Multiple Sign-in: https://support.google.com/accounts/answer/1721977
String? login_hint,
/// **Not recommended.** Specifies any string value that your application
/// uses to maintain state between your authorization request and the
/// authorization server's response.
String? state,
}) {
assert(scope == null || scope.isNotEmpty);
return OverridableTokenClientConfig._toJS(
scope: scope?.join(' ').toJS,
include_granted_scopes: include_granted_scopes?.toJS,
prompt: prompt?.toJS,
enable_granular_consent: enable_granular_consent?.toJS,
enable_serial_consent: enable_serial_consent?.toJS,
login_hint: login_hint?.toJS,
state: state?.toJS,
);
}
external factory OverridableTokenClientConfig._toJS({
JSString? scope,
JSBoolean? include_granted_scopes,
JSString? prompt,
JSBoolean? enable_granular_consent,
JSBoolean? enable_serial_consent,
JSString? login_hint,
JSString? state,
});
}
/// The object passed as the parameter of your [TokenClientCallbackFn].
///
/// Data type: TokenResponse
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#TokenResponse
@JS()
@staticInterop
abstract class TokenResponse {}
/// The fields that are contained in the code response object.
extension TokenResponseExtension on TokenResponse {
/// The access token of a successful token response.
String? get access_token => _access_token?.toDart;
@JS('access_token')
external JSString? get _access_token;
/// The lifetime in seconds of the access token.
int? get expires_in => _expires_in?.toDartInt;
@JS('expires_in')
external JSNumber? get _expires_in;
/// The hosted domain the signed-in user belongs to.
String? get hd => _hd?.toDart;
@JS('hd')
external JSString? get _hd;
/// The prompt value that was used from the possible list of values specified
/// by [TokenClientConfig] or [OverridableTokenClientConfig].
String? get prompt => _prompt?.toDart;
@JS('prompt')
external JSString? get _prompt;
/// The type of the token issued.
String? get token_type => _token_type?.toDart;
@JS('token_type')
external JSString? get _token_type;
/// A list of scopes that are approved by the user.
List<String> get scope => _scope?.toDart.split(' ') ?? List<String>.empty();
@JS('scope')
external JSString? get _scope;
/// The string value that your application uses to maintain state between your
/// authorization request and the response.
String? get state => _state?.toDart;
@JS('state')
external JSString? get _state;
/// A single ASCII error code.
String? get error => _error?.toDart;
@JS('error')
external JSString? get _error;
/// Human-readable ASCII text providing additional information, used to assist
/// the client developer in understanding the error that occurred.
String? get error_description => _error_description?.toDart;
@JS('error_description')
external JSString? get _error_description;
/// A URI identifying a human-readable web page with information about the
/// error, used to provide the client developer with additional information
/// about the error.
String? get error_uri => _error_uri?.toDart;
@JS('error_uri')
external JSString? get _error_uri;
}
/// The type of the `callback` function passed to [TokenClientConfig].
typedef TokenClientCallbackFn = void Function(TokenResponse response);
/// The type of the `error_callback` in both oauth2 initXClient calls.
typedef ErrorCallbackFn = void Function(GoogleIdentityServicesError? error);
/// An error returned by `initTokenClient` or `initDataClient`.
@JS()
@staticInterop
abstract class GoogleIdentityServicesError {}
/// Methods of the GoogleIdentityServicesError object.
extension GoogleIdentityServicesErrorExtension on GoogleIdentityServicesError {
/// The type of error
GoogleIdentityServicesErrorType get type =>
GoogleIdentityServicesErrorType.values.byName(_type.toDart);
@JS('type')
external JSString get _type;
/// A human-readable description of the error `type`.
///
/// (Undocumented)
String? get message => _message?.toDart;
@JS('message')
external JSString? get _message;
}
/// The signature of the `done` function for [revoke].
typedef RevokeTokenDoneFn = void Function(TokenRevocationResponse response);
/// The parameter passed to the `callback` of the [revoke] function.
///
/// Data type: RevocationResponse
/// https://developers.google.com/identity/oauth2/web/reference/js-reference#TokenResponse
@JS()
@staticInterop
abstract class TokenRevocationResponse {}
/// The fields that are contained in the [TokenRevocationResponse] object.
extension TokenRevocationResponseExtension on TokenRevocationResponse {
/// This field is a boolean value set to true if the revoke method call
/// succeeded or false on failure.
bool get successful => _successful.toDart;
@JS('successful')
external JSBoolean get _successful;
/// This field is a string value and contains a detailed error message if the
/// revoke method call failed, it is undefined on success.
String? get error => _error?.toDart;
@JS('error')
external JSString? get _error;
/// The description of the error.
String? get error_description => _error_description?.toDart;
@JS('error_description')
external JSString? get _error_description;
}
| packages/packages/google_identity_services_web/lib/src/js_interop/google_accounts_oauth2.dart/0 | {
"file_path": "packages/packages/google_identity_services_web/lib/src/js_interop/google_accounts_oauth2.dart",
"repo_id": "packages",
"token_count": 5968
} | 1,045 |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>CFBundleDevelopmentRegion</key>
<string>en</string>
<key>CFBundleExecutable</key>
<string>$(EXECUTABLE_NAME)</string>
<key>CFBundleIdentifier</key>
<string>$(PRODUCT_BUNDLE_IDENTIFIER)</string>
<key>CFBundleInfoDictionaryVersion</key>
<string>6.0</string>
<key>CFBundleName</key>
<string>google_maps_flutter_example</string>
<key>CFBundlePackageType</key>
<string>APPL</string>
<key>CFBundleShortVersionString</key>
<string>1.0</string>
<key>CFBundleSignature</key>
<string>????</string>
<key>CFBundleVersion</key>
<string>1</string>
<key>LSRequiresIPhoneOS</key>
<true/>
<key>NSLocationWhenInUseUsageDescription</key>
<string>This app needs your location to test the location feature of the Google Maps plugin.</string>
<key>UILaunchStoryboardName</key>
<string>LaunchScreen</string>
<key>UIMainStoryboardFile</key>
<string>Main</string>
<key>UIRequiredDeviceCapabilities</key>
<array>
<string>arm64</string>
</array>
<key>UISupportedInterfaceOrientations</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
<key>UISupportedInterfaceOrientations~ipad</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationPortraitUpsideDown</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
<key>CADisableMinimumFrameDurationOnPhone</key>
<true/>
<key>UIApplicationSupportsIndirectInputEvents</key>
<true/>
</dict>
</plist>
| packages/packages/google_maps_flutter/google_maps_flutter/example/ios/Runner/Info.plist/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter/example/ios/Runner/Info.plist",
"repo_id": "packages",
"token_count": 674
} | 1,046 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
part of '../google_maps_flutter.dart';
/// Callback method for when the map is ready to be used.
///
/// Pass to [GoogleMap.onMapCreated] to receive a [GoogleMapController] when the
/// map is created.
typedef MapCreatedCallback = void Function(GoogleMapController controller);
// This counter is used to provide a stable "constant" initialization id
// to the buildView function, so the web implementation can use it as a
// cache key. This needs to be provided from the outside, because web
// views seem to re-render much more often that mobile platform views.
int _nextMapCreationId = 0;
/// Error thrown when an unknown map object ID is provided to a method.
class UnknownMapObjectIdError extends Error {
/// Creates an assertion error with the provided [message].
UnknownMapObjectIdError(this.objectType, this.objectId, [this.context]);
/// The name of the map object whose ID is unknown.
final String objectType;
/// The unknown maps object ID.
final MapsObjectId<Object> objectId;
/// The context where the error occurred.
final String? context;
@override
String toString() {
if (context != null) {
return 'Unknown $objectType ID "${objectId.value}" in $context';
}
return 'Unknown $objectType ID "${objectId.value}"';
}
}
/// Android specific settings for [GoogleMap].
@Deprecated(
'See https://pub.dev/packages/google_maps_flutter_android#display-mode')
class AndroidGoogleMapsFlutter {
@Deprecated(
'See https://pub.dev/packages/google_maps_flutter_android#display-mode')
AndroidGoogleMapsFlutter._();
/// Whether to render [GoogleMap] with a [AndroidViewSurface] to build the Google Maps widget.
///
/// This implementation uses hybrid composition to render the Google Maps
/// Widget on Android. This comes at the cost of some performance on Android
/// versions below 10. See
/// https://flutter.dev/docs/development/platform-integration/platform-views#performance for more
/// information.
@Deprecated(
'See https://pub.dev/packages/google_maps_flutter_android#display-mode')
static bool get useAndroidViewSurface {
final GoogleMapsFlutterPlatform platform =
GoogleMapsFlutterPlatform.instance;
if (platform is GoogleMapsFlutterAndroid) {
return platform.useAndroidViewSurface;
}
return false;
}
/// Set whether to render [GoogleMap] with a [AndroidViewSurface] to build the Google Maps widget.
///
/// This implementation uses hybrid composition to render the Google Maps
/// Widget on Android. This comes at the cost of some performance on Android
/// versions below 10. See
/// https://flutter.dev/docs/development/platform-integration/platform-views#performance for more
/// information.
@Deprecated(
'See https://pub.dev/packages/google_maps_flutter_android#display-mode')
static set useAndroidViewSurface(bool useAndroidViewSurface) {
final GoogleMapsFlutterPlatform platform =
GoogleMapsFlutterPlatform.instance;
if (platform is GoogleMapsFlutterAndroid) {
platform.useAndroidViewSurface = useAndroidViewSurface;
}
}
}
/// A widget which displays a map with data obtained from the Google Maps service.
class GoogleMap extends StatefulWidget {
/// Creates a widget displaying data from Google Maps services.
///
/// [AssertionError] will be thrown if [initialCameraPosition] is null;
const GoogleMap({
super.key,
required this.initialCameraPosition,
this.style,
this.onMapCreated,
this.gestureRecognizers = const <Factory<OneSequenceGestureRecognizer>>{},
this.webGestureHandling,
this.compassEnabled = true,
this.mapToolbarEnabled = true,
this.cameraTargetBounds = CameraTargetBounds.unbounded,
this.mapType = MapType.normal,
this.minMaxZoomPreference = MinMaxZoomPreference.unbounded,
this.rotateGesturesEnabled = true,
this.scrollGesturesEnabled = true,
this.zoomControlsEnabled = true,
this.zoomGesturesEnabled = true,
this.liteModeEnabled = false,
this.tiltGesturesEnabled = true,
this.fortyFiveDegreeImageryEnabled = false,
this.myLocationEnabled = false,
this.myLocationButtonEnabled = true,
this.layoutDirection,
/// If no padding is specified default padding will be 0.
this.padding = EdgeInsets.zero,
this.indoorViewEnabled = false,
this.trafficEnabled = false,
this.buildingsEnabled = true,
this.markers = const <Marker>{},
this.polygons = const <Polygon>{},
this.polylines = const <Polyline>{},
this.circles = const <Circle>{},
this.onCameraMoveStarted,
this.tileOverlays = const <TileOverlay>{},
this.onCameraMove,
this.onCameraIdle,
this.onTap,
this.onLongPress,
this.cloudMapId,
});
/// Callback method for when the map is ready to be used.
///
/// Used to receive a [GoogleMapController] for this [GoogleMap].
final MapCreatedCallback? onMapCreated;
/// The initial position of the map's camera.
final CameraPosition initialCameraPosition;
/// The style for the map.
///
/// Set to null to clear any previous custom styling.
///
/// If problems were detected with the [mapStyle], including un-parsable
/// styling JSON, unrecognized feature type, unrecognized element type, or
/// invalid styler keys, the style is left unchanged, and the error can be
/// retrieved with [GoogleMapController.getStyleError].
///
/// The style string can be generated using the
/// [map style tool](https://mapstyle.withgoogle.com/).
final String? style;
/// True if the map should show a compass when rotated.
final bool compassEnabled;
/// True if the map should show a toolbar when you interact with the map. Android only.
final bool mapToolbarEnabled;
/// Geographical bounding box for the camera target.
final CameraTargetBounds cameraTargetBounds;
/// Type of map tiles to be rendered.
final MapType mapType;
/// The layout direction to use for the embedded view.
///
/// If this is null, the ambient [Directionality] is used instead. If there is
/// no ambient [Directionality], [TextDirection.ltr] is used.
final TextDirection? layoutDirection;
/// Preferred bounds for the camera zoom level.
///
/// Actual bounds depend on map data and device.
final MinMaxZoomPreference minMaxZoomPreference;
/// True if the map view should respond to rotate gestures.
final bool rotateGesturesEnabled;
/// True if the map view should respond to scroll gestures.
final bool scrollGesturesEnabled;
/// True if the map view should show zoom controls. This includes two buttons
/// to zoom in and zoom out. The default value is to show zoom controls.
///
/// This is only supported on Android. And this field is silently ignored on iOS.
final bool zoomControlsEnabled;
/// True if the map view should respond to zoom gestures.
final bool zoomGesturesEnabled;
/// True if the map view should be in lite mode. Android only.
///
/// See https://developers.google.com/maps/documentation/android-sdk/lite#overview_of_lite_mode for more details.
final bool liteModeEnabled;
/// True if the map view should respond to tilt gestures.
final bool tiltGesturesEnabled;
/// True if 45 degree imagery should be enabled. Web only.
final bool fortyFiveDegreeImageryEnabled;
/// Padding to be set on map. See https://developers.google.com/maps/documentation/android-sdk/map#map_padding for more details.
final EdgeInsets padding;
/// Markers to be placed on the map.
final Set<Marker> markers;
/// Polygons to be placed on the map.
final Set<Polygon> polygons;
/// Polylines to be placed on the map.
final Set<Polyline> polylines;
/// Circles to be placed on the map.
final Set<Circle> circles;
/// Tile overlays to be placed on the map.
final Set<TileOverlay> tileOverlays;
/// Called when the camera starts moving.
///
/// This can be initiated by the following:
/// 1. Non-gesture animation initiated in response to user actions.
/// For example: zoom buttons, my location button, or marker clicks.
/// 2. Programmatically initiated animation.
/// 3. Camera motion initiated in response to user gestures on the map.
/// For example: pan, tilt, pinch to zoom, or rotate.
final VoidCallback? onCameraMoveStarted;
/// Called repeatedly as the camera continues to move after an
/// onCameraMoveStarted call.
///
/// This may be called as often as once every frame and should
/// not perform expensive operations.
final CameraPositionCallback? onCameraMove;
/// Called when camera movement has ended, there are no pending
/// animations and the user has stopped interacting with the map.
final VoidCallback? onCameraIdle;
/// Called every time a [GoogleMap] is tapped.
final ArgumentCallback<LatLng>? onTap;
/// Called every time a [GoogleMap] is long pressed.
final ArgumentCallback<LatLng>? onLongPress;
/// True if a "My Location" layer should be shown on the map.
///
/// This layer includes a location indicator at the current device location,
/// as well as a My Location button.
/// * The indicator is a small blue dot if the device is stationary, or a
/// chevron if the device is moving.
/// * The My Location button animates to focus on the user's current location
/// if the user's location is currently known.
///
/// Enabling this feature requires adding location permissions to both native
/// platforms of your app.
/// * On Android add either
/// `<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />`
/// or `<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />`
/// to your `AndroidManifest.xml` file. `ACCESS_COARSE_LOCATION` returns a
/// location with an accuracy approximately equivalent to a city block, while
/// `ACCESS_FINE_LOCATION` returns as precise a location as possible, although
/// it consumes more battery power. You will also need to request these
/// permissions during run-time. If they are not granted, the My Location
/// feature will fail silently.
/// * On iOS add a `NSLocationWhenInUseUsageDescription` key to your
/// `Info.plist` file. This will automatically prompt the user for permissions
/// when the map tries to turn on the My Location layer.
final bool myLocationEnabled;
/// Enables or disables the my-location button.
///
/// The my-location button causes the camera to move such that the user's
/// location is in the center of the map. If the button is enabled, it is
/// only shown when the my-location layer is enabled.
///
/// By default, the my-location button is enabled (and hence shown when the
/// my-location layer is enabled).
///
/// See also:
/// * [myLocationEnabled] parameter.
final bool myLocationButtonEnabled;
/// Enables or disables the indoor view from the map
final bool indoorViewEnabled;
/// Enables or disables the traffic layer of the map
final bool trafficEnabled;
/// Enables or disables showing 3D buildings where available
final bool buildingsEnabled;
/// Which gestures should be consumed by the map.
///
/// It is possible for other gesture recognizers to be competing with the map on pointer
/// events, e.g if the map is inside a [ListView] the [ListView] will want to handle
/// vertical drags. The map will claim gestures that are recognized by any of the
/// recognizers on this list.
///
/// When this set is empty, the map will only handle pointer events for gestures that
/// were not claimed by any other gesture recognizer.
final Set<Factory<OneSequenceGestureRecognizer>> gestureRecognizers;
/// This setting controls how the API handles gestures on the map. Web only.
///
/// See [WebGestureHandling] for more details.
final WebGestureHandling? webGestureHandling;
/// Identifier that's associated with a specific cloud-based map style.
///
/// See https://developers.google.com/maps/documentation/get-map-id
/// for more details.
final String? cloudMapId;
/// Creates a [State] for this [GoogleMap].
@override
State createState() => _GoogleMapState();
}
class _GoogleMapState extends State<GoogleMap> {
final int _mapId = _nextMapCreationId++;
final Completer<GoogleMapController> _controller =
Completer<GoogleMapController>();
Map<MarkerId, Marker> _markers = <MarkerId, Marker>{};
Map<PolygonId, Polygon> _polygons = <PolygonId, Polygon>{};
Map<PolylineId, Polyline> _polylines = <PolylineId, Polyline>{};
Map<CircleId, Circle> _circles = <CircleId, Circle>{};
late MapConfiguration _mapConfiguration;
@override
Widget build(BuildContext context) {
return GoogleMapsFlutterPlatform.instance.buildViewWithConfiguration(
_mapId,
onPlatformViewCreated,
widgetConfiguration: MapWidgetConfiguration(
textDirection: widget.layoutDirection ??
Directionality.maybeOf(context) ??
TextDirection.ltr,
initialCameraPosition: widget.initialCameraPosition,
gestureRecognizers: widget.gestureRecognizers,
),
mapObjects: MapObjects(
markers: widget.markers,
polygons: widget.polygons,
polylines: widget.polylines,
circles: widget.circles,
),
mapConfiguration: _mapConfiguration,
);
}
@override
void initState() {
super.initState();
_mapConfiguration = _configurationFromMapWidget(widget);
_markers = keyByMarkerId(widget.markers);
_polygons = keyByPolygonId(widget.polygons);
_polylines = keyByPolylineId(widget.polylines);
_circles = keyByCircleId(widget.circles);
}
@override
void dispose() {
_disposeController();
super.dispose();
}
Future<void> _disposeController() async {
final GoogleMapController controller = await _controller.future;
controller.dispose();
}
@override
void didUpdateWidget(GoogleMap oldWidget) {
super.didUpdateWidget(oldWidget);
_updateOptions();
_updateMarkers();
_updatePolygons();
_updatePolylines();
_updateCircles();
_updateTileOverlays();
}
Future<void> _updateOptions() async {
final MapConfiguration newConfig = _configurationFromMapWidget(widget);
final MapConfiguration updates = newConfig.diffFrom(_mapConfiguration);
if (updates.isEmpty) {
return;
}
final GoogleMapController controller = await _controller.future;
unawaited(controller._updateMapConfiguration(updates));
_mapConfiguration = newConfig;
}
Future<void> _updateMarkers() async {
final GoogleMapController controller = await _controller.future;
unawaited(controller._updateMarkers(
MarkerUpdates.from(_markers.values.toSet(), widget.markers)));
_markers = keyByMarkerId(widget.markers);
}
Future<void> _updatePolygons() async {
final GoogleMapController controller = await _controller.future;
unawaited(controller._updatePolygons(
PolygonUpdates.from(_polygons.values.toSet(), widget.polygons)));
_polygons = keyByPolygonId(widget.polygons);
}
Future<void> _updatePolylines() async {
final GoogleMapController controller = await _controller.future;
unawaited(controller._updatePolylines(
PolylineUpdates.from(_polylines.values.toSet(), widget.polylines)));
_polylines = keyByPolylineId(widget.polylines);
}
Future<void> _updateCircles() async {
final GoogleMapController controller = await _controller.future;
unawaited(controller._updateCircles(
CircleUpdates.from(_circles.values.toSet(), widget.circles)));
_circles = keyByCircleId(widget.circles);
}
Future<void> _updateTileOverlays() async {
final GoogleMapController controller = await _controller.future;
unawaited(controller._updateTileOverlays(widget.tileOverlays));
}
Future<void> onPlatformViewCreated(int id) async {
final GoogleMapController controller = await GoogleMapController.init(
id,
widget.initialCameraPosition,
this,
);
_controller.complete(controller);
unawaited(_updateTileOverlays());
final MapCreatedCallback? onMapCreated = widget.onMapCreated;
if (onMapCreated != null) {
onMapCreated(controller);
}
}
void onMarkerTap(MarkerId markerId) {
final Marker? marker = _markers[markerId];
if (marker == null) {
throw UnknownMapObjectIdError('marker', markerId, 'onTap');
}
final VoidCallback? onTap = marker.onTap;
if (onTap != null) {
onTap();
}
}
void onMarkerDragStart(MarkerId markerId, LatLng position) {
final Marker? marker = _markers[markerId];
if (marker == null) {
throw UnknownMapObjectIdError('marker', markerId, 'onDragStart');
}
final ValueChanged<LatLng>? onDragStart = marker.onDragStart;
if (onDragStart != null) {
onDragStart(position);
}
}
void onMarkerDrag(MarkerId markerId, LatLng position) {
final Marker? marker = _markers[markerId];
if (marker == null) {
throw UnknownMapObjectIdError('marker', markerId, 'onDrag');
}
final ValueChanged<LatLng>? onDrag = marker.onDrag;
if (onDrag != null) {
onDrag(position);
}
}
void onMarkerDragEnd(MarkerId markerId, LatLng position) {
final Marker? marker = _markers[markerId];
if (marker == null) {
throw UnknownMapObjectIdError('marker', markerId, 'onDragEnd');
}
final ValueChanged<LatLng>? onDragEnd = marker.onDragEnd;
if (onDragEnd != null) {
onDragEnd(position);
}
}
void onPolygonTap(PolygonId polygonId) {
final Polygon? polygon = _polygons[polygonId];
if (polygon == null) {
throw UnknownMapObjectIdError('polygon', polygonId, 'onTap');
}
final VoidCallback? onTap = polygon.onTap;
if (onTap != null) {
onTap();
}
}
void onPolylineTap(PolylineId polylineId) {
final Polyline? polyline = _polylines[polylineId];
if (polyline == null) {
throw UnknownMapObjectIdError('polyline', polylineId, 'onTap');
}
final VoidCallback? onTap = polyline.onTap;
if (onTap != null) {
onTap();
}
}
void onCircleTap(CircleId circleId) {
final Circle? circle = _circles[circleId];
if (circle == null) {
throw UnknownMapObjectIdError('marker', circleId, 'onTap');
}
final VoidCallback? onTap = circle.onTap;
if (onTap != null) {
onTap();
}
}
void onInfoWindowTap(MarkerId markerId) {
final Marker? marker = _markers[markerId];
if (marker == null) {
throw UnknownMapObjectIdError('marker', markerId, 'InfoWindow onTap');
}
final VoidCallback? onTap = marker.infoWindow.onTap;
if (onTap != null) {
onTap();
}
}
void onTap(LatLng position) {
final ArgumentCallback<LatLng>? onTap = widget.onTap;
if (onTap != null) {
onTap(position);
}
}
void onLongPress(LatLng position) {
final ArgumentCallback<LatLng>? onLongPress = widget.onLongPress;
if (onLongPress != null) {
onLongPress(position);
}
}
}
/// Builds a [MapConfiguration] from the given [map].
MapConfiguration _configurationFromMapWidget(GoogleMap map) {
assert(!map.liteModeEnabled || Platform.isAndroid);
return MapConfiguration(
webGestureHandling: map.webGestureHandling,
compassEnabled: map.compassEnabled,
mapToolbarEnabled: map.mapToolbarEnabled,
cameraTargetBounds: map.cameraTargetBounds,
mapType: map.mapType,
minMaxZoomPreference: map.minMaxZoomPreference,
rotateGesturesEnabled: map.rotateGesturesEnabled,
scrollGesturesEnabled: map.scrollGesturesEnabled,
tiltGesturesEnabled: map.tiltGesturesEnabled,
fortyFiveDegreeImageryEnabled: map.fortyFiveDegreeImageryEnabled,
trackCameraPosition: map.onCameraMove != null,
zoomControlsEnabled: map.zoomControlsEnabled,
zoomGesturesEnabled: map.zoomGesturesEnabled,
liteModeEnabled: map.liteModeEnabled,
myLocationEnabled: map.myLocationEnabled,
myLocationButtonEnabled: map.myLocationButtonEnabled,
padding: map.padding,
indoorViewEnabled: map.indoorViewEnabled,
trafficEnabled: map.trafficEnabled,
buildingsEnabled: map.buildingsEnabled,
cloudMapId: map.cloudMapId,
// A null style in the widget means no style, which is expressed as '' in
// the configuration to distinguish from no change (null).
style: map.style ?? '',
);
}
| packages/packages/google_maps_flutter/google_maps_flutter/lib/src/google_map.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter/lib/src/google_map.dart",
"repo_id": "packages",
"token_count": 6510
} | 1,047 |
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="io.flutter.plugins.googlemaps">
</manifest>
| packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/AndroidManifest.xml/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/AndroidManifest.xml",
"repo_id": "packages",
"token_count": 45
} | 1,048 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.googlemaps;
import com.google.android.gms.maps.model.BitmapDescriptor;
import com.google.android.gms.maps.model.LatLng;
/** Receiver of Marker configuration options. */
interface MarkerOptionsSink {
void setAlpha(float alpha);
void setAnchor(float u, float v);
void setConsumeTapEvents(boolean consumeTapEvents);
void setDraggable(boolean draggable);
void setFlat(boolean flat);
void setIcon(BitmapDescriptor bitmapDescriptor);
void setInfoWindowAnchor(float u, float v);
void setInfoWindowText(String title, String snippet);
void setPosition(LatLng position);
void setRotation(float rotation);
void setVisible(boolean visible);
void setZIndex(float zIndex);
}
| packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/java/io/flutter/plugins/googlemaps/MarkerOptionsSink.java/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/java/io/flutter/plugins/googlemaps/MarkerOptionsSink.java",
"repo_id": "packages",
"token_count": 269
} | 1,049 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.googlemaps;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.spy;
import com.google.android.gms.internal.maps.zzl;
import com.google.android.gms.maps.model.Circle;
import org.junit.Test;
import org.mockito.Mockito;
public class CircleControllerTest {
@Test
public void controller_SetsStrokeDensity() {
final zzl z = mock(zzl.class);
final Circle circle = spy(new Circle(z));
final float density = 5;
final float strokeWidth = 3;
final CircleController controller = new CircleController(circle, false, density);
controller.setStrokeWidth(strokeWidth);
Mockito.verify(circle).setStrokeWidth(density * strokeWidth);
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/test/java/io/flutter/plugins/googlemaps/CircleControllerTest.java/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/test/java/io/flutter/plugins/googlemaps/CircleControllerTest.java",
"repo_id": "packages",
"token_count": 286
} | 1,050 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.googlemapsexample;
import static org.junit.Assert.assertTrue;
import androidx.test.core.app.ActivityScenario;
import io.flutter.plugins.googlemaps.GoogleMapsPlugin;
import org.junit.Test;
public class GoogleMapsTest {
@Test
public void googleMapsPluginIsAdded() {
final ActivityScenario<GoogleMapsTestActivity> scenario =
ActivityScenario.launch(GoogleMapsTestActivity.class);
scenario.onActivity(
activity -> {
assertTrue(activity.engine.getPlugins().has(GoogleMapsPlugin.class));
});
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_android/example/android/app/src/androidTest/java/io/flutter/plugins/googlemapsexample/GoogleMapsTest.java/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/example/android/app/src/androidTest/java/io/flutter/plugins/googlemapsexample/GoogleMapsTest.java",
"repo_id": "packages",
"token_count": 236
} | 1,051 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: public_member_api_docs
// ignore_for_file: unawaited_futures
import 'package:flutter/material.dart';
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart';
import 'example_google_map.dart';
import 'page.dart';
class MarkerIconsPage extends GoogleMapExampleAppPage {
const MarkerIconsPage({Key? key})
: super(const Icon(Icons.image), 'Marker icons', key: key);
@override
Widget build(BuildContext context) {
return const MarkerIconsBody();
}
}
class MarkerIconsBody extends StatefulWidget {
const MarkerIconsBody({super.key});
@override
State<StatefulWidget> createState() => MarkerIconsBodyState();
}
const LatLng _kMapCenter = LatLng(52.4478, -3.5402);
class MarkerIconsBodyState extends State<MarkerIconsBody> {
ExampleGoogleMapController? controller;
BitmapDescriptor? _markerIcon;
@override
Widget build(BuildContext context) {
_createMarkerImageFromAsset(context);
return Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Center(
child: SizedBox(
width: 350.0,
height: 300.0,
child: ExampleGoogleMap(
initialCameraPosition: const CameraPosition(
target: _kMapCenter,
zoom: 7.0,
),
markers: <Marker>{_createMarker()},
onMapCreated: _onMapCreated,
),
),
)
],
);
}
Marker _createMarker() {
if (_markerIcon != null) {
return Marker(
markerId: const MarkerId('marker_1'),
position: _kMapCenter,
icon: _markerIcon!,
);
} else {
return const Marker(
markerId: MarkerId('marker_1'),
position: _kMapCenter,
);
}
}
Future<void> _createMarkerImageFromAsset(BuildContext context) async {
if (_markerIcon == null) {
final ImageConfiguration imageConfiguration =
createLocalImageConfiguration(context, size: const Size.square(48));
BitmapDescriptor.fromAssetImage(
imageConfiguration, 'assets/red_square.png')
.then(_updateBitmap);
}
}
void _updateBitmap(BitmapDescriptor bitmap) {
setState(() {
_markerIcon = bitmap;
});
}
void _onMapCreated(ExampleGoogleMapController controllerParam) {
setState(() {
controller = controllerParam;
});
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_android/example/lib/marker_icons.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/example/lib/marker_icons.dart",
"repo_id": "packages",
"token_count": 1084
} | 1,052 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:typed_data';
import 'dart:ui' as ui;
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart';
import 'package:integration_test/integration_test.dart';
import 'package:maps_example_dart/example_google_map.dart';
const LatLng _kInitialMapCenter = LatLng(0, 0);
const double _kInitialZoomLevel = 5;
const CameraPosition _kInitialCameraPosition =
CameraPosition(target: _kInitialMapCenter, zoom: _kInitialZoomLevel);
const String _kCloudMapId = '000000000000000'; // Dummy map ID.
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
GoogleMapsFlutterPlatform.instance.enableDebugInspection();
testWidgets('testCompassToggle', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
compassEnabled: false,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
bool compassEnabled = await inspector.isCompassEnabled(mapId: mapId);
expect(compassEnabled, false);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
compassEnabled = await inspector.isCompassEnabled(mapId: mapId);
expect(compassEnabled, true);
});
testWidgets('testMapToolbar returns false', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
final bool mapToolbarEnabled =
await inspector.isMapToolbarEnabled(mapId: mapId);
// This is only supported on Android, so should always return false.
expect(mapToolbarEnabled, false);
});
testWidgets('updateMinMaxZoomLevels', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
const MinMaxZoomPreference initialZoomLevel = MinMaxZoomPreference(4, 8);
const MinMaxZoomPreference finalZoomLevel = MinMaxZoomPreference(6, 10);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
minMaxZoomPreference: initialZoomLevel,
onMapCreated: (ExampleGoogleMapController c) async {
controllerCompleter.complete(c);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
MinMaxZoomPreference zoomLevel =
await inspector.getMinMaxZoomLevels(mapId: controller.mapId);
expect(zoomLevel, equals(initialZoomLevel));
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
minMaxZoomPreference: finalZoomLevel,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
zoomLevel = await inspector.getMinMaxZoomLevels(mapId: controller.mapId);
expect(zoomLevel, equals(finalZoomLevel));
});
testWidgets('testZoomGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
zoomGesturesEnabled: false,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
bool zoomGesturesEnabled =
await inspector.areZoomGesturesEnabled(mapId: mapId);
expect(zoomGesturesEnabled, false);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
zoomGesturesEnabled = await inspector.areZoomGesturesEnabled(mapId: mapId);
expect(zoomGesturesEnabled, true);
});
testWidgets('testZoomControlsEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
final bool zoomControlsEnabled =
await inspector.areZoomControlsEnabled(mapId: mapId);
/// Zoom Controls functionality is not available on iOS at the moment.
expect(zoomControlsEnabled, false);
});
testWidgets('testRotateGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
rotateGesturesEnabled: false,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
bool rotateGesturesEnabled =
await inspector.areRotateGesturesEnabled(mapId: mapId);
expect(rotateGesturesEnabled, false);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
rotateGesturesEnabled =
await inspector.areRotateGesturesEnabled(mapId: mapId);
expect(rotateGesturesEnabled, true);
});
testWidgets('testTiltGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
tiltGesturesEnabled: false,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
bool tiltGesturesEnabled =
await inspector.areTiltGesturesEnabled(mapId: mapId);
expect(tiltGesturesEnabled, false);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
tiltGesturesEnabled = await inspector.areTiltGesturesEnabled(mapId: mapId);
expect(tiltGesturesEnabled, true);
});
testWidgets('testScrollGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
scrollGesturesEnabled: false,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
bool scrollGesturesEnabled =
await inspector.areScrollGesturesEnabled(mapId: mapId);
expect(scrollGesturesEnabled, false);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
scrollGesturesEnabled =
await inspector.areScrollGesturesEnabled(mapId: mapId);
expect(scrollGesturesEnabled, true);
});
testWidgets('testInitialCenterLocationAtCenter', (WidgetTester tester) async {
await tester.binding.setSurfaceSize(const Size(800, 600));
final Completer<ExampleGoogleMapController> mapControllerCompleter =
Completer<ExampleGoogleMapController>();
final Key key = GlobalKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
mapControllerCompleter.complete(controller);
},
),
),
);
final ExampleGoogleMapController mapController =
await mapControllerCompleter.future;
await tester.pumpAndSettle();
// TODO(cyanglaz): Remove this after we added `mapRendered` callback, and `mapControllerCompleter.complete(controller)` above should happen
// in `mapRendered`.
// https://github.com/flutter/flutter/issues/54758
await Future<void>.delayed(const Duration(seconds: 1));
final ScreenCoordinate coordinate =
await mapController.getScreenCoordinate(_kInitialCameraPosition.target);
final Rect rect = tester.getRect(find.byKey(key));
expect(coordinate.x, (rect.center.dx - rect.topLeft.dx).round());
expect(coordinate.y, (rect.center.dy - rect.topLeft.dy).round());
await tester.binding.setSurfaceSize(null);
},
// TODO(stuartmorgan): Re-enable; see https://github.com/flutter/flutter/issues/139825
skip: true);
testWidgets('testGetVisibleRegion', (WidgetTester tester) async {
final Key key = GlobalKey();
final LatLngBounds zeroLatLngBounds = LatLngBounds(
southwest: const LatLng(0, 0), northeast: const LatLng(0, 0));
final Completer<ExampleGoogleMapController> mapControllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
mapControllerCompleter.complete(controller);
},
),
));
await tester.pumpAndSettle();
final ExampleGoogleMapController mapController =
await mapControllerCompleter.future;
final LatLngBounds firstVisibleRegion =
await mapController.getVisibleRegion();
expect(firstVisibleRegion, isNotNull);
expect(firstVisibleRegion.southwest, isNotNull);
expect(firstVisibleRegion.northeast, isNotNull);
expect(firstVisibleRegion, isNot(zeroLatLngBounds));
expect(firstVisibleRegion.contains(_kInitialMapCenter), isTrue);
// Making a new `LatLngBounds` about (10, 10) distance south west to the `firstVisibleRegion`.
// The size of the `LatLngBounds` is 10 by 10.
final LatLng southWest = LatLng(firstVisibleRegion.southwest.latitude - 20,
firstVisibleRegion.southwest.longitude - 20);
final LatLng northEast = LatLng(firstVisibleRegion.southwest.latitude - 10,
firstVisibleRegion.southwest.longitude - 10);
final LatLng newCenter = LatLng(
(northEast.latitude + southWest.latitude) / 2,
(northEast.longitude + southWest.longitude) / 2,
);
expect(firstVisibleRegion.contains(northEast), isFalse);
expect(firstVisibleRegion.contains(southWest), isFalse);
final LatLngBounds latLngBounds =
LatLngBounds(southwest: southWest, northeast: northEast);
// TODO(iskakaushik): non-zero padding is needed for some device configurations
// https://github.com/flutter/flutter/issues/30575
const double padding = 0;
await mapController
.moveCamera(CameraUpdate.newLatLngBounds(latLngBounds, padding));
await tester.pumpAndSettle(const Duration(seconds: 3));
final LatLngBounds secondVisibleRegion =
await mapController.getVisibleRegion();
expect(secondVisibleRegion, isNotNull);
expect(secondVisibleRegion.southwest, isNotNull);
expect(secondVisibleRegion.northeast, isNotNull);
expect(secondVisibleRegion, isNot(zeroLatLngBounds));
expect(firstVisibleRegion, isNot(secondVisibleRegion));
expect(secondVisibleRegion.contains(newCenter), isTrue);
},
// TODO(stuartmorgan): Re-enable; see https://github.com/flutter/flutter/issues/139825
skip: true);
testWidgets('testTraffic', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
trafficEnabled: true,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
bool isTrafficEnabled = await inspector.isTrafficEnabled(mapId: mapId);
expect(isTrafficEnabled, true);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
isTrafficEnabled = await inspector.isTrafficEnabled(mapId: mapId);
expect(isTrafficEnabled, false);
});
testWidgets('testBuildings', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
final bool isBuildingsEnabled =
await inspector.areBuildingsEnabled(mapId: mapId);
expect(isBuildingsEnabled, true);
});
testWidgets('testMyLocationButtonToggle', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
bool myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, true);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
myLocationButtonEnabled: false,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
));
myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, false);
});
testWidgets('testMyLocationButton initial value false',
(WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
myLocationButtonEnabled: false,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
final bool myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, false);
});
testWidgets('testMyLocationButton initial value true',
(WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
final bool myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, true);
});
testWidgets('testSetMapStyle valid Json String', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
const String mapStyle =
'[{"elementType":"geometry","stylers":[{"color":"#242f3e"}]}]';
await GoogleMapsFlutterPlatform.instance
.setMapStyle(mapStyle, mapId: controller.mapId);
});
testWidgets('testSetMapStyle invalid Json String',
(WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
try {
await GoogleMapsFlutterPlatform.instance
.setMapStyle('invalid_value', mapId: controller.mapId);
fail('expected MapStyleException');
} on MapStyleException catch (e) {
expect(e.cause, isNotNull);
expect(await controller.getStyleError(), isNotNull);
}
});
testWidgets('testSetMapStyle null string', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
await GoogleMapsFlutterPlatform.instance
.setMapStyle(null, mapId: controller.mapId);
});
testWidgets('testGetLatLng', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
await tester.pumpAndSettle();
// TODO(cyanglaz): Remove this after we added `mapRendered` callback, and `mapControllerCompleter.complete(controller)` above should happen
// in `mapRendered`.
// https://github.com/flutter/flutter/issues/54758
await Future<void>.delayed(const Duration(seconds: 1));
final LatLngBounds visibleRegion = await controller.getVisibleRegion();
final LatLng topLeft =
await controller.getLatLng(const ScreenCoordinate(x: 0, y: 0));
final LatLng northWest = LatLng(
visibleRegion.northeast.latitude,
visibleRegion.southwest.longitude,
);
expect(topLeft, northWest);
});
testWidgets('testGetZoomLevel', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
await tester.pumpAndSettle();
// TODO(cyanglaz): Remove this after we added `mapRendered` callback, and `mapControllerCompleter.complete(controller)` above should happen
// in `mapRendered`.
// https://github.com/flutter/flutter/issues/54758
await Future<void>.delayed(const Duration(seconds: 1));
double zoom = await controller.getZoomLevel();
expect(zoom, _kInitialZoomLevel);
await controller.moveCamera(CameraUpdate.zoomTo(7));
await tester.pumpAndSettle();
zoom = await controller.getZoomLevel();
expect(zoom, equals(7));
},
// TODO(stuartmorgan): Re-enable; see https://github.com/flutter/flutter/issues/139825
skip: true);
testWidgets('testScreenCoordinate', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
await tester.pumpAndSettle();
// TODO(cyanglaz): Remove this after we added `mapRendered` callback, and `mapControllerCompleter.complete(controller)` above should happen
// in `mapRendered`.
// https://github.com/flutter/flutter/issues/54758
await Future<void>.delayed(const Duration(seconds: 1));
final LatLngBounds visibleRegion = await controller.getVisibleRegion();
final LatLng northWest = LatLng(
visibleRegion.northeast.latitude,
visibleRegion.southwest.longitude,
);
final ScreenCoordinate topLeft =
await controller.getScreenCoordinate(northWest);
expect(topLeft, const ScreenCoordinate(x: 0, y: 0));
},
// TODO(stuartmorgan): Re-enable; see https://github.com/flutter/flutter/issues/139825
skip: true);
testWidgets('testResizeWidget', (WidgetTester tester) async {
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
final ExampleGoogleMap map = ExampleGoogleMap(
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) async {
controllerCompleter.complete(controller);
},
);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: MaterialApp(
home: Scaffold(
body: SizedBox(height: 100, width: 100, child: map)))));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: MaterialApp(
home: Scaffold(
body: SizedBox(height: 400, width: 400, child: map)))));
await tester.pumpAndSettle();
// TODO(cyanglaz): Remove this after we added `mapRendered` callback, and `mapControllerCompleter.complete(controller)` above should happen
// in `mapRendered`.
// https://github.com/flutter/flutter/issues/54758
await Future<void>.delayed(const Duration(seconds: 1));
// Simple call to make sure that the app hasn't crashed.
final LatLngBounds bounds1 = await controller.getVisibleRegion();
final LatLngBounds bounds2 = await controller.getVisibleRegion();
expect(bounds1, bounds2);
});
testWidgets('testToggleInfoWindow', (WidgetTester tester) async {
const Marker marker = Marker(
markerId: MarkerId('marker'),
infoWindow: InfoWindow(title: 'InfoWindow'));
final Set<Marker> markers = <Marker>{marker};
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
initialCameraPosition: const CameraPosition(target: LatLng(10.0, 15.0)),
markers: markers,
onMapCreated: (ExampleGoogleMapController googleMapController) {
controllerCompleter.complete(googleMapController);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
bool iwVisibleStatus =
await controller.isMarkerInfoWindowShown(marker.markerId);
expect(iwVisibleStatus, false);
await controller.showMarkerInfoWindow(marker.markerId);
iwVisibleStatus = await controller.isMarkerInfoWindowShown(marker.markerId);
expect(iwVisibleStatus, true);
await controller.hideMarkerInfoWindow(marker.markerId);
iwVisibleStatus = await controller.isMarkerInfoWindowShown(marker.markerId);
expect(iwVisibleStatus, false);
});
testWidgets('fromAssetImage', (WidgetTester tester) async {
const double pixelRatio = 2;
const ImageConfiguration imageConfiguration =
ImageConfiguration(devicePixelRatio: pixelRatio);
final BitmapDescriptor mip = await BitmapDescriptor.fromAssetImage(
imageConfiguration, 'red_square.png');
final BitmapDescriptor scaled = await BitmapDescriptor.fromAssetImage(
imageConfiguration, 'red_square.png',
mipmaps: false);
expect((mip.toJson() as List<dynamic>)[2], 1);
expect((scaled.toJson() as List<dynamic>)[2], 2);
});
testWidgets('testTakeSnapshot', (WidgetTester tester) async {
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
),
);
await tester.pumpAndSettle(const Duration(seconds: 3));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
final Uint8List? bytes = await controller.takeSnapshot();
expect(bytes?.isNotEmpty, true);
},
// TODO(stuartmorgan): Re-enable; see https://github.com/flutter/flutter/issues/139825
skip: true);
testWidgets(
'set tileOverlay correctly',
(WidgetTester tester) async {
final Completer<int> mapIdCompleter = Completer<int>();
final TileOverlay tileOverlay1 = TileOverlay(
tileOverlayId: const TileOverlayId('tile_overlay_1'),
tileProvider: _DebugTileProvider(),
zIndex: 2,
transparency: 0.2,
);
final TileOverlay tileOverlay2 = TileOverlay(
tileOverlayId: const TileOverlayId('tile_overlay_2'),
tileProvider: _DebugTileProvider(),
zIndex: 1,
visible: false,
transparency: 0.3,
fadeIn: false,
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
initialCameraPosition: _kInitialCameraPosition,
tileOverlays: <TileOverlay>{tileOverlay1, tileOverlay2},
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
),
);
await tester.pumpAndSettle(const Duration(seconds: 3));
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
final TileOverlay tileOverlayInfo1 = (await inspector
.getTileOverlayInfo(tileOverlay1.mapsId, mapId: mapId))!;
final TileOverlay tileOverlayInfo2 = (await inspector
.getTileOverlayInfo(tileOverlay2.mapsId, mapId: mapId))!;
expect(tileOverlayInfo1.visible, isTrue);
expect(tileOverlayInfo1.fadeIn, isTrue);
expect(
tileOverlayInfo1.transparency, moreOrLessEquals(0.2, epsilon: 0.001));
expect(tileOverlayInfo1.zIndex, 2);
expect(tileOverlayInfo2.visible, isFalse);
expect(tileOverlayInfo2.fadeIn, isFalse);
expect(
tileOverlayInfo2.transparency, moreOrLessEquals(0.3, epsilon: 0.001));
expect(tileOverlayInfo2.zIndex, 1);
},
);
testWidgets(
'update tileOverlays correctly',
(WidgetTester tester) async {
final Completer<int> mapIdCompleter = Completer<int>();
final Key key = GlobalKey();
final TileOverlay tileOverlay1 = TileOverlay(
tileOverlayId: const TileOverlayId('tile_overlay_1'),
tileProvider: _DebugTileProvider(),
zIndex: 2,
transparency: 0.2,
);
final TileOverlay tileOverlay2 = TileOverlay(
tileOverlayId: const TileOverlayId('tile_overlay_2'),
tileProvider: _DebugTileProvider(),
zIndex: 3,
transparency: 0.5,
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
tileOverlays: <TileOverlay>{tileOverlay1, tileOverlay2},
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
),
);
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
final TileOverlay tileOverlay1New = TileOverlay(
tileOverlayId: const TileOverlayId('tile_overlay_1'),
tileProvider: _DebugTileProvider(),
zIndex: 1,
visible: false,
transparency: 0.3,
fadeIn: false,
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
tileOverlays: <TileOverlay>{tileOverlay1New},
onMapCreated: (ExampleGoogleMapController controller) {
fail('update: OnMapCreated should get called only once.');
},
),
),
);
await tester.pumpAndSettle(const Duration(seconds: 3));
final TileOverlay tileOverlayInfo1 = (await inspector
.getTileOverlayInfo(tileOverlay1.mapsId, mapId: mapId))!;
final TileOverlay? tileOverlayInfo2 =
await inspector.getTileOverlayInfo(tileOverlay2.mapsId, mapId: mapId);
expect(tileOverlayInfo1.visible, isFalse);
expect(tileOverlayInfo1.fadeIn, isFalse);
expect(
tileOverlayInfo1.transparency, moreOrLessEquals(0.3, epsilon: 0.001));
expect(tileOverlayInfo1.zIndex, 1);
expect(tileOverlayInfo2, isNull);
},
);
testWidgets(
'remove tileOverlays correctly',
(WidgetTester tester) async {
final Completer<int> mapIdCompleter = Completer<int>();
final Key key = GlobalKey();
final TileOverlay tileOverlay1 = TileOverlay(
tileOverlayId: const TileOverlayId('tile_overlay_1'),
tileProvider: _DebugTileProvider(),
zIndex: 2,
transparency: 0.2,
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
tileOverlays: <TileOverlay>{tileOverlay1},
onMapCreated: (ExampleGoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
),
);
final int mapId = await mapIdCompleter.future;
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
onMapCreated: (ExampleGoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
),
);
await tester.pumpAndSettle(const Duration(seconds: 3));
final TileOverlay? tileOverlayInfo1 =
await inspector.getTileOverlayInfo(tileOverlay1.mapsId, mapId: mapId);
expect(tileOverlayInfo1, isNull);
},
);
testWidgets('testSetStyleMapId', (WidgetTester tester) async {
final Key key = GlobalKey();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
cloudMapId: _kCloudMapId,
),
));
});
testWidgets('getStyleError reports last error', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
style: '[[[this is an invalid style',
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
final String? error = await controller.getStyleError();
expect(error, isNotNull);
});
testWidgets('getStyleError returns null for a valid style',
(WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<ExampleGoogleMapController> controllerCompleter =
Completer<ExampleGoogleMapController>();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ExampleGoogleMap(
key: key,
initialCameraPosition: _kInitialCameraPosition,
// An empty array is the simplest valid style.
style: '[]',
onMapCreated: (ExampleGoogleMapController controller) {
controllerCompleter.complete(controller);
},
),
));
final ExampleGoogleMapController controller =
await controllerCompleter.future;
final String? error = await controller.getStyleError();
expect(error, isNull);
});
}
class _DebugTileProvider implements TileProvider {
_DebugTileProvider() {
boxPaint.isAntiAlias = true;
boxPaint.color = Colors.blue;
boxPaint.strokeWidth = 2.0;
boxPaint.style = PaintingStyle.stroke;
}
static const int width = 100;
static const int height = 100;
static final Paint boxPaint = Paint();
static const TextStyle textStyle = TextStyle(
color: Colors.red,
fontSize: 20,
);
@override
Future<Tile> getTile(int x, int y, int? zoom) async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final Canvas canvas = Canvas(recorder);
final TextSpan textSpan = TextSpan(
text: '$x,$y',
style: textStyle,
);
final TextPainter textPainter = TextPainter(
text: textSpan,
textDirection: TextDirection.ltr,
);
textPainter.layout(
maxWidth: width.toDouble(),
);
textPainter.paint(canvas, Offset.zero);
canvas.drawRect(
Rect.fromLTRB(0, 0, width.toDouble(), width.toDouble()), boxPaint);
final ui.Picture picture = recorder.endRecording();
final Uint8List byteData = await picture
.toImage(width, height)
.then((ui.Image image) =>
image.toByteData(format: ui.ImageByteFormat.png))
.then((ByteData? byteData) => byteData!.buffer.asUint8List());
return Tile(width, height, byteData);
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_ios/example/ios12/integration_test/google_maps_test.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_ios/example/ios12/integration_test/google_maps_test.dart",
"repo_id": "packages",
"token_count": 15454
} | 1,053 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart';
// This is a pared down version of the Dart code from the app-facing package,
// to allow running the same examples for package-local testing.
// TODO(stuartmorgan): Consider extracting this to a shared package. See also
// https://github.com/flutter/flutter/issues/46716.
/// Controller for a single ExampleGoogleMap instance running on the host platform.
class ExampleGoogleMapController {
ExampleGoogleMapController._(
this._googleMapState, {
required this.mapId,
}) {
_connectStreams(mapId);
}
/// The mapId for this controller
final int mapId;
/// Initialize control of a [ExampleGoogleMap] with [id].
///
/// Mainly for internal use when instantiating a [ExampleGoogleMapController] passed
/// in [ExampleGoogleMap.onMapCreated] callback.
static Future<ExampleGoogleMapController> _init(
int id,
CameraPosition initialCameraPosition,
_ExampleGoogleMapState googleMapState,
) async {
await GoogleMapsFlutterPlatform.instance.init(id);
return ExampleGoogleMapController._(
googleMapState,
mapId: id,
);
}
final _ExampleGoogleMapState _googleMapState;
void _connectStreams(int mapId) {
if (_googleMapState.widget.onCameraMoveStarted != null) {
GoogleMapsFlutterPlatform.instance
.onCameraMoveStarted(mapId: mapId)
.listen((_) => _googleMapState.widget.onCameraMoveStarted!());
}
if (_googleMapState.widget.onCameraMove != null) {
GoogleMapsFlutterPlatform.instance.onCameraMove(mapId: mapId).listen(
(CameraMoveEvent e) => _googleMapState.widget.onCameraMove!(e.value));
}
if (_googleMapState.widget.onCameraIdle != null) {
GoogleMapsFlutterPlatform.instance
.onCameraIdle(mapId: mapId)
.listen((_) => _googleMapState.widget.onCameraIdle!());
}
GoogleMapsFlutterPlatform.instance
.onMarkerTap(mapId: mapId)
.listen((MarkerTapEvent e) => _googleMapState.onMarkerTap(e.value));
GoogleMapsFlutterPlatform.instance.onMarkerDragStart(mapId: mapId).listen(
(MarkerDragStartEvent e) =>
_googleMapState.onMarkerDragStart(e.value, e.position));
GoogleMapsFlutterPlatform.instance.onMarkerDrag(mapId: mapId).listen(
(MarkerDragEvent e) =>
_googleMapState.onMarkerDrag(e.value, e.position));
GoogleMapsFlutterPlatform.instance.onMarkerDragEnd(mapId: mapId).listen(
(MarkerDragEndEvent e) =>
_googleMapState.onMarkerDragEnd(e.value, e.position));
GoogleMapsFlutterPlatform.instance.onInfoWindowTap(mapId: mapId).listen(
(InfoWindowTapEvent e) => _googleMapState.onInfoWindowTap(e.value));
GoogleMapsFlutterPlatform.instance
.onPolylineTap(mapId: mapId)
.listen((PolylineTapEvent e) => _googleMapState.onPolylineTap(e.value));
GoogleMapsFlutterPlatform.instance
.onPolygonTap(mapId: mapId)
.listen((PolygonTapEvent e) => _googleMapState.onPolygonTap(e.value));
GoogleMapsFlutterPlatform.instance
.onCircleTap(mapId: mapId)
.listen((CircleTapEvent e) => _googleMapState.onCircleTap(e.value));
GoogleMapsFlutterPlatform.instance
.onTap(mapId: mapId)
.listen((MapTapEvent e) => _googleMapState.onTap(e.position));
GoogleMapsFlutterPlatform.instance.onLongPress(mapId: mapId).listen(
(MapLongPressEvent e) => _googleMapState.onLongPress(e.position));
}
/// Updates configuration options of the map user interface.
Future<void> _updateMapConfiguration(MapConfiguration update) {
return GoogleMapsFlutterPlatform.instance
.updateMapConfiguration(update, mapId: mapId);
}
/// Updates marker configuration.
Future<void> _updateMarkers(MarkerUpdates markerUpdates) {
return GoogleMapsFlutterPlatform.instance
.updateMarkers(markerUpdates, mapId: mapId);
}
/// Updates polygon configuration.
Future<void> _updatePolygons(PolygonUpdates polygonUpdates) {
return GoogleMapsFlutterPlatform.instance
.updatePolygons(polygonUpdates, mapId: mapId);
}
/// Updates polyline configuration.
Future<void> _updatePolylines(PolylineUpdates polylineUpdates) {
return GoogleMapsFlutterPlatform.instance
.updatePolylines(polylineUpdates, mapId: mapId);
}
/// Updates circle configuration.
Future<void> _updateCircles(CircleUpdates circleUpdates) {
return GoogleMapsFlutterPlatform.instance
.updateCircles(circleUpdates, mapId: mapId);
}
/// Updates tile overlays configuration.
Future<void> _updateTileOverlays(Set<TileOverlay> newTileOverlays) {
return GoogleMapsFlutterPlatform.instance
.updateTileOverlays(newTileOverlays: newTileOverlays, mapId: mapId);
}
/// Clears the tile cache so that all tiles will be requested again from the
/// [TileProvider].
Future<void> clearTileCache(TileOverlayId tileOverlayId) async {
return GoogleMapsFlutterPlatform.instance
.clearTileCache(tileOverlayId, mapId: mapId);
}
/// Starts an animated change of the map camera position.
Future<void> animateCamera(CameraUpdate cameraUpdate) {
return GoogleMapsFlutterPlatform.instance
.animateCamera(cameraUpdate, mapId: mapId);
}
/// Changes the map camera position.
Future<void> moveCamera(CameraUpdate cameraUpdate) {
return GoogleMapsFlutterPlatform.instance
.moveCamera(cameraUpdate, mapId: mapId);
}
/// Return [LatLngBounds] defining the region that is visible in a map.
Future<LatLngBounds> getVisibleRegion() {
return GoogleMapsFlutterPlatform.instance.getVisibleRegion(mapId: mapId);
}
/// Return [ScreenCoordinate] of the [LatLng] in the current map view.
Future<ScreenCoordinate> getScreenCoordinate(LatLng latLng) {
return GoogleMapsFlutterPlatform.instance
.getScreenCoordinate(latLng, mapId: mapId);
}
/// Returns [LatLng] corresponding to the [ScreenCoordinate] in the current map view.
Future<LatLng> getLatLng(ScreenCoordinate screenCoordinate) {
return GoogleMapsFlutterPlatform.instance
.getLatLng(screenCoordinate, mapId: mapId);
}
/// Programmatically show the Info Window for a [Marker].
Future<void> showMarkerInfoWindow(MarkerId markerId) {
return GoogleMapsFlutterPlatform.instance
.showMarkerInfoWindow(markerId, mapId: mapId);
}
/// Programmatically hide the Info Window for a [Marker].
Future<void> hideMarkerInfoWindow(MarkerId markerId) {
return GoogleMapsFlutterPlatform.instance
.hideMarkerInfoWindow(markerId, mapId: mapId);
}
/// Returns `true` when the [InfoWindow] is showing, `false` otherwise.
Future<bool> isMarkerInfoWindowShown(MarkerId markerId) {
return GoogleMapsFlutterPlatform.instance
.isMarkerInfoWindowShown(markerId, mapId: mapId);
}
/// Returns the current zoom level of the map
Future<double> getZoomLevel() {
return GoogleMapsFlutterPlatform.instance.getZoomLevel(mapId: mapId);
}
/// Returns the image bytes of the map
Future<Uint8List?> takeSnapshot() {
return GoogleMapsFlutterPlatform.instance.takeSnapshot(mapId: mapId);
}
/// Returns the last style error, if any.
Future<String?> getStyleError() {
return GoogleMapsFlutterPlatform.instance.getStyleError(mapId: mapId);
}
/// Disposes of the platform resources
void dispose() {
GoogleMapsFlutterPlatform.instance.dispose(mapId: mapId);
}
}
// The next map ID to create.
int _nextMapCreationId = 0;
/// A widget which displays a map with data obtained from the Google Maps service.
class ExampleGoogleMap extends StatefulWidget {
/// Creates a widget displaying data from Google Maps services.
///
/// [AssertionError] will be thrown if [initialCameraPosition] is null;
const ExampleGoogleMap({
super.key,
required this.initialCameraPosition,
this.onMapCreated,
this.gestureRecognizers = const <Factory<OneSequenceGestureRecognizer>>{},
this.compassEnabled = true,
this.mapToolbarEnabled = true,
this.cameraTargetBounds = CameraTargetBounds.unbounded,
this.mapType = MapType.normal,
this.minMaxZoomPreference = MinMaxZoomPreference.unbounded,
this.rotateGesturesEnabled = true,
this.scrollGesturesEnabled = true,
this.zoomControlsEnabled = true,
this.zoomGesturesEnabled = true,
this.liteModeEnabled = false,
this.tiltGesturesEnabled = true,
this.myLocationEnabled = false,
this.myLocationButtonEnabled = true,
this.layoutDirection,
/// If no padding is specified default padding will be 0.
this.padding = EdgeInsets.zero,
this.indoorViewEnabled = false,
this.trafficEnabled = false,
this.buildingsEnabled = true,
this.markers = const <Marker>{},
this.polygons = const <Polygon>{},
this.polylines = const <Polyline>{},
this.circles = const <Circle>{},
this.onCameraMoveStarted,
this.tileOverlays = const <TileOverlay>{},
this.onCameraMove,
this.onCameraIdle,
this.onTap,
this.onLongPress,
this.cloudMapId,
this.style,
});
/// Callback method for when the map is ready to be used.
///
/// Used to receive a [ExampleGoogleMapController] for this [ExampleGoogleMap].
final void Function(ExampleGoogleMapController controller)? onMapCreated;
/// The initial position of the map's camera.
final CameraPosition initialCameraPosition;
/// True if the map should show a compass when rotated.
final bool compassEnabled;
/// True if the map should show a toolbar when you interact with the map. Android only.
final bool mapToolbarEnabled;
/// Geographical bounding box for the camera target.
final CameraTargetBounds cameraTargetBounds;
/// Type of map tiles to be rendered.
final MapType mapType;
/// The layout direction to use for the embedded view.
final TextDirection? layoutDirection;
/// Preferred bounds for the camera zoom level.
///
/// Actual bounds depend on map data and device.
final MinMaxZoomPreference minMaxZoomPreference;
/// True if the map view should respond to rotate gestures.
final bool rotateGesturesEnabled;
/// True if the map view should respond to scroll gestures.
final bool scrollGesturesEnabled;
/// True if the map view should show zoom controls. This includes two buttons
/// to zoom in and zoom out. The default value is to show zoom controls.
final bool zoomControlsEnabled;
/// True if the map view should respond to zoom gestures.
final bool zoomGesturesEnabled;
/// True if the map view should be in lite mode. Android only.
final bool liteModeEnabled;
/// True if the map view should respond to tilt gestures.
final bool tiltGesturesEnabled;
/// Padding to be set on map.
final EdgeInsets padding;
/// Markers to be placed on the map.
final Set<Marker> markers;
/// Polygons to be placed on the map.
final Set<Polygon> polygons;
/// Polylines to be placed on the map.
final Set<Polyline> polylines;
/// Circles to be placed on the map.
final Set<Circle> circles;
/// Tile overlays to be placed on the map.
final Set<TileOverlay> tileOverlays;
/// Called when the camera starts moving.
final VoidCallback? onCameraMoveStarted;
/// Called repeatedly as the camera continues to move after an
/// onCameraMoveStarted call.
final CameraPositionCallback? onCameraMove;
/// Called when camera movement has ended, there are no pending
/// animations and the user has stopped interacting with the map.
final VoidCallback? onCameraIdle;
/// Called every time a [ExampleGoogleMap] is tapped.
final ArgumentCallback<LatLng>? onTap;
/// Called every time a [ExampleGoogleMap] is long pressed.
final ArgumentCallback<LatLng>? onLongPress;
/// True if a "My Location" layer should be shown on the map.
final bool myLocationEnabled;
/// Enables or disables the my-location button.
final bool myLocationButtonEnabled;
/// Enables or disables the indoor view from the map
final bool indoorViewEnabled;
/// Enables or disables the traffic layer of the map
final bool trafficEnabled;
/// Enables or disables showing 3D buildings where available
final bool buildingsEnabled;
/// Which gestures should be consumed by the map.
final Set<Factory<OneSequenceGestureRecognizer>> gestureRecognizers;
/// Identifier that's associated with a specific cloud-based map style.
///
/// See https://developers.google.com/maps/documentation/get-map-id
/// for more details.
final String? cloudMapId;
/// The locally configured style for the map.
final String? style;
/// Creates a [State] for this [ExampleGoogleMap].
@override
State createState() => _ExampleGoogleMapState();
}
class _ExampleGoogleMapState extends State<ExampleGoogleMap> {
final int _mapId = _nextMapCreationId++;
final Completer<ExampleGoogleMapController> _controller =
Completer<ExampleGoogleMapController>();
Map<MarkerId, Marker> _markers = <MarkerId, Marker>{};
Map<PolygonId, Polygon> _polygons = <PolygonId, Polygon>{};
Map<PolylineId, Polyline> _polylines = <PolylineId, Polyline>{};
Map<CircleId, Circle> _circles = <CircleId, Circle>{};
late MapConfiguration _mapConfiguration;
@override
Widget build(BuildContext context) {
return GoogleMapsFlutterPlatform.instance.buildViewWithConfiguration(
_mapId,
onPlatformViewCreated,
widgetConfiguration: MapWidgetConfiguration(
textDirection: widget.layoutDirection ??
Directionality.maybeOf(context) ??
TextDirection.ltr,
initialCameraPosition: widget.initialCameraPosition,
gestureRecognizers: widget.gestureRecognizers,
),
mapObjects: MapObjects(
markers: widget.markers,
polygons: widget.polygons,
polylines: widget.polylines,
circles: widget.circles,
),
mapConfiguration: _mapConfiguration,
);
}
@override
void initState() {
super.initState();
_mapConfiguration = _configurationFromMapWidget(widget);
_markers = keyByMarkerId(widget.markers);
_polygons = keyByPolygonId(widget.polygons);
_polylines = keyByPolylineId(widget.polylines);
_circles = keyByCircleId(widget.circles);
}
@override
void dispose() {
_controller.future
.then((ExampleGoogleMapController controller) => controller.dispose());
super.dispose();
}
@override
void didUpdateWidget(ExampleGoogleMap oldWidget) {
super.didUpdateWidget(oldWidget);
_updateOptions();
_updateMarkers();
_updatePolygons();
_updatePolylines();
_updateCircles();
_updateTileOverlays();
}
Future<void> _updateOptions() async {
final MapConfiguration newConfig = _configurationFromMapWidget(widget);
final MapConfiguration updates = newConfig.diffFrom(_mapConfiguration);
if (updates.isEmpty) {
return;
}
final ExampleGoogleMapController controller = await _controller.future;
unawaited(controller._updateMapConfiguration(updates));
_mapConfiguration = newConfig;
}
Future<void> _updateMarkers() async {
final ExampleGoogleMapController controller = await _controller.future;
unawaited(controller._updateMarkers(
MarkerUpdates.from(_markers.values.toSet(), widget.markers)));
_markers = keyByMarkerId(widget.markers);
}
Future<void> _updatePolygons() async {
final ExampleGoogleMapController controller = await _controller.future;
unawaited(controller._updatePolygons(
PolygonUpdates.from(_polygons.values.toSet(), widget.polygons)));
_polygons = keyByPolygonId(widget.polygons);
}
Future<void> _updatePolylines() async {
final ExampleGoogleMapController controller = await _controller.future;
unawaited(controller._updatePolylines(
PolylineUpdates.from(_polylines.values.toSet(), widget.polylines)));
_polylines = keyByPolylineId(widget.polylines);
}
Future<void> _updateCircles() async {
final ExampleGoogleMapController controller = await _controller.future;
unawaited(controller._updateCircles(
CircleUpdates.from(_circles.values.toSet(), widget.circles)));
_circles = keyByCircleId(widget.circles);
}
Future<void> _updateTileOverlays() async {
final ExampleGoogleMapController controller = await _controller.future;
unawaited(controller._updateTileOverlays(widget.tileOverlays));
}
Future<void> onPlatformViewCreated(int id) async {
final ExampleGoogleMapController controller =
await ExampleGoogleMapController._init(
id,
widget.initialCameraPosition,
this,
);
_controller.complete(controller);
unawaited(_updateTileOverlays());
widget.onMapCreated?.call(controller);
}
void onMarkerTap(MarkerId markerId) {
_markers[markerId]!.onTap?.call();
}
void onMarkerDragStart(MarkerId markerId, LatLng position) {
_markers[markerId]!.onDragStart?.call(position);
}
void onMarkerDrag(MarkerId markerId, LatLng position) {
_markers[markerId]!.onDrag?.call(position);
}
void onMarkerDragEnd(MarkerId markerId, LatLng position) {
_markers[markerId]!.onDragEnd?.call(position);
}
void onPolygonTap(PolygonId polygonId) {
_polygons[polygonId]!.onTap?.call();
}
void onPolylineTap(PolylineId polylineId) {
_polylines[polylineId]!.onTap?.call();
}
void onCircleTap(CircleId circleId) {
_circles[circleId]!.onTap?.call();
}
void onInfoWindowTap(MarkerId markerId) {
_markers[markerId]!.infoWindow.onTap?.call();
}
void onTap(LatLng position) {
widget.onTap?.call(position);
}
void onLongPress(LatLng position) {
widget.onLongPress?.call(position);
}
}
/// Builds a [MapConfiguration] from the given [map].
MapConfiguration _configurationFromMapWidget(ExampleGoogleMap map) {
return MapConfiguration(
compassEnabled: map.compassEnabled,
mapToolbarEnabled: map.mapToolbarEnabled,
cameraTargetBounds: map.cameraTargetBounds,
mapType: map.mapType,
minMaxZoomPreference: map.minMaxZoomPreference,
rotateGesturesEnabled: map.rotateGesturesEnabled,
scrollGesturesEnabled: map.scrollGesturesEnabled,
tiltGesturesEnabled: map.tiltGesturesEnabled,
trackCameraPosition: map.onCameraMove != null,
zoomControlsEnabled: map.zoomControlsEnabled,
zoomGesturesEnabled: map.zoomGesturesEnabled,
liteModeEnabled: map.liteModeEnabled,
myLocationEnabled: map.myLocationEnabled,
myLocationButtonEnabled: map.myLocationButtonEnabled,
padding: map.padding,
indoorViewEnabled: map.indoorViewEnabled,
trafficEnabled: map.trafficEnabled,
buildingsEnabled: map.buildingsEnabled,
cloudMapId: map.cloudMapId,
style: map.style,
);
}
| packages/packages/google_maps_flutter/google_maps_flutter_ios/example/shared/maps_example_dart/lib/example_google_map.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_ios/example/shared/maps_example_dart/lib/example_google_map.dart",
"repo_id": "packages",
"token_count": 6339
} | 1,054 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: public_member_api_docs
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart';
import 'example_google_map.dart';
import 'page.dart';
const CameraPosition _kInitialPosition =
CameraPosition(target: LatLng(-33.852, 151.211), zoom: 11.0);
class SnapshotPage extends GoogleMapExampleAppPage {
const SnapshotPage({Key? key})
: super(const Icon(Icons.camera_alt), 'Take a snapshot of the map',
key: key);
@override
Widget build(BuildContext context) {
return _SnapshotBody();
}
}
class _SnapshotBody extends StatefulWidget {
@override
_SnapshotBodyState createState() => _SnapshotBodyState();
}
class _SnapshotBodyState extends State<_SnapshotBody> {
ExampleGoogleMapController? _mapController;
Uint8List? _imageBytes;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
SizedBox(
height: 180,
child: ExampleGoogleMap(
onMapCreated: onMapCreated,
initialCameraPosition: _kInitialPosition,
),
),
TextButton(
child: const Text('Take a snapshot'),
onPressed: () async {
final Uint8List? imageBytes =
await _mapController?.takeSnapshot();
setState(() {
_imageBytes = imageBytes;
});
},
),
Container(
decoration: BoxDecoration(color: Colors.blueGrey[50]),
height: 180,
child: _imageBytes != null ? Image.memory(_imageBytes!) : null,
),
],
),
);
}
// ignore: use_setters_to_change_properties
void onMapCreated(ExampleGoogleMapController controller) {
_mapController = controller;
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_ios/example/shared/maps_example_dart/lib/snapshot.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_ios/example/shared/maps_example_dart/lib/snapshot.dart",
"repo_id": "packages",
"token_count": 898
} | 1,055 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import <Flutter/Flutter.h>
#import <GoogleMaps/GoogleMaps.h>
NS_ASSUME_NONNULL_BEGIN
@interface FLTGoogleMapController (Test)
/// Initializes a map controller with a concrete map view.
///
/// @param mapView A map view that will be displayed by the controller
/// @param viewId A unique identifier for the controller.
/// @param args Parameters for initialising the map view.
/// @param registrar The plugin registrar passed from Flutter.
- (instancetype)initWithMapView:(GMSMapView *)mapView
viewIdentifier:(int64_t)viewId
arguments:(id _Nullable)args
registrar:(NSObject<FlutterPluginRegistrar> *)registrar;
@end
NS_ASSUME_NONNULL_END
| packages/packages/google_maps_flutter/google_maps_flutter_ios/ios/Classes/GoogleMapController_Test.h/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_ios/ios/Classes/GoogleMapController_Test.h",
"repo_id": "packages",
"token_count": 298
} | 1,056 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart' show immutable;
import 'types.dart';
/// Uniquely identifies a [ClusterManager] among [GoogleMap] clusters.
///
/// This does not have to be globally unique, only unique among the list.
@immutable
class ClusterManagerId extends MapsObjectId<ClusterManager> {
/// Creates an immutable identifier for a [ClusterManager].
const ClusterManagerId(super.value);
}
/// [ClusterManager] manages marker clustering for set of [Marker]s that have
/// the same [ClusterManagerId] set.
@immutable
class ClusterManager implements MapsObject<ClusterManager> {
/// Creates an immutable object for managing clustering for set of markers.
const ClusterManager({
required this.clusterManagerId,
this.onClusterTap,
});
/// Uniquely identifies a [ClusterManager].
final ClusterManagerId clusterManagerId;
@override
ClusterManagerId get mapsId => clusterManagerId;
/// Callback to receive tap events for cluster markers placed on this map.
final ArgumentCallback<Cluster>? onClusterTap;
/// Creates a new [ClusterManager] object whose values are the same as this instance,
/// unless overwritten by the specified parameters.
ClusterManager copyWith({
ArgumentCallback<Cluster>? onClusterTapParam,
}) {
return ClusterManager(
clusterManagerId: clusterManagerId,
onClusterTap: onClusterTapParam ?? onClusterTap,
);
}
/// Creates a new [ClusterManager] object whose values are the same as this instance.
@override
ClusterManager clone() => copyWith();
/// Converts this object to something serializable in JSON.
@override
Object toJson() {
final Map<String, Object> json = <String, Object>{};
void addIfPresent(String fieldName, Object? value) {
if (value != null) {
json[fieldName] = value;
}
}
addIfPresent('clusterManagerId', clusterManagerId.value);
return json;
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ClusterManager &&
clusterManagerId == other.clusterManagerId;
}
@override
int get hashCode => clusterManagerId.hashCode;
@override
String toString() {
return 'Cluster{clusterManagerId: $clusterManagerId, onClusterTap: $onClusterTap}';
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/lib/src/types/cluster_manager.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/lib/src/types/cluster_manager.dart",
"repo_id": "packages",
"token_count": 752
} | 1,057 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart' show immutable, objectRuntimeType;
/// Represents a point coordinate in the [GoogleMap]'s view.
///
/// The screen location is specified in screen pixels (not display pixels) relative
/// to the top left of the map, not top left of the whole screen. (x, y) = (0, 0)
/// corresponds to top-left of the [GoogleMap] not the whole screen.
@immutable
class ScreenCoordinate {
/// Creates an immutable representation of a point coordinate in the [GoogleMap]'s view.
const ScreenCoordinate({
required this.x,
required this.y,
});
/// Represents the number of pixels from the left of the [GoogleMap].
final int x;
/// Represents the number of pixels from the top of the [GoogleMap].
final int y;
/// Converts this object to something serializable in JSON.
Object toJson() {
return <String, int>{
'x': x,
'y': y,
};
}
@override
String toString() => '${objectRuntimeType(this, 'ScreenCoordinate')}($x, $y)';
@override
bool operator ==(Object other) {
return other is ScreenCoordinate && other.x == x && other.y == y;
}
@override
int get hashCode => Object.hash(x, y);
}
| packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/lib/src/types/screen_coordinate.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/lib/src/types/screen_coordinate.dart",
"repo_id": "packages",
"token_count": 410
} | 1,058 |
name: google_maps_flutter_platform_interface
description: A common platform interface for the google_maps_flutter plugin.
repository: https://github.com/flutter/packages/tree/main/packages/google_maps_flutter/google_maps_flutter_platform_interface
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+maps%22
# NOTE: We strongly prefer non-breaking changes, even at the expense of a
# less-clean API. See https://flutter.dev/go/platform-interface-breaking-changes
version: 2.6.0
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
dependencies:
collection: ^1.15.0
flutter:
sdk: flutter
plugin_platform_interface: ^2.1.7
stream_transform: ^2.0.0
dev_dependencies:
async: ^2.5.0
flutter_test:
sdk: flutter
mockito: 5.4.4
topics:
- google-maps
- google-maps-flutter
- map
| packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/pubspec.yaml/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/pubspec.yaml",
"repo_id": "packages",
"token_count": 318
} | 1,059 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:typed_data';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
group('tile tests', () {
test('toJson returns correct format', () async {
final Uint8List data = Uint8List.fromList(<int>[0, 1]);
final Tile tile = Tile(100, 200, data);
final Object json = tile.toJson();
expect(json, <String, Object>{
'width': 100,
'height': 200,
'data': data,
});
});
test('toJson handles null data', () async {
const Tile tile = Tile(0, 0, null);
final Object json = tile.toJson();
expect(json, <String, Object>{
'width': 0,
'height': 0,
});
});
});
}
| packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/test/types/tile_test.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/test/types/tile_test.dart",
"repo_id": "packages",
"token_count": 386
} | 1,060 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_maps/google_maps.dart' as gmaps;
import 'package:google_maps_flutter/google_maps_flutter.dart';
import 'package:google_maps_flutter_web/google_maps_flutter_web.dart'
hide GoogleMapController;
// ignore: implementation_imports
import 'package:google_maps_flutter_web/src/utils.dart';
import 'package:integration_test/integration_test.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'package:web/web.dart';
@GenerateNiceMocks(<MockSpec<dynamic>>[MockSpec<TileProvider>()])
import 'overlays_test.mocks.dart';
MockTileProvider neverTileProvider() {
final MockTileProvider tileProvider = MockTileProvider();
when(tileProvider.getTile(any, any, any))
.thenAnswer((_) => Completer<Tile>().future);
return tileProvider;
}
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
group('TileOverlaysController', () {
late TileOverlaysController controller;
late gmaps.GMap map;
late List<MockTileProvider> tileProviders;
late List<TileOverlay> tileOverlays;
/// Queries the current overlay map types for tiles at x = 0, y = 0, zoom =
/// 0.
void probeTiles() {
for (final gmaps.MapType? mapType in map.overlayMapTypes!.array!) {
mapType?.getTile!(gmaps.Point(0, 0), 0, document);
}
}
setUp(() {
controller = TileOverlaysController();
map = gmaps.GMap(createDivElement());
controller.googleMap = map;
tileProviders = <MockTileProvider>[
for (int i = 0; i < 3; ++i) neverTileProvider()
];
tileOverlays = <TileOverlay>[
for (int i = 0; i < 3; ++i)
TileOverlay(
tileOverlayId: TileOverlayId('$i'),
tileProvider: tileProviders[i],
zIndex: i)
];
});
testWidgets('addTileOverlays', (WidgetTester tester) async {
controller.addTileOverlays(<TileOverlay>{...tileOverlays});
probeTiles();
verifyInOrder(<dynamic>[
tileProviders[0].getTile(any, any, any),
tileProviders[1].getTile(any, any, any),
tileProviders[2].getTile(any, any, any),
]);
verifyNoMoreInteractions(tileProviders[0]);
verifyNoMoreInteractions(tileProviders[1]);
verifyNoMoreInteractions(tileProviders[2]);
});
testWidgets('changeTileOverlays', (WidgetTester tester) async {
controller.addTileOverlays(<TileOverlay>{...tileOverlays});
// Set overlay 0 visiblity to false; flip z ordering of 1 and 2, leaving 1
// unchanged.
controller.changeTileOverlays(<TileOverlay>{
tileOverlays[0].copyWith(visibleParam: false),
tileOverlays[2].copyWith(zIndexParam: 0),
});
probeTiles();
verifyInOrder(<dynamic>[
tileProviders[2].getTile(any, any, any),
tileProviders[1].getTile(any, any, any),
]);
verifyZeroInteractions(tileProviders[0]);
verifyNoMoreInteractions(tileProviders[1]);
verifyNoMoreInteractions(tileProviders[2]);
// Re-enable overlay 0.
controller.changeTileOverlays(
<TileOverlay>{tileOverlays[0].copyWith(visibleParam: true)});
probeTiles();
verify(tileProviders[2].getTile(any, any, any));
verifyInOrder(<dynamic>[
tileProviders[0].getTile(any, any, any),
tileProviders[1].getTile(any, any, any),
]);
verifyNoMoreInteractions(tileProviders[0]);
verifyNoMoreInteractions(tileProviders[1]);
verifyNoMoreInteractions(tileProviders[2]);
});
testWidgets(
'updating the z index of a hidden layer does not make it visible',
(WidgetTester tester) async {
controller.addTileOverlays(<TileOverlay>{...tileOverlays});
controller.changeTileOverlays(<TileOverlay>{
tileOverlays[0].copyWith(zIndexParam: -1, visibleParam: false),
});
probeTiles();
verifyZeroInteractions(tileProviders[0]);
});
testWidgets('removeTileOverlays', (WidgetTester tester) async {
controller.addTileOverlays(<TileOverlay>{...tileOverlays});
controller.removeTileOverlays(<TileOverlayId>{
tileOverlays[0].tileOverlayId,
tileOverlays[2].tileOverlayId,
});
probeTiles();
verify(tileProviders[1].getTile(any, any, any));
verifyZeroInteractions(tileProviders[0]);
verifyZeroInteractions(tileProviders[2]);
});
testWidgets('clearTileCache', (WidgetTester tester) async {
final Completer<GoogleMapController> controllerCompleter =
Completer<GoogleMapController>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: GoogleMap(
initialCameraPosition: const CameraPosition(
target: LatLng(43.3078, -5.6958),
zoom: 14,
),
tileOverlays: <TileOverlay>{...tileOverlays.take(2)},
onMapCreated: (GoogleMapController value) {
controllerCompleter.complete(value);
addTearDown(() => value.dispose());
},
))));
// This is needed to kick-off the rendering of the JS Map flutter widget
await tester.pump();
final GoogleMapController controller = await controllerCompleter.future;
await tester.pump();
verify(tileProviders[0].getTile(any, any, any));
verify(tileProviders[1].getTile(any, any, any));
await controller.clearTileCache(tileOverlays[0].tileOverlayId);
await tester.pump();
verify(tileProviders[0].getTile(any, any, any));
verifyNoMoreInteractions(tileProviders[1]);
});
});
}
| packages/packages/google_maps_flutter/google_maps_flutter_web/example/integration_test/overlays_test.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_web/example/integration_test/overlays_test.dart",
"repo_id": "packages",
"token_count": 2363
} | 1,061 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
part of '../google_maps_flutter_web.dart';
/// Type used when passing an override to the _createMap function.
@visibleForTesting
typedef DebugCreateMapFunction = gmaps.GMap Function(
HTMLElement div, gmaps.MapOptions options);
/// Type used when passing an override to the _setOptions function.
@visibleForTesting
typedef DebugSetOptionsFunction = void Function(gmaps.MapOptions options);
/// Encapsulates a [gmaps.GMap], its events, and where in the DOM it's rendered.
class GoogleMapController {
/// Initializes the GMap, and the sub-controllers related to it. Wires events.
GoogleMapController({
required int mapId,
required StreamController<MapEvent<Object?>> streamController,
required MapWidgetConfiguration widgetConfiguration,
MapObjects mapObjects = const MapObjects(),
MapConfiguration mapConfiguration = const MapConfiguration(),
}) : _mapId = mapId,
_streamController = streamController,
_initialCameraPosition = widgetConfiguration.initialCameraPosition,
_markers = mapObjects.markers,
_polygons = mapObjects.polygons,
_polylines = mapObjects.polylines,
_circles = mapObjects.circles,
_tileOverlays = mapObjects.tileOverlays,
_lastMapConfiguration = mapConfiguration {
_circlesController = CirclesController(stream: _streamController);
_polygonsController = PolygonsController(stream: _streamController);
_polylinesController = PolylinesController(stream: _streamController);
_markersController = MarkersController(stream: _streamController);
_tileOverlaysController = TileOverlaysController();
_updateStylesFromConfiguration(mapConfiguration);
// Register the view factory that will hold the `_div` that holds the map in the DOM.
// The `_div` needs to be created outside of the ViewFactory (and cached!) so we can
// use it to create the [gmaps.GMap] in the `init()` method of this class.
_div = createDivElement()
..id = _getViewType(mapId)
..style.width = '100%'
..style.height = '100%';
ui_web.platformViewRegistry.registerViewFactory(
_getViewType(mapId),
(int viewId) => _div,
);
}
// The internal ID of the map. Used to broadcast events, DOM IDs and everything where a unique ID is needed.
final int _mapId;
final CameraPosition _initialCameraPosition;
final Set<Marker> _markers;
final Set<Polygon> _polygons;
final Set<Polyline> _polylines;
final Set<Circle> _circles;
Set<TileOverlay> _tileOverlays;
// The configuration passed by the user, before converting to gmaps.
// Caching this allows us to re-create the map faithfully when needed.
MapConfiguration _lastMapConfiguration = const MapConfiguration();
List<gmaps.MapTypeStyle> _lastStyles = const <gmaps.MapTypeStyle>[];
// The last error resulting from providing a map style, if any.
String? _lastStyleError;
/// Configuration accessor for the [GoogleMapsInspectorWeb].
///
/// Returns the latest value of [MapConfiguration] set by the programmer.
///
/// This should only be used by an inspector instance created when a test
/// calls [GoogleMapsPlugin.enableDebugInspection].
MapConfiguration get configuration => _lastMapConfiguration;
// Creates the 'viewType' for the _widget
String _getViewType(int mapId) => 'plugins.flutter.io/google_maps_$mapId';
// The Flutter widget that contains the rendered Map.
HtmlElementView? _widget;
late HTMLElement _div;
/// The Flutter widget that will contain the rendered Map. Used for caching.
Widget? get widget {
if (_widget == null && !_streamController.isClosed) {
_widget = HtmlElementView(
viewType: _getViewType(_mapId),
);
}
return _widget;
}
// The currently-enabled traffic layer.
gmaps.TrafficLayer? _trafficLayer;
/// A getter for the current traffic layer. Only for tests.
@visibleForTesting
gmaps.TrafficLayer? get trafficLayer => _trafficLayer;
// The underlying GMap instance. This is the interface with the JS SDK.
gmaps.GMap? _googleMap;
// The StreamController used by this controller and the geometry ones.
final StreamController<MapEvent<Object?>> _streamController;
/// The StreamController for the events of this Map. Only for integration testing.
@visibleForTesting
StreamController<MapEvent<Object?>> get stream => _streamController;
/// The Stream over which this controller broadcasts events.
Stream<MapEvent<Object?>> get events => _streamController.stream;
// Geometry controllers, for different features of the map.
CirclesController? _circlesController;
PolygonsController? _polygonsController;
PolylinesController? _polylinesController;
MarkersController? _markersController;
TileOverlaysController? _tileOverlaysController;
// Keeps track if _attachGeometryControllers has been called or not.
bool _controllersBoundToMap = false;
// Keeps track if the map is moving or not.
bool _mapIsMoving = false;
/// Overrides certain properties to install mocks defined during testing.
@visibleForTesting
void debugSetOverrides({
DebugCreateMapFunction? createMap,
DebugSetOptionsFunction? setOptions,
MarkersController? markers,
CirclesController? circles,
PolygonsController? polygons,
PolylinesController? polylines,
TileOverlaysController? tileOverlays,
}) {
_overrideCreateMap = createMap;
_overrideSetOptions = setOptions;
_markersController = markers ?? _markersController;
_circlesController = circles ?? _circlesController;
_polygonsController = polygons ?? _polygonsController;
_polylinesController = polylines ?? _polylinesController;
_tileOverlaysController = tileOverlays ?? _tileOverlaysController;
}
DebugCreateMapFunction? _overrideCreateMap;
DebugSetOptionsFunction? _overrideSetOptions;
gmaps.GMap _createMap(HTMLElement div, gmaps.MapOptions options) {
if (_overrideCreateMap != null) {
return _overrideCreateMap!(div, options);
}
return gmaps.GMap(div, options);
}
/// A flag that returns true if the controller has been initialized or not.
@visibleForTesting
bool get isInitialized => _googleMap != null;
/// Starts the JS Maps SDK into the target [_div] with `rawOptions`.
///
/// (Also initializes the geometry/traffic layers.)
///
/// The first part of this method starts the rendering of a [gmaps.GMap] inside
/// of the target [_div], with configuration from `rawOptions`. It then stores
/// the created GMap in the [_googleMap] attribute.
///
/// Not *everything* is rendered with the initial `rawOptions` configuration,
/// geometry and traffic layers (and possibly others in the future) have their
/// own configuration and are rendered on top of a GMap instance later. This
/// happens in the second half of this method.
///
/// This method is eagerly called from the [GoogleMapsPlugin.buildView] method
/// so the internal [GoogleMapsController] of a Web Map initializes as soon as
/// possible. Check [_attachMapEvents] to see how this controller notifies the
/// plugin of it being fully ready (through the `onTilesloaded.first` event).
///
/// Failure to call this method would result in the GMap not rendering at all,
/// and most of the public methods on this class no-op'ing.
void init() {
gmaps.MapOptions options = _configurationAndStyleToGmapsOptions(
_lastMapConfiguration, _lastStyles);
// Initial position can only to be set here!
options = _applyInitialPosition(_initialCameraPosition, options);
// Fully disable 45 degree imagery if desired
if (options.rotateControl == false) {
options.tilt = 0;
}
// Create the map...
final gmaps.GMap map = _createMap(_div, options);
_googleMap = map;
_attachMapEvents(map);
_attachGeometryControllers(map);
// Now attach the geometry, traffic and any other layers...
_renderInitialGeometry();
_setTrafficLayer(map, _lastMapConfiguration.trafficEnabled ?? false);
}
// Funnels map gmap events into the plugin's stream controller.
void _attachMapEvents(gmaps.GMap map) {
map.onTilesloaded.first.then((void _) {
// Report the map as ready to go the first time the tiles load
_streamController.add(WebMapReadyEvent(_mapId));
});
map.onClick.listen((gmaps.IconMouseEvent event) {
assert(event.latLng != null);
_streamController.add(
MapTapEvent(_mapId, _gmLatLngToLatLng(event.latLng!)),
);
});
map.onRightclick.listen((gmaps.MapMouseEvent event) {
assert(event.latLng != null);
_streamController.add(
MapLongPressEvent(_mapId, _gmLatLngToLatLng(event.latLng!)),
);
});
map.onBoundsChanged.listen((void _) {
if (!_mapIsMoving) {
_mapIsMoving = true;
_streamController.add(CameraMoveStartedEvent(_mapId));
}
_streamController.add(
CameraMoveEvent(_mapId, _gmViewportToCameraPosition(map)),
);
});
map.onIdle.listen((void _) {
_mapIsMoving = false;
_streamController.add(CameraIdleEvent(_mapId));
});
}
// Binds the Geometry controllers to a map instance
void _attachGeometryControllers(gmaps.GMap map) {
// Now we can add the initial geometry.
// And bind the (ready) map instance to the other geometry controllers.
//
// These controllers are either created in the constructor of this class, or
// overriden (for testing) by the [debugSetOverrides] method. They can't be
// null.
assert(_circlesController != null,
'Cannot attach a map to a null CirclesController instance.');
assert(_polygonsController != null,
'Cannot attach a map to a null PolygonsController instance.');
assert(_polylinesController != null,
'Cannot attach a map to a null PolylinesController instance.');
assert(_markersController != null,
'Cannot attach a map to a null MarkersController instance.');
assert(_tileOverlaysController != null,
'Cannot attach a map to a null TileOverlaysController instance.');
_circlesController!.bindToMap(_mapId, map);
_polygonsController!.bindToMap(_mapId, map);
_polylinesController!.bindToMap(_mapId, map);
_markersController!.bindToMap(_mapId, map);
_tileOverlaysController!.bindToMap(_mapId, map);
_controllersBoundToMap = true;
}
// Renders the initial sets of geometry.
void _renderInitialGeometry() {
assert(
_controllersBoundToMap,
'Geometry controllers must be bound to a map before any geometry can '
'be added to them. Ensure _attachGeometryControllers is called first.');
// The above assert will only succeed if the controllers have been bound to a map
// in the [_attachGeometryControllers] method, which ensures that all these
// controllers below are *not* null.
_markersController!.addMarkers(_markers);
_circlesController!.addCircles(_circles);
_polygonsController!.addPolygons(_polygons);
_polylinesController!.addPolylines(_polylines);
_tileOverlaysController!.addTileOverlays(_tileOverlays);
}
// Merges new options coming from the plugin into _lastConfiguration.
//
// Returns the updated _lastConfiguration object.
MapConfiguration _mergeConfigurations(MapConfiguration update) {
_lastMapConfiguration = _lastMapConfiguration.applyDiff(update);
return _lastMapConfiguration;
}
// TODO(stuartmorgan): Refactor so that _lastMapConfiguration.style is the
// source of truth for style info. Currently it's tracked and handled
// separately since style didn't used to be part of the configuration.
List<gmaps.MapTypeStyle> _updateStylesFromConfiguration(
MapConfiguration update) {
if (update.style != null) {
// Provide async access to the error rather than throwing, to match the
// behavior of other platforms where there's no mechanism to return errors
// from configuration updates.
try {
_lastStyles = _mapStyles(update.style);
_lastStyleError = null;
} on MapStyleException catch (e) {
_lastStyleError = e.cause;
}
}
return _lastStyles;
}
/// Updates the map options from a [MapConfiguration].
///
/// This method converts the map into the proper [gmaps.MapOptions].
void updateMapConfiguration(MapConfiguration update) {
assert(_googleMap != null, 'Cannot update options on a null map.');
final List<gmaps.MapTypeStyle> styles =
_updateStylesFromConfiguration(update);
final MapConfiguration newConfiguration = _mergeConfigurations(update);
final gmaps.MapOptions newOptions =
_configurationAndStyleToGmapsOptions(newConfiguration, styles);
_setOptions(newOptions);
_setTrafficLayer(_googleMap!, newConfiguration.trafficEnabled ?? false);
}
/// Updates the map options with a new list of [styles].
void updateStyles(List<gmaps.MapTypeStyle> styles) {
_lastStyles = styles;
_setOptions(
_configurationAndStyleToGmapsOptions(_lastMapConfiguration, styles));
}
/// A getter for the current styles. Only for tests.
@visibleForTesting
List<gmaps.MapTypeStyle> get styles => _lastStyles;
/// Returns the last error from setting the map's style, if any.
String? get lastStyleError => _lastStyleError;
// Sets new [gmaps.MapOptions] on the wrapped map.
// ignore: use_setters_to_change_properties
void _setOptions(gmaps.MapOptions options) {
if (_overrideSetOptions != null) {
return _overrideSetOptions!(options);
}
_googleMap?.options = options;
}
// Attaches/detaches a Traffic Layer on the passed `map` if `attach` is true/false.
void _setTrafficLayer(gmaps.GMap map, bool attach) {
if (attach && _trafficLayer == null) {
_trafficLayer = gmaps.TrafficLayer()..set('map', map);
}
if (!attach && _trafficLayer != null) {
_trafficLayer!.set('map', null);
_trafficLayer = null;
}
}
// _googleMap manipulation
// Viewport
/// Returns the [LatLngBounds] of the current viewport.
Future<LatLngBounds> getVisibleRegion() async {
assert(_googleMap != null, 'Cannot get the visible region of a null map.');
final gmaps.LatLngBounds bounds =
await Future<gmaps.LatLngBounds?>.value(_googleMap!.bounds) ??
_nullGmapsLatLngBounds;
return _gmLatLngBoundsTolatLngBounds(bounds);
}
/// Returns the [ScreenCoordinate] for a given viewport [LatLng].
Future<ScreenCoordinate> getScreenCoordinate(LatLng latLng) async {
assert(_googleMap != null,
'Cannot get the screen coordinates with a null map.');
final gmaps.Point point =
toScreenLocation(_googleMap!, _latLngToGmLatLng(latLng));
return ScreenCoordinate(x: point.x!.toInt(), y: point.y!.toInt());
}
/// Returns the [LatLng] for a `screenCoordinate` (in pixels) of the viewport.
Future<LatLng> getLatLng(ScreenCoordinate screenCoordinate) async {
assert(_googleMap != null,
'Cannot get the lat, lng of a screen coordinate with a null map.');
final gmaps.LatLng latLng =
_pixelToLatLng(_googleMap!, screenCoordinate.x, screenCoordinate.y);
return _gmLatLngToLatLng(latLng);
}
/// Applies a `cameraUpdate` to the current viewport.
Future<void> moveCamera(CameraUpdate cameraUpdate) async {
assert(_googleMap != null, 'Cannot update the camera of a null map.');
return _applyCameraUpdate(_googleMap!, cameraUpdate);
}
/// Returns the zoom level of the current viewport.
Future<double> getZoomLevel() async {
assert(_googleMap != null, 'Cannot get zoom level of a null map.');
assert(_googleMap!.zoom != null,
'Zoom level should not be null. Is the map correctly initialized?');
return _googleMap!.zoom!.toDouble();
}
// Geometry manipulation
/// Applies [CircleUpdates] to the currently managed circles.
void updateCircles(CircleUpdates updates) {
assert(
_circlesController != null, 'Cannot update circles after dispose().');
_circlesController?.addCircles(updates.circlesToAdd);
_circlesController?.changeCircles(updates.circlesToChange);
_circlesController?.removeCircles(updates.circleIdsToRemove);
}
/// Applies [PolygonUpdates] to the currently managed polygons.
void updatePolygons(PolygonUpdates updates) {
assert(
_polygonsController != null, 'Cannot update polygons after dispose().');
_polygonsController?.addPolygons(updates.polygonsToAdd);
_polygonsController?.changePolygons(updates.polygonsToChange);
_polygonsController?.removePolygons(updates.polygonIdsToRemove);
}
/// Applies [PolylineUpdates] to the currently managed lines.
void updatePolylines(PolylineUpdates updates) {
assert(_polylinesController != null,
'Cannot update polylines after dispose().');
_polylinesController?.addPolylines(updates.polylinesToAdd);
_polylinesController?.changePolylines(updates.polylinesToChange);
_polylinesController?.removePolylines(updates.polylineIdsToRemove);
}
/// Applies [MarkerUpdates] to the currently managed markers.
void updateMarkers(MarkerUpdates updates) {
assert(
_markersController != null, 'Cannot update markers after dispose().');
_markersController?.addMarkers(updates.markersToAdd);
_markersController?.changeMarkers(updates.markersToChange);
_markersController?.removeMarkers(updates.markerIdsToRemove);
}
/// Updates the set of [TileOverlay]s.
void updateTileOverlays(Set<TileOverlay> newOverlays) {
final MapsObjectUpdates<TileOverlay> updates =
MapsObjectUpdates<TileOverlay>.from(_tileOverlays, newOverlays,
objectName: 'tileOverlay');
assert(_tileOverlaysController != null,
'Cannot update tile overlays after dispose().');
_tileOverlaysController?.addTileOverlays(updates.objectsToAdd);
_tileOverlaysController?.changeTileOverlays(updates.objectsToChange);
_tileOverlaysController
?.removeTileOverlays(updates.objectIdsToRemove.cast<TileOverlayId>());
_tileOverlays = newOverlays;
}
/// Clears the tile cache associated with the given [TileOverlayId].
void clearTileCache(TileOverlayId id) {
_tileOverlaysController?.clearTileCache(id);
}
/// Shows the [InfoWindow] of the marker identified by its [MarkerId].
void showInfoWindow(MarkerId markerId) {
assert(_markersController != null,
'Cannot show infowindow of marker [${markerId.value}] after dispose().');
_markersController?.showMarkerInfoWindow(markerId);
}
/// Hides the [InfoWindow] of the marker identified by its [MarkerId].
void hideInfoWindow(MarkerId markerId) {
assert(_markersController != null,
'Cannot hide infowindow of marker [${markerId.value}] after dispose().');
_markersController?.hideMarkerInfoWindow(markerId);
}
/// Returns true if the [InfoWindow] of the marker identified by [MarkerId] is shown.
bool isInfoWindowShown(MarkerId markerId) {
return _markersController?.isInfoWindowShown(markerId) ?? false;
}
// Cleanup
/// Disposes of this controller and its resources.
///
/// You won't be able to call many of the methods on this controller after
/// calling `dispose`!
void dispose() {
_widget = null;
_googleMap = null;
_circlesController = null;
_polygonsController = null;
_polylinesController = null;
_markersController = null;
_tileOverlaysController = null;
_streamController.close();
}
}
/// A MapEvent event fired when a [mapId] on web is interactive.
class WebMapReadyEvent extends MapEvent<Object?> {
/// Build a WebMapReady Event for the map represented by `mapId`.
WebMapReadyEvent(int mapId) : super(mapId, null);
}
| packages/packages/google_maps_flutter/google_maps_flutter_web/lib/src/google_maps_controller.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_web/lib/src/google_maps_controller.dart",
"repo_id": "packages",
"token_count": 6462
} | 1,062 |
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="io.flutter.plugins.googlesigninexample">
<uses-permission android:name="android.permission.INTERNET"/>
<application>
<activity android:name="io.flutter.embedding.android.FlutterActivity"
android:theme="@android:style/Theme.Black.NoTitleBar"
android:configChanges="orientation|keyboardHidden|keyboard|screenSize|locale|layoutDirection"
android:hardwareAccelerated="true"
android:windowSoftInputMode="adjustResize">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<meta-data android:name="flutterEmbedding" android:value="2"/>
</application>
</manifest>
| packages/packages/google_sign_in/google_sign_in/example/android/app/src/main/AndroidManifest.xml/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in/example/android/app/src/main/AndroidManifest.xml",
"repo_id": "packages",
"token_count": 388
} | 1,063 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
/// The type of the onClick callback for the (mobile) Sign In Button.
typedef HandleSignInFn = Future<void> Function();
/// Renders a SIGN IN button that (maybe) calls the `handleSignIn` onclick.
Widget buildSignInButton({HandleSignInFn? onPressed}) {
return Container();
}
| packages/packages/google_sign_in/google_sign_in/example/lib/src/sign_in_button/stub.dart/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in/example/lib/src/sign_in_button/stub.dart",
"repo_id": "packages",
"token_count": 148
} | 1,064 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart' show PlatformException;
import 'package:google_sign_in_platform_interface/google_sign_in_platform_interface.dart';
import 'src/common.dart';
export 'package:google_sign_in_platform_interface/google_sign_in_platform_interface.dart'
show SignInOption;
export 'src/common.dart';
export 'widgets.dart';
/// Holds authentication tokens after sign in.
class GoogleSignInAuthentication {
GoogleSignInAuthentication._(this._data);
final GoogleSignInTokenData _data;
/// An OpenID Connect ID token that identifies the user.
String? get idToken => _data.idToken;
/// The OAuth2 access token to access Google services.
String? get accessToken => _data.accessToken;
/// Server auth code used to access Google Login
@Deprecated('Use the `GoogleSignInAccount.serverAuthCode` property instead')
String? get serverAuthCode => _data.serverAuthCode;
@override
String toString() => 'GoogleSignInAuthentication:$_data';
}
/// Holds fields describing a signed in user's identity, following
/// [GoogleSignInUserData].
///
/// [id] is guaranteed to be non-null.
@immutable
class GoogleSignInAccount implements GoogleIdentity {
GoogleSignInAccount._(this._googleSignIn, GoogleSignInUserData data)
: displayName = data.displayName,
email = data.email,
id = data.id,
photoUrl = data.photoUrl,
serverAuthCode = data.serverAuthCode,
_idToken = data.idToken;
// These error codes must match with ones declared on Android and iOS sides.
/// Error code indicating there was a failed attempt to recover user authentication.
static const String kFailedToRecoverAuthError = 'failed_to_recover_auth';
/// Error indicating that authentication can be recovered with user action;
static const String kUserRecoverableAuthError = 'user_recoverable_auth';
@override
final String? displayName;
@override
final String email;
@override
final String id;
@override
final String? photoUrl;
@override
final String? serverAuthCode;
final String? _idToken;
final GoogleSignIn _googleSignIn;
/// Retrieve [GoogleSignInAuthentication] for this account.
///
/// [shouldRecoverAuth] sets whether to attempt to recover authentication if
/// user action is needed. If an attempt to recover authentication fails a
/// [PlatformException] is thrown with possible error code
/// [kFailedToRecoverAuthError].
///
/// Otherwise, if [shouldRecoverAuth] is false and the authentication can be
/// recovered by user action a [PlatformException] is thrown with error code
/// [kUserRecoverableAuthError].
Future<GoogleSignInAuthentication> get authentication async {
if (_googleSignIn.currentUser != this) {
throw StateError('User is no longer signed in.');
}
final GoogleSignInTokenData response =
await GoogleSignInPlatform.instance.getTokens(
email: email,
shouldRecoverAuth: true,
);
// On Android, there isn't an API for refreshing the idToken, so re-use
// the one we obtained on login.
response.idToken ??= _idToken;
return GoogleSignInAuthentication._(response);
}
/// Convenience method returning a `<String, String>` map of HTML Authorization
/// headers, containing the current `authentication.accessToken`.
///
/// See also https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Authorization.
Future<Map<String, String>> get authHeaders async {
final String? token = (await authentication).accessToken;
return <String, String>{
'Authorization': 'Bearer $token',
// TODO(kevmoo): Use the correct value once it's available from authentication
// See https://github.com/flutter/flutter/issues/80905
'X-Goog-AuthUser': '0',
};
}
/// Clears any client side cache that might be holding invalid tokens.
///
/// If client runs into 401 errors using a token, it is expected to call
/// this method and grab `authHeaders` once again.
Future<void> clearAuthCache() async {
final String token = (await authentication).accessToken!;
await GoogleSignInPlatform.instance.clearAuthCache(token: token);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other is! GoogleSignInAccount) {
return false;
}
final GoogleSignInAccount otherAccount = other;
return displayName == otherAccount.displayName &&
email == otherAccount.email &&
id == otherAccount.id &&
photoUrl == otherAccount.photoUrl &&
serverAuthCode == otherAccount.serverAuthCode &&
_idToken == otherAccount._idToken;
}
@override
int get hashCode =>
Object.hash(displayName, email, id, photoUrl, _idToken, serverAuthCode);
@override
String toString() {
final Map<String, dynamic> data = <String, dynamic>{
'displayName': displayName,
'email': email,
'id': id,
'photoUrl': photoUrl,
'serverAuthCode': serverAuthCode
};
return 'GoogleSignInAccount:$data';
}
}
/// GoogleSignIn allows you to authenticate Google users.
class GoogleSignIn {
/// Initializes global sign-in configuration settings.
///
/// The [signInOption] determines the user experience. [SigninOption.games]
/// is only supported on Android.
///
/// The list of [scopes] are OAuth scope codes to request when signing in.
/// These scope codes will determine the level of data access that is granted
/// to your application by the user. The full list of available scopes can
/// be found here:
/// <https://developers.google.com/identity/protocols/googlescopes>
///
/// The [hostedDomain] argument specifies a hosted domain restriction. By
/// setting this, sign in will be restricted to accounts of the user in the
/// specified domain. By default, the list of accounts will not be restricted.
///
/// The [forceCodeForRefreshToken] is used on Android to ensure the authentication
/// code can be exchanged for a refresh token after the first request.
GoogleSignIn({
this.signInOption = SignInOption.standard,
this.scopes = const <String>[],
this.hostedDomain,
this.clientId,
this.serverClientId,
this.forceCodeForRefreshToken = false,
}) {
// Start initializing.
if (kIsWeb) {
// Start initializing the plugin ASAP, so the `userDataEvents` Stream for
// the web can be used without calling any other methods of the plugin
// (like `silentSignIn` or `isSignedIn`).
unawaited(_ensureInitialized());
}
}
/// Factory for creating default sign in user experience.
factory GoogleSignIn.standard({
List<String> scopes = const <String>[],
String? hostedDomain,
}) {
return GoogleSignIn(scopes: scopes, hostedDomain: hostedDomain);
}
/// Factory for creating sign in suitable for games. This option is only
/// supported on Android.
factory GoogleSignIn.games() {
return GoogleSignIn(signInOption: SignInOption.games);
}
// These error codes must match with ones declared on Android and iOS sides.
/// Error code indicating there is no signed in user and interactive sign in
/// flow is required.
static const String kSignInRequiredError = 'sign_in_required';
/// Error code indicating that interactive sign in process was canceled by the
/// user.
static const String kSignInCanceledError = 'sign_in_canceled';
/// Error code indicating network error. Retrying should resolve the problem.
static const String kNetworkError = 'network_error';
/// Error code indicating that attempt to sign in failed.
static const String kSignInFailedError = 'sign_in_failed';
/// Option to determine the sign in user experience. [SignInOption.games] is
/// only supported on Android.
final SignInOption signInOption;
/// The list of [scopes] are OAuth scope codes requested when signing in.
final List<String> scopes;
/// Domain to restrict sign-in to.
final String? hostedDomain;
/// Client ID being used to connect to google sign-in.
///
/// This option is not supported on all platforms (e.g. Android). It is
/// optional if file-based configuration is used.
///
/// The value specified here has precedence over a value from a configuration
/// file.
final String? clientId;
/// Client ID of the backend server to which the app needs to authenticate
/// itself.
///
/// Optional and not supported on all platforms (e.g. web). By default, it
/// is initialized from a configuration file if available.
///
/// The value specified here has precedence over a value from a configuration
/// file.
///
/// [GoogleSignInAuthentication.idToken] and
/// [GoogleSignInAccount.serverAuthCode] will be specific to the backend
/// server.
final String? serverClientId;
/// Force the authorization code to be valid for a refresh token every time. Only needed on Android.
final bool forceCodeForRefreshToken;
final StreamController<GoogleSignInAccount?> _currentUserController =
StreamController<GoogleSignInAccount?>.broadcast();
/// Subscribe to this stream to be notified when the current user changes.
Stream<GoogleSignInAccount?> get onCurrentUserChanged {
return _currentUserController.stream;
}
Future<GoogleSignInAccount?> _callMethod(
Future<dynamic> Function() method) async {
await _ensureInitialized();
final dynamic response = await method();
return _setCurrentUser(response != null && response is GoogleSignInUserData
? GoogleSignInAccount._(this, response)
: null);
}
// Sets the current user, and propagates it through the _currentUserController.
GoogleSignInAccount? _setCurrentUser(GoogleSignInAccount? currentUser) {
if (currentUser != _currentUser) {
_currentUser = currentUser;
_currentUserController.add(_currentUser);
}
return _currentUser;
}
// Future that completes when `init` has completed on the native side.
Future<void>? _initialization;
// Performs initialization, guarding it with the _initialization future.
Future<void> _ensureInitialized() async {
_initialization ??= _doInitialization().catchError((Object e) {
// Invalidate initialization if it errors out.
_initialization = null;
// ignore: only_throw_errors
throw e;
});
return _initialization;
}
// Actually performs the initialization.
//
// This method calls initWithParams, and then, if the plugin instance has a
// userDataEvents Stream, connects it to the [_setCurrentUser] method.
Future<void> _doInitialization() async {
await GoogleSignInPlatform.instance.initWithParams(SignInInitParameters(
signInOption: signInOption,
scopes: scopes,
hostedDomain: hostedDomain,
clientId: clientId,
serverClientId: serverClientId,
forceCodeForRefreshToken: forceCodeForRefreshToken,
));
unawaited(GoogleSignInPlatform.instance.userDataEvents
?.map((GoogleSignInUserData? userData) {
return userData != null ? GoogleSignInAccount._(this, userData) : null;
}).forEach(_setCurrentUser));
}
/// The most recently scheduled method call.
Future<void>? _lastMethodCall;
/// Returns a [Future] that completes with a success after [future], whether
/// it completed with a value or an error.
static Future<void> _waitFor(Future<void> future) {
final Completer<void> completer = Completer<void>();
future.whenComplete(completer.complete).catchError((dynamic _) {
// Ignore if previous call completed with an error.
// TODO(ditman): Should we log errors here, if debug or similar?
});
return completer.future;
}
/// Adds call to [method] in a queue for execution.
///
/// At most one in flight call is allowed to prevent concurrent (out of order)
/// updates to [currentUser] and [onCurrentUserChanged].
///
/// The optional, named parameter [canSkipCall] lets the plugin know that the
/// method call may be skipped, if there's already [_currentUser] information.
/// This is used from the [signIn] and [signInSilently] methods.
Future<GoogleSignInAccount?> _addMethodCall(
Future<dynamic> Function() method, {
bool canSkipCall = false,
}) async {
Future<GoogleSignInAccount?> response;
if (_lastMethodCall == null) {
response = _callMethod(method);
} else {
response = _lastMethodCall!.then((_) {
// If after the last completed call `currentUser` is not `null` and requested
// method can be skipped (`canSkipCall`), re-use the same authenticated user
// instead of making extra call to the native side.
if (canSkipCall && _currentUser != null) {
return _currentUser;
}
return _callMethod(method);
});
}
// Add the current response to the currently running Promise of all pending responses
_lastMethodCall = _waitFor(response);
return response;
}
/// The currently signed in account, or null if the user is signed out.
GoogleSignInAccount? get currentUser => _currentUser;
GoogleSignInAccount? _currentUser;
/// Attempts to sign in a previously authenticated user without interaction.
///
/// Returned Future resolves to an instance of [GoogleSignInAccount] for a
/// successful sign in or `null` if there is no previously authenticated user.
/// Use [signIn] method to trigger interactive sign in process.
///
/// Authentication is triggered if there is no currently signed in
/// user (that is when `currentUser == null`), otherwise this method returns
/// a Future which resolves to the same user instance.
///
/// Re-authentication can be triggered after [signOut] or [disconnect]. It can
/// also be triggered by setting [reAuthenticate] to `true` if a new ID token
/// is required.
///
/// When [suppressErrors] is set to `false` and an error occurred during sign in
/// returned Future completes with [PlatformException] whose `code` can be
/// one of [kSignInRequiredError] (when there is no authenticated user) ,
/// [kNetworkError] (when a network error occurred) or [kSignInFailedError]
/// (when an unknown error occurred).
Future<GoogleSignInAccount?> signInSilently({
bool suppressErrors = true,
bool reAuthenticate = false,
}) async {
try {
return await _addMethodCall(GoogleSignInPlatform.instance.signInSilently,
canSkipCall: !reAuthenticate);
} catch (_) {
if (suppressErrors) {
return null;
} else {
rethrow;
}
}
}
/// Returns a future that resolves to whether a user is currently signed in.
Future<bool> isSignedIn() async {
await _ensureInitialized();
return GoogleSignInPlatform.instance.isSignedIn();
}
/// Starts the interactive sign-in process.
///
/// Returned Future resolves to an instance of [GoogleSignInAccount] for a
/// successful sign in or `null` in case sign in process was aborted.
///
/// Authentication process is triggered only if there is no currently signed in
/// user (that is when `currentUser == null`), otherwise this method returns
/// a Future which resolves to the same user instance.
///
/// Re-authentication can be triggered only after [signOut] or [disconnect].
Future<GoogleSignInAccount?> signIn() {
final Future<GoogleSignInAccount?> result =
_addMethodCall(GoogleSignInPlatform.instance.signIn, canSkipCall: true);
bool isCanceled(dynamic error) =>
error is PlatformException && error.code == kSignInCanceledError;
return result.catchError((dynamic _) => null, test: isCanceled);
}
/// Marks current user as being in the signed out state.
Future<GoogleSignInAccount?> signOut() =>
_addMethodCall(GoogleSignInPlatform.instance.signOut);
/// Disconnects the current user from the app and revokes previous
/// authentication.
Future<GoogleSignInAccount?> disconnect() =>
_addMethodCall(GoogleSignInPlatform.instance.disconnect);
/// Requests the user grants additional Oauth [scopes].
Future<bool> requestScopes(List<String> scopes) async {
await _ensureInitialized();
return GoogleSignInPlatform.instance.requestScopes(scopes);
}
/// Checks if the current user has granted access to all the specified [scopes].
///
/// Optionally, an [accessToken] can be passed to perform this check. This
/// may be useful when an application holds on to a cached, potentially
/// long-lived [accessToken].
Future<bool> canAccessScopes(
List<String> scopes, {
String? accessToken,
}) async {
await _ensureInitialized();
final String? token =
accessToken ?? (await _currentUser?.authentication)?.accessToken;
return GoogleSignInPlatform.instance.canAccessScopes(
scopes,
accessToken: token,
);
}
}
| packages/packages/google_sign_in/google_sign_in/lib/google_sign_in.dart/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in/lib/google_sign_in.dart",
"repo_id": "packages",
"token_count": 5145
} | 1,065 |
group 'io.flutter.plugins.googlesignin'
version '1.0-SNAPSHOT'
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.2.1'
}
}
rootProject.allprojects {
repositories {
google()
mavenCentral()
}
}
apply plugin: 'com.android.library'
android {
// Conditional for compatibility with AGP <4.2.
if (project.android.hasProperty("namespace")) {
namespace 'io.flutter.plugins.googlesignin'
}
compileSdk 34
defaultConfig {
minSdkVersion 16
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
lintOptions {
checkAllWarnings true
warningsAsErrors true
disable 'AndroidGradlePluginVersion', 'InvalidPackage', 'GradleDependency'
}
testOptions {
unitTests.includeAndroidResources = true
unitTests.returnDefaultValues = true
unitTests.all {
testLogging {
events "passed", "skipped", "failed", "standardOut", "standardError"
outputs.upToDateWhen {false}
showStandardStreams = true
}
}
}
}
dependencies {
implementation 'com.google.android.gms:play-services-auth:21.0.0'
implementation 'com.google.guava:guava:32.0.1-android'
testImplementation 'junit:junit:4.13.2'
testImplementation 'org.mockito:mockito-inline:5.0.0'
}
| packages/packages/google_sign_in/google_sign_in_android/android/build.gradle/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_android/android/build.gradle",
"repo_id": "packages",
"token_count": 689
} | 1,066 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:google_sign_in_platform_interface/google_sign_in_platform_interface.dart';
import 'package:integration_test/integration_test.dart';
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
testWidgets('Can initialize the plugin', (WidgetTester tester) async {
final GoogleSignInPlatform signIn = GoogleSignInPlatform.instance;
expect(signIn, isNotNull);
});
testWidgets('Method channel handler is present', (WidgetTester tester) async {
// isSignedIn can be called without initialization, so use it to validate
// that the native method handler is present (e.g., that the channel name
// is correct).
final GoogleSignInPlatform signIn = GoogleSignInPlatform.instance;
await expectLater(signIn.isSignedIn(), completes);
});
}
| packages/packages/google_sign_in/google_sign_in_android/example/integration_test/google_sign_in_test.dart/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_android/example/integration_test/google_sign_in_test.dart",
"repo_id": "packages",
"token_count": 297
} | 1,067 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#if TARGET_OS_OSX
#import <FlutterMacOS/FlutterMacOS.h>
#else
#import <Flutter/Flutter.h>
#endif
#import "messages.g.h"
@interface FLTGoogleSignInPlugin : NSObject <FlutterPlugin, FSIGoogleSignInApi>
- (instancetype)init NS_UNAVAILABLE;
@end
| packages/packages/google_sign_in/google_sign_in_ios/darwin/Classes/FLTGoogleSignInPlugin.h/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_ios/darwin/Classes/FLTGoogleSignInPlugin.h",
"repo_id": "packages",
"token_count": 145
} | 1,068 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:pigeon/pigeon.dart';
@ConfigurePigeon(PigeonOptions(
dartOut: 'lib/src/messages.g.dart',
objcOptions: ObjcOptions(prefix: 'FSI'),
objcHeaderOut: 'darwin/Classes/messages.g.h',
objcSourceOut: 'darwin/Classes/messages.g.m',
copyrightHeader: 'pigeons/copyright.txt',
))
/// Pigeon version of SignInInitParams.
///
/// See SignInInitParams for details.
class InitParams {
/// The parameters to use when initializing the sign in process.
const InitParams({
this.scopes = const <String>[],
this.hostedDomain,
this.clientId,
this.serverClientId,
});
// TODO(stuartmorgan): Make the generic type non-nullable once supported.
// https://github.com/flutter/flutter/issues/97848
// The Obj-C code treats the values as non-nullable.
final List<String?> scopes;
final String? hostedDomain;
final String? clientId;
final String? serverClientId;
}
/// Pigeon version of GoogleSignInUserData.
///
/// See GoogleSignInUserData for details.
class UserData {
UserData({
required this.email,
required this.userId,
this.displayName,
this.photoUrl,
this.serverAuthCode,
this.idToken,
});
final String? displayName;
final String email;
final String userId;
final String? photoUrl;
final String? serverAuthCode;
final String? idToken;
}
/// Pigeon version of GoogleSignInTokenData.
///
/// See GoogleSignInTokenData for details.
class TokenData {
TokenData({
this.idToken,
this.accessToken,
});
final String? idToken;
final String? accessToken;
}
@HostApi()
abstract class GoogleSignInApi {
/// Initializes a sign in request with the given parameters.
@ObjCSelector('initializeSignInWithParameters:')
void init(InitParams params);
/// Starts a silent sign in.
@async
UserData signInSilently();
/// Starts a sign in with user interaction.
@async
UserData signIn();
/// Requests the access token for the current sign in.
@async
TokenData getAccessToken();
/// Signs out the current user.
void signOut();
/// Revokes scope grants to the application.
@async
void disconnect();
/// Returns whether the user is currently signed in.
bool isSignedIn();
/// Requests access to the given scopes.
@async
@ObjCSelector('requestScopes:')
bool requestScopes(List<String> scopes);
}
| packages/packages/google_sign_in/google_sign_in_ios/pigeons/messages.dart/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_ios/pigeons/messages.dart",
"repo_id": "packages",
"token_count": 814
} | 1,069 |
## 0.12.4
* Updates dependencies to `web: ^0.5.0` and `google_identity_services_web: ^0.3.1`.
## 0.12.3+3
* Updates SDK version to Dart `^3.3.0`. Flutter `^3.19.0`.
* Prepares update to package `web: ^0.5.0`.
## 0.12.3+2
* Fixes new lint warnings.
## 0.12.3+1
* Updates `FlexHtmlElementView` (the widget backing `renderButton`) to not
rely on web engine knowledge (a platform view CSS selector) to operate.
## 0.12.3
* Migrates to `package:web`.
* Updates minimum supported SDK version to Flutter 3.16.0/Dart 3.2.0.
## 0.12.2+1
* Re-publishes `0.12.2` with a small fix to the CodeClient initialization.
## 0.12.2 (withdrawn)
* Adds server auth code retrieval to google_sign_in_web.
* Adds `web_only` library to access web-only methods more easily.
## 0.12.1
* Enables FedCM on browsers that support this authentication mechanism.
* Uses the expiration timestamps of Credential and Token responses to improve
the accuracy of `isSignedIn` and `canAccessScopes` methods.
* Deprecates `signIn()` method.
* Users should migrate to `renderButton` and `silentSignIn`, as described in
the README.
## 0.12.0+5
* Migrates to `dart:ui_web` APIs.
* Updates minimum supported SDK version to Flutter 3.13.0/Dart 3.1.0.
## 0.12.0+4
* Adds pub topics to package metadata.
* Updates minimum supported SDK version to Flutter 3.7/Dart 2.19.
## 0.12.0+3
* Fixes null cast error on accounts without picture or name details.
## 0.12.0+2
* Adds compatibility with `http` 1.0.
## 0.12.0+1
* Fixes unawaited_futures violations.
## 0.12.0
* Authentication:
* Adds web-only `renderButton` method and its configuration object, as a new
authentication mechanism.
* Prepares a `userDataEvents` Stream, so the Google Sign In Button can propagate
authentication changes to the core plugin.
* **Breaking Change:** `signInSilently` now returns an authenticated (but not authorized) user.
* Authorization:
* Implements the new `canAccessScopes` method.
* Ensures that the `requestScopes` call doesn't trigger user selection when the
current user is known (similar to what `signIn` does).
* Updates minimum Flutter version to 3.3.
## 0.11.0+2
* Clarifies explanation of endorsement in README.
* Aligns Dart and Flutter SDK constraints.
## 0.11.0+1
* Updates links for the merge of flutter/plugins into flutter/packages.
## 0.11.0
* **Breaking Change:** Migrates JS-interop to `package:google_identity_services_web`
* Uses the new Google Identity Authentication and Authorization JS SDKs. [Docs](https://developers.google.com/identity).
* Added "Migrating to v0.11" section to the `README.md`.
* Updates minimum Flutter version to 3.0.
## 0.10.2+1
* Updates code for `no_leading_underscores_for_local_identifiers` lint.
* Updates minimum Flutter version to 2.10.
* Renames generated folder to js_interop.
## 0.10.2
* Migrates to new platform-interface `initWithParams` method.
* Throws when unsupported `serverClientId` option is provided.
## 0.10.1+3
* Updates references to the obsolete master branch.
## 0.10.1+2
* Minor fixes for new analysis options.
## 0.10.1+1
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 0.10.1
* Updates minimum Flutter version to 2.8.
* Passes `plugin_name` to Google Sign-In's `init` method so new applications can
continue using this plugin after April 30th 2022. Issue [#88084](https://github.com/flutter/flutter/issues/88084).
## 0.10.0+5
* Internal code cleanup for stricter analysis options.
## 0.10.0+4
* Removes dependency on `meta`.
## 0.10.0+3
* Updated URL to the `google_sign_in` package in README.
## 0.10.0+2
* Add `implements` to pubspec.
## 0.10.0+1
* Updated installation instructions in README.
## 0.10.0
* Migrate to null-safety.
## 0.9.2+1
* Update Flutter SDK constraint.
## 0.9.2
* Throw PlatformExceptions from where the GMaps SDK may throw exceptions: `init()` and `signIn()`.
* Add two new JS-interop types to be able to unwrap JS errors in release mode.
* Align the fields of the thrown PlatformExceptions with the mobile version.
* Migrate tests to run with `flutter drive`
## 0.9.1+2
* Update package:e2e reference to use the local version in the flutter/plugins
repository.
## 0.9.1+1
* Remove Android folder from `google_sign_in_web`.
## 0.9.1
* Ensure the web code returns `null` when the user is not signed in, instead of a `null-object` User. Fixes [issue 52338](https://github.com/flutter/flutter/issues/52338).
## 0.9.0
* Add support for methods introduced in `google_sign_in_platform_interface` 1.1.0.
## 0.8.4
* Remove all `fakeConstructor$` from the generated facade. JS interop classes do not support non-external constructors.
## 0.8.3+2
* Make the pedantic dev_dependency explicit.
## 0.8.3+1
* Updated documentation with more instructions about Google Sign In web setup.
## 0.8.3
* Fix initialization error that causes https://github.com/flutter/flutter/issues/48527
* Throw a PlatformException when there's an initialization problem (like wrong server-side config).
* Throw a StateError when checking .initialized before calling .init()
* Update setup instructions in the README.
## 0.8.2+1
* Add a non-op Android implementation to avoid a flaky Gradle issue.
## 0.8.2
* Require Flutter SDK 1.12.13+hotfix.4 or greater.
## 0.8.1+2
* Remove the deprecated `author:` field from pubspec.yaml
* Require Flutter SDK 1.10.0 or greater.
## 0.8.1+1
* Add missing documentation.
## 0.8.1
* Add podspec to enable compilation on iOS.
## 0.8.0
* Flutter for web initial release
| packages/packages/google_sign_in/google_sign_in_web/CHANGELOG.md/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_web/CHANGELOG.md",
"repo_id": "packages",
"token_count": 1864
} | 1,070 |
group 'io.flutter.plugins.imagepicker'
version '1.0-SNAPSHOT'
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.2.1'
}
}
rootProject.allprojects {
repositories {
google()
mavenCentral()
}
}
apply plugin: 'com.android.library'
android {
// Conditional for compatibility with AGP <4.2.
if (project.android.hasProperty("namespace")) {
namespace 'io.flutter.plugins.imagepicker'
}
compileSdk 34
defaultConfig {
minSdkVersion 16
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
lintOptions {
checkAllWarnings true
warningsAsErrors true
disable 'AndroidGradlePluginVersion', 'InvalidPackage', 'GradleDependency'
}
dependencies {
implementation 'androidx.core:core:1.10.1'
implementation 'androidx.annotation:annotation:1.7.1'
implementation 'androidx.exifinterface:exifinterface:1.3.6'
implementation 'androidx.activity:activity:1.7.2'
// org.jetbrains.kotlin:kotlin-bom artifact purpose is to align kotlin stdlib and related code versions.
// See: https://youtrack.jetbrains.com/issue/KT-55297/kotlin-stdlib-should-declare-constraints-on-kotlin-stdlib-jdk8-and-kotlin-stdlib-jdk7
implementation(platform("org.jetbrains.kotlin:kotlin-bom:1.8.22"))
testImplementation 'junit:junit:4.13.2'
testImplementation 'org.mockito:mockito-core:5.1.1'
testImplementation 'androidx.test:core:1.4.0'
testImplementation "org.robolectric:robolectric:4.10.3"
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
testOptions {
unitTests.includeAndroidResources = true
unitTests.returnDefaultValues = true
unitTests.all {
testLogging {
events "passed", "skipped", "failed", "standardOut", "standardError"
outputs.upToDateWhen {false}
showStandardStreams = true
}
}
}
}
| packages/packages/image_picker/image_picker_android/android/build.gradle/0 | {
"file_path": "packages/packages/image_picker/image_picker_android/android/build.gradle",
"repo_id": "packages",
"token_count": 943
} | 1,071 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.imagepicker;
import static java.nio.charset.StandardCharsets.UTF_8;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.when;
import static org.robolectric.Shadows.shadowOf;
import android.content.ContentProvider;
import android.content.ContentResolver;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.MatrixCursor;
import android.net.Uri;
import android.provider.MediaStore;
import android.webkit.MimeTypeMap;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.test.core.app.ApplicationProvider;
import java.io.BufferedInputStream;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.robolectric.Robolectric;
import org.robolectric.RobolectricTestRunner;
import org.robolectric.shadows.ShadowContentResolver;
import org.robolectric.shadows.ShadowMimeTypeMap;
@RunWith(RobolectricTestRunner.class)
public class FileUtilTest {
private Context context;
private FileUtils fileUtils;
ShadowContentResolver shadowContentResolver;
@Before
public void before() {
context = ApplicationProvider.getApplicationContext();
shadowContentResolver = shadowOf(context.getContentResolver());
fileUtils = new FileUtils();
ShadowMimeTypeMap mimeTypeMap = shadowOf(MimeTypeMap.getSingleton());
mimeTypeMap.addExtensionMimeTypMapping("jpg", "image/jpeg");
mimeTypeMap.addExtensionMimeTypMapping("png", "image/png");
mimeTypeMap.addExtensionMimeTypMapping("webp", "image/webp");
}
@Test
public void FileUtil_GetPathFromUri() throws IOException {
Uri uri = Uri.parse("content://dummy/dummy.png");
shadowContentResolver.registerInputStream(
uri, new ByteArrayInputStream("imageStream".getBytes(UTF_8)));
String path = fileUtils.getPathFromUri(context, uri);
File file = new File(path);
int size = (int) file.length();
byte[] bytes = new byte[size];
BufferedInputStream buf = new BufferedInputStream(new FileInputStream(file));
buf.read(bytes, 0, bytes.length);
buf.close();
assertTrue(bytes.length > 0);
String imageStream = new String(bytes, UTF_8);
assertEquals("imageStream", imageStream);
}
@Test
public void FileUtil_GetPathFromUri_securityException() throws IOException {
Uri uri = Uri.parse("content://dummy/dummy.png");
ContentResolver mockContentResolver = mock(ContentResolver.class);
when(mockContentResolver.openInputStream(any(Uri.class))).thenThrow(SecurityException.class);
Context mockContext = mock(Context.class);
when(mockContext.getContentResolver()).thenReturn(mockContentResolver);
String path = fileUtils.getPathFromUri(mockContext, uri);
assertNull(path);
}
@Test
public void FileUtil_getImageExtension() throws IOException {
Uri uri = Uri.parse("content://dummy/dummy.png");
shadowContentResolver.registerInputStream(
uri, new ByteArrayInputStream("imageStream".getBytes(UTF_8)));
String path = fileUtils.getPathFromUri(context, uri);
assertTrue(path.endsWith(".jpg"));
}
@Test
public void FileUtil_getImageName() throws IOException {
Uri uri = MockContentProvider.PNG_URI;
Robolectric.buildContentProvider(MockContentProvider.class).create("dummy");
shadowContentResolver.registerInputStream(
uri, new ByteArrayInputStream("imageStream".getBytes(UTF_8)));
String path = fileUtils.getPathFromUri(context, uri);
assertTrue(path.endsWith("a.b.png"));
}
@Test
public void FileUtil_getPathFromUri_noExtensionInBaseName() throws IOException {
Uri uri = MockContentProvider.NO_EXTENSION_URI;
Robolectric.buildContentProvider(MockContentProvider.class).create("dummy");
shadowContentResolver.registerInputStream(
uri, new ByteArrayInputStream("imageStream".getBytes(UTF_8)));
String path = fileUtils.getPathFromUri(context, uri);
assertTrue(path.endsWith("abc.png"));
}
@Test
public void FileUtil_getImageName_mismatchedType() throws IOException {
Uri uri = MockContentProvider.WEBP_URI;
Robolectric.buildContentProvider(MockContentProvider.class).create("dummy");
shadowContentResolver.registerInputStream(
uri, new ByteArrayInputStream("imageStream".getBytes(UTF_8)));
String path = fileUtils.getPathFromUri(context, uri);
assertTrue(path.endsWith("c.d.webp"));
}
@Test
public void FileUtil_getImageName_unknownType() throws IOException {
Uri uri = MockContentProvider.UNKNOWN_URI;
Robolectric.buildContentProvider(MockContentProvider.class).create("dummy");
shadowContentResolver.registerInputStream(
uri, new ByteArrayInputStream("imageStream".getBytes(UTF_8)));
String path = fileUtils.getPathFromUri(context, uri);
assertTrue(path.endsWith("e.f.g"));
}
private static class MockContentProvider extends ContentProvider {
public static final Uri PNG_URI = Uri.parse("content://dummy/a.b.png");
public static final Uri WEBP_URI = Uri.parse("content://dummy/c.d.png");
public static final Uri UNKNOWN_URI = Uri.parse("content://dummy/e.f.g");
public static final Uri NO_EXTENSION_URI = Uri.parse("content://dummy/abc");
@Override
public boolean onCreate() {
return true;
}
@Nullable
@Override
public Cursor query(
@NonNull Uri uri,
@Nullable String[] projection,
@Nullable String selection,
@Nullable String[] selectionArgs,
@Nullable String sortOrder) {
MatrixCursor cursor = new MatrixCursor(new String[] {MediaStore.MediaColumns.DISPLAY_NAME});
cursor.addRow(new Object[] {uri.getLastPathSegment()});
return cursor;
}
@Nullable
@Override
public String getType(@NonNull Uri uri) {
if (uri.equals(PNG_URI)) return "image/png";
if (uri.equals(WEBP_URI)) return "image/webp";
if (uri.equals(NO_EXTENSION_URI)) return "image/png";
return null;
}
@Nullable
@Override
public Uri insert(@NonNull Uri uri, @Nullable ContentValues values) {
return null;
}
@Override
public int delete(
@NonNull Uri uri, @Nullable String selection, @Nullable String[] selectionArgs) {
return 0;
}
@Override
public int update(
@NonNull Uri uri,
@Nullable ContentValues values,
@Nullable String selection,
@Nullable String[] selectionArgs) {
return 0;
}
}
}
| packages/packages/image_picker/image_picker_android/android/src/test/java/io/flutter/plugins/imagepicker/FileUtilTest.java/0 | {
"file_path": "packages/packages/image_picker/image_picker_android/android/src/test/java/io/flutter/plugins/imagepicker/FileUtilTest.java",
"repo_id": "packages",
"token_count": 2446
} | 1,072 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.imagepickerexample;
import static androidx.test.espresso.flutter.EspressoFlutter.onFlutterWidget;
import static androidx.test.espresso.flutter.action.FlutterActions.click;
import static androidx.test.espresso.flutter.assertion.FlutterAssertions.matches;
import static androidx.test.espresso.flutter.matcher.FlutterMatchers.withText;
import static androidx.test.espresso.flutter.matcher.FlutterMatchers.withValueKey;
import static androidx.test.espresso.intent.Intents.intended;
import static androidx.test.espresso.intent.Intents.intending;
import static androidx.test.espresso.intent.matcher.IntentMatchers.hasAction;
import android.app.Activity;
import android.app.Instrumentation;
import android.content.Intent;
import android.net.Uri;
import androidx.test.espresso.intent.rule.IntentsTestRule;
import org.junit.Ignore;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.TestRule;
public class ImagePickerPickTest {
@Rule public TestRule rule = new IntentsTestRule<>(DriverExtensionActivity.class);
@Test
@Ignore("Doesn't run in Firebase Test Lab: https://github.com/flutter/flutter/issues/94748")
public void imageIsPickedWithOriginalName() {
Instrumentation.ActivityResult result =
new Instrumentation.ActivityResult(
Activity.RESULT_OK, new Intent().setData(Uri.parse("content://dummy/dummy.png")));
intending(hasAction(Intent.ACTION_GET_CONTENT)).respondWith(result);
onFlutterWidget(withValueKey("image_picker_example_from_gallery")).perform(click());
onFlutterWidget(withText("PICK")).perform(click());
intended(hasAction(Intent.ACTION_GET_CONTENT));
onFlutterWidget(withValueKey("image_picker_example_picked_image_name"))
.check(matches(withText("dummy.png")));
}
}
| packages/packages/image_picker/image_picker_android/example/android/app/src/androidTest/java/io/flutter/plugins/imagepickerexample/ImagePickerPickTest.java/0 | {
"file_path": "packages/packages/image_picker/image_picker_android/example/android/app/src/androidTest/java/io/flutter/plugins/imagepickerexample/ImagePickerPickTest.java",
"repo_id": "packages",
"token_count": 640
} | 1,073 |
# image\_picker\_for\_web
A web implementation of [`image_picker`][1].
## Limitations on the web platform
### `XFile`
This plugin uses `XFile` objects to abstract files picked/created by the user.
Read more about `XFile` on the web in
[`package:cross_file`'s README](https://pub.dev/packages/cross_file).
### input file "accept"
In order to filter only video/image content, some browsers offer an [`accept` attribute](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/accept) in their `input type="file"` form elements:
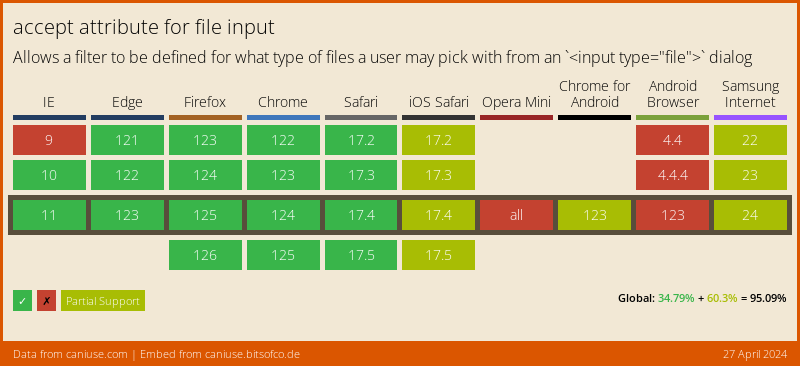
This feature is just a convenience for users, **not validation**.
Users can override this setting on their browsers. You must validate in your app (or server)
that the user has picked the file type that you can handle.
### input file "capture"
In order to "take a photo", some mobile browsers offer a [`capture` attribute](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/capture):
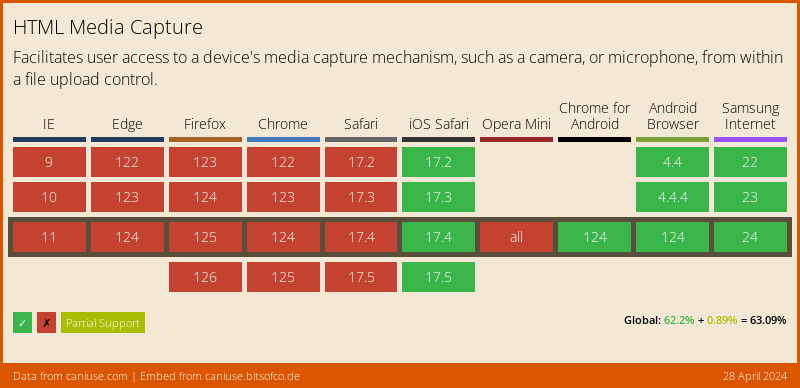
Each browser may implement `capture` any way they please, so it may (or may not) make a
difference in your users' experience.
### input file "cancel"
The [`cancel` event](https://caniuse.com/mdn-api_htmlinputelement_cancel_event)
used by the plugin to detect when users close the file selector without picking
a file is relatively new, and will only work in recent browsers.
### `ImagePickerOptions` support
The `ImagePickerOptions` configuration object allows passing resize (`maxWidth`,
`maxHeight`) and quality (`imageQuality`) parameters to some methods of this
plugin, which in other platforms control how selected images are resized or
re-encoded.
On the web:
* `maxWidth`, `maxHeight` and `imageQuality` are not supported for `gif` images.
* `imageQuality` only affects `jpg` and `webp` images.
### `getVideo()`
The argument `maxDuration` is not supported on the web.
## Usage
### Import the package
This package is [endorsed](https://flutter.dev/docs/development/packages-and-plugins/developing-packages#endorsed-federated-plugin),
which means you can simply use `image_picker`
normally. This package will be automatically included in your app when you do,
so you do not need to add it to your `pubspec.yaml`.
However, if you `import` this package to use any of its APIs directly, you
should add it to your `pubspec.yaml` as usual.
### Use the plugin
You should be able to use `package:image_picker` _almost_ as normal.
Once the user has picked a file, the returned `XFile` instance will contain a
`network`-accessible `Blob` URL (pointing to a location within the browser).
The instance will also let you retrieve the bytes of the selected file across all platforms.
If you want to use the path directly, your code would need look like this:
```dart
...
if (kIsWeb) {
Image.network(pickedFile.path);
} else {
Image.file(File(pickedFile.path));
}
...
```
Or, using bytes:
```dart
...
Image.memory(await pickedFile.readAsBytes())
...
```
[1]: https://pub.dev/packages/image_picker
| packages/packages/image_picker/image_picker_for_web/README.md/0 | {
"file_path": "packages/packages/image_picker/image_picker_for_web/README.md",
"repo_id": "packages",
"token_count": 971
} | 1,074 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import <XCTest/XCTest.h>
#import <os/log.h>
const int kLimitedElementWaitingTime = 30;
@interface ImagePickerFromLimitedGalleryUITests : XCTestCase
@property(nonatomic, strong) XCUIApplication *app;
@property(nonatomic, assign) BOOL interceptedPermissionInterruption;
@end
@implementation ImagePickerFromLimitedGalleryUITests
- (void)setUp {
[super setUp];
self.continueAfterFailure = NO;
self.app = [[XCUIApplication alloc] init];
if (@available(iOS 13.4, *)) {
// Reset the authorization status for Photos to test permission popups
[self.app resetAuthorizationStatusForResource:XCUIProtectedResourcePhotos];
}
[self.app launch];
__weak typeof(self) weakSelf = self;
[self addUIInterruptionMonitorWithDescription:@"Permission popups"
handler:^BOOL(XCUIElement *_Nonnull interruptingElement) {
XCUIElement *limitedPhotoPermission =
[interruptingElement.buttons elementBoundByIndex:0];
if (![limitedPhotoPermission
waitForExistenceWithTimeout:
kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@",
weakSelf.app.debugDescription);
XCTFail(@"Failed due to not able to find "
@"selectPhotos button with %@ seconds",
@(kLimitedElementWaitingTime));
}
[limitedPhotoPermission tap];
weakSelf.interceptedPermissionInterruption = YES;
return YES;
}];
}
- (void)tearDown {
[super tearDown];
[self.app terminate];
}
- (void)handlePermissionInterruption {
// addUIInterruptionMonitorWithDescription is only invoked when trying to interact with an element
// (the app in this case) the alert is blocking. We expect a permission popup here so do a swipe
// up action (which should be harmless).
[self.app swipeUp];
if (@available(iOS 17, *)) {
// addUIInterruptionMonitorWithDescription does not work consistently on Xcode 15 simulators, so
// use a backup method of accepting permissions popup.
if (self.interceptedPermissionInterruption == YES) {
return;
}
// If cancel button exists, permission has already been given.
XCUIElement *cancelButton = self.app.buttons[@"Cancel"].firstMatch;
if ([cancelButton waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
return;
}
XCUIApplication *springboardApp =
[[XCUIApplication alloc] initWithBundleIdentifier:@"com.apple.springboard"];
XCUIElement *allowButton = springboardApp.buttons[@"Limit Access…"];
if (![allowButton waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@", self.app.debugDescription);
XCTFail(@"Failed due to not able to find Limit Access button with %@ seconds",
@(kLimitedElementWaitingTime));
}
[allowButton tap];
}
}
// Test the `Select Photos` button which is available after iOS 14.
- (void)testSelectingFromGallery API_AVAILABLE(ios(14)) {
// Find and tap on the pick from gallery button.
XCUIElement *imageFromGalleryButton =
self.app.otherElements[@"image_picker_example_from_gallery"].firstMatch;
if (![imageFromGalleryButton waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@", self.app.debugDescription);
XCTFail(@"Failed due to not able to find image from gallery button with %@ seconds",
@(kLimitedElementWaitingTime));
}
[imageFromGalleryButton tap];
// Find and tap on the `pick` button.
XCUIElement *pickButton = self.app.buttons[@"PICK"].firstMatch;
if (![pickButton waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@", self.app.debugDescription);
XCTSkip(@"Pick button isn't found so the test is skipped...");
}
[pickButton tap];
[self handlePermissionInterruption];
// Find an image and tap on it.
XCUIElement *aImage = [self.app.scrollViews.firstMatch.images elementBoundByIndex:1];
os_log_error(OS_LOG_DEFAULT, "description before picking image %@", self.app.debugDescription);
if (![aImage waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@", self.app.debugDescription);
XCTFail(@"Failed due to not able to find an image with %@ seconds",
@(kLimitedElementWaitingTime));
}
[aImage tap];
// Find and tap on the `Done` button.
XCUIElement *doneButton = self.app.buttons[@"Done"].firstMatch;
if (![doneButton waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@", self.app.debugDescription);
XCTSkip(@"Permissions popup could not fired so the test is skipped...");
}
[doneButton tap];
// Find an image and tap on it to have access to selected photos.
aImage = [self.app.scrollViews.firstMatch.images elementBoundByIndex:1];
os_log_error(OS_LOG_DEFAULT, "description before picking image %@", self.app.debugDescription);
if (![aImage waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@", self.app.debugDescription);
XCTFail(@"Failed due to not able to find an image with %@ seconds",
@(kLimitedElementWaitingTime));
}
[aImage tap];
// Find the picked image.
XCUIElement *pickedImage = self.app.images[@"image_picker_example_picked_image"].firstMatch;
if (![pickedImage waitForExistenceWithTimeout:kLimitedElementWaitingTime]) {
os_log_error(OS_LOG_DEFAULT, "%@", self.app.debugDescription);
XCTFail(@"Failed due to not able to find pickedImage with %@ seconds",
@(kLimitedElementWaitingTime));
}
}
@end
| packages/packages/image_picker/image_picker_ios/example/ios/RunnerUITests/ImagePickerFromLimitedGalleryUITests.m/0 | {
"file_path": "packages/packages/image_picker/image_picker_ios/example/ios/RunnerUITests/ImagePickerFromLimitedGalleryUITests.m",
"repo_id": "packages",
"token_count": 2591
} | 1,075 |
name: image_picker_example
description: Demonstrates how to use the image_picker plugin.
publish_to: none
environment:
sdk: ^3.2.3
flutter: ">=3.16.6"
dependencies:
flutter:
sdk: flutter
image_picker_ios:
# When depending on this package from a real application you should use:
# image_picker_ios: ^x.y.z
# See https://dart.dev/tools/pub/dependencies#version-constraints
# The example app is bundled with the plugin so we use a path dependency on
# the parent directory to use the current plugin's version.
path: ../
image_picker_platform_interface: ^2.8.0
mime: ^1.0.4
video_player: ^2.1.4
dev_dependencies:
flutter_test:
sdk: flutter
integration_test:
sdk: flutter
flutter:
uses-material-design: true
| packages/packages/image_picker/image_picker_ios/example/pubspec.yaml/0 | {
"file_path": "packages/packages/image_picker/image_picker_ios/example/pubspec.yaml",
"repo_id": "packages",
"token_count": 288
} | 1,076 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v13.0.0), do not edit directly.
// See also: https://pub.dev/packages/pigeon
#import <Foundation/Foundation.h>
@protocol FlutterBinaryMessenger;
@protocol FlutterMessageCodec;
@class FlutterError;
@class FlutterStandardTypedData;
NS_ASSUME_NONNULL_BEGIN
typedef NS_ENUM(NSUInteger, FLTSourceCamera) {
FLTSourceCameraRear = 0,
FLTSourceCameraFront = 1,
};
/// Wrapper for FLTSourceCamera to allow for nullability.
@interface FLTSourceCameraBox : NSObject
@property(nonatomic, assign) FLTSourceCamera value;
- (instancetype)initWithValue:(FLTSourceCamera)value;
@end
typedef NS_ENUM(NSUInteger, FLTSourceType) {
FLTSourceTypeCamera = 0,
FLTSourceTypeGallery = 1,
};
/// Wrapper for FLTSourceType to allow for nullability.
@interface FLTSourceTypeBox : NSObject
@property(nonatomic, assign) FLTSourceType value;
- (instancetype)initWithValue:(FLTSourceType)value;
@end
@class FLTMaxSize;
@class FLTMediaSelectionOptions;
@class FLTSourceSpecification;
@interface FLTMaxSize : NSObject
+ (instancetype)makeWithWidth:(nullable NSNumber *)width height:(nullable NSNumber *)height;
@property(nonatomic, strong, nullable) NSNumber *width;
@property(nonatomic, strong, nullable) NSNumber *height;
@end
@interface FLTMediaSelectionOptions : NSObject
/// `init` unavailable to enforce nonnull fields, see the `make` class method.
- (instancetype)init NS_UNAVAILABLE;
+ (instancetype)makeWithMaxSize:(FLTMaxSize *)maxSize
imageQuality:(nullable NSNumber *)imageQuality
requestFullMetadata:(BOOL)requestFullMetadata
allowMultiple:(BOOL)allowMultiple;
@property(nonatomic, strong) FLTMaxSize *maxSize;
@property(nonatomic, strong, nullable) NSNumber *imageQuality;
@property(nonatomic, assign) BOOL requestFullMetadata;
@property(nonatomic, assign) BOOL allowMultiple;
@end
@interface FLTSourceSpecification : NSObject
/// `init` unavailable to enforce nonnull fields, see the `make` class method.
- (instancetype)init NS_UNAVAILABLE;
+ (instancetype)makeWithType:(FLTSourceType)type camera:(FLTSourceCamera)camera;
@property(nonatomic, assign) FLTSourceType type;
@property(nonatomic, assign) FLTSourceCamera camera;
@end
/// The codec used by FLTImagePickerApi.
NSObject<FlutterMessageCodec> *FLTImagePickerApiGetCodec(void);
@protocol FLTImagePickerApi
- (void)pickImageWithSource:(FLTSourceSpecification *)source
maxSize:(FLTMaxSize *)maxSize
quality:(nullable NSNumber *)imageQuality
fullMetadata:(BOOL)requestFullMetadata
completion:(void (^)(NSString *_Nullable, FlutterError *_Nullable))completion;
- (void)pickMultiImageWithMaxSize:(FLTMaxSize *)maxSize
quality:(nullable NSNumber *)imageQuality
fullMetadata:(BOOL)requestFullMetadata
completion:(void (^)(NSArray<NSString *> *_Nullable,
FlutterError *_Nullable))completion;
- (void)pickVideoWithSource:(FLTSourceSpecification *)source
maxDuration:(nullable NSNumber *)maxDurationSeconds
completion:(void (^)(NSString *_Nullable, FlutterError *_Nullable))completion;
/// Selects images and videos and returns their paths.
- (void)pickMediaWithMediaSelectionOptions:(FLTMediaSelectionOptions *)mediaSelectionOptions
completion:(void (^)(NSArray<NSString *> *_Nullable,
FlutterError *_Nullable))completion;
@end
extern void SetUpFLTImagePickerApi(id<FlutterBinaryMessenger> binaryMessenger,
NSObject<FLTImagePickerApi> *_Nullable api);
NS_ASSUME_NONNULL_END
| packages/packages/image_picker/image_picker_ios/ios/Classes/messages.g.h/0 | {
"file_path": "packages/packages/image_picker/image_picker_ios/ios/Classes/messages.g.h",
"repo_id": "packages",
"token_count": 1495
} | 1,077 |
# image\_picker\_macos
A macOS implementation of [`image_picker`][1].
## Limitations
`ImageSource.camera` is not supported unless a `cameraDelegate` is set.
### pickImage()
The arguments `maxWidth`, `maxHeight`, and `imageQuality` are not currently supported.
### pickVideo()
The argument `maxDuration` is not currently supported.
## Usage
### Import the package
This package is [endorsed][2], which means you can simply use `file_selector`
normally. This package will be automatically included in your app when you do,
so you do not need to add it to your `pubspec.yaml`.
However, if you `import` this package to use any of its APIs directly, you
should add it to your `pubspec.yaml` as usual.
### Entitlements
This package is currently implemented using [`file_selector`][3], so you will
need to add a read-only file acces [entitlement][4]:
```xml
<key>com.apple.security.files.user-selected.read-only</key>
<true/>
```
[1]: https://pub.dev/packages/image_picker
[2]: https://flutter.dev/docs/development/packages-and-plugins/developing-packages#endorsed-federated-plugin
[3]: https://pub.dev/packages/file_selector
[4]: https://docs.flutter.dev/platform-integration/macos/building#entitlements-and-the-app-sandbox
| packages/packages/image_picker/image_picker_macos/README.md/0 | {
"file_path": "packages/packages/image_picker/image_picker_macos/README.md",
"repo_id": "packages",
"token_count": 390
} | 1,078 |
name: image_picker_macos
description: macOS platform implementation of image_picker
repository: https://github.com/flutter/packages/tree/main/packages/image_picker/image_picker_macos
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+image_picker%22
version: 0.2.1+1
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
flutter:
plugin:
implements: image_picker
platforms:
macos:
dartPluginClass: ImagePickerMacOS
dependencies:
file_selector_macos: ^0.9.1+1
file_selector_platform_interface: ^2.3.0
flutter:
sdk: flutter
image_picker_platform_interface: ^2.8.0
dev_dependencies:
build_runner: ^2.1.5
flutter_test:
sdk: flutter
mockito: 5.4.4
topics:
- image-picker
- files
- file-selection
| packages/packages/image_picker/image_picker_macos/pubspec.yaml/0 | {
"file_path": "packages/packages/image_picker/image_picker_macos/pubspec.yaml",
"repo_id": "packages",
"token_count": 334
} | 1,079 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The type of media to allow the user to select with [ImagePickerPlatform.getMedia].
enum MediaSelectionType {
/// Static pictures.
image,
/// Videos.
video,
}
| packages/packages/image_picker/image_picker_platform_interface/lib/src/types/media_selection_type.dart/0 | {
"file_path": "packages/packages/image_picker/image_picker_platform_interface/lib/src/types/media_selection_type.dart",
"repo_id": "packages",
"token_count": 92
} | 1,080 |
org.gradle.jvmargs=-Xmx4G
android.useAndroidX=true
android.enableJetifier=true
| packages/packages/in_app_purchase/in_app_purchase/example/android/gradle.properties/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase/example/android/gradle.properties",
"repo_id": "packages",
"token_count": 30
} | 1,081 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:in_app_purchase_android/in_app_purchase_android.dart';
import 'package:in_app_purchase_platform_interface/in_app_purchase_platform_interface.dart';
import 'package:in_app_purchase_storekit/in_app_purchase_storekit.dart';
export 'package:in_app_purchase_platform_interface/in_app_purchase_platform_interface.dart'
show
IAPError,
InAppPurchaseException,
ProductDetails,
ProductDetailsResponse,
PurchaseDetails,
PurchaseParam,
PurchaseStatus,
PurchaseVerificationData;
/// Basic API for making in app purchases across multiple platforms.
class InAppPurchase implements InAppPurchasePlatformAdditionProvider {
InAppPurchase._();
static InAppPurchase? _instance;
/// The instance of the [InAppPurchase] to use.
static InAppPurchase get instance => _getOrCreateInstance();
static InAppPurchase _getOrCreateInstance() {
if (_instance != null) {
return _instance!;
}
if (defaultTargetPlatform == TargetPlatform.android) {
InAppPurchaseAndroidPlatform.registerPlatform();
} else if (defaultTargetPlatform == TargetPlatform.iOS ||
defaultTargetPlatform == TargetPlatform.macOS) {
InAppPurchaseStoreKitPlatform.registerPlatform();
}
_instance = InAppPurchase._();
return _instance!;
}
@override
T getPlatformAddition<T extends InAppPurchasePlatformAddition?>() {
return InAppPurchasePlatformAddition.instance as T;
}
/// Listen to this broadcast stream to get real time update for purchases.
///
/// This stream will never close as long as the app is active.
///
/// Purchase updates can happen in several situations:
/// * When a purchase is triggered by user in the app.
/// * When a purchase is triggered by user from the platform-specific store front.
/// * When a purchase is restored on the device by the user in the app.
/// * If a purchase is not completed ([completePurchase] is not called on the
/// purchase object) from the last app session. Purchase updates will happen
/// when a new app session starts instead.
///
/// IMPORTANT! You must subscribe to this stream as soon as your app launches,
/// preferably before returning your main App Widget in main(). Otherwise you
/// will miss purchase updated made before this stream is subscribed to.
///
/// We also recommend listening to the stream with one subscription at a given
/// time. If you choose to have multiple subscription at the same time, you
/// should be careful at the fact that each subscription will receive all the
/// events after they start to listen.
Stream<List<PurchaseDetails>> get purchaseStream =>
InAppPurchasePlatform.instance.purchaseStream;
/// Returns `true` if the payment platform is ready and available.
Future<bool> isAvailable() => InAppPurchasePlatform.instance.isAvailable();
/// Query product details for the given set of IDs.
///
/// Identifiers in the underlying payment platform, for example, [App Store
/// Connect](https://appstoreconnect.apple.com/) for iOS and [Google Play
/// Console](https://play.google.com/) for Android.
Future<ProductDetailsResponse> queryProductDetails(Set<String> identifiers) =>
InAppPurchasePlatform.instance.queryProductDetails(identifiers);
/// Buy a non consumable product or subscription.
///
/// Non consumable items can only be bought once. For example, a purchase that
/// unlocks a special content in your app. Subscriptions are also non
/// consumable products.
///
/// You always need to restore all the non consumable products for user when
/// they switch their phones.
///
/// This method does not return the result of the purchase. Instead, after
/// triggering this method, purchase updates will be sent to
/// [purchaseStream]. You should [Stream.listen] to [purchaseStream] to get
/// [PurchaseDetails] objects in different [PurchaseDetails.status] and update
/// your UI accordingly. When the [PurchaseDetails.status] is
/// [PurchaseStatus.purchased], [PurchaseStatus.restored] or
/// [PurchaseStatus.error] you should deliver the content or handle the error,
/// then call [completePurchase] to finish the purchasing process.
///
/// This method does return whether or not the purchase request was initially
/// sent successfully.
///
/// Consumable items are defined differently by the different underlying
/// payment platforms, and there's no way to query for whether or not the
/// [ProductDetail] is a consumable at runtime.
///
/// See also:
///
/// * [buyConsumable], for buying a consumable product.
/// * [restorePurchases], for restoring non consumable products.
///
/// Calling this method for consumable items will cause unwanted behaviors!
Future<bool> buyNonConsumable({required PurchaseParam purchaseParam}) =>
InAppPurchasePlatform.instance.buyNonConsumable(
purchaseParam: purchaseParam,
);
/// Buy a consumable product.
///
/// Consumable items can be "consumed" to mark that they've been used and then
/// bought additional times. For example, a health potion.
///
/// To restore consumable purchases across devices, you should keep track of
/// those purchase on your own server and restore the purchase for your users.
/// Consumed products are no longer considered to be "owned" by payment
/// platforms and will not be delivered by calling [restorePurchases].
///
/// Consumable items are defined differently by the different underlying
/// payment platforms, and there's no way to query for whether or not the
/// [ProductDetail] is a consumable at runtime.
///
/// `autoConsume` is provided as a utility and will instruct the plugin to
/// automatically consume the product after a succesful purchase.
/// `autoConsume` is `true` by default.
///
/// This method does not return the result of the purchase. Instead, after
/// triggering this method, purchase updates will be sent to
/// [purchaseStream]. You should [Stream.listen] to
/// [purchaseStream] to get [PurchaseDetails] objects in different
/// [PurchaseDetails.status] and update your UI accordingly. When the
/// [PurchaseDetails.status] is [PurchaseStatus.purchased] or
/// [PurchaseStatus.error], you should deliver the content or handle the
/// error, then call [completePurchase] to finish the purchasing process.
///
/// This method does return whether or not the purchase request was initially
/// sent succesfully.
///
/// See also:
///
/// * [buyNonConsumable], for buying a non consumable product or
/// subscription.
/// * [restorePurchases], for restoring non consumable products.
///
/// Calling this method for non consumable items will cause unwanted
/// behaviors!
Future<bool> buyConsumable({
required PurchaseParam purchaseParam,
bool autoConsume = true,
}) =>
InAppPurchasePlatform.instance.buyConsumable(
purchaseParam: purchaseParam,
autoConsume: autoConsume,
);
/// Mark that purchased content has been delivered to the user.
///
/// You are responsible for completing every [PurchaseDetails] whose
/// [PurchaseDetails.status] is [PurchaseStatus.purchased] or
/// [PurchaseStatus.restored].
/// Completing a [PurchaseStatus.pending] purchase will cause an exception.
/// For convenience, [PurchaseDetails.pendingCompletePurchase] indicates if a
/// purchase is pending for completion.
///
/// The method will throw a [PurchaseException] when the purchase could not be
/// finished. Depending on the [PurchaseException.errorCode] the developer
/// should try to complete the purchase via this method again, or retry the
/// [completePurchase] method at a later time. If the
/// [PurchaseException.errorCode] indicates you should not retry there might
/// be some issue with the app's code or the configuration of the app in the
/// respective store. The developer is responsible to fix this issue. The
/// [PurchaseException.message] field might provide more information on what
/// went wrong.
Future<void> completePurchase(PurchaseDetails purchase) =>
InAppPurchasePlatform.instance.completePurchase(purchase);
/// Restore all previous purchases.
///
/// The `applicationUserName` should match whatever was sent in the initial
/// `PurchaseParam`, if anything. If no `applicationUserName` was specified in the initial
/// `PurchaseParam`, use `null`.
///
/// Restored purchases are delivered through the [purchaseStream] with a
/// status of [PurchaseStatus.restored]. You should listen for these purchases,
/// validate their receipts, deliver the content and mark the purchase complete
/// by calling the [completePurchase] method for each purchase.
///
/// This does not return consumed products. If you want to restore unused
/// consumable products, you need to persist consumable product information
/// for your user on your own server.
///
/// See also:
///
/// * [refreshPurchaseVerificationData], for reloading failed
/// [PurchaseDetails.verificationData].
Future<void> restorePurchases({String? applicationUserName}) =>
InAppPurchasePlatform.instance.restorePurchases(
applicationUserName: applicationUserName,
);
}
| packages/packages/in_app_purchase/in_app_purchase/lib/in_app_purchase.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase/lib/in_app_purchase.dart",
"repo_id": "packages",
"token_count": 2535
} | 1,082 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
export 'src/billing_client_wrappers/alternative_billing_only_reporting_details_wrapper.dart';
export 'src/billing_client_wrappers/billing_client_manager.dart';
export 'src/billing_client_wrappers/billing_client_wrapper.dart';
export 'src/billing_client_wrappers/billing_response_wrapper.dart';
export 'src/billing_client_wrappers/one_time_purchase_offer_details_wrapper.dart';
export 'src/billing_client_wrappers/product_details_wrapper.dart';
export 'src/billing_client_wrappers/product_wrapper.dart';
export 'src/billing_client_wrappers/purchase_wrapper.dart';
export 'src/billing_client_wrappers/subscription_offer_details_wrapper.dart';
export 'src/billing_client_wrappers/user_choice_details_wrapper.dart';
| packages/packages/in_app_purchase/in_app_purchase_android/lib/billing_client_wrappers.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_android/lib/billing_client_wrappers.dart",
"repo_id": "packages",
"token_count": 282
} | 1,083 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:json_annotation/json_annotation.dart';
import '../../billing_client_wrappers.dart';
// WARNING: Changes to `@JsonSerializable` classes need to be reflected in the
// below generated file. Run `flutter packages pub run build_runner watch` to
// rebuild and watch for further changes.
part 'product_wrapper.g.dart';
/// Dart wrapper around [`com.android.billingclient.api.Product`](https://developer.android.com/reference/com/android/billingclient/api/QueryProductDetailsParams.Product).
@JsonSerializable(createToJson: true)
@immutable
class ProductWrapper {
/// Creates a new [ProductWrapper].
const ProductWrapper({
required this.productId,
required this.productType,
});
/// Creates a JSON representation of this product.
Map<String, dynamic> toJson() => _$ProductWrapperToJson(this);
/// The product identifier.
@JsonKey(defaultValue: '')
final String productId;
/// The product type.
final ProductType productType;
@override
bool operator ==(Object other) {
if (identical(other, this)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ProductWrapper &&
other.productId == productId &&
other.productType == productType;
}
@override
int get hashCode => Object.hash(productId, productType);
}
| packages/packages/in_app_purchase/in_app_purchase_android/lib/src/billing_client_wrappers/product_wrapper.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_android/lib/src/billing_client_wrappers/product_wrapper.dart",
"repo_id": "packages",
"token_count": 486
} | 1,084 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:in_app_purchase_platform_interface/in_app_purchase_platform_interface.dart';
import 'types.dart';
/// The response object for fetching the past purchases.
///
/// An instance of this class is returned in [InAppPurchaseConnection.queryPastPurchases].
class QueryPurchaseDetailsResponse {
/// Creates a new [QueryPurchaseDetailsResponse] object with the provider information.
QueryPurchaseDetailsResponse({required this.pastPurchases, this.error});
/// A list of successfully fetched past purchases.
///
/// If there are no past purchases, or there is an [error] fetching past purchases,
/// this variable is an empty List.
/// You should verify the purchase data using [PurchaseDetails.verificationData] before using the [PurchaseDetails] object.
final List<GooglePlayPurchaseDetails> pastPurchases;
/// The error when fetching past purchases.
///
/// If the fetch is successful, the value is `null`.
final IAPError? error;
}
| packages/packages/in_app_purchase/in_app_purchase_android/lib/src/types/query_purchase_details_response.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_android/lib/src/types/query_purchase_details_response.dart",
"repo_id": "packages",
"token_count": 292
} | 1,085 |
name: in_app_purchase_platform_interface
description: A common platform interface for the in_app_purchase plugin.
repository: https://github.com/flutter/packages/tree/main/packages/in_app_purchase/in_app_purchase_platform_interface
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+in_app_purchase%22
# NOTE: We strongly prefer non-breaking changes, even at the expense of a
# less-clean API. See https://flutter.dev/go/platform-interface-breaking-changes
version: 1.3.7
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
dependencies:
flutter:
sdk: flutter
plugin_platform_interface: ^2.1.7
dev_dependencies:
flutter_test:
sdk: flutter
mockito: 5.4.4
topics:
- in-app-purchase
- payment
| packages/packages/in_app_purchase/in_app_purchase_platform_interface/pubspec.yaml/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_platform_interface/pubspec.yaml",
"repo_id": "packages",
"token_count": 285
} | 1,086 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import <XCTest/XCTest.h>
#import "Stubs.h"
@import in_app_purchase_storekit;
#pragma tests start here
@interface RequestHandlerTest : XCTestCase
@end
@implementation RequestHandlerTest
- (void)testRequestHandlerWithProductRequestSuccess {
SKProductRequestStub *request =
[[SKProductRequestStub alloc] initWithProductIdentifiers:[NSSet setWithArray:@[ @"123" ]]];
FIAPRequestHandler *handler = [[FIAPRequestHandler alloc] initWithRequest:request];
XCTestExpectation *expectation =
[self expectationWithDescription:@"expect to get response with 1 product"];
__block SKProductsResponse *response;
[handler
startProductRequestWithCompletionHandler:^(SKProductsResponse *_Nullable r, NSError *error) {
response = r;
[expectation fulfill];
}];
[self waitForExpectations:@[ expectation ] timeout:5];
XCTAssertNotNil(response);
XCTAssertEqual(response.products.count, 1);
SKProduct *product = response.products.firstObject;
XCTAssertTrue([product.productIdentifier isEqualToString:@"123"]);
}
- (void)testRequestHandlerWithProductRequestFailure {
SKProductRequestStub *request = [[SKProductRequestStub alloc]
initWithFailureError:[NSError errorWithDomain:@"test" code:123 userInfo:@{}]];
FIAPRequestHandler *handler = [[FIAPRequestHandler alloc] initWithRequest:request];
XCTestExpectation *expectation =
[self expectationWithDescription:@"expect to get response with 1 product"];
__block NSError *error;
__block SKProductsResponse *response;
[handler startProductRequestWithCompletionHandler:^(SKProductsResponse *_Nullable r, NSError *e) {
error = e;
response = r;
[expectation fulfill];
}];
[self waitForExpectations:@[ expectation ] timeout:5];
XCTAssertNotNil(error);
XCTAssertEqual(error.domain, @"test");
XCTAssertNil(response);
}
- (void)testRequestHandlerWithRefreshReceiptSuccess {
SKReceiptRefreshRequestStub *request =
[[SKReceiptRefreshRequestStub alloc] initWithReceiptProperties:nil];
FIAPRequestHandler *handler = [[FIAPRequestHandler alloc] initWithRequest:request];
XCTestExpectation *expectation = [self expectationWithDescription:@"expect no error"];
__block NSError *e;
[handler
startProductRequestWithCompletionHandler:^(SKProductsResponse *_Nullable r, NSError *error) {
e = error;
[expectation fulfill];
}];
[self waitForExpectations:@[ expectation ] timeout:5];
XCTAssertNil(e);
}
- (void)testRequestHandlerWithRefreshReceiptFailure {
SKReceiptRefreshRequestStub *request = [[SKReceiptRefreshRequestStub alloc]
initWithFailureError:[NSError errorWithDomain:@"test" code:123 userInfo:@{}]];
FIAPRequestHandler *handler = [[FIAPRequestHandler alloc] initWithRequest:request];
XCTestExpectation *expectation = [self expectationWithDescription:@"expect error"];
__block NSError *error;
__block SKProductsResponse *response;
[handler startProductRequestWithCompletionHandler:^(SKProductsResponse *_Nullable r, NSError *e) {
error = e;
response = r;
[expectation fulfill];
}];
[self waitForExpectations:@[ expectation ] timeout:5];
XCTAssertNotNil(error);
XCTAssertEqual(error.domain, @"test");
XCTAssertNil(response);
}
@end
| packages/packages/in_app_purchase/in_app_purchase_storekit/example/shared/RunnerTests/ProductRequestHandlerTests.m/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_storekit/example/shared/RunnerTests/ProductRequestHandlerTests.m",
"repo_id": "packages",
"token_count": 1158
} | 1,087 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:json_annotation/json_annotation.dart';
import '../messages.g.dart';
import 'enum_converters.dart';
import 'sk_payment_queue_wrapper.dart';
import 'sk_product_wrapper.dart';
part 'sk_payment_transaction_wrappers.g.dart';
/// Callback handlers for transaction status changes.
///
/// Must be subclassed. Must be instantiated and added to the
/// [SKPaymentQueueWrapper] via [SKPaymentQueueWrapper.setTransactionObserver]
/// at app launch.
///
/// This class is a Dart wrapper around [SKTransactionObserver](https://developer.apple.com/documentation/storekit/skpaymenttransactionobserver?language=objc).
abstract class SKTransactionObserverWrapper {
/// Triggered when any transactions are updated.
void updatedTransactions(
{required List<SKPaymentTransactionWrapper> transactions});
/// Triggered when any transactions are removed from the payment queue.
void removedTransactions(
{required List<SKPaymentTransactionWrapper> transactions});
/// Triggered when there is an error while restoring transactions.
void restoreCompletedTransactionsFailed({required SKError error});
/// Triggered when payment queue has finished sending restored transactions.
void paymentQueueRestoreCompletedTransactionsFinished();
/// Triggered when a user initiates an in-app purchase from App Store.
///
/// Return `true` to continue the transaction in your app. If you have
/// multiple [SKTransactionObserverWrapper]s, the transaction will continue if
/// any [SKTransactionObserverWrapper] returns `true`. Return `false` to defer
/// or cancel the transaction. For example, you may need to defer a
/// transaction if the user is in the middle of onboarding. You can also
/// continue the transaction later by calling [addPayment] with the
/// `payment` param from this method.
bool shouldAddStorePayment(
{required SKPaymentWrapper payment, required SKProductWrapper product});
}
/// The state of a transaction.
///
/// Dart wrapper around StoreKit's
/// [SKPaymentTransactionState](https://developer.apple.com/documentation/storekit/skpaymenttransactionstate?language=objc).
enum SKPaymentTransactionStateWrapper {
/// Indicates the transaction is being processed in App Store.
///
/// You should update your UI to indicate that you are waiting for the
/// transaction to update to another state. Never complete a transaction that
/// is still in a purchasing state.
@JsonValue(0)
purchasing,
/// The user's payment has been succesfully processed.
///
/// You should provide the user the content that they purchased.
@JsonValue(1)
purchased,
/// The transaction failed.
///
/// Check the [SKPaymentTransactionWrapper.error] property from
/// [SKPaymentTransactionWrapper] for details.
@JsonValue(2)
failed,
/// This transaction is restoring content previously purchased by the user.
///
/// The previous transaction information can be obtained in
/// [SKPaymentTransactionWrapper.originalTransaction] from
/// [SKPaymentTransactionWrapper].
@JsonValue(3)
restored,
/// The transaction is in the queue but pending external action. Wait for
/// another callback to get the final state.
///
/// You should update your UI to indicate that you are waiting for the
/// transaction to update to another state.
@JsonValue(4)
deferred,
/// Indicates the transaction is in an unspecified state.
@JsonValue(-1)
unspecified;
/// Converts [SKPaymentTransactionStateMessages] into the dart equivalent
static SKPaymentTransactionStateWrapper convertFromPigeon(
SKPaymentTransactionStateMessage msg) {
switch (msg) {
case SKPaymentTransactionStateMessage.purchased:
return SKPaymentTransactionStateWrapper.purchased;
case SKPaymentTransactionStateMessage.purchasing:
return SKPaymentTransactionStateWrapper.purchasing;
case SKPaymentTransactionStateMessage.failed:
return SKPaymentTransactionStateWrapper.failed;
case SKPaymentTransactionStateMessage.restored:
return SKPaymentTransactionStateWrapper.restored;
case SKPaymentTransactionStateMessage.deferred:
return SKPaymentTransactionStateWrapper.deferred;
case SKPaymentTransactionStateMessage.unspecified:
return SKPaymentTransactionStateWrapper.unspecified;
}
}
}
/// Created when a payment is added to the [SKPaymentQueueWrapper].
///
/// Transactions are delivered to your app when a payment is finished
/// processing. Completed transactions provide a receipt and a transaction
/// identifier that the app can use to save a permanent record of the processed
/// payment.
///
/// Dart wrapper around StoreKit's
/// [SKPaymentTransaction](https://developer.apple.com/documentation/storekit/skpaymenttransaction?language=objc).
@JsonSerializable(createToJson: true)
@immutable
class SKPaymentTransactionWrapper {
/// Creates a new [SKPaymentTransactionWrapper] with the provided information.
// TODO(stuartmorgan): Temporarily ignore const warning in other parts of the
// federated package, and remove this.
// ignore: prefer_const_constructors_in_immutables
SKPaymentTransactionWrapper({
required this.payment,
required this.transactionState,
this.originalTransaction,
this.transactionTimeStamp,
this.transactionIdentifier,
this.error,
});
/// Constructs an instance of this from a key value map of data.
///
/// The map needs to have named string keys with values matching the names and
/// types of all of the members on this class. The `map` parameter must not be
/// null.
factory SKPaymentTransactionWrapper.fromJson(Map<String, dynamic> map) {
return _$SKPaymentTransactionWrapperFromJson(map);
}
/// Current transaction state.
@SKTransactionStatusConverter()
final SKPaymentTransactionStateWrapper transactionState;
/// The payment that has been created and added to the payment queue which
/// generated this transaction.
final SKPaymentWrapper payment;
/// The original Transaction.
///
/// Only available if the [transactionState] is [SKPaymentTransactionStateWrapper.restored].
/// Otherwise the value is `null`.
///
/// When the [transactionState]
/// is [SKPaymentTransactionStateWrapper.restored], the current transaction
/// object holds a new [transactionIdentifier].
final SKPaymentTransactionWrapper? originalTransaction;
/// The timestamp of the transaction.
///
/// Seconds since epoch. It is only defined when the [transactionState] is
/// [SKPaymentTransactionStateWrapper.purchased] or
/// [SKPaymentTransactionStateWrapper.restored].
/// Otherwise, the value is `null`.
final double? transactionTimeStamp;
/// The unique string identifer of the transaction.
///
/// It is only defined when the [transactionState] is
/// [SKPaymentTransactionStateWrapper.purchased] or
/// [SKPaymentTransactionStateWrapper.restored]. You may wish to record this
/// string as part of an audit trail for App Store purchases. The value of
/// this string corresponds to the same property in the receipt.
///
/// The value is `null` if it is an unsuccessful transaction.
final String? transactionIdentifier;
/// The error object
///
/// Only available if the [transactionState] is
/// [SKPaymentTransactionStateWrapper.failed].
final SKError? error;
@override
bool operator ==(Object other) {
if (identical(other, this)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is SKPaymentTransactionWrapper &&
other.payment == payment &&
other.transactionState == transactionState &&
other.originalTransaction == originalTransaction &&
other.transactionTimeStamp == transactionTimeStamp &&
other.transactionIdentifier == transactionIdentifier &&
other.error == error;
}
@override
int get hashCode => Object.hash(payment, transactionState,
originalTransaction, transactionTimeStamp, transactionIdentifier, error);
@override
String toString() => _$SKPaymentTransactionWrapperToJson(this).toString();
/// The payload that is used to finish this transaction.
Map<String, String?> toFinishMap() => <String, String?>{
'transactionIdentifier': transactionIdentifier,
'productIdentifier': payment.productIdentifier,
};
/// Converts [SKPaymentTransactionMessages] into the dart equivalent
static SKPaymentTransactionWrapper convertFromPigeon(
SKPaymentTransactionMessage msg) {
return SKPaymentTransactionWrapper(
payment: SKPaymentWrapper.convertFromPigeon(msg.payment),
transactionState: SKPaymentTransactionStateWrapper.convertFromPigeon(
msg.transactionState),
originalTransaction: msg.originalTransaction == null
? null
: convertFromPigeon(msg.originalTransaction!),
transactionTimeStamp: msg.transactionTimeStamp,
transactionIdentifier: msg.transactionIdentifier,
error:
msg.error == null ? null : SKError.convertFromPigeon(msg.error!));
}
}
| packages/packages/in_app_purchase/in_app_purchase_storekit/lib/src/store_kit_wrappers/sk_payment_transaction_wrappers.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_storekit/lib/src/store_kit_wrappers/sk_payment_transaction_wrappers.dart",
"repo_id": "packages",
"token_count": 2684
} | 1,088 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:in_app_purchase_storekit/in_app_purchase_storekit.dart';
import 'package:in_app_purchase_storekit/src/messages.g.dart';
import 'package:in_app_purchase_storekit/store_kit_wrappers.dart';
import '../store_kit_wrappers/sk_test_stub_objects.dart';
import '../test_api.g.dart';
class FakeStoreKitPlatform implements TestInAppPurchaseApi {
// pre-configured store information
String? receiptData;
late Set<String> validProductIDs;
late Map<String, SKProductWrapper> validProducts;
late List<SKPaymentTransactionWrapper> transactionList;
late List<SKPaymentTransactionWrapper> finishedTransactions;
late bool testRestoredTransactionsNull;
late bool testTransactionFail;
late int testTransactionCancel;
PlatformException? queryProductException;
PlatformException? restoreException;
SKError? testRestoredError;
bool queueIsActive = false;
Map<String, dynamic> discountReceived = <String, dynamic>{};
bool isPaymentQueueDelegateRegistered = false;
void reset() {
transactionList = <SKPaymentTransactionWrapper>[];
receiptData = 'dummy base64data';
validProductIDs = <String>{'123', '456'};
validProducts = <String, SKProductWrapper>{};
for (final String validID in validProductIDs) {
final Map<String, dynamic> productWrapperMap =
buildProductMap(dummyProductWrapper);
productWrapperMap['productIdentifier'] = validID;
if (validID == '456') {
productWrapperMap['priceLocale'] = buildLocaleMap(noSymbolLocale);
}
validProducts[validID] = SKProductWrapper.fromJson(productWrapperMap);
}
finishedTransactions = <SKPaymentTransactionWrapper>[];
testRestoredTransactionsNull = false;
testTransactionFail = false;
testTransactionCancel = -1;
queryProductException = null;
restoreException = null;
testRestoredError = null;
queueIsActive = false;
discountReceived = <String, dynamic>{};
isPaymentQueueDelegateRegistered = false;
}
SKPaymentTransactionWrapper createPendingTransaction(String id,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
transactionIdentifier: '',
payment: SKPaymentWrapper(productIdentifier: id, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.purchasing,
transactionTimeStamp: 123123.121,
);
}
SKPaymentTransactionWrapper createPurchasedTransaction(
String productId, String transactionId,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.purchased,
transactionTimeStamp: 123123.121,
transactionIdentifier: transactionId);
}
SKPaymentTransactionWrapper createFailedTransaction(String productId,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
transactionIdentifier: '',
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.failed,
transactionTimeStamp: 123123.121,
error: const SKError(
code: 0,
domain: 'ios_domain',
userInfo: <String, Object>{'message': 'an error message'}));
}
SKPaymentTransactionWrapper createCanceledTransaction(
String productId, int errorCode,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
transactionIdentifier: '',
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.failed,
transactionTimeStamp: 123123.121,
error: SKError(
code: errorCode,
domain: 'ios_domain',
userInfo: const <String, Object>{'message': 'an error message'}));
}
SKPaymentTransactionWrapper createRestoredTransaction(
String productId, String transactionId,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.restored,
transactionTimeStamp: 123123.121,
transactionIdentifier: transactionId);
}
@override
bool canMakePayments() {
return true;
}
@override
void addPayment(Map<String?, Object?> paymentMap) {
final String id = paymentMap['productIdentifier']! as String;
final int quantity = paymentMap['quantity']! as int;
// Keep the received paymentDiscount parameter when testing payment with discount.
if (paymentMap['applicationUsername']! == 'userWithDiscount') {
final Map<Object?, Object?>? discountArgument =
paymentMap['paymentDiscount'] as Map<Object?, Object?>?;
if (discountArgument != null) {
discountReceived = discountArgument.cast<String, Object?>();
} else {
discountReceived = <String, Object?>{};
}
}
final SKPaymentTransactionWrapper transaction =
createPendingTransaction(id, quantity: quantity);
transactionList.add(transaction);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transaction]);
if (testTransactionFail) {
final SKPaymentTransactionWrapper transactionFailed =
createFailedTransaction(id, quantity: quantity);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transactionFailed]);
} else if (testTransactionCancel > 0) {
final SKPaymentTransactionWrapper transactionCanceled =
createCanceledTransaction(id, testTransactionCancel,
quantity: quantity);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transactionCanceled]);
} else {
final SKPaymentTransactionWrapper transactionFinished =
createPurchasedTransaction(
id, transaction.transactionIdentifier ?? '',
quantity: quantity);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transactionFinished]);
}
}
@override
SKStorefrontMessage storefront() {
throw UnimplementedError();
}
@override
List<SKPaymentTransactionMessage?> transactions() {
throw UnimplementedError();
}
@override
void finishTransaction(Map<String?, String?> finishMap) {
finishedTransactions.add(createPurchasedTransaction(
finishMap['productIdentifier']!, finishMap['transactionIdentifier']!,
quantity: transactionList.first.payment.quantity));
}
@override
void presentCodeRedemptionSheet() {}
@override
void restoreTransactions(String? applicationUserName) {
if (restoreException != null) {
throw restoreException!;
}
if (testRestoredError != null) {
InAppPurchaseStoreKitPlatform.observer
.restoreCompletedTransactionsFailed(error: testRestoredError!);
return;
}
if (!testRestoredTransactionsNull) {
InAppPurchaseStoreKitPlatform.observer
.updatedTransactions(transactions: transactionList);
}
InAppPurchaseStoreKitPlatform.observer
.paymentQueueRestoreCompletedTransactionsFinished();
}
@override
Future<SKProductsResponseMessage> startProductRequest(
List<String?> productIdentifiers) {
if (queryProductException != null) {
throw queryProductException!;
}
final List<String?> productIDS = productIdentifiers;
final List<String> invalidFound = <String>[];
final List<SKProductWrapper> products = <SKProductWrapper>[];
for (final String? productID in productIDS) {
if (!validProductIDs.contains(productID)) {
invalidFound.add(productID!);
} else {
products.add(validProducts[productID]!);
}
}
final SkProductResponseWrapper response = SkProductResponseWrapper(
products: products, invalidProductIdentifiers: invalidFound);
return Future<SKProductsResponseMessage>.value(
SkProductResponseWrapper.convertToPigeon(response));
}
@override
Future<void> refreshReceipt({Map<String?, dynamic>? receiptProperties}) {
receiptData = 'refreshed receipt data';
return Future<void>.sync(() {});
}
@override
void registerPaymentQueueDelegate() {
isPaymentQueueDelegateRegistered = true;
}
@override
void removePaymentQueueDelegate() {
isPaymentQueueDelegateRegistered = false;
}
@override
String retrieveReceiptData() {
if (receiptData != null) {
return receiptData!;
} else {
throw PlatformException(code: 'no_receipt_data');
}
}
@override
void showPriceConsentIfNeeded() {}
@override
void startObservingPaymentQueue() {
queueIsActive = true;
}
@override
void stopObservingPaymentQueue() {
queueIsActive = false;
}
}
| packages/packages/in_app_purchase/in_app_purchase_storekit/test/fakes/fake_storekit_platform.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_storekit/test/fakes/fake_storekit_platform.dart",
"repo_id": "packages",
"token_count": 3220
} | 1,089 |
# iOS Platform Images
A Flutter plugin to share images between Flutter and iOS.
This allows Flutter to load images from Images.xcassets and iOS code to load
Flutter images.
When loading images from Image.xcassets the device specific variant is chosen
([iOS documentation](https://developer.apple.com/design/human-interface-guidelines/ios/icons-and-images/image-size-and-resolution/)).
| | iOS |
|-------------|-------|
| **Support** | 12.0+ |
## Usage
### iOS->Flutter Example
<?code-excerpt "example/lib/main.dart (Usage)"?>
```dart
// "flutter" is a resource in Assets.xcassets.
final Image xcassetImage = Image(
image: IosPlatformImages.load('flutter'),
semanticLabel: 'Flutter logo',
);
```
`IosPlatformImages.load` works similarly to [`UIImage(named:)`](https://developer.apple.com/documentation/uikit/uiimage/1624146-imagenamed).
### Flutter->iOS Example
```swift
import ios_platform_images
func makeImage() -> UIImageView {
let image = UIImage.flutterImageWithName("assets/foo.png")
return UIImageView(image: image)
}
```
| packages/packages/ios_platform_images/README.md/0 | {
"file_path": "packages/packages/ios_platform_images/README.md",
"repo_id": "packages",
"token_count": 353
} | 1,090 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:pigeon/pigeon.dart';
@ConfigurePigeon(PigeonOptions(
dartOut: 'lib/src/messages.g.dart',
swiftOut: 'ios/Classes/messages.g.swift',
copyrightHeader: 'pigeons/copyright.txt',
))
/// A serialization of a platform image's data.
class PlatformImageData {
PlatformImageData(this.data, this.scale);
/// The image data.
final Uint8List data;
/// The image's scale factor.
final double scale;
}
@HostApi()
abstract class PlatformImagesApi {
/// Returns the URL for the given resource, or null if no such resource is
/// found.
String? resolveUrl(String resourceName, String? extension);
/// Returns the data for the image resource with the given name, or null if
/// no such resource is found.
PlatformImageData? loadImage(String name);
}
| packages/packages/ios_platform_images/pigeons/messages.dart/0 | {
"file_path": "packages/packages/ios_platform_images/pigeons/messages.dart",
"repo_id": "packages",
"token_count": 287
} | 1,091 |
## NEXT
* Updates minimum supported SDK version to Flutter 3.13/Dart 3.1.
## 1.0.37
* Adds compatibility with `intl` 0.19.0.
* Updates compileSdk version to 34.
## 1.0.36
* Updates androidx.fragment version to 1.6.2.
## 1.0.35
* Updates androidx.fragment version to 1.6.1.
## 1.0.34
* Updates pigeon to 11.0.0 and removes enum wrappers.
## 1.0.33
* Adds pub topics to package metadata.
* Updates minimum supported SDK version to Flutter 3.7/Dart 2.19.
## 1.0.32
* Fixes stale ignore: prefer_const_constructors.
* Updates minimum supported SDK version to Flutter 3.10/Dart 3.0.
* Updates androidx.fragment version to 1.6.0.
## 1.0.31
* Updates androidx.fragment version to 1.5.7.
* Updates androidx.core version to 1.10.1.
## 1.0.30
* Updates androidx.fragment version to 1.5.6
## 1.0.29
* Fixes a regression in 1.0.23 that caused canceled auths to return success.
* Updates minimum supported SDK version to Flutter 3.3/Dart 2.18.
## 1.0.28
* Removes unused resources as indicated by Android lint warnings.
## 1.0.27
* Fixes compatibility with AGP versions older than 4.2.
## 1.0.26
* Adds `targetCompatibilty` matching `sourceCompatibility` for older toolchains.
## 1.0.25
* Adds a namespace for compatibility with AGP 8.0.
## 1.0.24
* Fixes `getEnrolledBiometrics` return value handling.
## 1.0.23
* Switches internals to Pigeon and fixes Java warnings.
## 1.0.22
* Sets an explicit Java compatibility version.
## 1.0.21
* Clarifies explanation of endorsement in README.
* Aligns Dart and Flutter SDK constraints.
## 1.0.20
* Fixes compilation warnings.
* Updates compileSdkVersion to 33.
## 1.0.19
* Updates links for the merge of flutter/plugins into flutter/packages.
## 1.0.18
* Updates minimum Flutter version to 3.0.
* Updates androidx.core version to 1.9.0.
* Upgrades compile SDK version to 33.
## 1.0.17
* Adds compatibility with `intl` 0.18.0.
## 1.0.16
* Updates androidx.fragment version to 1.5.5.
## 1.0.15
* Updates androidx.fragment version to 1.5.4.
## 1.0.14
* Fixes device credential authentication for API versions before R.
## 1.0.13
* Updates imports for `prefer_relative_imports`.
## 1.0.12
* Updates androidx.fragment version to 1.5.2.
* Updates minimum Flutter version to 2.10.
## 1.0.11
* Fixes avoid_redundant_argument_values lint warnings and minor typos.
## 1.0.10
* Updates `local_auth_platform_interface` constraint to the correct minimum
version.
## 1.0.9
* Updates androidx.fragment version to 1.5.1.
## 1.0.8
* Removes usages of `FingerprintManager` and other `BiometricManager` deprecated method usages.
## 1.0.7
* Updates gradle version to 7.2.1.
## 1.0.6
* Updates androidx.core version to 1.8.0.
## 1.0.5
* Updates references to the obsolete master branch.
## 1.0.4
* Minor fixes for new analysis options.
## 1.0.3
* Removes unnecessary imports.
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 1.0.2
* Fixes `getEnrolledBiometrics` to match documented behaviour:
Present biometrics that are not enrolled are no longer returned.
* `getEnrolledBiometrics` now only returns `weak` and `strong` biometric types.
* `deviceSupportsBiometrics` now returns the correct value regardless of enrollment state.
## 1.0.1
* Adopts `Object.hash`.
## 1.0.0
* Initial release from migration to federated architecture.
| packages/packages/local_auth/local_auth_android/CHANGELOG.md/0 | {
"file_path": "packages/packages/local_auth/local_auth_android/CHANGELOG.md",
"repo_id": "packages",
"token_count": 1180
} | 1,092 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:local_auth_platform_interface/default_method_channel_platform.dart';
import 'package:local_auth_platform_interface/local_auth_platform_interface.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
const MethodChannel channel = MethodChannel(
'plugins.flutter.io/local_auth',
);
late List<MethodCall> log;
late LocalAuthPlatform localAuthentication;
setUp(() async {
log = <MethodCall>[];
});
test(
'DefaultLocalAuthPlatform is registered as the default platform implementation',
() async {
expect(LocalAuthPlatform.instance,
const TypeMatcher<DefaultLocalAuthPlatform>());
});
test('getAvailableBiometrics', () async {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.setMockMethodCallHandler(channel, (MethodCall methodCall) {
log.add(methodCall);
return Future<dynamic>.value(<String>[]);
});
localAuthentication = DefaultLocalAuthPlatform();
await localAuthentication.getEnrolledBiometrics();
expect(
log,
<Matcher>[
isMethodCall('getAvailableBiometrics', arguments: null),
],
);
});
test('deviceSupportsBiometrics handles special sentinal value', () async {
// The pre-federation implementation of the platform channels, which the
// default implementation retains compatibility with for the benefit of any
// existing unendorsed implementations, used 'undefined' as a special
// return value from `getAvailableBiometrics` to indicate that nothing was
// enrolled, but that the hardware does support biometrics.
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.setMockMethodCallHandler(channel, (MethodCall methodCall) {
log.add(methodCall);
return Future<dynamic>.value(<String>['undefined']);
});
localAuthentication = DefaultLocalAuthPlatform();
final bool supportsBiometrics =
await localAuthentication.deviceSupportsBiometrics();
expect(supportsBiometrics, true);
expect(
log,
<Matcher>[
isMethodCall('getAvailableBiometrics', arguments: null),
],
);
});
group('Boolean returning methods', () {
setUp(() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.setMockMethodCallHandler(channel, (MethodCall methodCall) {
log.add(methodCall);
return Future<dynamic>.value(true);
});
localAuthentication = DefaultLocalAuthPlatform();
});
test('isDeviceSupported', () async {
await localAuthentication.isDeviceSupported();
expect(
log,
<Matcher>[
isMethodCall('isDeviceSupported', arguments: null),
],
);
});
test('stopAuthentication', () async {
await localAuthentication.stopAuthentication();
expect(
log,
<Matcher>[
isMethodCall('stopAuthentication', arguments: null),
],
);
});
group('authenticate with device auth fail over', () {
test('authenticate with no args.', () async {
await localAuthentication.authenticate(
authMessages: <AuthMessages>[],
localizedReason: 'Needs secure',
options: const AuthenticationOptions(biometricOnly: true),
);
expect(
log,
<Matcher>[
isMethodCall(
'authenticate',
arguments: <String, dynamic>{
'localizedReason': 'Needs secure',
'useErrorDialogs': true,
'stickyAuth': false,
'sensitiveTransaction': true,
'biometricOnly': true,
},
),
],
);
});
test('authenticate with no sensitive transaction.', () async {
await localAuthentication.authenticate(
authMessages: <AuthMessages>[],
localizedReason: 'Insecure',
options: const AuthenticationOptions(
sensitiveTransaction: false,
useErrorDialogs: false,
biometricOnly: true,
),
);
expect(
log,
<Matcher>[
isMethodCall(
'authenticate',
arguments: <String, dynamic>{
'localizedReason': 'Insecure',
'useErrorDialogs': false,
'stickyAuth': false,
'sensitiveTransaction': false,
'biometricOnly': true,
},
),
],
);
});
});
group('authenticate with biometrics only', () {
test('authenticate with no args.', () async {
await localAuthentication.authenticate(
authMessages: <AuthMessages>[],
localizedReason: 'Needs secure',
);
expect(
log,
<Matcher>[
isMethodCall(
'authenticate',
arguments: <String, dynamic>{
'localizedReason': 'Needs secure',
'useErrorDialogs': true,
'stickyAuth': false,
'sensitiveTransaction': true,
'biometricOnly': false,
},
),
],
);
});
test('authenticate with no sensitive transaction.', () async {
await localAuthentication.authenticate(
authMessages: <AuthMessages>[],
localizedReason: 'Insecure',
options: const AuthenticationOptions(
sensitiveTransaction: false,
useErrorDialogs: false,
),
);
expect(
log,
<Matcher>[
isMethodCall(
'authenticate',
arguments: <String, dynamic>{
'localizedReason': 'Insecure',
'useErrorDialogs': false,
'stickyAuth': false,
'sensitiveTransaction': false,
'biometricOnly': false,
},
),
],
);
});
});
});
}
| packages/packages/local_auth/local_auth_platform_interface/test/default_method_channel_platform_test.dart/0 | {
"file_path": "packages/packages/local_auth/local_auth_platform_interface/test/default_method_channel_platform_test.dart",
"repo_id": "packages",
"token_count": 2703
} | 1,093 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:pigeon/pigeon.dart';
@ConfigurePigeon(PigeonOptions(
dartOut: 'lib/src/messages.g.dart',
cppOptions: CppOptions(namespace: 'local_auth_windows'),
cppHeaderOut: 'windows/messages.g.h',
cppSourceOut: 'windows/messages.g.cpp',
copyrightHeader: 'pigeons/copyright.txt',
))
@HostApi()
abstract class LocalAuthApi {
/// Returns true if this device supports authentication.
@async
bool isDeviceSupported();
/// Attempts to authenticate the user with the provided [localizedReason] as
/// the user-facing explanation for the authorization request.
///
/// Returns true if authorization succeeds, false if it is attempted but is
/// not successful, and an error if authorization could not be attempted.
@async
bool authenticate(String localizedReason);
}
| packages/packages/local_auth/local_auth_windows/pigeons/messages.dart/0 | {
"file_path": "packages/packages/local_auth/local_auth_windows/pigeons/messages.dart",
"repo_id": "packages",
"token_count": 283
} | 1,094 |
# Metrics Center
Metrics center is a minimal set of code and services to support multiple perf
metrics generators (e.g., Cocoon device lab, Cirrus bots, LUCI bots, Firebase
Test Lab) and destinations (e.g., old Cocoon perf dashboard, Skia perf
dashboard). The work and maintenance it requires is very close to that of just
supporting a single generator and destination (e.g., engine bots to Skia perf),
and the small amount of extra work is designed to make it easy to support more
generators and destinations in the future.
| packages/packages/metrics_center/README.md/0 | {
"file_path": "packages/packages/metrics_center/README.md",
"repo_id": "packages",
"token_count": 130
} | 1,095 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@Timeout(Duration(seconds: 3600))
library;
import 'dart:async';
import 'dart:convert';
import 'package:gcloud/storage.dart';
import 'package:googleapis/storage/v1.dart'
show DetailedApiRequestError, StorageApi;
import 'package:googleapis_auth/auth_io.dart';
import 'package:metrics_center/metrics_center.dart';
import 'package:metrics_center/src/gcs_lock.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'common.dart';
import 'skiaperf_test.mocks.dart';
import 'utility.dart';
class MockGcsLock implements GcsLock {
@override
Future<void> protectedRun(
String exclusiveObjectName, Future<void> Function() f) async {
await f();
}
}
class MockSkiaPerfGcsAdaptor implements SkiaPerfGcsAdaptor {
MockSkiaPerfGcsAdaptor({
this.writePointsOverride,
});
final Future<void> Function()? writePointsOverride;
@override
Future<List<SkiaPerfPoint>> readPoints(String objectName) async {
return _storage[objectName] ?? <SkiaPerfPoint>[];
}
@override
Future<void> writePoints(
String objectName, List<SkiaPerfPoint> points) async {
if (writePointsOverride != null) {
return writePointsOverride!();
}
_storage[objectName] = points.toList();
}
// Map from the object name to the list of SkiaPoint that mocks the GCS.
final Map<String, List<SkiaPerfPoint>> _storage =
<String, List<SkiaPerfPoint>>{};
}
@GenerateMocks(<Type>[Bucket, ObjectInfo])
Future<void> main() async {
const double kValue1 = 1.0;
const double kValue2 = 2.0;
const double kValue3 = 3.0;
const String kFrameworkRevision1 = '9011cece2595447eea5dd91adaa241c1c9ef9a33';
const String kFrameworkRevision2 = '372fe290e4d4f3f97cbf02a57d235771a9412f10';
const String kEngineRevision1 = '617938024315e205f26ed72ff0f0647775fa6a71';
const String kEngineRevision2 = '5858519139c22484aaff1cf5b26bdf7951259344';
const String kTaskName = 'analyzer_benchmark';
const String kMetric1 = 'flutter_repo_batch_maximum';
const String kMetric2 = 'flutter_repo_watch_maximum';
final MetricPoint cocoonPointRev1Metric1 = MetricPoint(
kValue1,
const <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kGitRevisionKey: kFrameworkRevision1,
kNameKey: kTaskName,
kSubResultKey: kMetric1,
kUnitKey: 's',
},
);
final MetricPoint cocoonPointRev1Metric2 = MetricPoint(
kValue2,
const <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kGitRevisionKey: kFrameworkRevision1,
kNameKey: kTaskName,
kSubResultKey: kMetric2,
kUnitKey: 's',
},
);
final MetricPoint cocoonPointRev2Metric1 = MetricPoint(
kValue3,
const <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kGitRevisionKey: kFrameworkRevision2,
kNameKey: kTaskName,
kSubResultKey: kMetric1,
kUnitKey: 's',
},
);
final MetricPoint cocoonPointBetaRev1Metric1 = MetricPoint(
kValue1,
const <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kGitRevisionKey: kFrameworkRevision1,
kNameKey: 'beta/$kTaskName',
kSubResultKey: kMetric1,
kUnitKey: 's',
'branch': 'beta',
},
);
final MetricPoint cocoonPointBetaRev1Metric1BadBranch = MetricPoint(
kValue1,
const <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kGitRevisionKey: kFrameworkRevision1,
kNameKey: kTaskName,
kSubResultKey: kMetric1,
kUnitKey: 's',
// If we only add this 'branch' tag without changing the test or sub-result name, an exception
// would be thrown as Skia Perf currently only supports the same set of tags for a pair of
// kNameKey and kSubResultKey values. So to support branches, one also has to add the branch
// name to the test name.
'branch': 'beta',
},
);
const String engineMetricName = 'BM_PaintRecordInit';
const String engineRevision = 'ca799fa8b2254d09664b78ee80c43b434788d112';
const double engineValue1 = 101;
const double engineValue2 = 102;
final FlutterEngineMetricPoint enginePoint1 = FlutterEngineMetricPoint(
engineMetricName,
engineValue1,
engineRevision,
moreTags: const <String, String>{
kSubResultKey: 'cpu_time',
kUnitKey: 'ns',
'date': '2019-12-17 15:14:14',
'num_cpus': '56',
'mhz_per_cpu': '2594',
'cpu_scaling_enabled': 'true',
'library_build_type': 'release',
},
);
final FlutterEngineMetricPoint enginePoint2 = FlutterEngineMetricPoint(
engineMetricName,
engineValue2,
engineRevision,
moreTags: const <String, String>{
kSubResultKey: 'real_time',
kUnitKey: 'ns',
'date': '2019-12-17 15:14:14',
'num_cpus': '56',
'mhz_per_cpu': '2594',
'cpu_scaling_enabled': 'true',
'library_build_type': 'release',
},
);
test('Throw if invalid points are converted to SkiaPoint', () {
final MetricPoint noGithubRepoPoint = MetricPoint(
kValue1,
const <String, String>{
kGitRevisionKey: kFrameworkRevision1,
kNameKey: kTaskName,
},
);
final MetricPoint noGitRevisionPoint = MetricPoint(
kValue1,
const <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kNameKey: kTaskName,
},
);
final MetricPoint noTestNamePoint = MetricPoint(
kValue1,
const <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kGitRevisionKey: kFrameworkRevision1,
},
);
expect(() => SkiaPerfPoint.fromPoint(noGithubRepoPoint), throwsA(anything));
expect(
() => SkiaPerfPoint.fromPoint(noGitRevisionPoint), throwsA(anything));
expect(() => SkiaPerfPoint.fromPoint(noTestNamePoint), throwsA(anything));
});
test('Correctly convert a metric point from cocoon to SkiaPoint', () {
final SkiaPerfPoint skiaPoint1 =
SkiaPerfPoint.fromPoint(cocoonPointRev1Metric1);
expect(skiaPoint1, isNotNull);
expect(skiaPoint1.testName, equals(kTaskName));
expect(skiaPoint1.subResult, equals(kMetric1));
expect(skiaPoint1.value, equals(cocoonPointRev1Metric1.value));
expect(skiaPoint1.jsonUrl, isNull); // Not inserted yet
});
test('Cocoon points correctly encode into Skia perf json format', () {
final SkiaPerfPoint p1 = SkiaPerfPoint.fromPoint(cocoonPointRev1Metric1);
final SkiaPerfPoint p2 = SkiaPerfPoint.fromPoint(cocoonPointRev1Metric2);
final SkiaPerfPoint p3 =
SkiaPerfPoint.fromPoint(cocoonPointBetaRev1Metric1);
const JsonEncoder encoder = JsonEncoder.withIndent(' ');
expect(
encoder
.convert(SkiaPerfPoint.toSkiaPerfJson(<SkiaPerfPoint>[p1, p2, p3])),
equals('''
{
"gitHash": "9011cece2595447eea5dd91adaa241c1c9ef9a33",
"results": {
"analyzer_benchmark": {
"default": {
"flutter_repo_batch_maximum": 1.0,
"options": {
"unit": "s"
},
"flutter_repo_watch_maximum": 2.0
}
},
"beta/analyzer_benchmark": {
"default": {
"flutter_repo_batch_maximum": 1.0,
"options": {
"branch": "beta",
"unit": "s"
}
}
}
}
}'''));
});
test('Engine metric points correctly encode into Skia perf json format', () {
const JsonEncoder encoder = JsonEncoder.withIndent(' ');
expect(
encoder.convert(SkiaPerfPoint.toSkiaPerfJson(<SkiaPerfPoint>[
SkiaPerfPoint.fromPoint(enginePoint1),
SkiaPerfPoint.fromPoint(enginePoint2),
])),
equals(
'''
{
"gitHash": "ca799fa8b2254d09664b78ee80c43b434788d112",
"results": {
"BM_PaintRecordInit": {
"default": {
"cpu_time": 101.0,
"options": {
"cpu_scaling_enabled": "true",
"library_build_type": "release",
"mhz_per_cpu": "2594",
"num_cpus": "56",
"unit": "ns"
},
"real_time": 102.0
}
}
}
}''',
),
);
});
test(
'Throw if engine points with the same test name but different options are converted to '
'Skia perf points', () {
final FlutterEngineMetricPoint enginePoint1 = FlutterEngineMetricPoint(
'BM_PaintRecordInit',
101,
'ca799fa8b2254d09664b78ee80c43b434788d112',
moreTags: const <String, String>{
kSubResultKey: 'cpu_time',
kUnitKey: 'ns',
'cpu_scaling_enabled': 'true',
},
);
final FlutterEngineMetricPoint enginePoint2 = FlutterEngineMetricPoint(
'BM_PaintRecordInit',
102,
'ca799fa8b2254d09664b78ee80c43b434788d112',
moreTags: const <String, String>{
kSubResultKey: 'real_time',
kUnitKey: 'ns',
'cpu_scaling_enabled': 'false',
},
);
const JsonEncoder encoder = JsonEncoder.withIndent(' ');
expect(
() => encoder.convert(SkiaPerfPoint.toSkiaPerfJson(<SkiaPerfPoint>[
SkiaPerfPoint.fromPoint(enginePoint1),
SkiaPerfPoint.fromPoint(enginePoint2),
])),
throwsA(anything),
);
});
test(
'Throw if two Cocoon metric points with the same name and subResult keys '
'but different options are converted to Skia perf points', () {
final SkiaPerfPoint p1 = SkiaPerfPoint.fromPoint(cocoonPointRev1Metric1);
final SkiaPerfPoint p2 =
SkiaPerfPoint.fromPoint(cocoonPointBetaRev1Metric1BadBranch);
expect(
() => SkiaPerfPoint.toSkiaPerfJson(<SkiaPerfPoint>[p1, p2]),
throwsA(anything),
);
});
test('SkiaPerfGcsAdaptor computes name correctly', () async {
expect(
await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision1,
DateTime.utc(2019, 12, 04, 23),
'test',
),
equals(
'flutter-flutter/2019/12/04/23/$kFrameworkRevision1/test_values.json'),
);
expect(
await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterEngineRepo,
kEngineRevision1,
DateTime.utc(2019, 12, 03, 20),
'test',
),
equals('flutter-engine/2019/12/03/20/$kEngineRevision1/test_values.json'),
);
expect(
await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterEngineRepo,
kEngineRevision2,
DateTime.utc(2020, 01, 03, 15),
'test',
),
equals('flutter-engine/2020/01/03/15/$kEngineRevision2/test_values.json'),
);
});
test('Successfully read mock GCS that fails 1st time with 504', () async {
final MockBucket testBucket = MockBucket();
final SkiaPerfGcsAdaptor skiaPerfGcs = SkiaPerfGcsAdaptor(testBucket);
final String testObjectName = await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision1,
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
final List<SkiaPerfPoint> writePoints = <SkiaPerfPoint>[
SkiaPerfPoint.fromPoint(cocoonPointRev1Metric1),
];
final String skiaPerfJson =
jsonEncode(SkiaPerfPoint.toSkiaPerfJson(writePoints));
when(testBucket.writeBytes(testObjectName, utf8.encode(skiaPerfJson)))
.thenAnswer((_) async => FakeObjectInfo());
await skiaPerfGcs.writePoints(testObjectName, writePoints);
verify(testBucket.writeBytes(testObjectName, utf8.encode(skiaPerfJson)));
// Emulate the first network request to fail with 504.
when(testBucket.info(testObjectName))
.thenThrow(DetailedApiRequestError(504, 'Test Failure'));
final MockObjectInfo mockObjectInfo = MockObjectInfo();
when(mockObjectInfo.downloadLink)
.thenReturn(Uri.https('test.com', 'mock.json'));
when(testBucket.info(testObjectName))
.thenAnswer((_) => Future<ObjectInfo>.value(mockObjectInfo));
when(testBucket.read(testObjectName))
.thenAnswer((_) => Stream<List<int>>.value(utf8.encode(skiaPerfJson)));
final List<SkiaPerfPoint> readPoints =
await skiaPerfGcs.readPoints(testObjectName);
expect(readPoints.length, equals(1));
expect(readPoints[0].testName, kTaskName);
expect(readPoints[0].subResult, kMetric1);
expect(readPoints[0].value, kValue1);
expect(readPoints[0].githubRepo, kFlutterFrameworkRepo);
expect(readPoints[0].gitHash, kFrameworkRevision1);
expect(readPoints[0].jsonUrl, 'https://test.com/mock.json');
});
test('Return empty list if the GCS file does not exist', () async {
final MockBucket testBucket = MockBucket();
final SkiaPerfGcsAdaptor skiaPerfGcs = SkiaPerfGcsAdaptor(testBucket);
final String testObjectName = await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision1,
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
when(testBucket.info(testObjectName))
.thenThrow(Exception('No such object'));
expect((await skiaPerfGcs.readPoints(testObjectName)).length, 0);
});
// The following is for integration tests.
Bucket? testBucket;
GcsLock? testLock;
final Map<String, dynamic>? credentialsJson = getTestGcpCredentialsJson();
if (credentialsJson != null) {
final ServiceAccountCredentials credentials =
ServiceAccountCredentials.fromJson(credentialsJson);
final AutoRefreshingAuthClient client =
await clientViaServiceAccount(credentials, Storage.SCOPES);
final Storage storage =
Storage(client, credentialsJson['project_id'] as String);
const String kTestBucketName = 'flutter-skia-perf-test';
assert(await storage.bucketExists(kTestBucketName));
testBucket = storage.bucket(kTestBucketName);
testLock = GcsLock(StorageApi(client), kTestBucketName);
}
Future<void> skiaPerfGcsAdapterIntegrationTest() async {
final SkiaPerfGcsAdaptor skiaPerfGcs = SkiaPerfGcsAdaptor(testBucket!);
final String testObjectName = await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision1,
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
await skiaPerfGcs.writePoints(testObjectName, <SkiaPerfPoint>[
SkiaPerfPoint.fromPoint(cocoonPointRev1Metric1),
SkiaPerfPoint.fromPoint(cocoonPointRev1Metric2),
]);
final List<SkiaPerfPoint> points =
await skiaPerfGcs.readPoints(testObjectName);
expect(points.length, equals(2));
expectSetMatch(
points.map((SkiaPerfPoint p) => p.testName), <String>[kTaskName]);
expectSetMatch(points.map((SkiaPerfPoint p) => p.subResult),
<String>[kMetric1, kMetric2]);
expectSetMatch(
points.map((SkiaPerfPoint p) => p.value), <double>[kValue1, kValue2]);
expectSetMatch(points.map((SkiaPerfPoint p) => p.githubRepo),
<String>[kFlutterFrameworkRepo]);
expectSetMatch(points.map((SkiaPerfPoint p) => p.gitHash),
<String>[kFrameworkRevision1]);
for (int i = 0; i < 2; i += 1) {
expect(points[0].jsonUrl, startsWith('https://'));
}
}
Future<void> skiaPerfGcsIntegrationTestWithEnginePoints() async {
final SkiaPerfGcsAdaptor skiaPerfGcs = SkiaPerfGcsAdaptor(testBucket!);
final String testObjectName = await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterEngineRepo,
engineRevision,
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
await skiaPerfGcs.writePoints(testObjectName, <SkiaPerfPoint>[
SkiaPerfPoint.fromPoint(enginePoint1),
SkiaPerfPoint.fromPoint(enginePoint2),
]);
final List<SkiaPerfPoint> points =
await skiaPerfGcs.readPoints(testObjectName);
expect(points.length, equals(2));
expectSetMatch(
points.map((SkiaPerfPoint p) => p.testName),
<String>[engineMetricName, engineMetricName],
);
expectSetMatch(
points.map((SkiaPerfPoint p) => p.value),
<double>[engineValue1, engineValue2],
);
expectSetMatch(
points.map((SkiaPerfPoint p) => p.githubRepo),
<String>[kFlutterEngineRepo],
);
expectSetMatch(
points.map((SkiaPerfPoint p) => p.gitHash), <String>[engineRevision]);
for (int i = 0; i < 2; i += 1) {
expect(points[0].jsonUrl, startsWith('https://'));
}
}
// To run the following integration tests, there must be a valid Google Cloud
// Project service account credentials in secret/test_gcp_credentials.json so
// `testBucket` won't be null. Currently, these integration tests are skipped
// in the CI, and only verified locally.
test(
'SkiaPerfGcsAdaptor passes integration test with Google Cloud Storage',
skiaPerfGcsAdapterIntegrationTest,
skip: testBucket == null,
);
test(
'SkiaPerfGcsAdaptor integration test with engine points',
skiaPerfGcsIntegrationTestWithEnginePoints,
skip: testBucket == null,
);
// `SkiaPerfGcsAdaptor.computeObjectName` uses `GithubHelper` which requires
// network connections. Hence we put them as integration tests instead of unit
// tests.
test(
'SkiaPerfGcsAdaptor integration test for name computations',
() async {
expect(
await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision1,
DateTime.utc(2019, 12, 04, 23),
'test',
),
equals(
'flutter-flutter/2019/12/04/23/$kFrameworkRevision1/test_values.json'),
);
expect(
await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterEngineRepo,
kEngineRevision1,
DateTime.utc(2019, 12, 03, 20),
'test',
),
equals(
'flutter-engine/2019/12/03/20/$kEngineRevision1/test_values.json'),
);
expect(
await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterEngineRepo,
kEngineRevision2,
DateTime.utc(2020, 01, 03, 15),
'test',
),
equals(
'flutter-engine/2020/01/03/15/$kEngineRevision2/test_values.json'),
);
},
skip: testBucket == null,
);
test('SkiaPerfDestination.update awaits locks', () async {
bool updateCompleted = false;
final Completer<void> callbackCompleter = Completer<void>();
final SkiaPerfGcsAdaptor mockGcs = MockSkiaPerfGcsAdaptor(
writePointsOverride: () => callbackCompleter.future,
);
final GcsLock mockLock = MockGcsLock();
final SkiaPerfDestination dst = SkiaPerfDestination(mockGcs, mockLock);
final Future<void> updateFuture = dst.update(
<MetricPoint>[cocoonPointRev1Metric1],
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
final Future<void> markedUpdateCompleted = updateFuture.then<void>((_) {
updateCompleted = true;
});
// spin event loop to make sure function hasn't done anything yet
await (Completer<void>()..complete()).future;
// Ensure that the .update() method is waiting for callbackCompleter
expect(updateCompleted, false);
callbackCompleter.complete();
await markedUpdateCompleted;
expect(updateCompleted, true);
});
test('SkiaPerfDestination correctly updates points', () async {
final SkiaPerfGcsAdaptor mockGcs = MockSkiaPerfGcsAdaptor();
final GcsLock mockLock = MockGcsLock();
final SkiaPerfDestination dst = SkiaPerfDestination(mockGcs, mockLock);
await dst.update(
<MetricPoint>[cocoonPointRev1Metric1],
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
await dst.update(
<MetricPoint>[cocoonPointRev1Metric2],
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
List<SkiaPerfPoint> points =
await mockGcs.readPoints(await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision1,
DateTime.fromMillisecondsSinceEpoch(123),
'test',
));
expect(points.length, equals(2));
expectSetMatch(
points.map((SkiaPerfPoint p) => p.testName), <String>[kTaskName]);
expectSetMatch(points.map((SkiaPerfPoint p) => p.subResult),
<String>[kMetric1, kMetric2]);
expectSetMatch(
points.map((SkiaPerfPoint p) => p.value), <double>[kValue1, kValue2]);
final MetricPoint updated =
MetricPoint(kValue3, cocoonPointRev1Metric1.tags);
await dst.update(
<MetricPoint>[updated, cocoonPointRev2Metric1],
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
points =
await mockGcs.readPoints(await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision2,
DateTime.fromMillisecondsSinceEpoch(123),
'test',
));
expect(points.length, equals(1));
expect(points[0].gitHash, equals(kFrameworkRevision2));
expect(points[0].value, equals(kValue3));
points =
await mockGcs.readPoints(await SkiaPerfGcsAdaptor.computeObjectName(
kFlutterFrameworkRepo,
kFrameworkRevision1,
DateTime.fromMillisecondsSinceEpoch(123),
'test',
));
expectSetMatch(
points.map((SkiaPerfPoint p) => p.value), <double>[kValue2, kValue3]);
});
Future<void> skiaPerfDestinationIntegrationTest() async {
final SkiaPerfDestination destination =
SkiaPerfDestination(SkiaPerfGcsAdaptor(testBucket!), testLock);
await destination.update(
<MetricPoint>[cocoonPointRev1Metric1],
DateTime.fromMillisecondsSinceEpoch(123),
'test',
);
}
test(
'SkiaPerfDestination integration test',
skiaPerfDestinationIntegrationTest,
skip: testBucket == null,
);
}
class FakeObjectInfo extends ObjectInfo {
@override
int get crc32CChecksum => throw UnimplementedError();
@override
Uri get downloadLink => throw UnimplementedError();
@override
String get etag => throw UnimplementedError();
@override
ObjectGeneration get generation => throw UnimplementedError();
@override
int get length => throw UnimplementedError();
@override
List<int> get md5Hash => throw UnimplementedError();
@override
ObjectMetadata get metadata => throw UnimplementedError();
@override
String get name => throw UnimplementedError();
@override
DateTime get updated => throw UnimplementedError();
}
| packages/packages/metrics_center/test/skiaperf_test.dart/0 | {
"file_path": "packages/packages/metrics_center/test/skiaperf_test.dart",
"repo_id": "packages",
"token_count": 9234
} | 1,096 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:io';
import 'dart:typed_data';
import 'package:meta/meta.dart';
import 'constants.dart';
import 'packet.dart';
/// Enumeration of support resource record types.
abstract class ResourceRecordType {
// This class is intended to be used as a namespace, and should not be
// extended directly.
ResourceRecordType._();
/// An IPv4 Address record, also known as an "A" record. It has a value of 1.
static const int addressIPv4 = 1;
/// An IPv6 Address record, also known as an "AAAA" record. It has a vaule of
/// 28.
static const int addressIPv6 = 28;
/// An IP Address reverse map record, also known as a "PTR" recored. It has a
/// value of 12.
static const int serverPointer = 12;
/// An available service record, also known as an "SRV" record. It has a
/// value of 33.
static const int service = 33;
/// A text record, also known as a "TXT" record. It has a value of 16.
static const int text = 16;
// TODO(dnfield): Support ANY in some meaningful way. Might be server only.
// /// A query for all records of all types known to the name server.
// static const int any = 255;
/// Checks that a given int is a valid ResourceRecordType.
///
/// This method is intended to be called only from an `assert()`.
static bool debugAssertValid(int resourceRecordType) {
return resourceRecordType == addressIPv4 ||
resourceRecordType == addressIPv6 ||
resourceRecordType == serverPointer ||
resourceRecordType == service ||
resourceRecordType == text;
}
/// Prints a debug-friendly version of the resource record type value.
static String toDebugString(int resourceRecordType) {
switch (resourceRecordType) {
case addressIPv4:
return 'A (IPv4 Address)';
case addressIPv6:
return 'AAAA (IPv6 Address)';
case serverPointer:
return 'PTR (Domain Name Pointer)';
case service:
return 'SRV (Service record)';
case text:
return 'TXT (Text)';
}
return 'Unknown ($resourceRecordType)';
}
}
/// Represents a DNS query.
@immutable
class ResourceRecordQuery {
/// Creates a new ResourceRecordQuery.
///
/// Most callers should prefer one of the named constructors.
ResourceRecordQuery(
this.resourceRecordType,
this.fullyQualifiedName,
this.questionType,
) : assert(ResourceRecordType.debugAssertValid(resourceRecordType));
/// An A (IPv4) query.
ResourceRecordQuery.addressIPv4(
String name, {
bool isMulticast = true,
}) : this(
ResourceRecordType.addressIPv4,
name,
isMulticast ? QuestionType.multicast : QuestionType.unicast,
);
/// An AAAA (IPv6) query.
ResourceRecordQuery.addressIPv6(
String name, {
bool isMulticast = true,
}) : this(
ResourceRecordType.addressIPv6,
name,
isMulticast ? QuestionType.multicast : QuestionType.unicast,
);
/// A PTR (Server pointer) query.
ResourceRecordQuery.serverPointer(
String name, {
bool isMulticast = true,
}) : this(
ResourceRecordType.serverPointer,
name,
isMulticast ? QuestionType.multicast : QuestionType.unicast,
);
/// An SRV (Service) query.
ResourceRecordQuery.service(
String name, {
bool isMulticast = true,
}) : this(
ResourceRecordType.service,
name,
isMulticast ? QuestionType.multicast : QuestionType.unicast,
);
/// A TXT (Text record) query.
ResourceRecordQuery.text(
String name, {
bool isMulticast = true,
}) : this(
ResourceRecordType.text,
name,
isMulticast ? QuestionType.multicast : QuestionType.unicast,
);
/// Tye type of resource record - one of [ResourceRecordType]'s values.
final int resourceRecordType;
/// The Fully Qualified Domain Name associated with the request.
final String fullyQualifiedName;
/// The [QuestionType], i.e. multicast or unicast.
final int questionType;
/// Convenience accessor to determine whether the question type is multicast.
bool get isMulticast => questionType == QuestionType.multicast;
/// Convenience accessor to determine whether the question type is unicast.
bool get isUnicast => questionType == QuestionType.unicast;
/// Encodes this query to the raw wire format.
List<int> encode() {
return encodeMDnsQuery(
fullyQualifiedName,
type: resourceRecordType,
multicast: isMulticast,
);
}
@override
int get hashCode =>
Object.hash(resourceRecordType, fullyQualifiedName, questionType);
@override
bool operator ==(Object other) {
return other is ResourceRecordQuery &&
other.resourceRecordType == resourceRecordType &&
other.fullyQualifiedName == fullyQualifiedName &&
other.questionType == questionType;
}
@override
String toString() =>
'ResourceRecordQuery{$fullyQualifiedName, type: ${ResourceRecordType.toDebugString(resourceRecordType)}, isMulticast: $isMulticast}';
}
/// Base implementation of DNS resource records (RRs).
@immutable
abstract class ResourceRecord {
/// Creates a new ResourceRecord.
const ResourceRecord(this.resourceRecordType, this.name, this.validUntil);
/// The FQDN for this record.
final String name;
/// The epoch time at which point this record is valid for in the cache.
final int validUntil;
/// The raw resource record value. See [ResourceRecordType] for supported values.
final int resourceRecordType;
String get _additionalInfo;
@override
String toString() =>
'$runtimeType{$name, validUntil: ${DateTime.fromMillisecondsSinceEpoch(validUntil)}, $_additionalInfo}';
@override
int get hashCode => Object.hash(name, validUntil, resourceRecordType);
@override
bool operator ==(Object other) {
return other is ResourceRecord &&
other.name == name &&
other.validUntil == validUntil &&
other.resourceRecordType == resourceRecordType;
}
/// Low level method for encoding this record into an mDNS packet.
///
/// Subclasses should provide the packet format of their encapsulated data
/// into a `Uint8List`, which could then be used to write a pakcet to send
/// as a response for this record type.
Uint8List encodeResponseRecord();
}
/// A Service Pointer for reverse mapping an IP address (DNS "PTR").
class PtrResourceRecord extends ResourceRecord {
/// Creates a new PtrResourceRecord.
const PtrResourceRecord(
String name,
int validUntil, {
required this.domainName,
}) : super(ResourceRecordType.serverPointer, name, validUntil);
/// The FQDN for this record.
final String domainName;
@override
String get _additionalInfo => 'domainName: $domainName';
@override
int get hashCode => Object.hash(domainName.hashCode, super.hashCode);
@override
bool operator ==(Object other) {
return super == other &&
other is PtrResourceRecord &&
other.domainName == domainName;
}
@override
Uint8List encodeResponseRecord() {
return Uint8List.fromList(utf8.encode(domainName));
}
}
/// An IP Address record for IPv4 (DNS "A") or IPv6 (DNS "AAAA") records.
class IPAddressResourceRecord extends ResourceRecord {
/// Creates a new IPAddressResourceRecord.
IPAddressResourceRecord(
String name,
int validUntil, {
required this.address,
}) : super(
address.type == InternetAddressType.IPv4
? ResourceRecordType.addressIPv4
: ResourceRecordType.addressIPv6,
name,
validUntil);
/// The [InternetAddress] for this record.
final InternetAddress address;
@override
String get _additionalInfo => 'address: $address';
@override
int get hashCode => Object.hash(address.hashCode, super.hashCode);
@override
bool operator ==(Object other) {
return super == other &&
other is IPAddressResourceRecord &&
other.address == address;
}
@override
Uint8List encodeResponseRecord() {
return Uint8List.fromList(address.rawAddress);
}
}
/// A Service record, capturing a host target and port (DNS "SRV").
class SrvResourceRecord extends ResourceRecord {
/// Creates a new service record.
const SrvResourceRecord(
String name,
int validUntil, {
required this.target,
required this.port,
required this.priority,
required this.weight,
}) : super(ResourceRecordType.service, name, validUntil);
/// The hostname for this record.
final String target;
/// The port for this record.
final int port;
/// The relative priority of this service.
final int priority;
/// The weight (used when multiple services have the same priority).
final int weight;
@override
String get _additionalInfo =>
'target: $target, port: $port, priority: $priority, weight: $weight';
@override
int get hashCode =>
Object.hash(target, port, priority, weight, super.hashCode);
@override
bool operator ==(Object other) {
return super == other &&
other is SrvResourceRecord &&
other.target == target &&
other.port == port &&
other.priority == priority &&
other.weight == weight;
}
@override
Uint8List encodeResponseRecord() {
final List<int> data = utf8.encode(target);
final Uint8List result = Uint8List(data.length + 7);
final ByteData resultData = ByteData.view(result.buffer);
resultData.setUint16(0, priority);
resultData.setUint16(2, weight);
resultData.setUint16(4, port);
result[6] = data.length;
return result..setRange(7, data.length, data);
}
}
/// A Text record, contianing additional textual data (DNS "TXT").
class TxtResourceRecord extends ResourceRecord {
/// Creates a new text record.
const TxtResourceRecord(
String name,
int validUntil, {
required this.text,
}) : super(ResourceRecordType.text, name, validUntil);
/// The raw text from this record.
final String text;
@override
String get _additionalInfo => 'text: $text';
@override
int get hashCode => Object.hash(text.hashCode, super.hashCode);
@override
bool operator ==(Object other) =>
super == other && other is TxtResourceRecord && other.text == text;
@override
Uint8List encodeResponseRecord() {
return Uint8List.fromList(utf8.encode(text));
}
}
| packages/packages/multicast_dns/lib/src/resource_record.dart/0 | {
"file_path": "packages/packages/multicast_dns/lib/src/resource_record.dart",
"repo_id": "packages",
"token_count": 3544
} | 1,097 |
def localProperties = new Properties()
def localPropertiesFile = rootProject.file('local.properties')
if (localPropertiesFile.exists()) {
localPropertiesFile.withReader('UTF-8') { reader ->
localProperties.load(reader)
}
}
def flutterRoot = localProperties.getProperty('flutter.sdk')
if (flutterRoot == null) {
throw new GradleException("Flutter SDK not found. Define location with flutter.sdk in the local.properties file.")
}
def flutterVersionName = localProperties.getProperty('flutter.versionName')
if (flutterVersionName == null) {
throw new GradleException("versionName not found. Define flutter.versionName in the local.properties file.")
}
apply plugin: 'com.android.application'
apply from: "$flutterRoot/packages/flutter_tools/gradle/flutter.gradle"
android {
compileSdk flutter.compileSdkVersion
defaultConfig {
// TODO: Specify your own unique Application ID (https://developer.android.com/studio/build/application-id.html).
applicationId "io.flutter.packages.palettegenerator.imagecolors"
minSdkVersion 19
targetSdkVersion 33
versionCode 1
versionName flutterVersionName
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
// TODO: Add your own signing config for the release build.
// Signing with the debug keys for now, so `flutter run --release` works.
signingConfig signingConfigs.debug
}
}
namespace 'io.flutter.packages.palettegenerator.imagecolors'
lint {
disable 'InvalidPackage'
}
}
flutter {
source '../..'
}
dependencies {
testImplementation 'junit:junit:4.12'
androidTestImplementation 'androidx.test:runner:1.1.0'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.1.0'
}
| packages/packages/palette_generator/example/android/app/build.gradle/0 | {
"file_path": "packages/packages/palette_generator/example/android/app/build.gradle",
"repo_id": "packages",
"token_count": 667
} | 1,098 |
buildFlags:
_pluginToolsConfigGlobalKey:
- "--no-tree-shake-icons"
- "--dart-define=buildmode=testing"
| packages/packages/path_provider/path_provider/example/.pluginToolsConfig.yaml/0 | {
"file_path": "packages/packages/path_provider/path_provider/example/.pluginToolsConfig.yaml",
"repo_id": "packages",
"token_count": 45
} | 1,099 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
import 'package:path_provider/path_provider.dart';
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
testWidgets('getTemporaryDirectory', (WidgetTester tester) async {
final Directory result = await getTemporaryDirectory();
_verifySampleFile(result, 'temporaryDirectory');
});
testWidgets('getApplicationDocumentsDirectory', (WidgetTester tester) async {
final Directory result = await getApplicationDocumentsDirectory();
_verifySampleFile(result, 'applicationDocuments');
});
testWidgets('getApplicationSupportDirectory', (WidgetTester tester) async {
final Directory result = await getApplicationSupportDirectory();
_verifySampleFile(result, 'applicationSupport');
});
testWidgets('getApplicationCacheDirectory', (WidgetTester tester) async {
final Directory result = await getApplicationCacheDirectory();
_verifySampleFile(result, 'applicationCache');
});
testWidgets('getLibraryDirectory', (WidgetTester tester) async {
if (Platform.isIOS) {
final Directory result = await getLibraryDirectory();
_verifySampleFile(result, 'library');
} else if (Platform.isAndroid) {
final Future<Directory?> result = getLibraryDirectory();
await expectLater(result, throwsA(isInstanceOf<UnsupportedError>()));
}
});
testWidgets('getExternalStorageDirectory', (WidgetTester tester) async {
if (Platform.isIOS) {
final Future<Directory?> result = getExternalStorageDirectory();
await expectLater(result, throwsA(isInstanceOf<UnsupportedError>()));
} else if (Platform.isAndroid) {
final Directory? result = await getExternalStorageDirectory();
_verifySampleFile(result, 'externalStorage');
}
});
testWidgets('getExternalCacheDirectories', (WidgetTester tester) async {
if (Platform.isIOS) {
final Future<List<Directory>?> result = getExternalCacheDirectories();
await expectLater(result, throwsA(isInstanceOf<UnsupportedError>()));
} else if (Platform.isAndroid) {
final List<Directory>? directories = await getExternalCacheDirectories();
expect(directories, isNotNull);
for (final Directory result in directories!) {
_verifySampleFile(result, 'externalCache');
}
}
});
final List<StorageDirectory?> allDirs = <StorageDirectory?>[
null,
StorageDirectory.music,
StorageDirectory.podcasts,
StorageDirectory.ringtones,
StorageDirectory.alarms,
StorageDirectory.notifications,
StorageDirectory.pictures,
StorageDirectory.movies,
];
for (final StorageDirectory? type in allDirs) {
testWidgets('getExternalStorageDirectories (type: $type)',
(WidgetTester tester) async {
if (Platform.isIOS) {
final Future<List<Directory>?> result = getExternalStorageDirectories();
await expectLater(result, throwsA(isInstanceOf<UnsupportedError>()));
} else if (Platform.isAndroid) {
final List<Directory>? directories =
await getExternalStorageDirectories(type: type);
expect(directories, isNotNull);
for (final Directory result in directories!) {
_verifySampleFile(result, '$type');
}
}
});
}
testWidgets('getDownloadsDirectory', (WidgetTester tester) async {
final Directory? result = await getDownloadsDirectory();
// On recent versions of macOS, actually using the downloads directory
// requires a user prompt (so will fail on CI), and on some platforms the
// directory may not exist. Instead of verifying that it exists, just
// check that it returned a path.
expect(result?.path, isNotEmpty);
});
}
/// Verify a file called [name] in [directory] by recreating it with test
/// contents when necessary.
void _verifySampleFile(Directory? directory, String name) {
expect(directory, isNotNull);
if (directory == null) {
return;
}
final File file = File('${directory.path}/$name');
if (file.existsSync()) {
file.deleteSync();
expect(file.existsSync(), isFalse);
}
file.writeAsStringSync('Hello world!');
expect(file.readAsStringSync(), 'Hello world!');
// This check intentionally avoids using Directory.listSync on Android due to
// https://github.com/dart-lang/sdk/issues/54287.
if (Platform.isAndroid) {
expect(
Process.runSync('ls', <String>[directory.path]).stdout, contains(name));
} else {
expect(directory.listSync(), isNotEmpty);
}
file.deleteSync();
}
| packages/packages/path_provider/path_provider/example/integration_test/path_provider_test.dart/0 | {
"file_path": "packages/packages/path_provider/path_provider/example/integration_test/path_provider_test.dart",
"repo_id": "packages",
"token_count": 1533
} | 1,100 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@import path_provider;
@import XCTest;
@interface PathProviderTests : XCTestCase
@end
@implementation PathProviderTests
- (void)testPlugin {
FLTPathProviderPlugin *plugin = [[FLTPathProviderPlugin alloc] init];
XCTAssertNotNil(plugin);
}
@end
| packages/packages/path_provider/path_provider/example/ios/RunnerTests/PathProviderTests.m/0 | {
"file_path": "packages/packages/path_provider/path_provider/example/ios/RunnerTests/PathProviderTests.m",
"repo_id": "packages",
"token_count": 129
} | 1,101 |
rootProject.name = 'path_provider_android'
| packages/packages/path_provider/path_provider_android/android/settings.gradle/0 | {
"file_path": "packages/packages/path_provider/path_provider_android/android/settings.gradle",
"repo_id": "packages",
"token_count": 14
} | 1,102 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:path_provider_android/messages.g.dart' as messages;
import 'package:path_provider_android/path_provider_android.dart';
import 'package:path_provider_platform_interface/path_provider_platform_interface.dart';
import 'messages_test.g.dart';
const String kTemporaryPath = 'temporaryPath';
const String kApplicationSupportPath = 'applicationSupportPath';
const String kApplicationDocumentsPath = 'applicationDocumentsPath';
const String kApplicationCachePath = 'applicationCachePath';
const String kExternalCachePaths = 'externalCachePaths';
const String kExternalStoragePaths = 'externalStoragePaths';
class _Api implements TestPathProviderApi {
_Api({this.returnsExternalStoragePaths = true});
final bool returnsExternalStoragePaths;
@override
String? getApplicationDocumentsPath() => kApplicationDocumentsPath;
@override
String? getApplicationSupportPath() => kApplicationSupportPath;
@override
String? getApplicationCachePath() => kApplicationCachePath;
@override
List<String?> getExternalCachePaths() => <String>[kExternalCachePaths];
@override
String? getExternalStoragePath() => kExternalStoragePaths;
@override
List<String?> getExternalStoragePaths(messages.StorageDirectory directory) {
return <String?>[if (returnsExternalStoragePaths) kExternalStoragePaths];
}
@override
String? getTemporaryPath() => kTemporaryPath;
}
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
group('PathProviderAndroid', () {
late PathProviderAndroid pathProvider;
setUp(() async {
pathProvider = PathProviderAndroid();
TestPathProviderApi.setup(_Api());
});
test('getTemporaryPath', () async {
final String? path = await pathProvider.getTemporaryPath();
expect(path, kTemporaryPath);
});
test('getApplicationSupportPath', () async {
final String? path = await pathProvider.getApplicationSupportPath();
expect(path, kApplicationSupportPath);
});
test('getApplicationCachePath', () async {
final String? path = await pathProvider.getApplicationCachePath();
expect(path, kApplicationCachePath);
});
test('getLibraryPath fails', () async {
try {
await pathProvider.getLibraryPath();
fail('should throw UnsupportedError');
} catch (e) {
expect(e, isUnsupportedError);
}
});
test('getApplicationDocumentsPath', () async {
final String? path = await pathProvider.getApplicationDocumentsPath();
expect(path, kApplicationDocumentsPath);
});
test('getExternalCachePaths succeeds', () async {
final List<String>? result = await pathProvider.getExternalCachePaths();
expect(result!.length, 1);
expect(result.first, kExternalCachePaths);
});
for (final StorageDirectory? type in <StorageDirectory?>[
...StorageDirectory.values
]) {
test('getExternalStoragePaths (type: $type) android succeeds', () async {
final List<String>? result =
await pathProvider.getExternalStoragePaths(type: type);
expect(result!.length, 1);
expect(result.first, kExternalStoragePaths);
});
} // end of for-loop
test('getDownloadsPath succeeds', () async {
final String? path = await pathProvider.getDownloadsPath();
expect(path, kExternalStoragePaths);
});
test(
'getDownloadsPath returns null, when getExternalStoragePaths returns '
'an empty list', () async {
final PathProviderAndroid pathProvider = PathProviderAndroid();
TestPathProviderApi.setup(_Api(returnsExternalStoragePaths: false));
final String? path = await pathProvider.getDownloadsPath();
expect(path, null);
});
});
}
| packages/packages/path_provider/path_provider_android/test/path_provider_android_test.dart/0 | {
"file_path": "packages/packages/path_provider/path_provider_android/test/path_provider_android_test.dart",
"repo_id": "packages",
"token_count": 1268
} | 1,103 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ffi';
import 'package:ffi/ffi.dart';
import 'package:flutter/foundation.dart' show visibleForTesting;
// GApplication* g_application_get_default();
typedef _GApplicationGetDefaultC = IntPtr Function();
typedef _GApplicationGetDefaultDart = int Function();
// const gchar* g_application_get_application_id(GApplication* application);
typedef _GApplicationGetApplicationIdC = Pointer<Utf8> Function(IntPtr);
typedef _GApplicationGetApplicationIdDart = Pointer<Utf8> Function(int);
/// Interface for interacting with libgio.
@visibleForTesting
class GioUtils {
/// Creates a default instance that uses the real libgio.
GioUtils() {
try {
_gio = DynamicLibrary.open('libgio-2.0.so');
} on ArgumentError {
_gio = null;
}
}
DynamicLibrary? _gio;
/// True if libgio was opened successfully.
bool get libraryIsPresent => _gio != null;
/// Wraps `g_application_get_default`.
int gApplicationGetDefault() {
if (_gio == null) {
return 0;
}
final _GApplicationGetDefaultDart getDefault = _gio!
.lookupFunction<_GApplicationGetDefaultC, _GApplicationGetDefaultDart>(
'g_application_get_default');
return getDefault();
}
/// Wraps g_application_get_application_id.
Pointer<Utf8> gApplicationGetApplicationId(int app) {
if (_gio == null) {
return nullptr;
}
final _GApplicationGetApplicationIdDart gApplicationGetApplicationId = _gio!
.lookupFunction<_GApplicationGetApplicationIdC,
_GApplicationGetApplicationIdDart>(
'g_application_get_application_id');
return gApplicationGetApplicationId(app);
}
}
/// Allows overriding the default GioUtils instance with a fake for testing.
@visibleForTesting
GioUtils? gioUtilsOverride;
/// Gets the application ID for this app.
String? getApplicationId() {
final GioUtils gio = gioUtilsOverride ?? GioUtils();
if (!gio.libraryIsPresent) {
return null;
}
final int app = gio.gApplicationGetDefault();
if (app == 0) {
return null;
}
final Pointer<Utf8> appId = gio.gApplicationGetApplicationId(app);
if (appId == nullptr) {
return null;
}
return appId.toDartString();
}
| packages/packages/path_provider/path_provider_linux/lib/src/get_application_id_real.dart/0 | {
"file_path": "packages/packages/path_provider/path_provider_linux/lib/src/get_application_id_real.dart",
"repo_id": "packages",
"token_count": 813
} | 1,104 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:code_builder/code_builder.dart' as cb;
import 'package:dart_style/dart_style.dart';
import 'package:path/path.dart' as path;
import 'ast.dart';
import 'dart/templates.dart';
import 'functional.dart';
import 'generator.dart';
import 'generator_tools.dart';
/// Documentation comment open symbol.
const String _docCommentPrefix = '///';
/// Prefix for all local variables in host API methods.
///
/// This lowers the chances of variable name collisions with
/// user defined parameters.
const String _varNamePrefix = '__pigeon_';
/// Name of the `InstanceManager` variable for a ProxyApi class;
const String _instanceManagerVarName =
'${classMemberNamePrefix}instanceManager';
/// Name of field used for host API codec.
const String _pigeonChannelCodec = 'pigeonChannelCodec';
/// Documentation comment spec.
const DocumentCommentSpecification _docCommentSpec =
DocumentCommentSpecification(_docCommentPrefix);
/// The standard codec for Flutter, used for any non custom codecs and extended for custom codecs.
const String _standardMessageCodec = 'StandardMessageCodec';
/// Options that control how Dart code will be generated.
class DartOptions {
/// Constructor for DartOptions.
const DartOptions({
this.copyrightHeader,
this.sourceOutPath,
this.testOutPath,
});
/// A copyright header that will get prepended to generated code.
final Iterable<String>? copyrightHeader;
/// Path to output generated Dart file.
final String? sourceOutPath;
/// Path to output generated Test file for tests.
final String? testOutPath;
/// Creates a [DartOptions] from a Map representation where:
/// `x = DartOptions.fromMap(x.toMap())`.
static DartOptions fromMap(Map<String, Object> map) {
final Iterable<dynamic>? copyrightHeader =
map['copyrightHeader'] as Iterable<dynamic>?;
return DartOptions(
copyrightHeader: copyrightHeader?.cast<String>(),
sourceOutPath: map['sourceOutPath'] as String?,
testOutPath: map['testOutPath'] as String?,
);
}
/// Converts a [DartOptions] to a Map representation where:
/// `x = DartOptions.fromMap(x.toMap())`.
Map<String, Object> toMap() {
final Map<String, Object> result = <String, Object>{
if (copyrightHeader != null) 'copyrightHeader': copyrightHeader!,
if (sourceOutPath != null) 'sourceOutPath': sourceOutPath!,
if (testOutPath != null) 'testOutPath': testOutPath!,
};
return result;
}
/// Overrides any non-null parameters from [options] into this to make a new
/// [DartOptions].
DartOptions merge(DartOptions options) {
return DartOptions.fromMap(mergeMaps(toMap(), options.toMap()));
}
}
/// Class that manages all Dart code generation.
class DartGenerator extends StructuredGenerator<DartOptions> {
/// Instantiates a Dart Generator.
const DartGenerator();
@override
void writeFilePrologue(
DartOptions generatorOptions,
Root root,
Indent indent, {
required String dartPackageName,
}) {
if (generatorOptions.copyrightHeader != null) {
addLines(indent, generatorOptions.copyrightHeader!, linePrefix: '// ');
}
indent.writeln('// ${getGeneratedCodeWarning()}');
indent.writeln('// $seeAlsoWarning');
indent.writeln(
'// ignore_for_file: public_member_api_docs, non_constant_identifier_names, avoid_as, unused_import, unnecessary_parenthesis, prefer_null_aware_operators, omit_local_variable_types, unused_shown_name, unnecessary_import, no_leading_underscores_for_local_identifiers',
);
indent.newln();
}
@override
void writeFileImports(
DartOptions generatorOptions,
Root root,
Indent indent, {
required String dartPackageName,
}) {
indent.writeln("import 'dart:async';");
indent.writeln(
"import 'dart:typed_data' show Float64List, Int32List, Int64List, Uint8List;",
);
indent.newln();
final bool hasProxyApi = root.apis.any((Api api) => api is AstProxyApi);
indent.writeln(
"import 'package:flutter/foundation.dart' show ReadBuffer, WriteBuffer${hasProxyApi ? ', immutable, protected' : ''};");
indent.writeln("import 'package:flutter/services.dart';");
if (hasProxyApi) {
indent.writeln(
"import 'package:flutter/widgets.dart' show WidgetsFlutterBinding;",
);
}
}
@override
void writeEnum(
DartOptions generatorOptions,
Root root,
Indent indent,
Enum anEnum, {
required String dartPackageName,
}) {
indent.newln();
addDocumentationComments(
indent, anEnum.documentationComments, _docCommentSpec);
indent.write('enum ${anEnum.name} ');
indent.addScoped('{', '}', () {
for (final EnumMember member in anEnum.members) {
addDocumentationComments(
indent, member.documentationComments, _docCommentSpec);
indent.writeln('${member.name},');
}
});
}
@override
void writeDataClass(
DartOptions generatorOptions,
Root root,
Indent indent,
Class classDefinition, {
required String dartPackageName,
}) {
indent.newln();
addDocumentationComments(
indent, classDefinition.documentationComments, _docCommentSpec);
indent.write('class ${classDefinition.name} ');
indent.addScoped('{', '}', () {
_writeConstructor(indent, classDefinition);
indent.newln();
for (final NamedType field
in getFieldsInSerializationOrder(classDefinition)) {
addDocumentationComments(
indent, field.documentationComments, _docCommentSpec);
final String datatype = _addGenericTypesNullable(field.type);
indent.writeln('$datatype ${field.name};');
indent.newln();
}
writeClassEncode(
generatorOptions,
root,
indent,
classDefinition,
dartPackageName: dartPackageName,
);
indent.newln();
writeClassDecode(
generatorOptions,
root,
indent,
classDefinition,
dartPackageName: dartPackageName,
);
});
}
void _writeConstructor(Indent indent, Class classDefinition) {
indent.write(classDefinition.name);
indent.addScoped('({', '});', () {
for (final NamedType field
in getFieldsInSerializationOrder(classDefinition)) {
final String required =
!field.type.isNullable && field.defaultValue == null
? 'required '
: '';
final String defaultValueString =
field.defaultValue == null ? '' : ' = ${field.defaultValue}';
indent.writeln('${required}this.${field.name}$defaultValueString,');
}
});
}
@override
void writeClassEncode(
DartOptions generatorOptions,
Root root,
Indent indent,
Class classDefinition, {
required String dartPackageName,
}) {
indent.write('Object encode() ');
indent.addScoped('{', '}', () {
indent.write(
'return <Object?>',
);
indent.addScoped('[', '];', () {
for (final NamedType field
in getFieldsInSerializationOrder(classDefinition)) {
final String conditional = field.type.isNullable ? '?' : '';
if (field.type.isClass) {
indent.writeln(
'${field.name}$conditional.encode(),',
);
} else if (field.type.isEnum) {
indent.writeln(
'${field.name}$conditional.index,',
);
} else {
indent.writeln('${field.name},');
}
}
});
});
}
@override
void writeClassDecode(
DartOptions generatorOptions,
Root root,
Indent indent,
Class classDefinition, {
required String dartPackageName,
}) {
void writeValueDecode(NamedType field, int index) {
final String resultAt = 'result[$index]';
final String castCallPrefix = field.type.isNullable ? '?' : '!';
final String genericType = _makeGenericTypeArguments(field.type);
final String castCall = _makeGenericCastCall(field.type);
final String nullableTag = field.type.isNullable ? '?' : '';
if (field.type.isClass) {
final String nonNullValue =
'${field.type.baseName}.decode($resultAt! as List<Object?>)';
if (field.type.isNullable) {
indent.format('''
$resultAt != null
\t\t? $nonNullValue
\t\t: null''', leadingSpace: false, trailingNewline: false);
} else {
indent.add(nonNullValue);
}
} else if (field.type.isEnum) {
final String nonNullValue =
'${field.type.baseName}.values[$resultAt! as int]';
if (field.type.isNullable) {
indent.format('''
$resultAt != null
\t\t? $nonNullValue
\t\t: null''', leadingSpace: false, trailingNewline: false);
} else {
indent.add(nonNullValue);
}
} else if (field.type.typeArguments.isNotEmpty) {
indent.add(
'($resultAt as $genericType?)$castCallPrefix$castCall',
);
} else {
final String castCallForcePrefix = field.type.isNullable ? '' : '!';
final String castString = field.type.baseName == 'Object'
? ''
: ' as $genericType$nullableTag';
indent.add(
'$resultAt$castCallForcePrefix$castString',
);
}
}
indent.write(
'static ${classDefinition.name} decode(Object result) ',
);
indent.addScoped('{', '}', () {
indent.writeln('result as List<Object?>;');
indent.write('return ${classDefinition.name}');
indent.addScoped('(', ');', () {
enumerate(getFieldsInSerializationOrder(classDefinition),
(int index, final NamedType field) {
indent.write('${field.name}: ');
writeValueDecode(field, index);
indent.addln(',');
});
});
});
}
/// Writes the code for host [Api], [api].
/// Example:
/// class FooCodec extends StandardMessageCodec {...}
///
/// abstract class Foo {
/// static const MessageCodec<Object?> codec = FooCodec();
/// int add(int x, int y);
/// static void setup(Foo api, {BinaryMessenger? binaryMessenger}) {...}
/// }
@override
void writeFlutterApi(
DartOptions generatorOptions,
Root root,
Indent indent,
AstFlutterApi api, {
String Function(Method)? channelNameFunc,
bool isMockHandler = false,
required String dartPackageName,
}) {
String codecName = _standardMessageCodec;
if (getCodecClasses(api, root).isNotEmpty) {
codecName = _getCodecName(api);
_writeCodec(indent, codecName, api, root);
}
indent.newln();
addDocumentationComments(
indent, api.documentationComments, _docCommentSpec);
indent.write('abstract class ${api.name} ');
indent.addScoped('{', '}', () {
if (isMockHandler) {
indent.writeln(
'static TestDefaultBinaryMessengerBinding? get _testBinaryMessengerBinding => TestDefaultBinaryMessengerBinding.instance;');
}
indent.writeln(
'static const MessageCodec<Object?> $_pigeonChannelCodec = $codecName();');
indent.newln();
for (final Method func in api.methods) {
addDocumentationComments(
indent, func.documentationComments, _docCommentSpec);
final bool isAsync = func.isAsynchronous;
final String returnType = isAsync
? 'Future<${_addGenericTypesNullable(func.returnType)}>'
: _addGenericTypesNullable(func.returnType);
final String argSignature =
_getMethodParameterSignature(func.parameters);
indent.writeln('$returnType ${func.name}($argSignature);');
indent.newln();
}
indent.write(
'static void setup(${api.name}? api, {BinaryMessenger? binaryMessenger}) ');
indent.addScoped('{', '}', () {
for (final Method func in api.methods) {
_writeFlutterMethodMessageHandler(
indent,
name: func.name,
parameters: func.parameters,
returnType: func.returnType,
channelName: channelNameFunc == null
? makeChannelName(api, func, dartPackageName)
: channelNameFunc(func),
isMockHandler: isMockHandler,
isAsynchronous: func.isAsynchronous,
);
}
});
});
}
/// Writes the code for host [Api], [api].
/// Example:
/// class FooCodec extends StandardMessageCodec {...}
///
/// class Foo {
/// Foo(BinaryMessenger? binaryMessenger) {}
/// static const MessageCodec<Object?> codec = FooCodec();
/// Future<int> add(int x, int y) async {...}
/// }
///
/// Messages will be sent and received in a list.
///
/// If the message received was successful,
/// the result will be contained at the 0'th index.
///
/// If the message was a failure, the list will contain 3 items:
/// a code, a message, and details in that order.
@override
void writeHostApi(
DartOptions generatorOptions,
Root root,
Indent indent,
AstHostApi api, {
required String dartPackageName,
}) {
String codecName = _standardMessageCodec;
if (getCodecClasses(api, root).isNotEmpty) {
codecName = _getCodecName(api);
_writeCodec(indent, codecName, api, root);
}
indent.newln();
bool first = true;
addDocumentationComments(
indent, api.documentationComments, _docCommentSpec);
indent.write('class ${api.name} ');
indent.addScoped('{', '}', () {
indent.format('''
/// Constructor for [${api.name}]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
${api.name}({BinaryMessenger? binaryMessenger})
\t\t: ${_varNamePrefix}binaryMessenger = binaryMessenger;
final BinaryMessenger? ${_varNamePrefix}binaryMessenger;
''');
indent.writeln(
'static const MessageCodec<Object?> $_pigeonChannelCodec = $codecName();');
indent.newln();
for (final Method func in api.methods) {
if (!first) {
indent.newln();
} else {
first = false;
}
_writeHostMethod(
indent,
name: func.name,
parameters: func.parameters,
returnType: func.returnType,
documentationComments: func.documentationComments,
channelName: makeChannelName(api, func, dartPackageName),
);
}
});
}
@override
void writeInstanceManager(
DartOptions generatorOptions,
Root root,
Indent indent, {
required String dartPackageName,
}) {
indent.format(proxyApiBaseClass);
indent.format(
instanceManagerTemplate(
allProxyApiNames: root.apis
.whereType<AstProxyApi>()
.map((AstProxyApi api) => api.name),
),
);
}
@override
void writeInstanceManagerApi(
DartOptions generatorOptions,
Root root,
Indent indent, {
required String dartPackageName,
}) {
indent.format(
instanceManagerApiTemplate(
dartPackageName: dartPackageName,
pigeonChannelCodecVarName: _pigeonChannelCodec,
),
);
}
@override
void writeProxyApiBaseCodec(
DartOptions generatorOptions,
Root root,
Indent indent,
) {
indent.format(proxyApiBaseCodec);
}
@override
void writeProxyApi(
DartOptions generatorOptions,
Root root,
Indent indent,
AstProxyApi api, {
required String dartPackageName,
}) {
const String codecName = '_${classNamePrefix}ProxyApiBaseCodec';
// Each API has a private codec instance used by every host method,
// constructor, or non-static field.
final String codecInstanceName = '${_varNamePrefix}codec${api.name}';
// AST class used by code_builder to generate the code.
final cb.Class proxyApi = cb.Class(
(cb.ClassBuilder builder) => builder
..name = api.name
..extend = api.superClass != null
? cb.refer(api.superClass!.baseName)
: cb.refer(proxyApiBaseClassName)
..implements.addAll(
api.interfaces.map(
(TypeDeclaration type) => cb.refer(type.baseName),
),
)
..docs.addAll(
asDocumentationComments(api.documentationComments, _docCommentSpec),
)
..constructors.addAll(_proxyApiConstructors(
api.constructors,
apiName: api.name,
dartPackageName: dartPackageName,
codecName: codecName,
codecInstanceName: codecInstanceName,
superClassApi: api.superClass?.associatedProxyApi,
unattachedFields: api.unattachedFields,
flutterMethodsFromSuperClasses: api.flutterMethodsFromSuperClasses(),
flutterMethodsFromInterfaces: api.flutterMethodsFromInterfaces(),
declaredFlutterMethods: api.flutterMethods,
))
..constructors.add(
_proxyApiDetachedConstructor(
apiName: api.name,
superClassApi: api.superClass?.associatedProxyApi,
unattachedFields: api.unattachedFields,
flutterMethodsFromSuperClasses:
api.flutterMethodsFromSuperClasses(),
flutterMethodsFromInterfaces: api.flutterMethodsFromInterfaces(),
declaredFlutterMethods: api.flutterMethods,
),
)
..fields.addAll(<cb.Field>[
if (api.constructors.isNotEmpty ||
api.attachedFields.any((ApiField field) => !field.isStatic) ||
api.hostMethods.isNotEmpty)
_proxyApiCodecInstanceField(
codecInstanceName: codecInstanceName,
codecName: codecName,
),
])
..fields.addAll(_proxyApiUnattachedFields(api.unattachedFields))
..fields.addAll(_proxyApiFlutterMethodFields(
api.flutterMethods,
apiName: api.name,
))
..fields.addAll(_proxyApiInterfaceApiFields(api.apisOfInterfaces()))
..fields.addAll(_proxyApiAttachedFields(api.attachedFields))
..methods.add(
_proxyApiSetUpMessageHandlerMethod(
flutterMethods: api.flutterMethods,
apiName: api.name,
dartPackageName: dartPackageName,
codecName: codecName,
unattachedFields: api.unattachedFields,
hasCallbackConstructor: api.hasCallbackConstructor(),
),
)
..methods.addAll(
_proxyApiAttachedFieldMethods(
api.attachedFields,
apiName: api.name,
dartPackageName: dartPackageName,
codecInstanceName: codecInstanceName,
codecName: codecName,
),
)
..methods.addAll(_proxyApiHostMethods(
api.hostMethods,
apiName: api.name,
dartPackageName: dartPackageName,
codecInstanceName: codecInstanceName,
codecName: codecName,
))
..methods.add(
_proxyApiCopyMethod(
apiName: api.name,
unattachedFields: api.unattachedFields,
declaredAndInheritedFlutterMethods: api
.flutterMethodsFromSuperClasses()
.followedBy(api.flutterMethodsFromInterfaces())
.followedBy(api.flutterMethods),
),
),
);
final cb.DartEmitter emitter = cb.DartEmitter(useNullSafetySyntax: true);
indent.format(DartFormatter().format('${proxyApi.accept(emitter)}'));
}
/// Generates Dart source code for test support libraries based on the given AST
/// represented by [root], outputting the code to [sink]. [sourceOutPath] is the
/// path of the generated dart code to be tested. [testOutPath] is where the
/// test code will be generated.
void generateTest(
DartOptions generatorOptions,
Root root,
StringSink sink, {
required String dartPackageName,
required String dartOutputPackageName,
}) {
final Indent indent = Indent(sink);
final String sourceOutPath = generatorOptions.sourceOutPath ?? '';
final String testOutPath = generatorOptions.testOutPath ?? '';
_writeTestPrologue(generatorOptions, root, indent);
_writeTestImports(generatorOptions, root, indent);
final String relativeDartPath =
path.Context(style: path.Style.posix).relative(
_posixify(sourceOutPath),
from: _posixify(path.dirname(testOutPath)),
);
if (!relativeDartPath.contains('/lib/')) {
// If we can't figure out the package name or the relative path doesn't
// include a 'lib' directory, try relative path import which only works in
// certain (older) versions of Dart.
// TODO(gaaclarke): We should add a command-line parameter to override this import.
indent.writeln(
"import '${_escapeForDartSingleQuotedString(relativeDartPath)}';");
} else {
final String path =
relativeDartPath.replaceFirst(RegExp(r'^.*/lib/'), '');
indent.writeln("import 'package:$dartOutputPackageName/$path';");
}
for (final AstHostApi api in root.apis.whereType<AstHostApi>()) {
if (api.dartHostTestHandler != null) {
final AstFlutterApi mockApi = AstFlutterApi(
name: api.dartHostTestHandler!,
methods: api.methods,
documentationComments: api.documentationComments,
);
writeFlutterApi(
generatorOptions,
root,
indent,
mockApi,
channelNameFunc: (Method func) =>
makeChannelName(api, func, dartPackageName),
isMockHandler: true,
dartPackageName: dartPackageName,
);
}
}
}
/// Writes file header to sink.
void _writeTestPrologue(DartOptions opt, Root root, Indent indent) {
if (opt.copyrightHeader != null) {
addLines(indent, opt.copyrightHeader!, linePrefix: '// ');
}
indent.writeln('// ${getGeneratedCodeWarning()}');
indent.writeln('// $seeAlsoWarning');
indent.writeln(
'// ignore_for_file: public_member_api_docs, non_constant_identifier_names, avoid_as, unused_import, unnecessary_parenthesis, unnecessary_import, no_leading_underscores_for_local_identifiers',
);
indent.writeln('// ignore_for_file: avoid_relative_lib_imports');
}
/// Writes file imports to sink.
void _writeTestImports(DartOptions opt, Root root, Indent indent) {
indent.writeln("import 'dart:async';");
indent.writeln(
"import 'dart:typed_data' show Float64List, Int32List, Int64List, Uint8List;",
);
indent.writeln(
"import 'package:flutter/foundation.dart' show ReadBuffer, WriteBuffer;");
indent.writeln("import 'package:flutter/services.dart';");
indent.writeln("import 'package:flutter_test/flutter_test.dart';");
indent.newln();
}
@override
void writeGeneralUtilities(
DartOptions generatorOptions,
Root root,
Indent indent, {
required String dartPackageName,
}) {
final bool hasHostMethod = root.apis
.whereType<AstHostApi>()
.any((AstHostApi api) => api.methods.isNotEmpty) ||
root.apis.whereType<AstProxyApi>().any((AstProxyApi api) =>
api.constructors.isNotEmpty ||
api.attachedFields.isNotEmpty ||
api.hostMethods.isNotEmpty);
final bool hasFlutterMethod = root.apis
.whereType<AstFlutterApi>()
.any((AstFlutterApi api) => api.methods.isNotEmpty) ||
root.apis.any((Api api) => api is AstProxyApi);
if (hasHostMethod) {
_writeCreateConnectionError(indent);
}
if (hasFlutterMethod || generatorOptions.testOutPath != null) {
_writeWrapResponse(generatorOptions, root, indent);
}
}
/// Writes [wrapResponse] method.
void _writeWrapResponse(DartOptions opt, Root root, Indent indent) {
indent.newln();
indent.writeScoped(
'List<Object?> wrapResponse({Object? result, PlatformException? error, bool empty = false}) {',
'}', () {
indent.writeScoped('if (empty) {', '}', () {
indent.writeln('return <Object?>[];');
});
indent.writeScoped('if (error == null) {', '}', () {
indent.writeln('return <Object?>[result];');
});
indent.writeln(
'return <Object?>[error.code, error.message, error.details];');
});
}
void _writeCreateConnectionError(Indent indent) {
indent.newln();
indent.format('''
PlatformException _createConnectionError(String channelName) {
\treturn PlatformException(
\t\tcode: 'channel-error',
\t\tmessage: 'Unable to establish connection on channel: "\$channelName".',
\t);
}''');
}
void _writeHostMethod(
Indent indent, {
required String name,
required Iterable<Parameter> parameters,
required TypeDeclaration returnType,
required List<String> documentationComments,
required String channelName,
}) {
addDocumentationComments(indent, documentationComments, _docCommentSpec);
String argSignature = '';
if (parameters.isNotEmpty) {
argSignature = _getMethodParameterSignature(parameters);
}
indent.write(
'Future<${_addGenericTypesNullable(returnType)}> $name($argSignature) async ',
);
indent.addScoped('{', '}', () {
_writeHostMethodMessageCall(
indent,
channelName: channelName,
parameters: parameters,
returnType: returnType,
);
});
}
void _writeHostMethodMessageCall(
Indent indent, {
required String channelName,
required Iterable<Parameter> parameters,
required TypeDeclaration returnType,
}) {
String sendArgument = 'null';
if (parameters.isNotEmpty) {
final Iterable<String> argExpressions =
indexMap(parameters, (int index, NamedType type) {
final String name = _getParameterName(index, type);
if (type.type.isEnum) {
return '$name${type.type.isNullable ? '?' : ''}.index';
} else {
return name;
}
});
sendArgument = '<Object?>[${argExpressions.join(', ')}]';
}
indent
.writeln("const String ${_varNamePrefix}channelName = '$channelName';");
indent.writeScoped(
'final BasicMessageChannel<Object?> ${_varNamePrefix}channel = BasicMessageChannel<Object?>(',
');', () {
indent.writeln('${_varNamePrefix}channelName,');
indent.writeln('$_pigeonChannelCodec,');
indent.writeln('binaryMessenger: ${_varNamePrefix}binaryMessenger,');
});
final String returnTypeName = _makeGenericTypeArguments(returnType);
final String genericCastCall = _makeGenericCastCall(returnType);
const String accessor = '${_varNamePrefix}replyList[0]';
// Avoid warnings from pointlessly casting to `Object?`.
final String nullablyTypedAccessor = returnTypeName == 'Object'
? accessor
: '($accessor as $returnTypeName?)';
final String nullHandler =
returnType.isNullable ? (genericCastCall.isEmpty ? '' : '?') : '!';
String returnStatement = 'return';
if (returnType.isEnum) {
if (returnType.isNullable) {
returnStatement =
'$returnStatement ($accessor as int?) == null ? null : $returnTypeName.values[$accessor! as int]';
} else {
returnStatement =
'$returnStatement $returnTypeName.values[$accessor! as int]';
}
} else if (!returnType.isVoid) {
returnStatement =
'$returnStatement $nullablyTypedAccessor$nullHandler$genericCastCall';
}
returnStatement = '$returnStatement;';
indent.format('''
final List<Object?>? ${_varNamePrefix}replyList =
\t\tawait ${_varNamePrefix}channel.send($sendArgument) as List<Object?>?;
if (${_varNamePrefix}replyList == null) {
\tthrow _createConnectionError(${_varNamePrefix}channelName);
} else if (${_varNamePrefix}replyList.length > 1) {
\tthrow PlatformException(
\t\tcode: ${_varNamePrefix}replyList[0]! as String,
\t\tmessage: ${_varNamePrefix}replyList[1] as String?,
\t\tdetails: ${_varNamePrefix}replyList[2],
\t);''');
// On iOS we can return nil from functions to accommodate error
// handling. Returning a nil value and not returning an error is an
// exception.
if (!returnType.isNullable && !returnType.isVoid) {
indent.format('''
} else if (${_varNamePrefix}replyList[0] == null) {
\tthrow PlatformException(
\t\tcode: 'null-error',
\t\tmessage: 'Host platform returned null value for non-null return value.',
\t);''');
}
indent.format('''
} else {
\t$returnStatement
}''');
}
void _writeFlutterMethodMessageHandler(
Indent indent, {
required String name,
required Iterable<Parameter> parameters,
required TypeDeclaration returnType,
required String channelName,
required bool isMockHandler,
required bool isAsynchronous,
String nullHandlerExpression = 'api == null',
String Function(String methodName, Iterable<Parameter> parameters,
Iterable<String> safeArgumentNames)
onCreateApiCall = _createFlutterApiMethodCall,
}) {
indent.write('');
indent.addScoped('{', '}', () {
indent.writeln(
'final BasicMessageChannel<Object?> ${_varNamePrefix}channel = BasicMessageChannel<Object?>(',
);
indent.nest(2, () {
indent.writeln("'$channelName', $_pigeonChannelCodec,");
indent.writeln(
'binaryMessenger: binaryMessenger);',
);
});
final String messageHandlerSetterWithOpeningParentheses = isMockHandler
? '_testBinaryMessengerBinding!.defaultBinaryMessenger.setMockDecodedMessageHandler<Object?>(${_varNamePrefix}channel, '
: '${_varNamePrefix}channel.setMessageHandler(';
indent.write('if ($nullHandlerExpression) ');
indent.addScoped('{', '}', () {
indent.writeln('${messageHandlerSetterWithOpeningParentheses}null);');
}, addTrailingNewline: false);
indent.add(' else ');
indent.addScoped('{', '}', () {
indent.write(
'$messageHandlerSetterWithOpeningParentheses(Object? message) async ',
);
indent.addScoped('{', '});', () {
final String returnTypeString = _addGenericTypesNullable(returnType);
final bool isAsync = isAsynchronous;
const String emptyReturnStatement =
'return wrapResponse(empty: true);';
String call;
if (parameters.isEmpty) {
call = 'api.$name()';
} else {
indent.writeln('assert(message != null,');
indent.writeln("'Argument for $channelName was null.');");
const String argsArray = 'args';
indent.writeln(
'final List<Object?> $argsArray = (message as List<Object?>?)!;');
String argNameFunc(int index, NamedType type) =>
_getSafeArgumentName(index, type);
enumerate(parameters, (int count, NamedType arg) {
final String argType = _addGenericTypes(arg.type);
final String argName = argNameFunc(count, arg);
final String genericArgType = _makeGenericTypeArguments(arg.type);
final String castCall = _makeGenericCastCall(arg.type);
final String leftHandSide = 'final $argType? $argName';
if (arg.type.isEnum) {
indent.writeln(
'$leftHandSide = $argsArray[$count] == null ? null : $argType.values[$argsArray[$count]! as int];');
} else {
indent.writeln(
'$leftHandSide = ($argsArray[$count] as $genericArgType?)${castCall.isEmpty ? '' : '?$castCall'};');
}
if (!arg.type.isNullable) {
indent.writeln('assert($argName != null,');
indent.writeln(
" 'Argument for $channelName was null, expected non-null $argType.');");
}
});
final Iterable<String> argNames =
indexMap(parameters, (int index, Parameter field) {
final String name = _getSafeArgumentName(index, field);
return '${field.isNamed ? '${field.name}: ' : ''}$name${field.type.isNullable ? '' : '!'}';
});
call = onCreateApiCall(name, parameters, argNames);
}
indent.writeScoped('try {', '} ', () {
if (returnType.isVoid) {
if (isAsync) {
indent.writeln('await $call;');
} else {
indent.writeln('$call;');
}
indent.writeln(emptyReturnStatement);
} else {
if (isAsync) {
indent.writeln('final $returnTypeString output = await $call;');
} else {
indent.writeln('final $returnTypeString output = $call;');
}
const String returnExpression = 'output';
final String nullability = returnType.isNullable ? '?' : '';
final String valueExtraction =
returnType.isEnum ? '$nullability.index' : '';
final String returnStatement = isMockHandler
? 'return <Object?>[$returnExpression$valueExtraction];'
: 'return wrapResponse(result: $returnExpression$valueExtraction);';
indent.writeln(returnStatement);
}
}, addTrailingNewline: false);
indent.addScoped('on PlatformException catch (e) {', '}', () {
indent.writeln('return wrapResponse(error: e);');
}, addTrailingNewline: false);
indent.writeScoped('catch (e) {', '}', () {
indent.writeln(
"return wrapResponse(error: PlatformException(code: 'error', message: e.toString()));");
});
});
});
});
}
static String _createFlutterApiMethodCall(
String methodName,
Iterable<Parameter> parameters,
Iterable<String> safeArgumentNames,
) {
return 'api.$methodName(${safeArgumentNames.join(', ')})';
}
/// Converts Constructors from the pigeon AST to a `code_builder` Constructor
/// for a ProxyApi.
Iterable<cb.Constructor> _proxyApiConstructors(
Iterable<Constructor> constructors, {
required String apiName,
required String dartPackageName,
required String codecName,
required String codecInstanceName,
required AstProxyApi? superClassApi,
required Iterable<ApiField> unattachedFields,
required Iterable<Method> flutterMethodsFromSuperClasses,
required Iterable<Method> flutterMethodsFromInterfaces,
required Iterable<Method> declaredFlutterMethods,
}) sync* {
final cb.Parameter binaryMessengerParameter = cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = '${classMemberNamePrefix}binaryMessenger'
..named = true
..toSuper = true,
);
final cb.Parameter instanceManagerParameter = cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = _instanceManagerVarName
..named = true
..toSuper = true,
);
for (final Constructor constructor in constructors) {
yield cb.Constructor(
(cb.ConstructorBuilder builder) {
final String channelName = makeChannelNameWithStrings(
apiName: apiName,
methodName: constructor.name.isNotEmpty
? constructor.name
: '${classMemberNamePrefix}defaultConstructor',
dartPackageName: dartPackageName,
);
builder
..name = constructor.name.isNotEmpty ? constructor.name : null
..docs.addAll(asDocumentationComments(
constructor.documentationComments,
_docCommentSpec,
))
..optionalParameters.addAll(
<cb.Parameter>[
binaryMessengerParameter,
instanceManagerParameter,
for (final ApiField field in unattachedFields)
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = field.name
..named = true
..toThis = true
..required = !field.type.isNullable,
),
for (final Method method in flutterMethodsFromSuperClasses)
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = method.name
..named = true
..toSuper = true
..required = method.isRequired,
),
for (final Method method in flutterMethodsFromInterfaces
.followedBy(declaredFlutterMethods))
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = method.name
..named = true
..toThis = true
..required = method.isRequired,
),
...indexMap(
constructor.parameters,
(int index, NamedType parameter) => cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = _getParameterName(index, parameter)
..type = _refer(parameter.type)
..named = true
..required = !parameter.type.isNullable,
),
)
],
)
..initializers.addAll(
<cb.Code>[
if (superClassApi != null)
const cb.Code('super.${classMemberNamePrefix}detached()')
],
)
..body = cb.Block(
(cb.BlockBuilder builder) {
final StringBuffer messageCallSink = StringBuffer();
_writeHostMethodMessageCall(
Indent(messageCallSink),
channelName: channelName,
parameters: <Parameter>[
Parameter(
name: '${_varNamePrefix}instanceIdentifier',
type: const TypeDeclaration(
baseName: 'int',
isNullable: false,
),
),
...unattachedFields.map(
(ApiField field) => Parameter(
name: field.name,
type: field.type,
),
),
...constructor.parameters,
],
returnType: const TypeDeclaration.voidDeclaration(),
);
builder.statements.addAll(<cb.Code>[
const cb.Code(
'final int ${_varNamePrefix}instanceIdentifier = $_instanceManagerVarName.addDartCreatedInstance(this);',
),
cb.Code('final $codecName $_pigeonChannelCodec =\n'
' $codecInstanceName;'),
cb.Code(
'final BinaryMessenger? ${_varNamePrefix}binaryMessenger = ${binaryMessengerParameter.name};',
),
const cb.Code('() async {'),
cb.Code(messageCallSink.toString()),
const cb.Code('}();'),
]);
},
);
},
);
}
}
/// The detached constructor present for every ProxyApi.
///
/// This constructor doesn't include a host method call to create a new native
/// class instance. It is mainly used when the native side wants to create a
/// Dart instance or when the `InstanceManager` wants to create a copy for
/// automatic garbage collection.
cb.Constructor _proxyApiDetachedConstructor({
required String apiName,
required AstProxyApi? superClassApi,
required Iterable<ApiField> unattachedFields,
required Iterable<Method> flutterMethodsFromSuperClasses,
required Iterable<Method> flutterMethodsFromInterfaces,
required Iterable<Method> declaredFlutterMethods,
}) {
final cb.Parameter binaryMessengerParameter = cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = '${classMemberNamePrefix}binaryMessenger'
..named = true
..toSuper = true,
);
final cb.Parameter instanceManagerParameter = cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = _instanceManagerVarName
..named = true
..toSuper = true,
);
return cb.Constructor(
(cb.ConstructorBuilder builder) => builder
..name = '${classMemberNamePrefix}detached'
..docs.addAll(<String>[
'/// Constructs [$apiName] without creating the associated native object.',
'///',
'/// This should only be used by subclasses created by this library or to',
'/// create copies for an [$instanceManagerClassName].',
])
..annotations.add(cb.refer('protected'))
..optionalParameters.addAll(<cb.Parameter>[
binaryMessengerParameter,
instanceManagerParameter,
for (final ApiField field in unattachedFields)
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = field.name
..named = true
..toThis = true
..required = !field.type.isNullable,
),
for (final Method method in flutterMethodsFromSuperClasses)
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = method.name
..named = true
..toSuper = true
..required = method.isRequired,
),
for (final Method method in flutterMethodsFromInterfaces
.followedBy(declaredFlutterMethods))
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = method.name
..named = true
..toThis = true
..required = method.isRequired,
),
])
..initializers.addAll(<cb.Code>[
if (superClassApi != null)
const cb.Code('super.${classMemberNamePrefix}detached()'),
]),
);
}
/// A private Field of the base codec.
cb.Field _proxyApiCodecInstanceField({
required String codecInstanceName,
required String codecName,
}) {
return cb.Field(
(cb.FieldBuilder builder) => builder
..name = codecInstanceName
..type = cb.refer(codecName)
..late = true
..modifier = cb.FieldModifier.final$
..assignment = cb.Code('$codecName($_instanceManagerVarName)'),
);
}
/// Converts unattached fields from the pigeon AST to `code_builder`
/// Fields.
Iterable<cb.Field> _proxyApiUnattachedFields(
Iterable<ApiField> fields,
) sync* {
for (final ApiField field in fields) {
yield cb.Field(
(cb.FieldBuilder builder) => builder
..name = field.name
..type = cb.refer(_addGenericTypesNullable(field.type))
..modifier = cb.FieldModifier.final$
..docs.addAll(asDocumentationComments(
field.documentationComments,
_docCommentSpec,
)),
);
}
}
/// Converts Flutter methods from the pigeon AST to `code_builder` Fields.
///
/// Flutter methods of a ProxyApi are set as an anonymous function of a class
/// instance, so this converts methods to a `Function` type field instance.
Iterable<cb.Field> _proxyApiFlutterMethodFields(
Iterable<Method> methods, {
required String apiName,
}) sync* {
for (final Method method in methods) {
yield cb.Field(
(cb.FieldBuilder builder) => builder
..name = method.name
..modifier = cb.FieldModifier.final$
..docs.addAll(asDocumentationComments(
<String>[
...method.documentationComments,
...<String>[
if (method.documentationComments.isEmpty) 'Callback method.',
'',
'For the associated Native object to be automatically garbage collected,',
"it is required that the implementation of this `Function` doesn't have a",
'strong reference to the encapsulating class instance. When this `Function`',
'references a non-local variable, it is strongly recommended to access it',
'with a `WeakReference`:',
'',
'```dart',
'final WeakReference weakMyVariable = WeakReference(myVariable);',
'final $apiName instance = $apiName(',
' ${method.name}: ($apiName ${classMemberNamePrefix}instance, ...) {',
' print(weakMyVariable?.target);',
' },',
');',
'```',
'',
'Alternatively, [$instanceManagerClassName.removeWeakReference] can be used to',
'release the associated Native object manually.',
],
],
_docCommentSpec,
))
..type = cb.FunctionType(
(cb.FunctionTypeBuilder builder) => builder
..returnType = _refer(
method.returnType,
asFuture: method.isAsynchronous,
)
..isNullable = !method.isRequired
..requiredParameters.addAll(<cb.Reference>[
cb.refer('$apiName ${classMemberNamePrefix}instance'),
...indexMap(
method.parameters,
(int index, NamedType parameter) {
return cb.refer(
'${_addGenericTypesNullable(parameter.type)} ${_getParameterName(index, parameter)}',
);
},
),
]),
),
);
}
}
/// Converts the Flutter methods from the pigeon AST to `code_builder` Fields.
///
/// Flutter methods of a ProxyApi are set as an anonymous function of a class
/// instance, so this converts methods to a `Function` type field instance.
///
/// This is similar to [_proxyApiFlutterMethodFields] except all the methods are
/// inherited from apis that are being implemented (following the `implements`
/// keyword).
Iterable<cb.Field> _proxyApiInterfaceApiFields(
Iterable<AstProxyApi> apisOfInterfaces,
) sync* {
for (final AstProxyApi proxyApi in apisOfInterfaces) {
for (final Method method in proxyApi.methods) {
yield cb.Field(
(cb.FieldBuilder builder) => builder
..name = method.name
..modifier = cb.FieldModifier.final$
..annotations.add(cb.refer('override'))
..docs.addAll(asDocumentationComments(
method.documentationComments,
_docCommentSpec,
))
..type = cb.FunctionType(
(cb.FunctionTypeBuilder builder) => builder
..returnType = _refer(
method.returnType,
asFuture: method.isAsynchronous,
)
..isNullable = !method.isRequired
..requiredParameters.addAll(<cb.Reference>[
cb.refer(
'${proxyApi.name} ${classMemberNamePrefix}instance',
),
...indexMap(
method.parameters,
(int index, NamedType parameter) {
return cb.refer(
'${_addGenericTypesNullable(parameter.type)} ${_getParameterName(index, parameter)}',
);
},
),
]),
),
);
}
}
}
/// Converts attached Fields from the pigeon AST to `code_builder` Field.
///
/// Attached fields are set lazily by calling a private method that returns
/// it.
///
/// Example Output:
///
/// ```dart
/// final MyOtherProxyApiClass value = _pigeon_value();
/// ```
Iterable<cb.Field> _proxyApiAttachedFields(Iterable<ApiField> fields) sync* {
for (final ApiField field in fields) {
yield cb.Field(
(cb.FieldBuilder builder) => builder
..name = field.name
..type = cb.refer(_addGenericTypesNullable(field.type))
..modifier = cb.FieldModifier.final$
..static = field.isStatic
..late = !field.isStatic
..docs.addAll(asDocumentationComments(
field.documentationComments,
_docCommentSpec,
))
..assignment = cb.Code('$_varNamePrefix${field.name}()'),
);
}
}
/// Creates the static `setUpMessageHandlers` method for a ProxyApi.
///
/// This method handles setting the message handler for every un-inherited
/// Flutter method.
///
/// This also adds a handler to receive a call from the platform to
/// instantiate a new Dart instance if [hasCallbackConstructor] is set to
/// true.
cb.Method _proxyApiSetUpMessageHandlerMethod({
required Iterable<Method> flutterMethods,
required String apiName,
required String dartPackageName,
required String codecName,
required Iterable<ApiField> unattachedFields,
required bool hasCallbackConstructor,
}) {
final bool hasAnyMessageHandlers =
hasCallbackConstructor || flutterMethods.isNotEmpty;
return cb.Method.returnsVoid(
(cb.MethodBuilder builder) => builder
..name = '${classMemberNamePrefix}setUpMessageHandlers'
..returns = cb.refer('void')
..static = true
..optionalParameters.addAll(<cb.Parameter>[
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = '${classMemberNamePrefix}clearHandlers'
..type = cb.refer('bool')
..named = true
..defaultTo = const cb.Code('false'),
),
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = '${classMemberNamePrefix}binaryMessenger'
..named = true
..type = cb.refer('BinaryMessenger?'),
),
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = _instanceManagerVarName
..named = true
..type = cb.refer('$instanceManagerClassName?'),
),
if (hasCallbackConstructor)
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = '${classMemberNamePrefix}newInstance'
..named = true
..type = cb.FunctionType(
(cb.FunctionTypeBuilder builder) => builder
..returnType = cb.refer(apiName)
..isNullable = true
..requiredParameters.addAll(
indexMap(
unattachedFields,
(int index, ApiField field) {
return cb.refer(
'${_addGenericTypesNullable(field.type)} ${_getParameterName(index, field)}',
);
},
),
),
),
),
for (final Method method in flutterMethods)
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = method.name
..type = cb.FunctionType(
(cb.FunctionTypeBuilder builder) => builder
..returnType = _refer(
method.returnType,
asFuture: method.isAsynchronous,
)
..isNullable = true
..requiredParameters.addAll(<cb.Reference>[
cb.refer('$apiName ${classMemberNamePrefix}instance'),
...indexMap(
method.parameters,
(int index, NamedType parameter) {
return cb.refer(
'${_addGenericTypesNullable(parameter.type)} ${_getParameterName(index, parameter)}',
);
},
),
]),
),
),
])
..body = cb.Block.of(<cb.Code>[
if (hasAnyMessageHandlers) ...<cb.Code>[
cb.Code(
'final $codecName $_pigeonChannelCodec = $codecName($_instanceManagerVarName ?? $instanceManagerClassName.instance);',
),
const cb.Code(
'final BinaryMessenger? binaryMessenger = ${classMemberNamePrefix}binaryMessenger;',
)
],
if (hasCallbackConstructor)
...cb.Block((cb.BlockBuilder builder) {
final StringBuffer messageHandlerSink = StringBuffer();
const String methodName = '${classMemberNamePrefix}newInstance';
_writeFlutterMethodMessageHandler(
Indent(messageHandlerSink),
name: methodName,
parameters: <Parameter>[
Parameter(
name: '${classMemberNamePrefix}instanceIdentifier',
type: const TypeDeclaration(
baseName: 'int',
isNullable: false,
),
),
...unattachedFields.map(
(ApiField field) {
return Parameter(name: field.name, type: field.type);
},
),
],
returnType: const TypeDeclaration.voidDeclaration(),
channelName: makeChannelNameWithStrings(
apiName: apiName,
methodName: methodName,
dartPackageName: dartPackageName,
),
isMockHandler: false,
isAsynchronous: false,
nullHandlerExpression: '${classMemberNamePrefix}clearHandlers',
onCreateApiCall: (
String methodName,
Iterable<Parameter> parameters,
Iterable<String> safeArgumentNames,
) {
final String argsAsNamedParams = map2(
parameters,
safeArgumentNames,
(Parameter parameter, String safeArgName) {
return '${parameter.name}: $safeArgName,\n';
},
).skip(1).join();
return '($_instanceManagerVarName ?? $instanceManagerClassName.instance)\n'
' .addHostCreatedInstance(\n'
' $methodName?.call(${safeArgumentNames.skip(1).join(',')}) ??\n'
' $apiName.${classMemberNamePrefix}detached('
' ${classMemberNamePrefix}binaryMessenger: ${classMemberNamePrefix}binaryMessenger,\n'
' $_instanceManagerVarName: $_instanceManagerVarName,\n'
' $argsAsNamedParams\n'
' ),\n'
' ${safeArgumentNames.first},\n'
')';
},
);
builder.statements.add(cb.Code(messageHandlerSink.toString()));
}).statements,
for (final Method method in flutterMethods)
...cb.Block((cb.BlockBuilder builder) {
final StringBuffer messageHandlerSink = StringBuffer();
_writeFlutterMethodMessageHandler(
Indent(messageHandlerSink),
name: method.name,
parameters: <Parameter>[
Parameter(
name: '${classMemberNamePrefix}instance',
type: TypeDeclaration(
baseName: apiName,
isNullable: false,
),
),
...method.parameters,
],
returnType: TypeDeclaration(
baseName: method.returnType.baseName,
isNullable:
!method.isRequired || method.returnType.isNullable,
typeArguments: method.returnType.typeArguments,
associatedEnum: method.returnType.associatedEnum,
associatedClass: method.returnType.associatedClass,
associatedProxyApi: method.returnType.associatedProxyApi,
),
channelName: makeChannelNameWithStrings(
apiName: apiName,
methodName: method.name,
dartPackageName: dartPackageName,
),
isMockHandler: false,
isAsynchronous: method.isAsynchronous,
nullHandlerExpression: '${classMemberNamePrefix}clearHandlers',
onCreateApiCall: (
String methodName,
Iterable<Parameter> parameters,
Iterable<String> safeArgumentNames,
) {
final String nullability = method.isRequired ? '' : '?';
return '($methodName ?? ${safeArgumentNames.first}.$methodName)$nullability.call(${safeArgumentNames.join(',')})';
},
);
builder.statements.add(cb.Code(messageHandlerSink.toString()));
}).statements,
]),
);
}
/// Converts attached fields from the pigeon AST to `code_builder` Methods.
///
/// These private methods are used to lazily instantiate attached fields. The
/// instance is created and returned synchronously while the native instance
/// is created asynchronously. This is similar to how constructors work.
Iterable<cb.Method> _proxyApiAttachedFieldMethods(
Iterable<ApiField> fields, {
required String apiName,
required String dartPackageName,
required String codecInstanceName,
required String codecName,
}) sync* {
for (final ApiField field in fields) {
yield cb.Method(
(cb.MethodBuilder builder) {
final String type = _addGenericTypesNullable(field.type);
const String instanceName = '${_varNamePrefix}instance';
const String identifierInstanceName =
'${_varNamePrefix}instanceIdentifier';
builder
..name = '$_varNamePrefix${field.name}'
..static = field.isStatic
..returns = cb.refer(type)
..body = cb.Block(
(cb.BlockBuilder builder) {
final StringBuffer messageCallSink = StringBuffer();
_writeHostMethodMessageCall(
Indent(messageCallSink),
channelName: makeChannelNameWithStrings(
apiName: apiName,
methodName: field.name,
dartPackageName: dartPackageName,
),
parameters: <Parameter>[
if (!field.isStatic)
Parameter(
name: 'this',
type: TypeDeclaration(
baseName: apiName,
isNullable: false,
),
),
Parameter(
name: identifierInstanceName,
type: const TypeDeclaration(
baseName: 'int',
isNullable: false,
),
),
],
returnType: const TypeDeclaration.voidDeclaration(),
);
builder.statements.addAll(<cb.Code>[
if (!field.isStatic) ...<cb.Code>[
cb.Code(
'final $type $instanceName = $type.${classMemberNamePrefix}detached(\n'
' pigeon_binaryMessenger: pigeon_binaryMessenger,\n'
' pigeon_instanceManager: pigeon_instanceManager,\n'
');',
),
cb.Code('final $codecName $_pigeonChannelCodec =\n'
' $codecInstanceName;'),
const cb.Code(
'final BinaryMessenger? ${_varNamePrefix}binaryMessenger = ${classMemberNamePrefix}binaryMessenger;',
),
const cb.Code(
'final int $identifierInstanceName = $_instanceManagerVarName.addDartCreatedInstance($instanceName);',
),
] else ...<cb.Code>[
cb.Code(
'final $type $instanceName = $type.${classMemberNamePrefix}detached();',
),
cb.Code(
'final $codecName $_pigeonChannelCodec = $codecName($instanceManagerClassName.instance);',
),
const cb.Code(
'final BinaryMessenger ${_varNamePrefix}binaryMessenger = ServicesBinding.instance.defaultBinaryMessenger;',
),
const cb.Code(
'final int $identifierInstanceName = $instanceManagerClassName.instance.addDartCreatedInstance($instanceName);',
),
],
const cb.Code('() async {'),
cb.Code(messageCallSink.toString()),
const cb.Code('}();'),
const cb.Code('return $instanceName;'),
]);
},
);
},
);
}
}
/// Converts host methods from pigeon AST to `code_builder` Methods.
///
/// This creates methods like a HostApi except that it includes the calling
/// instance if the method is not static.
Iterable<cb.Method> _proxyApiHostMethods(
Iterable<Method> methods, {
required String apiName,
required String dartPackageName,
required String codecInstanceName,
required String codecName,
}) sync* {
for (final Method method in methods) {
assert(method.location == ApiLocation.host);
yield cb.Method(
(cb.MethodBuilder builder) => builder
..name = method.name
..static = method.isStatic
..modifier = cb.MethodModifier.async
..docs.addAll(asDocumentationComments(
method.documentationComments,
_docCommentSpec,
))
..returns = _refer(method.returnType, asFuture: true)
..requiredParameters.addAll(
indexMap(
method.parameters,
(int index, NamedType parameter) => cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = _getParameterName(index, parameter)
..type = cb.refer(
_addGenericTypesNullable(parameter.type),
),
),
),
)
..optionalParameters.addAll(<cb.Parameter>[
if (method.isStatic) ...<cb.Parameter>[
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = '${classMemberNamePrefix}binaryMessenger'
..type = cb.refer('BinaryMessenger?')
..named = true,
),
cb.Parameter(
(cb.ParameterBuilder builder) => builder
..name = _instanceManagerVarName
..type = cb.refer('$instanceManagerClassName?'),
),
],
])
..body = cb.Block(
(cb.BlockBuilder builder) {
final StringBuffer messageCallSink = StringBuffer();
_writeHostMethodMessageCall(
Indent(messageCallSink),
channelName: makeChannelNameWithStrings(
apiName: apiName,
methodName: method.name,
dartPackageName: dartPackageName,
),
parameters: <Parameter>[
if (!method.isStatic)
Parameter(
name: 'this',
type: TypeDeclaration(
baseName: apiName,
isNullable: false,
),
),
...method.parameters,
],
returnType: method.returnType,
);
builder.statements.addAll(<cb.Code>[
if (!method.isStatic)
cb.Code('final $codecName $_pigeonChannelCodec =\n'
' $codecInstanceName;')
else
cb.Code(
'final $codecName $_pigeonChannelCodec = $codecName($_instanceManagerVarName ?? $instanceManagerClassName.instance);',
),
const cb.Code(
'final BinaryMessenger? ${_varNamePrefix}binaryMessenger = ${classMemberNamePrefix}binaryMessenger;',
),
cb.Code(messageCallSink.toString()),
]);
},
),
);
}
}
/// Creates the copy method for a ProxyApi.
///
/// This method returns a copy of the instance with all the Flutter methods
/// and unattached fields passed to the new instance. This method is inherited
/// from the base ProxyApi class.
cb.Method _proxyApiCopyMethod({
required String apiName,
required Iterable<ApiField> unattachedFields,
required Iterable<Method> declaredAndInheritedFlutterMethods,
}) {
return cb.Method(
(cb.MethodBuilder builder) => builder
..name = '${classMemberNamePrefix}copy'
..returns = cb.refer(apiName)
..annotations.add(cb.refer('override'))
..body = cb.Block.of(<cb.Code>[
cb
.refer('$apiName.${classMemberNamePrefix}detached')
.call(
<cb.Expression>[],
<String, cb.Expression>{
'${classMemberNamePrefix}binaryMessenger':
cb.refer('${classMemberNamePrefix}binaryMessenger'),
_instanceManagerVarName: cb.refer(_instanceManagerVarName),
for (final ApiField field in unattachedFields)
field.name: cb.refer(field.name),
for (final Method method
in declaredAndInheritedFlutterMethods)
method.name: cb.refer(method.name),
},
)
.returned
.statement,
]),
);
}
}
cb.Reference _refer(TypeDeclaration type, {bool asFuture = false}) {
final String symbol = _addGenericTypesNullable(type);
return cb.refer(asFuture ? 'Future<$symbol>' : symbol);
}
String _escapeForDartSingleQuotedString(String raw) {
return raw
.replaceAll(r'\', r'\\')
.replaceAll(r'$', r'\$')
.replaceAll(r"'", r"\'");
}
/// Calculates the name of the codec class that will be generated for [api].
String _getCodecName(Api api) => '_${api.name}Codec';
/// Writes the codec that will be used by [api].
/// Example:
///
/// class FooCodec extends StandardMessageCodec {...}
void _writeCodec(Indent indent, String codecName, Api api, Root root) {
assert(getCodecClasses(api, root).isNotEmpty);
final Iterable<EnumeratedClass> codecClasses = getCodecClasses(api, root);
indent.newln();
indent.write('class $codecName extends $_standardMessageCodec');
indent.addScoped(' {', '}', () {
indent.writeln('const $codecName();');
indent.writeln('@override');
indent.write('void writeValue(WriteBuffer buffer, Object? value) ');
indent.addScoped('{', '}', () {
enumerate(codecClasses, (int index, final EnumeratedClass customClass) {
final String ifValue = 'if (value is ${customClass.name}) ';
if (index == 0) {
indent.write('');
}
indent.add(ifValue);
indent.addScoped('{', '} else ', () {
indent.writeln('buffer.putUint8(${customClass.enumeration});');
indent.writeln('writeValue(buffer, value.encode());');
}, addTrailingNewline: false);
});
indent.addScoped('{', '}', () {
indent.writeln('super.writeValue(buffer, value);');
});
});
indent.newln();
indent.writeln('@override');
indent.write('Object? readValueOfType(int type, ReadBuffer buffer) ');
indent.addScoped('{', '}', () {
indent.write('switch (type) ');
indent.addScoped('{', '}', () {
for (final EnumeratedClass customClass in codecClasses) {
indent.writeln('case ${customClass.enumeration}: ');
indent.nest(1, () {
indent.writeln(
'return ${customClass.name}.decode(readValue(buffer)!);');
});
}
indent.writeln('default:');
indent.nest(1, () {
indent.writeln('return super.readValueOfType(type, buffer);');
});
});
});
});
}
/// Creates a Dart type where all type arguments are [Objects].
String _makeGenericTypeArguments(TypeDeclaration type) {
return type.typeArguments.isNotEmpty
? '${type.baseName}<${type.typeArguments.map<String>((TypeDeclaration e) => 'Object?').join(', ')}>'
: _addGenericTypes(type);
}
/// Creates a `.cast<>` call for an type. Returns an empty string if the
/// type has no type arguments.
String _makeGenericCastCall(TypeDeclaration type) {
return type.typeArguments.isNotEmpty
? '.cast<${_flattenTypeArguments(type.typeArguments)}>()'
: '';
}
/// Returns an argument name that can be used in a context where it is possible to collide.
String _getSafeArgumentName(int count, NamedType field) =>
field.name.isEmpty ? 'arg$count' : 'arg_${field.name}';
/// Generates a parameter name if one isn't defined.
String _getParameterName(int count, NamedType field) =>
field.name.isEmpty ? 'arg$count' : field.name;
/// Generates the parameters code for [func]
/// Example: (func, _getParameterName) -> 'String? foo, int bar'
String _getMethodParameterSignature(Iterable<Parameter> parameters) {
String signature = '';
if (parameters.isEmpty) {
return signature;
}
final List<Parameter> requiredPositionalParams = parameters
.where((Parameter p) => p.isPositional && !p.isOptional)
.toList();
final List<Parameter> optionalPositionalParams = parameters
.where((Parameter p) => p.isPositional && p.isOptional)
.toList();
final List<Parameter> namedParams =
parameters.where((Parameter p) => !p.isPositional).toList();
String getParameterString(Parameter p) {
final String required = p.isRequired && !p.isPositional ? 'required ' : '';
final String type = _addGenericTypesNullable(p.type);
final String defaultValue =
p.defaultValue == null ? '' : ' = ${p.defaultValue}';
return '$required$type ${p.name}$defaultValue';
}
final String baseParameterString = requiredPositionalParams
.map((Parameter p) => getParameterString(p))
.join(', ');
final String optionalParameterString = optionalPositionalParams
.map((Parameter p) => getParameterString(p))
.join(', ');
final String namedParameterString =
namedParams.map((Parameter p) => getParameterString(p)).join(', ');
// Parameter lists can end with either named or optional positional parameters, but not both.
if (requiredPositionalParams.isNotEmpty) {
signature = baseParameterString;
}
final String trailingComma =
optionalPositionalParams.isNotEmpty || namedParams.isNotEmpty ? ',' : '';
final String baseParams =
signature.isNotEmpty ? '$signature$trailingComma ' : '';
if (optionalPositionalParams.isNotEmpty) {
final String trailingComma =
requiredPositionalParams.length + optionalPositionalParams.length > 2
? ','
: '';
return '$baseParams[$optionalParameterString$trailingComma]';
}
if (namedParams.isNotEmpty) {
final String trailingComma =
requiredPositionalParams.length + namedParams.length > 2 ? ',' : '';
return '$baseParams{$namedParameterString$trailingComma}';
}
return signature;
}
/// Converts a [List] of [TypeDeclaration]s to a comma separated [String] to be
/// used in Dart code.
String _flattenTypeArguments(List<TypeDeclaration> args) {
return args
.map<String>((TypeDeclaration arg) => arg.typeArguments.isEmpty
? '${arg.baseName}?'
: '${arg.baseName}<${_flattenTypeArguments(arg.typeArguments)}>?')
.join(', ');
}
/// Creates the type declaration for use in Dart code from a [NamedType] making sure
/// that type arguments are used for primitive generic types.
String _addGenericTypes(TypeDeclaration type) {
final List<TypeDeclaration> typeArguments = type.typeArguments;
switch (type.baseName) {
case 'List':
return (typeArguments.isEmpty)
? 'List<Object?>'
: 'List<${_flattenTypeArguments(typeArguments)}>';
case 'Map':
return (typeArguments.isEmpty)
? 'Map<Object?, Object?>'
: 'Map<${_flattenTypeArguments(typeArguments)}>';
default:
return type.baseName;
}
}
String _addGenericTypesNullable(TypeDeclaration type) {
final String genericType = _addGenericTypes(type);
return type.isNullable ? '$genericType?' : genericType;
}
/// Converts [inputPath] to a posix absolute path.
String _posixify(String inputPath) {
final path.Context context = path.Context(style: path.Style.posix);
return context.fromUri(path.toUri(path.absolute(inputPath)));
}
| packages/packages/pigeon/lib/dart_generator.dart/0 | {
"file_path": "packages/packages/pigeon/lib/dart_generator.dart",
"repo_id": "packages",
"token_count": 33121
} | 1,105 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:pigeon/pigeon.dart';
class FlutterSearchRequest {
String? query;
}
class FlutterSearchReply {
String? result;
String? error;
}
class FlutterSearchRequests {
// ignore: always_specify_types, strict_raw_type
List? requests;
}
class FlutterSearchReplies {
// ignore: always_specify_types, strict_raw_type
List? replies;
}
@HostApi()
abstract class Api {
FlutterSearchReply search(FlutterSearchRequest request);
FlutterSearchReplies doSearches(FlutterSearchRequests request);
FlutterSearchRequests echo(FlutterSearchRequests requests);
int anInt(int value);
}
| packages/packages/pigeon/pigeons/flutter_unittests.dart/0 | {
"file_path": "packages/packages/pigeon/pigeons/flutter_unittests.dart",
"repo_id": "packages",
"token_count": 238
} | 1,106 |
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.alternate_language_test_plugin">
</manifest>
| packages/packages/pigeon/platform_tests/alternate_language_test_plugin/android/src/main/AndroidManifest.xml/0 | {
"file_path": "packages/packages/pigeon/platform_tests/alternate_language_test_plugin/android/src/main/AndroidManifest.xml",
"repo_id": "packages",
"token_count": 48
} | 1,107 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import "HandlerBinaryMessenger.h"
@interface HandlerBinaryMessenger ()
@property(nonatomic, strong) NSObject<FlutterMessageCodec> *codec;
@property(nonatomic, copy) HandlerBinaryMessengerHandler handler;
@end
@implementation HandlerBinaryMessenger {
int _count;
}
- (instancetype)initWithCodec:(NSObject<FlutterMessageCodec> *)codec
handler:(HandlerBinaryMessengerHandler)handler {
self = [super init];
if (self) {
_codec = codec;
_handler = [handler copy];
}
return self;
}
- (void)cleanUpConnection:(FlutterBinaryMessengerConnection)connection {
}
- (void)sendOnChannel:(nonnull NSString *)channel message:(NSData *_Nullable)message {
}
- (void)sendOnChannel:(nonnull NSString *)channel
message:(NSData *_Nullable)message
binaryReply:(FlutterBinaryReply _Nullable)callback {
NSArray *args = [self.codec decode:message];
id result = self.handler(args);
callback([self.codec encode:result]);
}
- (FlutterBinaryMessengerConnection)setMessageHandlerOnChannel:(nonnull NSString *)channel
binaryMessageHandler:
(FlutterBinaryMessageHandler _Nullable)handler {
return ++_count;
}
@end
| packages/packages/pigeon/platform_tests/alternate_language_test_plugin/example/ios/RunnerTests/HandlerBinaryMessenger.m/0 | {
"file_path": "packages/packages/pigeon/platform_tests/alternate_language_test_plugin/example/ios/RunnerTests/HandlerBinaryMessenger.m",
"repo_id": "packages",
"token_count": 528
} | 1,108 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/pigeon/platform_tests/alternate_language_test_plugin/example/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "packages/packages/pigeon/platform_tests/alternate_language_test_plugin/example/macos/Runner/Configs/Release.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 1,109 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// There is intentionally no code here; tests use generated Pigeon APIs
// directly, as wrapping them in a plugin would just add maintenance burden
// when changing tests.
| packages/packages/pigeon/platform_tests/alternate_language_test_plugin/lib/alternate_language_test_plugin.dart/0 | {
"file_path": "packages/packages/pigeon/platform_tests/alternate_language_test_plugin/lib/alternate_language_test_plugin.dart",
"repo_id": "packages",
"token_count": 79
} | 1,110 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
//
// Autogenerated from Pigeon, do not edit directly.
// See also: https://pub.dev/packages/pigeon
// ignore_for_file: public_member_api_docs, non_constant_identifier_names, avoid_as, unused_import, unnecessary_parenthesis, prefer_null_aware_operators, omit_local_variable_types, unused_shown_name, unnecessary_import, no_leading_underscores_for_local_identifiers
import 'dart:async';
import 'dart:typed_data' show Float64List, Int32List, Int64List, Uint8List;
import 'package:flutter/foundation.dart' show ReadBuffer, WriteBuffer;
import 'package:flutter/services.dart';
PlatformException _createConnectionError(String channelName) {
return PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel: "$channelName".',
);
}
List<Object?> wrapResponse(
{Object? result, PlatformException? error, bool empty = false}) {
if (empty) {
return <Object?>[];
}
if (error == null) {
return <Object?>[result];
}
return <Object?>[error.code, error.message, error.details];
}
enum AnEnum {
one,
two,
three,
fortyTwo,
fourHundredTwentyTwo,
}
/// A class containing all supported types.
class AllTypes {
AllTypes({
this.aBool = false,
this.anInt = 0,
this.anInt64 = 0,
this.aDouble = 0,
required this.aByteArray,
required this.a4ByteArray,
required this.a8ByteArray,
required this.aFloatArray,
this.aList = const <Object?>[],
this.aMap = const <String?, Object?>{},
this.anEnum = AnEnum.one,
this.aString = '',
this.anObject = 0,
});
bool aBool;
int anInt;
int anInt64;
double aDouble;
Uint8List aByteArray;
Int32List a4ByteArray;
Int64List a8ByteArray;
Float64List aFloatArray;
List<Object?> aList;
Map<Object?, Object?> aMap;
AnEnum anEnum;
String aString;
Object anObject;
Object encode() {
return <Object?>[
aBool,
anInt,
anInt64,
aDouble,
aByteArray,
a4ByteArray,
a8ByteArray,
aFloatArray,
aList,
aMap,
anEnum.index,
aString,
anObject,
];
}
static AllTypes decode(Object result) {
result as List<Object?>;
return AllTypes(
aBool: result[0]! as bool,
anInt: result[1]! as int,
anInt64: result[2]! as int,
aDouble: result[3]! as double,
aByteArray: result[4]! as Uint8List,
a4ByteArray: result[5]! as Int32List,
a8ByteArray: result[6]! as Int64List,
aFloatArray: result[7]! as Float64List,
aList: result[8]! as List<Object?>,
aMap: result[9]! as Map<Object?, Object?>,
anEnum: AnEnum.values[result[10]! as int],
aString: result[11]! as String,
anObject: result[12]!,
);
}
}
/// A class containing all supported nullable types.
class AllNullableTypes {
AllNullableTypes({
this.aNullableBool,
this.aNullableInt,
this.aNullableInt64,
this.aNullableDouble,
this.aNullableByteArray,
this.aNullable4ByteArray,
this.aNullable8ByteArray,
this.aNullableFloatArray,
this.aNullableList,
this.aNullableMap,
this.nullableNestedList,
this.nullableMapWithAnnotations,
this.nullableMapWithObject,
this.aNullableEnum,
this.aNullableString,
this.aNullableObject,
});
bool? aNullableBool;
int? aNullableInt;
int? aNullableInt64;
double? aNullableDouble;
Uint8List? aNullableByteArray;
Int32List? aNullable4ByteArray;
Int64List? aNullable8ByteArray;
Float64List? aNullableFloatArray;
List<Object?>? aNullableList;
Map<Object?, Object?>? aNullableMap;
List<List<bool?>?>? nullableNestedList;
Map<String?, String?>? nullableMapWithAnnotations;
Map<String?, Object?>? nullableMapWithObject;
AnEnum? aNullableEnum;
String? aNullableString;
Object? aNullableObject;
Object encode() {
return <Object?>[
aNullableBool,
aNullableInt,
aNullableInt64,
aNullableDouble,
aNullableByteArray,
aNullable4ByteArray,
aNullable8ByteArray,
aNullableFloatArray,
aNullableList,
aNullableMap,
nullableNestedList,
nullableMapWithAnnotations,
nullableMapWithObject,
aNullableEnum?.index,
aNullableString,
aNullableObject,
];
}
static AllNullableTypes decode(Object result) {
result as List<Object?>;
return AllNullableTypes(
aNullableBool: result[0] as bool?,
aNullableInt: result[1] as int?,
aNullableInt64: result[2] as int?,
aNullableDouble: result[3] as double?,
aNullableByteArray: result[4] as Uint8List?,
aNullable4ByteArray: result[5] as Int32List?,
aNullable8ByteArray: result[6] as Int64List?,
aNullableFloatArray: result[7] as Float64List?,
aNullableList: result[8] as List<Object?>?,
aNullableMap: result[9] as Map<Object?, Object?>?,
nullableNestedList: (result[10] as List<Object?>?)?.cast<List<bool?>?>(),
nullableMapWithAnnotations:
(result[11] as Map<Object?, Object?>?)?.cast<String?, String?>(),
nullableMapWithObject:
(result[12] as Map<Object?, Object?>?)?.cast<String?, Object?>(),
aNullableEnum:
result[13] != null ? AnEnum.values[result[13]! as int] : null,
aNullableString: result[14] as String?,
aNullableObject: result[15],
);
}
}
/// A class for testing nested class handling.
///
/// This is needed to test nested nullable and non-nullable classes,
/// `AllNullableTypes` is non-nullable here as it is easier to instantiate
/// than `AllTypes` when testing doesn't require both (ie. testing null classes).
class AllClassesWrapper {
AllClassesWrapper({
required this.allNullableTypes,
this.allTypes,
});
AllNullableTypes allNullableTypes;
AllTypes? allTypes;
Object encode() {
return <Object?>[
allNullableTypes.encode(),
allTypes?.encode(),
];
}
static AllClassesWrapper decode(Object result) {
result as List<Object?>;
return AllClassesWrapper(
allNullableTypes: AllNullableTypes.decode(result[0]! as List<Object?>),
allTypes: result[1] != null
? AllTypes.decode(result[1]! as List<Object?>)
: null,
);
}
}
/// A data class containing a List, used in unit tests.
class TestMessage {
TestMessage({
this.testList,
});
List<Object?>? testList;
Object encode() {
return <Object?>[
testList,
];
}
static TestMessage decode(Object result) {
result as List<Object?>;
return TestMessage(
testList: result[0] as List<Object?>?,
);
}
}
class _HostIntegrationCoreApiCodec extends StandardMessageCodec {
const _HostIntegrationCoreApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is AllClassesWrapper) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is AllNullableTypes) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is AllTypes) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else if (value is TestMessage) {
buffer.putUint8(131);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return AllClassesWrapper.decode(readValue(buffer)!);
case 129:
return AllNullableTypes.decode(readValue(buffer)!);
case 130:
return AllTypes.decode(readValue(buffer)!);
case 131:
return TestMessage.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// The core interface that each host language plugin must implement in
/// platform_test integration tests.
class HostIntegrationCoreApi {
/// Constructor for [HostIntegrationCoreApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
HostIntegrationCoreApi({BinaryMessenger? binaryMessenger})
: __pigeon_binaryMessenger = binaryMessenger;
final BinaryMessenger? __pigeon_binaryMessenger;
static const MessageCodec<Object?> pigeonChannelCodec =
_HostIntegrationCoreApiCodec();
/// A no-op function taking no arguments and returning no value, to sanity
/// test basic calling.
Future<void> noop() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.noop';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
/// Returns the passed object, to test serialization and deserialization.
Future<AllTypes> echoAllTypes(AllTypes everything) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAllTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[everything]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as AllTypes?)!;
}
}
/// Returns an error, to test error handling.
Future<Object?> throwError() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwError';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return __pigeon_replyList[0];
}
}
/// Returns an error from a void function, to test error handling.
Future<void> throwErrorFromVoid() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwErrorFromVoid';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
/// Returns a Flutter error, to test error handling.
Future<Object?> throwFlutterError() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwFlutterError';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return __pigeon_replyList[0];
}
}
/// Returns passed in int.
Future<int> echoInt(int anInt) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as int?)!;
}
}
/// Returns passed in double.
Future<double> echoDouble(double aDouble) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoDouble';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aDouble]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as double?)!;
}
}
/// Returns the passed in boolean.
Future<bool> echoBool(bool aBool) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoBool';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aBool]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as bool?)!;
}
}
/// Returns the passed in string.
Future<String> echoString(String aString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as String?)!;
}
}
/// Returns the passed in Uint8List.
Future<Uint8List> echoUint8List(Uint8List aUint8List) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoUint8List';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aUint8List]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as Uint8List?)!;
}
}
/// Returns the passed in generic Object.
Future<Object> echoObject(Object anObject) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoObject';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anObject]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return __pigeon_replyList[0]!;
}
}
/// Returns the passed list, to test serialization and deserialization.
Future<List<Object?>> echoList(List<Object?> aList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoList';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as List<Object?>?)!.cast<Object?>();
}
}
/// Returns the passed map, to test serialization and deserialization.
Future<Map<String?, Object?>> echoMap(Map<String?, Object?> aMap) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoMap';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aMap]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as Map<Object?, Object?>?)!
.cast<String?, Object?>();
}
}
/// Returns the passed map to test nested class serialization and deserialization.
Future<AllClassesWrapper> echoClassWrapper(AllClassesWrapper wrapper) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoClassWrapper';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[wrapper]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as AllClassesWrapper?)!;
}
}
/// Returns the passed enum to test serialization and deserialization.
Future<AnEnum> echoEnum(AnEnum anEnum) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoEnum';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anEnum.index]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return AnEnum.values[__pigeon_replyList[0]! as int];
}
}
/// Returns the default string.
Future<String> echoNamedDefaultString({String aString = 'default'}) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNamedDefaultString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as String?)!;
}
}
/// Returns passed in double.
Future<double> echoOptionalDefaultDouble([double aDouble = 3.14]) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoOptionalDefaultDouble';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aDouble]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as double?)!;
}
}
/// Returns passed in int.
Future<int> echoRequiredInt({required int anInt}) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoRequiredInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as int?)!;
}
}
/// Returns the passed object, to test serialization and deserialization.
Future<AllNullableTypes?> echoAllNullableTypes(
AllNullableTypes? everything) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAllNullableTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[everything]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as AllNullableTypes?);
}
}
/// Returns the inner `aString` value from the wrapped object, to test
/// sending of nested objects.
Future<String?> extractNestedNullableString(AllClassesWrapper wrapper) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.extractNestedNullableString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[wrapper]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as String?);
}
}
/// Returns the inner `aString` value from the wrapped object, to test
/// sending of nested objects.
Future<AllClassesWrapper> createNestedNullableString(
String? nullableString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.createNestedNullableString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[nullableString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as AllClassesWrapper?)!;
}
}
/// Returns passed in arguments of multiple types.
Future<AllNullableTypes> sendMultipleNullableTypes(
bool? aNullableBool, int? aNullableInt, String? aNullableString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.sendMultipleNullableTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[aNullableBool, aNullableInt, aNullableString])
as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as AllNullableTypes?)!;
}
}
/// Returns passed in int.
Future<int?> echoNullableInt(int? aNullableInt) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aNullableInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as int?);
}
}
/// Returns passed in double.
Future<double?> echoNullableDouble(double? aNullableDouble) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableDouble';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[aNullableDouble]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as double?);
}
}
/// Returns the passed in boolean.
Future<bool?> echoNullableBool(bool? aNullableBool) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableBool';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aNullableBool]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as bool?);
}
}
/// Returns the passed in string.
Future<String?> echoNullableString(String? aNullableString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[aNullableString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as String?);
}
}
/// Returns the passed in Uint8List.
Future<Uint8List?> echoNullableUint8List(
Uint8List? aNullableUint8List) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableUint8List';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[aNullableUint8List]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as Uint8List?);
}
}
/// Returns the passed in generic Object.
Future<Object?> echoNullableObject(Object? aNullableObject) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableObject';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[aNullableObject]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return __pigeon_replyList[0];
}
}
/// Returns the passed list, to test serialization and deserialization.
Future<List<Object?>?> echoNullableList(List<Object?>? aNullableList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableList';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aNullableList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as List<Object?>?)?.cast<Object?>();
}
}
/// Returns the passed map, to test serialization and deserialization.
Future<Map<String?, Object?>?> echoNullableMap(
Map<String?, Object?>? aNullableMap) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableMap';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aNullableMap]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as Map<Object?, Object?>?)
?.cast<String?, Object?>();
}
}
Future<AnEnum?> echoNullableEnum(AnEnum? anEnum) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableEnum';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anEnum?.index]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as int?) == null
? null
: AnEnum.values[__pigeon_replyList[0]! as int];
}
}
/// Returns passed in int.
Future<int?> echoOptionalNullableInt([int? aNullableInt]) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoOptionalNullableInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aNullableInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as int?);
}
}
/// Returns the passed in string.
Future<String?> echoNamedNullableString({String? aNullableString}) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNamedNullableString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[aNullableString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as String?);
}
}
/// A no-op function taking no arguments and returning no value, to sanity
/// test basic asynchronous calling.
Future<void> noopAsync() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.noopAsync';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
/// Returns passed in int asynchronously.
Future<int> echoAsyncInt(int anInt) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as int?)!;
}
}
/// Returns passed in double asynchronously.
Future<double> echoAsyncDouble(double aDouble) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncDouble';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aDouble]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as double?)!;
}
}
/// Returns the passed in boolean asynchronously.
Future<bool> echoAsyncBool(bool aBool) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncBool';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aBool]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as bool?)!;
}
}
/// Returns the passed string asynchronously.
Future<String> echoAsyncString(String aString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as String?)!;
}
}
/// Returns the passed in Uint8List asynchronously.
Future<Uint8List> echoAsyncUint8List(Uint8List aUint8List) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncUint8List';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aUint8List]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as Uint8List?)!;
}
}
/// Returns the passed in generic Object asynchronously.
Future<Object> echoAsyncObject(Object anObject) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncObject';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anObject]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return __pigeon_replyList[0]!;
}
}
/// Returns the passed list, to test asynchronous serialization and deserialization.
Future<List<Object?>> echoAsyncList(List<Object?> aList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncList';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as List<Object?>?)!.cast<Object?>();
}
}
/// Returns the passed map, to test asynchronous serialization and deserialization.
Future<Map<String?, Object?>> echoAsyncMap(Map<String?, Object?> aMap) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncMap';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aMap]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as Map<Object?, Object?>?)!
.cast<String?, Object?>();
}
}
/// Returns the passed enum, to test asynchronous serialization and deserialization.
Future<AnEnum> echoAsyncEnum(AnEnum anEnum) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncEnum';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anEnum.index]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return AnEnum.values[__pigeon_replyList[0]! as int];
}
}
/// Responds with an error from an async function returning a value.
Future<Object?> throwAsyncError() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwAsyncError';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return __pigeon_replyList[0];
}
}
/// Responds with an error from an async void function.
Future<void> throwAsyncErrorFromVoid() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwAsyncErrorFromVoid';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
/// Responds with a Flutter error from an async function returning a value.
Future<Object?> throwAsyncFlutterError() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwAsyncFlutterError';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return __pigeon_replyList[0];
}
}
/// Returns the passed object, to test async serialization and deserialization.
Future<AllTypes> echoAsyncAllTypes(AllTypes everything) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncAllTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[everything]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as AllTypes?)!;
}
}
/// Returns the passed object, to test serialization and deserialization.
Future<AllNullableTypes?> echoAsyncNullableAllNullableTypes(
AllNullableTypes? everything) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableAllNullableTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[everything]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as AllNullableTypes?);
}
}
/// Returns passed in int asynchronously.
Future<int?> echoAsyncNullableInt(int? anInt) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as int?);
}
}
/// Returns passed in double asynchronously.
Future<double?> echoAsyncNullableDouble(double? aDouble) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableDouble';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aDouble]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as double?);
}
}
/// Returns the passed in boolean asynchronously.
Future<bool?> echoAsyncNullableBool(bool? aBool) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableBool';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aBool]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as bool?);
}
}
/// Returns the passed string asynchronously.
Future<String?> echoAsyncNullableString(String? aString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as String?);
}
}
/// Returns the passed in Uint8List asynchronously.
Future<Uint8List?> echoAsyncNullableUint8List(Uint8List? aUint8List) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableUint8List';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aUint8List]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as Uint8List?);
}
}
/// Returns the passed in generic Object asynchronously.
Future<Object?> echoAsyncNullableObject(Object? anObject) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableObject';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anObject]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return __pigeon_replyList[0];
}
}
/// Returns the passed list, to test asynchronous serialization and deserialization.
Future<List<Object?>?> echoAsyncNullableList(List<Object?>? aList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableList';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as List<Object?>?)?.cast<Object?>();
}
}
/// Returns the passed map, to test asynchronous serialization and deserialization.
Future<Map<String?, Object?>?> echoAsyncNullableMap(
Map<String?, Object?>? aMap) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableMap';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aMap]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as Map<Object?, Object?>?)
?.cast<String?, Object?>();
}
}
/// Returns the passed enum, to test asynchronous serialization and deserialization.
Future<AnEnum?> echoAsyncNullableEnum(AnEnum? anEnum) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableEnum';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anEnum?.index]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as int?) == null
? null
: AnEnum.values[__pigeon_replyList[0]! as int];
}
}
Future<void> callFlutterNoop() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterNoop';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
Future<Object?> callFlutterThrowError() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterThrowError';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return __pigeon_replyList[0];
}
}
Future<void> callFlutterThrowErrorFromVoid() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterThrowErrorFromVoid';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
Future<AllTypes> callFlutterEchoAllTypes(AllTypes everything) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoAllTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[everything]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as AllTypes?)!;
}
}
Future<AllNullableTypes?> callFlutterEchoAllNullableTypes(
AllNullableTypes? everything) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoAllNullableTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[everything]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as AllNullableTypes?);
}
}
Future<AllNullableTypes> callFlutterSendMultipleNullableTypes(
bool? aNullableBool, int? aNullableInt, String? aNullableString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterSendMultipleNullableTypes';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList = await __pigeon_channel
.send(<Object?>[aNullableBool, aNullableInt, aNullableString])
as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as AllNullableTypes?)!;
}
}
Future<bool> callFlutterEchoBool(bool aBool) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoBool';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aBool]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as bool?)!;
}
}
Future<int> callFlutterEchoInt(int anInt) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as int?)!;
}
}
Future<double> callFlutterEchoDouble(double aDouble) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoDouble';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aDouble]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as double?)!;
}
}
Future<String> callFlutterEchoString(String aString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as String?)!;
}
}
Future<Uint8List> callFlutterEchoUint8List(Uint8List aList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoUint8List';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as Uint8List?)!;
}
}
Future<List<Object?>> callFlutterEchoList(List<Object?> aList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoList';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as List<Object?>?)!.cast<Object?>();
}
}
Future<Map<String?, Object?>> callFlutterEchoMap(
Map<String?, Object?> aMap) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoMap';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aMap]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as Map<Object?, Object?>?)!
.cast<String?, Object?>();
}
}
Future<AnEnum> callFlutterEchoEnum(AnEnum anEnum) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoEnum';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anEnum.index]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return AnEnum.values[__pigeon_replyList[0]! as int];
}
}
Future<bool?> callFlutterEchoNullableBool(bool? aBool) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableBool';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aBool]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as bool?);
}
}
Future<int?> callFlutterEchoNullableInt(int? anInt) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableInt';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anInt]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as int?);
}
}
Future<double?> callFlutterEchoNullableDouble(double? aDouble) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableDouble';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aDouble]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as double?);
}
}
Future<String?> callFlutterEchoNullableString(String? aString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableString';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as String?);
}
}
Future<Uint8List?> callFlutterEchoNullableUint8List(Uint8List? aList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableUint8List';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as Uint8List?);
}
}
Future<List<Object?>?> callFlutterEchoNullableList(
List<Object?>? aList) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableList';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aList]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as List<Object?>?)?.cast<Object?>();
}
}
Future<Map<String?, Object?>?> callFlutterEchoNullableMap(
Map<String?, Object?>? aMap) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableMap';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aMap]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as Map<Object?, Object?>?)
?.cast<String?, Object?>();
}
}
Future<AnEnum?> callFlutterEchoNullableEnum(AnEnum? anEnum) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableEnum';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[anEnum?.index]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return (__pigeon_replyList[0] as int?) == null
? null
: AnEnum.values[__pigeon_replyList[0]! as int];
}
}
}
class _FlutterIntegrationCoreApiCodec extends StandardMessageCodec {
const _FlutterIntegrationCoreApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is AllClassesWrapper) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is AllNullableTypes) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is AllTypes) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else if (value is TestMessage) {
buffer.putUint8(131);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return AllClassesWrapper.decode(readValue(buffer)!);
case 129:
return AllNullableTypes.decode(readValue(buffer)!);
case 130:
return AllTypes.decode(readValue(buffer)!);
case 131:
return TestMessage.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// The core interface that the Dart platform_test code implements for host
/// integration tests to call into.
abstract class FlutterIntegrationCoreApi {
static const MessageCodec<Object?> pigeonChannelCodec =
_FlutterIntegrationCoreApiCodec();
/// A no-op function taking no arguments and returning no value, to sanity
/// test basic calling.
void noop();
/// Responds with an error from an async function returning a value.
Object? throwError();
/// Responds with an error from an async void function.
void throwErrorFromVoid();
/// Returns the passed object, to test serialization and deserialization.
AllTypes echoAllTypes(AllTypes everything);
/// Returns the passed object, to test serialization and deserialization.
AllNullableTypes? echoAllNullableTypes(AllNullableTypes? everything);
/// Returns passed in arguments of multiple types.
///
/// Tests multiple-arity FlutterApi handling.
AllNullableTypes sendMultipleNullableTypes(
bool? aNullableBool, int? aNullableInt, String? aNullableString);
/// Returns the passed boolean, to test serialization and deserialization.
bool echoBool(bool aBool);
/// Returns the passed int, to test serialization and deserialization.
int echoInt(int anInt);
/// Returns the passed double, to test serialization and deserialization.
double echoDouble(double aDouble);
/// Returns the passed string, to test serialization and deserialization.
String echoString(String aString);
/// Returns the passed byte list, to test serialization and deserialization.
Uint8List echoUint8List(Uint8List aList);
/// Returns the passed list, to test serialization and deserialization.
List<Object?> echoList(List<Object?> aList);
/// Returns the passed map, to test serialization and deserialization.
Map<String?, Object?> echoMap(Map<String?, Object?> aMap);
/// Returns the passed enum to test serialization and deserialization.
AnEnum echoEnum(AnEnum anEnum);
/// Returns the passed boolean, to test serialization and deserialization.
bool? echoNullableBool(bool? aBool);
/// Returns the passed int, to test serialization and deserialization.
int? echoNullableInt(int? anInt);
/// Returns the passed double, to test serialization and deserialization.
double? echoNullableDouble(double? aDouble);
/// Returns the passed string, to test serialization and deserialization.
String? echoNullableString(String? aString);
/// Returns the passed byte list, to test serialization and deserialization.
Uint8List? echoNullableUint8List(Uint8List? aList);
/// Returns the passed list, to test serialization and deserialization.
List<Object?>? echoNullableList(List<Object?>? aList);
/// Returns the passed map, to test serialization and deserialization.
Map<String?, Object?>? echoNullableMap(Map<String?, Object?>? aMap);
/// Returns the passed enum to test serialization and deserialization.
AnEnum? echoNullableEnum(AnEnum? anEnum);
/// A no-op function taking no arguments and returning no value, to sanity
/// test basic asynchronous calling.
Future<void> noopAsync();
/// Returns the passed in generic Object asynchronously.
Future<String> echoAsyncString(String aString);
static void setup(FlutterIntegrationCoreApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.noop',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
try {
api.noop();
return wrapResponse(empty: true);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.throwError',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
try {
final Object? output = api.throwError();
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.throwErrorFromVoid',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
try {
api.throwErrorFromVoid();
return wrapResponse(empty: true);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAllTypes',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAllTypes was null.');
final List<Object?> args = (message as List<Object?>?)!;
final AllTypes? arg_everything = (args[0] as AllTypes?);
assert(arg_everything != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAllTypes was null, expected non-null AllTypes.');
try {
final AllTypes output = api.echoAllTypes(arg_everything!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAllNullableTypes',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAllNullableTypes was null.');
final List<Object?> args = (message as List<Object?>?)!;
final AllNullableTypes? arg_everything =
(args[0] as AllNullableTypes?);
try {
final AllNullableTypes? output =
api.echoAllNullableTypes(arg_everything);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.sendMultipleNullableTypes',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.sendMultipleNullableTypes was null.');
final List<Object?> args = (message as List<Object?>?)!;
final bool? arg_aNullableBool = (args[0] as bool?);
final int? arg_aNullableInt = (args[1] as int?);
final String? arg_aNullableString = (args[2] as String?);
try {
final AllNullableTypes output = api.sendMultipleNullableTypes(
arg_aNullableBool, arg_aNullableInt, arg_aNullableString);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoBool',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoBool was null.');
final List<Object?> args = (message as List<Object?>?)!;
final bool? arg_aBool = (args[0] as bool?);
assert(arg_aBool != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoBool was null, expected non-null bool.');
try {
final bool output = api.echoBool(arg_aBool!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoInt',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoInt was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_anInt = (args[0] as int?);
assert(arg_anInt != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoInt was null, expected non-null int.');
try {
final int output = api.echoInt(arg_anInt!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoDouble',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoDouble was null.');
final List<Object?> args = (message as List<Object?>?)!;
final double? arg_aDouble = (args[0] as double?);
assert(arg_aDouble != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoDouble was null, expected non-null double.');
try {
final double output = api.echoDouble(arg_aDouble!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoString',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoString was null.');
final List<Object?> args = (message as List<Object?>?)!;
final String? arg_aString = (args[0] as String?);
assert(arg_aString != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoString was null, expected non-null String.');
try {
final String output = api.echoString(arg_aString!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoUint8List',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoUint8List was null.');
final List<Object?> args = (message as List<Object?>?)!;
final Uint8List? arg_aList = (args[0] as Uint8List?);
assert(arg_aList != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoUint8List was null, expected non-null Uint8List.');
try {
final Uint8List output = api.echoUint8List(arg_aList!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoList',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoList was null.');
final List<Object?> args = (message as List<Object?>?)!;
final List<Object?>? arg_aList =
(args[0] as List<Object?>?)?.cast<Object?>();
assert(arg_aList != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoList was null, expected non-null List<Object?>.');
try {
final List<Object?> output = api.echoList(arg_aList!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoMap',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoMap was null.');
final List<Object?> args = (message as List<Object?>?)!;
final Map<String?, Object?>? arg_aMap =
(args[0] as Map<Object?, Object?>?)?.cast<String?, Object?>();
assert(arg_aMap != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoMap was null, expected non-null Map<String?, Object?>.');
try {
final Map<String?, Object?> output = api.echoMap(arg_aMap!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoEnum',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoEnum was null.');
final List<Object?> args = (message as List<Object?>?)!;
final AnEnum? arg_anEnum =
args[0] == null ? null : AnEnum.values[args[0]! as int];
assert(arg_anEnum != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoEnum was null, expected non-null AnEnum.');
try {
final AnEnum output = api.echoEnum(arg_anEnum!);
return wrapResponse(result: output.index);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableBool',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableBool was null.');
final List<Object?> args = (message as List<Object?>?)!;
final bool? arg_aBool = (args[0] as bool?);
try {
final bool? output = api.echoNullableBool(arg_aBool);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableInt',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableInt was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_anInt = (args[0] as int?);
try {
final int? output = api.echoNullableInt(arg_anInt);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableDouble',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableDouble was null.');
final List<Object?> args = (message as List<Object?>?)!;
final double? arg_aDouble = (args[0] as double?);
try {
final double? output = api.echoNullableDouble(arg_aDouble);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableString',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableString was null.');
final List<Object?> args = (message as List<Object?>?)!;
final String? arg_aString = (args[0] as String?);
try {
final String? output = api.echoNullableString(arg_aString);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableUint8List',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableUint8List was null.');
final List<Object?> args = (message as List<Object?>?)!;
final Uint8List? arg_aList = (args[0] as Uint8List?);
try {
final Uint8List? output = api.echoNullableUint8List(arg_aList);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableList',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableList was null.');
final List<Object?> args = (message as List<Object?>?)!;
final List<Object?>? arg_aList =
(args[0] as List<Object?>?)?.cast<Object?>();
try {
final List<Object?>? output = api.echoNullableList(arg_aList);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableMap',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableMap was null.');
final List<Object?> args = (message as List<Object?>?)!;
final Map<String?, Object?>? arg_aMap =
(args[0] as Map<Object?, Object?>?)?.cast<String?, Object?>();
try {
final Map<String?, Object?>? output = api.echoNullableMap(arg_aMap);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableEnum',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableEnum was null.');
final List<Object?> args = (message as List<Object?>?)!;
final AnEnum? arg_anEnum =
args[0] == null ? null : AnEnum.values[args[0]! as int];
try {
final AnEnum? output = api.echoNullableEnum(arg_anEnum);
return wrapResponse(result: output?.index);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.noopAsync',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
try {
await api.noopAsync();
return wrapResponse(empty: true);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAsyncString',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAsyncString was null.');
final List<Object?> args = (message as List<Object?>?)!;
final String? arg_aString = (args[0] as String?);
assert(arg_aString != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAsyncString was null, expected non-null String.');
try {
final String output = await api.echoAsyncString(arg_aString!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
}
}
/// An API that can be implemented for minimal, compile-only tests.
class HostTrivialApi {
/// Constructor for [HostTrivialApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
HostTrivialApi({BinaryMessenger? binaryMessenger})
: __pigeon_binaryMessenger = binaryMessenger;
final BinaryMessenger? __pigeon_binaryMessenger;
static const MessageCodec<Object?> pigeonChannelCodec =
StandardMessageCodec();
Future<void> noop() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostTrivialApi.noop';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
}
/// A simple API implemented in some unit tests.
class HostSmallApi {
/// Constructor for [HostSmallApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
HostSmallApi({BinaryMessenger? binaryMessenger})
: __pigeon_binaryMessenger = binaryMessenger;
final BinaryMessenger? __pigeon_binaryMessenger;
static const MessageCodec<Object?> pigeonChannelCodec =
StandardMessageCodec();
Future<String> echo(String aString) async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostSmallApi.echo';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(<Object?>[aString]) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else if (__pigeon_replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (__pigeon_replyList[0] as String?)!;
}
}
Future<void> voidVoid() async {
const String __pigeon_channelName =
'dev.flutter.pigeon.pigeon_integration_tests.HostSmallApi.voidVoid';
final BasicMessageChannel<Object?> __pigeon_channel =
BasicMessageChannel<Object?>(
__pigeon_channelName,
pigeonChannelCodec,
binaryMessenger: __pigeon_binaryMessenger,
);
final List<Object?>? __pigeon_replyList =
await __pigeon_channel.send(null) as List<Object?>?;
if (__pigeon_replyList == null) {
throw _createConnectionError(__pigeon_channelName);
} else if (__pigeon_replyList.length > 1) {
throw PlatformException(
code: __pigeon_replyList[0]! as String,
message: __pigeon_replyList[1] as String?,
details: __pigeon_replyList[2],
);
} else {
return;
}
}
}
class _FlutterSmallApiCodec extends StandardMessageCodec {
const _FlutterSmallApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is TestMessage) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return TestMessage.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// A simple API called in some unit tests.
abstract class FlutterSmallApi {
static const MessageCodec<Object?> pigeonChannelCodec =
_FlutterSmallApiCodec();
TestMessage echoWrappedList(TestMessage msg);
String echoString(String aString);
static void setup(FlutterSmallApi? api, {BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoWrappedList',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoWrappedList was null.');
final List<Object?> args = (message as List<Object?>?)!;
final TestMessage? arg_msg = (args[0] as TestMessage?);
assert(arg_msg != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoWrappedList was null, expected non-null TestMessage.');
try {
final TestMessage output = api.echoWrappedList(arg_msg!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
{
final BasicMessageChannel<Object?> __pigeon_channel = BasicMessageChannel<
Object?>(
'dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoString',
pigeonChannelCodec,
binaryMessenger: binaryMessenger);
if (api == null) {
__pigeon_channel.setMessageHandler(null);
} else {
__pigeon_channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoString was null.');
final List<Object?> args = (message as List<Object?>?)!;
final String? arg_aString = (args[0] as String?);
assert(arg_aString != null,
'Argument for dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoString was null, expected non-null String.');
try {
final String output = api.echoString(arg_aString!);
return wrapResponse(result: output);
} on PlatformException catch (e) {
return wrapResponse(error: e);
} catch (e) {
return wrapResponse(
error: PlatformException(code: 'error', message: e.toString()));
}
});
}
}
}
}
| packages/packages/pigeon/platform_tests/shared_test_plugin_code/lib/src/generated/core_tests.gen.dart/0 | {
"file_path": "packages/packages/pigeon/platform_tests/shared_test_plugin_code/lib/src/generated/core_tests.gen.dart",
"repo_id": "packages",
"token_count": 55419
} | 1,111 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
//
// Autogenerated from Pigeon, do not edit directly.
// See also: https://pub.dev/packages/pigeon
package com.example.test_plugin
import android.util.Log
import io.flutter.plugin.common.BasicMessageChannel
import io.flutter.plugin.common.BinaryMessenger
import io.flutter.plugin.common.MessageCodec
import io.flutter.plugin.common.StandardMessageCodec
import java.io.ByteArrayOutputStream
import java.nio.ByteBuffer
private fun wrapResult(result: Any?): List<Any?> {
return listOf(result)
}
private fun wrapError(exception: Throwable): List<Any?> {
if (exception is FlutterError) {
return listOf(exception.code, exception.message, exception.details)
} else {
return listOf(
exception.javaClass.simpleName,
exception.toString(),
"Cause: " + exception.cause + ", Stacktrace: " + Log.getStackTraceString(exception))
}
}
private fun createConnectionError(channelName: String): FlutterError {
return FlutterError(
"channel-error", "Unable to establish connection on channel: '$channelName'.", "")
}
enum class AnEnum(val raw: Int) {
ONE(0),
TWO(1),
THREE(2),
FORTY_TWO(3),
FOUR_HUNDRED_TWENTY_TWO(4);
companion object {
fun ofRaw(raw: Int): AnEnum? {
return values().firstOrNull { it.raw == raw }
}
}
}
/**
* A class containing all supported types.
*
* Generated class from Pigeon that represents data sent in messages.
*/
data class AllTypes(
val aBool: Boolean,
val anInt: Long,
val anInt64: Long,
val aDouble: Double,
val aByteArray: ByteArray,
val a4ByteArray: IntArray,
val a8ByteArray: LongArray,
val aFloatArray: DoubleArray,
val aList: List<Any?>,
val aMap: Map<Any, Any?>,
val anEnum: AnEnum,
val aString: String,
val anObject: Any
) {
companion object {
@Suppress("UNCHECKED_CAST")
fun fromList(list: List<Any?>): AllTypes {
val aBool = list[0] as Boolean
val anInt = list[1].let { if (it is Int) it.toLong() else it as Long }
val anInt64 = list[2].let { if (it is Int) it.toLong() else it as Long }
val aDouble = list[3] as Double
val aByteArray = list[4] as ByteArray
val a4ByteArray = list[5] as IntArray
val a8ByteArray = list[6] as LongArray
val aFloatArray = list[7] as DoubleArray
val aList = list[8] as List<Any?>
val aMap = list[9] as Map<Any, Any?>
val anEnum = AnEnum.ofRaw(list[10] as Int)!!
val aString = list[11] as String
val anObject = list[12] as Any
return AllTypes(
aBool,
anInt,
anInt64,
aDouble,
aByteArray,
a4ByteArray,
a8ByteArray,
aFloatArray,
aList,
aMap,
anEnum,
aString,
anObject)
}
}
fun toList(): List<Any?> {
return listOf<Any?>(
aBool,
anInt,
anInt64,
aDouble,
aByteArray,
a4ByteArray,
a8ByteArray,
aFloatArray,
aList,
aMap,
anEnum.raw,
aString,
anObject,
)
}
}
/**
* A class containing all supported nullable types.
*
* Generated class from Pigeon that represents data sent in messages.
*/
data class AllNullableTypes(
val aNullableBool: Boolean? = null,
val aNullableInt: Long? = null,
val aNullableInt64: Long? = null,
val aNullableDouble: Double? = null,
val aNullableByteArray: ByteArray? = null,
val aNullable4ByteArray: IntArray? = null,
val aNullable8ByteArray: LongArray? = null,
val aNullableFloatArray: DoubleArray? = null,
val aNullableList: List<Any?>? = null,
val aNullableMap: Map<Any, Any?>? = null,
val nullableNestedList: List<List<Boolean?>?>? = null,
val nullableMapWithAnnotations: Map<String?, String?>? = null,
val nullableMapWithObject: Map<String?, Any?>? = null,
val aNullableEnum: AnEnum? = null,
val aNullableString: String? = null,
val aNullableObject: Any? = null
) {
companion object {
@Suppress("UNCHECKED_CAST")
fun fromList(list: List<Any?>): AllNullableTypes {
val aNullableBool = list[0] as Boolean?
val aNullableInt = list[1].let { if (it is Int) it.toLong() else it as Long? }
val aNullableInt64 = list[2].let { if (it is Int) it.toLong() else it as Long? }
val aNullableDouble = list[3] as Double?
val aNullableByteArray = list[4] as ByteArray?
val aNullable4ByteArray = list[5] as IntArray?
val aNullable8ByteArray = list[6] as LongArray?
val aNullableFloatArray = list[7] as DoubleArray?
val aNullableList = list[8] as List<Any?>?
val aNullableMap = list[9] as Map<Any, Any?>?
val nullableNestedList = list[10] as List<List<Boolean?>?>?
val nullableMapWithAnnotations = list[11] as Map<String?, String?>?
val nullableMapWithObject = list[12] as Map<String?, Any?>?
val aNullableEnum: AnEnum? = (list[13] as Int?)?.let { AnEnum.ofRaw(it) }
val aNullableString = list[14] as String?
val aNullableObject = list[15]
return AllNullableTypes(
aNullableBool,
aNullableInt,
aNullableInt64,
aNullableDouble,
aNullableByteArray,
aNullable4ByteArray,
aNullable8ByteArray,
aNullableFloatArray,
aNullableList,
aNullableMap,
nullableNestedList,
nullableMapWithAnnotations,
nullableMapWithObject,
aNullableEnum,
aNullableString,
aNullableObject)
}
}
fun toList(): List<Any?> {
return listOf<Any?>(
aNullableBool,
aNullableInt,
aNullableInt64,
aNullableDouble,
aNullableByteArray,
aNullable4ByteArray,
aNullable8ByteArray,
aNullableFloatArray,
aNullableList,
aNullableMap,
nullableNestedList,
nullableMapWithAnnotations,
nullableMapWithObject,
aNullableEnum?.raw,
aNullableString,
aNullableObject,
)
}
}
/**
* A class for testing nested class handling.
*
* This is needed to test nested nullable and non-nullable classes, `AllNullableTypes` is
* non-nullable here as it is easier to instantiate than `AllTypes` when testing doesn't require
* both (ie. testing null classes).
*
* Generated class from Pigeon that represents data sent in messages.
*/
data class AllClassesWrapper(
val allNullableTypes: AllNullableTypes,
val allTypes: AllTypes? = null
) {
companion object {
@Suppress("UNCHECKED_CAST")
fun fromList(list: List<Any?>): AllClassesWrapper {
val allNullableTypes = AllNullableTypes.fromList(list[0] as List<Any?>)
val allTypes: AllTypes? = (list[1] as List<Any?>?)?.let { AllTypes.fromList(it) }
return AllClassesWrapper(allNullableTypes, allTypes)
}
}
fun toList(): List<Any?> {
return listOf<Any?>(
allNullableTypes.toList(),
allTypes?.toList(),
)
}
}
/**
* A data class containing a List, used in unit tests.
*
* Generated class from Pigeon that represents data sent in messages.
*/
data class TestMessage(val testList: List<Any?>? = null) {
companion object {
@Suppress("UNCHECKED_CAST")
fun fromList(list: List<Any?>): TestMessage {
val testList = list[0] as List<Any?>?
return TestMessage(testList)
}
}
fun toList(): List<Any?> {
return listOf<Any?>(
testList,
)
}
}
@Suppress("UNCHECKED_CAST")
private object HostIntegrationCoreApiCodec : StandardMessageCodec() {
override fun readValueOfType(type: Byte, buffer: ByteBuffer): Any? {
return when (type) {
128.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { AllClassesWrapper.fromList(it) }
}
129.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { AllNullableTypes.fromList(it) }
}
130.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { AllTypes.fromList(it) }
}
131.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { TestMessage.fromList(it) }
}
else -> super.readValueOfType(type, buffer)
}
}
override fun writeValue(stream: ByteArrayOutputStream, value: Any?) {
when (value) {
is AllClassesWrapper -> {
stream.write(128)
writeValue(stream, value.toList())
}
is AllNullableTypes -> {
stream.write(129)
writeValue(stream, value.toList())
}
is AllTypes -> {
stream.write(130)
writeValue(stream, value.toList())
}
is TestMessage -> {
stream.write(131)
writeValue(stream, value.toList())
}
else -> super.writeValue(stream, value)
}
}
}
/**
* The core interface that each host language plugin must implement in platform_test integration
* tests.
*
* Generated interface from Pigeon that represents a handler of messages from Flutter.
*/
interface HostIntegrationCoreApi {
/** A no-op function taking no arguments and returning no value, to sanity test basic calling. */
fun noop()
/** Returns the passed object, to test serialization and deserialization. */
fun echoAllTypes(everything: AllTypes): AllTypes
/** Returns an error, to test error handling. */
fun throwError(): Any?
/** Returns an error from a void function, to test error handling. */
fun throwErrorFromVoid()
/** Returns a Flutter error, to test error handling. */
fun throwFlutterError(): Any?
/** Returns passed in int. */
fun echoInt(anInt: Long): Long
/** Returns passed in double. */
fun echoDouble(aDouble: Double): Double
/** Returns the passed in boolean. */
fun echoBool(aBool: Boolean): Boolean
/** Returns the passed in string. */
fun echoString(aString: String): String
/** Returns the passed in Uint8List. */
fun echoUint8List(aUint8List: ByteArray): ByteArray
/** Returns the passed in generic Object. */
fun echoObject(anObject: Any): Any
/** Returns the passed list, to test serialization and deserialization. */
fun echoList(aList: List<Any?>): List<Any?>
/** Returns the passed map, to test serialization and deserialization. */
fun echoMap(aMap: Map<String?, Any?>): Map<String?, Any?>
/** Returns the passed map to test nested class serialization and deserialization. */
fun echoClassWrapper(wrapper: AllClassesWrapper): AllClassesWrapper
/** Returns the passed enum to test serialization and deserialization. */
fun echoEnum(anEnum: AnEnum): AnEnum
/** Returns the default string. */
fun echoNamedDefaultString(aString: String): String
/** Returns passed in double. */
fun echoOptionalDefaultDouble(aDouble: Double): Double
/** Returns passed in int. */
fun echoRequiredInt(anInt: Long): Long
/** Returns the passed object, to test serialization and deserialization. */
fun echoAllNullableTypes(everything: AllNullableTypes?): AllNullableTypes?
/**
* Returns the inner `aString` value from the wrapped object, to test sending of nested objects.
*/
fun extractNestedNullableString(wrapper: AllClassesWrapper): String?
/**
* Returns the inner `aString` value from the wrapped object, to test sending of nested objects.
*/
fun createNestedNullableString(nullableString: String?): AllClassesWrapper
/** Returns passed in arguments of multiple types. */
fun sendMultipleNullableTypes(
aNullableBool: Boolean?,
aNullableInt: Long?,
aNullableString: String?
): AllNullableTypes
/** Returns passed in int. */
fun echoNullableInt(aNullableInt: Long?): Long?
/** Returns passed in double. */
fun echoNullableDouble(aNullableDouble: Double?): Double?
/** Returns the passed in boolean. */
fun echoNullableBool(aNullableBool: Boolean?): Boolean?
/** Returns the passed in string. */
fun echoNullableString(aNullableString: String?): String?
/** Returns the passed in Uint8List. */
fun echoNullableUint8List(aNullableUint8List: ByteArray?): ByteArray?
/** Returns the passed in generic Object. */
fun echoNullableObject(aNullableObject: Any?): Any?
/** Returns the passed list, to test serialization and deserialization. */
fun echoNullableList(aNullableList: List<Any?>?): List<Any?>?
/** Returns the passed map, to test serialization and deserialization. */
fun echoNullableMap(aNullableMap: Map<String?, Any?>?): Map<String?, Any?>?
fun echoNullableEnum(anEnum: AnEnum?): AnEnum?
/** Returns passed in int. */
fun echoOptionalNullableInt(aNullableInt: Long?): Long?
/** Returns the passed in string. */
fun echoNamedNullableString(aNullableString: String?): String?
/**
* A no-op function taking no arguments and returning no value, to sanity test basic asynchronous
* calling.
*/
fun noopAsync(callback: (Result<Unit>) -> Unit)
/** Returns passed in int asynchronously. */
fun echoAsyncInt(anInt: Long, callback: (Result<Long>) -> Unit)
/** Returns passed in double asynchronously. */
fun echoAsyncDouble(aDouble: Double, callback: (Result<Double>) -> Unit)
/** Returns the passed in boolean asynchronously. */
fun echoAsyncBool(aBool: Boolean, callback: (Result<Boolean>) -> Unit)
/** Returns the passed string asynchronously. */
fun echoAsyncString(aString: String, callback: (Result<String>) -> Unit)
/** Returns the passed in Uint8List asynchronously. */
fun echoAsyncUint8List(aUint8List: ByteArray, callback: (Result<ByteArray>) -> Unit)
/** Returns the passed in generic Object asynchronously. */
fun echoAsyncObject(anObject: Any, callback: (Result<Any>) -> Unit)
/** Returns the passed list, to test asynchronous serialization and deserialization. */
fun echoAsyncList(aList: List<Any?>, callback: (Result<List<Any?>>) -> Unit)
/** Returns the passed map, to test asynchronous serialization and deserialization. */
fun echoAsyncMap(aMap: Map<String?, Any?>, callback: (Result<Map<String?, Any?>>) -> Unit)
/** Returns the passed enum, to test asynchronous serialization and deserialization. */
fun echoAsyncEnum(anEnum: AnEnum, callback: (Result<AnEnum>) -> Unit)
/** Responds with an error from an async function returning a value. */
fun throwAsyncError(callback: (Result<Any?>) -> Unit)
/** Responds with an error from an async void function. */
fun throwAsyncErrorFromVoid(callback: (Result<Unit>) -> Unit)
/** Responds with a Flutter error from an async function returning a value. */
fun throwAsyncFlutterError(callback: (Result<Any?>) -> Unit)
/** Returns the passed object, to test async serialization and deserialization. */
fun echoAsyncAllTypes(everything: AllTypes, callback: (Result<AllTypes>) -> Unit)
/** Returns the passed object, to test serialization and deserialization. */
fun echoAsyncNullableAllNullableTypes(
everything: AllNullableTypes?,
callback: (Result<AllNullableTypes?>) -> Unit
)
/** Returns passed in int asynchronously. */
fun echoAsyncNullableInt(anInt: Long?, callback: (Result<Long?>) -> Unit)
/** Returns passed in double asynchronously. */
fun echoAsyncNullableDouble(aDouble: Double?, callback: (Result<Double?>) -> Unit)
/** Returns the passed in boolean asynchronously. */
fun echoAsyncNullableBool(aBool: Boolean?, callback: (Result<Boolean?>) -> Unit)
/** Returns the passed string asynchronously. */
fun echoAsyncNullableString(aString: String?, callback: (Result<String?>) -> Unit)
/** Returns the passed in Uint8List asynchronously. */
fun echoAsyncNullableUint8List(aUint8List: ByteArray?, callback: (Result<ByteArray?>) -> Unit)
/** Returns the passed in generic Object asynchronously. */
fun echoAsyncNullableObject(anObject: Any?, callback: (Result<Any?>) -> Unit)
/** Returns the passed list, to test asynchronous serialization and deserialization. */
fun echoAsyncNullableList(aList: List<Any?>?, callback: (Result<List<Any?>?>) -> Unit)
/** Returns the passed map, to test asynchronous serialization and deserialization. */
fun echoAsyncNullableMap(
aMap: Map<String?, Any?>?,
callback: (Result<Map<String?, Any?>?>) -> Unit
)
/** Returns the passed enum, to test asynchronous serialization and deserialization. */
fun echoAsyncNullableEnum(anEnum: AnEnum?, callback: (Result<AnEnum?>) -> Unit)
fun callFlutterNoop(callback: (Result<Unit>) -> Unit)
fun callFlutterThrowError(callback: (Result<Any?>) -> Unit)
fun callFlutterThrowErrorFromVoid(callback: (Result<Unit>) -> Unit)
fun callFlutterEchoAllTypes(everything: AllTypes, callback: (Result<AllTypes>) -> Unit)
fun callFlutterEchoAllNullableTypes(
everything: AllNullableTypes?,
callback: (Result<AllNullableTypes?>) -> Unit
)
fun callFlutterSendMultipleNullableTypes(
aNullableBool: Boolean?,
aNullableInt: Long?,
aNullableString: String?,
callback: (Result<AllNullableTypes>) -> Unit
)
fun callFlutterEchoBool(aBool: Boolean, callback: (Result<Boolean>) -> Unit)
fun callFlutterEchoInt(anInt: Long, callback: (Result<Long>) -> Unit)
fun callFlutterEchoDouble(aDouble: Double, callback: (Result<Double>) -> Unit)
fun callFlutterEchoString(aString: String, callback: (Result<String>) -> Unit)
fun callFlutterEchoUint8List(aList: ByteArray, callback: (Result<ByteArray>) -> Unit)
fun callFlutterEchoList(aList: List<Any?>, callback: (Result<List<Any?>>) -> Unit)
fun callFlutterEchoMap(aMap: Map<String?, Any?>, callback: (Result<Map<String?, Any?>>) -> Unit)
fun callFlutterEchoEnum(anEnum: AnEnum, callback: (Result<AnEnum>) -> Unit)
fun callFlutterEchoNullableBool(aBool: Boolean?, callback: (Result<Boolean?>) -> Unit)
fun callFlutterEchoNullableInt(anInt: Long?, callback: (Result<Long?>) -> Unit)
fun callFlutterEchoNullableDouble(aDouble: Double?, callback: (Result<Double?>) -> Unit)
fun callFlutterEchoNullableString(aString: String?, callback: (Result<String?>) -> Unit)
fun callFlutterEchoNullableUint8List(aList: ByteArray?, callback: (Result<ByteArray?>) -> Unit)
fun callFlutterEchoNullableList(aList: List<Any?>?, callback: (Result<List<Any?>?>) -> Unit)
fun callFlutterEchoNullableMap(
aMap: Map<String?, Any?>?,
callback: (Result<Map<String?, Any?>?>) -> Unit
)
fun callFlutterEchoNullableEnum(anEnum: AnEnum?, callback: (Result<AnEnum?>) -> Unit)
companion object {
/** The codec used by HostIntegrationCoreApi. */
val codec: MessageCodec<Any?> by lazy { HostIntegrationCoreApiCodec }
/**
* Sets up an instance of `HostIntegrationCoreApi` to handle messages through the
* `binaryMessenger`.
*/
@Suppress("UNCHECKED_CAST")
fun setUp(binaryMessenger: BinaryMessenger, api: HostIntegrationCoreApi?) {
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.noop",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
var wrapped: List<Any?>
try {
api.noop()
wrapped = listOf<Any?>(null)
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAllTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val everythingArg = args[0] as AllTypes
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoAllTypes(everythingArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwError",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.throwError())
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwErrorFromVoid",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
var wrapped: List<Any?>
try {
api.throwErrorFromVoid()
wrapped = listOf<Any?>(null)
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwFlutterError",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.throwFlutterError())
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anIntArg = args[0].let { if (it is Int) it.toLong() else it as Long }
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoInt(anIntArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoDouble",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aDoubleArg = args[0] as Double
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoDouble(aDoubleArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoBool",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aBoolArg = args[0] as Boolean
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoBool(aBoolArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aStringArg = args[0] as String
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoString(aStringArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoUint8List",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aUint8ListArg = args[0] as ByteArray
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoUint8List(aUint8ListArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoObject",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anObjectArg = args[0] as Any
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoObject(anObjectArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoList",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aListArg = args[0] as List<Any?>
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoList(aListArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoMap",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aMapArg = args[0] as Map<String?, Any?>
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoMap(aMapArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoClassWrapper",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val wrapperArg = args[0] as AllClassesWrapper
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoClassWrapper(wrapperArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoEnum",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anEnumArg = AnEnum.ofRaw(args[0] as Int)!!
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoEnum(anEnumArg).raw)
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNamedDefaultString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aStringArg = args[0] as String
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNamedDefaultString(aStringArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoOptionalDefaultDouble",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aDoubleArg = args[0] as Double
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoOptionalDefaultDouble(aDoubleArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoRequiredInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anIntArg = args[0].let { if (it is Int) it.toLong() else it as Long }
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoRequiredInt(anIntArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAllNullableTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val everythingArg = args[0] as AllNullableTypes?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoAllNullableTypes(everythingArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.extractNestedNullableString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val wrapperArg = args[0] as AllClassesWrapper
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.extractNestedNullableString(wrapperArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.createNestedNullableString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val nullableStringArg = args[0] as String?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.createNestedNullableString(nullableStringArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.sendMultipleNullableTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableBoolArg = args[0] as Boolean?
val aNullableIntArg = args[1].let { if (it is Int) it.toLong() else it as Long? }
val aNullableStringArg = args[2] as String?
var wrapped: List<Any?>
try {
wrapped =
listOf<Any?>(
api.sendMultipleNullableTypes(
aNullableBoolArg, aNullableIntArg, aNullableStringArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableIntArg = args[0].let { if (it is Int) it.toLong() else it as Long? }
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableInt(aNullableIntArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableDouble",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableDoubleArg = args[0] as Double?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableDouble(aNullableDoubleArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableBool",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableBoolArg = args[0] as Boolean?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableBool(aNullableBoolArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableStringArg = args[0] as String?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableString(aNullableStringArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableUint8List",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableUint8ListArg = args[0] as ByteArray?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableUint8List(aNullableUint8ListArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableObject",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableObjectArg = args[0]
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableObject(aNullableObjectArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableList",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableListArg = args[0] as List<Any?>?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableList(aNullableListArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableMap",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableMapArg = args[0] as Map<String?, Any?>?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableMap(aNullableMapArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNullableEnum",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anEnumArg = if (args[0] == null) null else AnEnum.ofRaw(args[0] as Int)
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNullableEnum(anEnumArg)?.raw)
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoOptionalNullableInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableIntArg = args[0].let { if (it is Int) it.toLong() else it as Long? }
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoOptionalNullableInt(aNullableIntArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoNamedNullableString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableStringArg = args[0] as String?
var wrapped: List<Any?>
try {
wrapped = listOf<Any?>(api.echoNamedNullableString(aNullableStringArg))
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.noopAsync",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.noopAsync() { result: Result<Unit> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
reply.reply(wrapResult(null))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anIntArg = args[0].let { if (it is Int) it.toLong() else it as Long }
api.echoAsyncInt(anIntArg) { result: Result<Long> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncDouble",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aDoubleArg = args[0] as Double
api.echoAsyncDouble(aDoubleArg) { result: Result<Double> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncBool",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aBoolArg = args[0] as Boolean
api.echoAsyncBool(aBoolArg) { result: Result<Boolean> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aStringArg = args[0] as String
api.echoAsyncString(aStringArg) { result: Result<String> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncUint8List",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aUint8ListArg = args[0] as ByteArray
api.echoAsyncUint8List(aUint8ListArg) { result: Result<ByteArray> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncObject",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anObjectArg = args[0] as Any
api.echoAsyncObject(anObjectArg) { result: Result<Any> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncList",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aListArg = args[0] as List<Any?>
api.echoAsyncList(aListArg) { result: Result<List<Any?>> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncMap",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aMapArg = args[0] as Map<String?, Any?>
api.echoAsyncMap(aMapArg) { result: Result<Map<String?, Any?>> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncEnum",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anEnumArg = AnEnum.ofRaw(args[0] as Int)!!
api.echoAsyncEnum(anEnumArg) { result: Result<AnEnum> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data!!.raw))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwAsyncError",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.throwAsyncError() { result: Result<Any?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwAsyncErrorFromVoid",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.throwAsyncErrorFromVoid() { result: Result<Unit> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
reply.reply(wrapResult(null))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.throwAsyncFlutterError",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.throwAsyncFlutterError() { result: Result<Any?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncAllTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val everythingArg = args[0] as AllTypes
api.echoAsyncAllTypes(everythingArg) { result: Result<AllTypes> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableAllNullableTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val everythingArg = args[0] as AllNullableTypes?
api.echoAsyncNullableAllNullableTypes(everythingArg) { result: Result<AllNullableTypes?>
->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anIntArg = args[0].let { if (it is Int) it.toLong() else it as Long? }
api.echoAsyncNullableInt(anIntArg) { result: Result<Long?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableDouble",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aDoubleArg = args[0] as Double?
api.echoAsyncNullableDouble(aDoubleArg) { result: Result<Double?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableBool",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aBoolArg = args[0] as Boolean?
api.echoAsyncNullableBool(aBoolArg) { result: Result<Boolean?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aStringArg = args[0] as String?
api.echoAsyncNullableString(aStringArg) { result: Result<String?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableUint8List",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aUint8ListArg = args[0] as ByteArray?
api.echoAsyncNullableUint8List(aUint8ListArg) { result: Result<ByteArray?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableObject",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anObjectArg = args[0]
api.echoAsyncNullableObject(anObjectArg) { result: Result<Any?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableList",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aListArg = args[0] as List<Any?>?
api.echoAsyncNullableList(aListArg) { result: Result<List<Any?>?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableMap",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aMapArg = args[0] as Map<String?, Any?>?
api.echoAsyncNullableMap(aMapArg) { result: Result<Map<String?, Any?>?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.echoAsyncNullableEnum",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anEnumArg = if (args[0] == null) null else AnEnum.ofRaw(args[0] as Int)
api.echoAsyncNullableEnum(anEnumArg) { result: Result<AnEnum?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data?.raw))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterNoop",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.callFlutterNoop() { result: Result<Unit> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
reply.reply(wrapResult(null))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterThrowError",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.callFlutterThrowError() { result: Result<Any?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterThrowErrorFromVoid",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.callFlutterThrowErrorFromVoid() { result: Result<Unit> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
reply.reply(wrapResult(null))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoAllTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val everythingArg = args[0] as AllTypes
api.callFlutterEchoAllTypes(everythingArg) { result: Result<AllTypes> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoAllNullableTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val everythingArg = args[0] as AllNullableTypes?
api.callFlutterEchoAllNullableTypes(everythingArg) { result: Result<AllNullableTypes?>
->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterSendMultipleNullableTypes",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aNullableBoolArg = args[0] as Boolean?
val aNullableIntArg = args[1].let { if (it is Int) it.toLong() else it as Long? }
val aNullableStringArg = args[2] as String?
api.callFlutterSendMultipleNullableTypes(
aNullableBoolArg, aNullableIntArg, aNullableStringArg) {
result: Result<AllNullableTypes> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoBool",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aBoolArg = args[0] as Boolean
api.callFlutterEchoBool(aBoolArg) { result: Result<Boolean> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anIntArg = args[0].let { if (it is Int) it.toLong() else it as Long }
api.callFlutterEchoInt(anIntArg) { result: Result<Long> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoDouble",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aDoubleArg = args[0] as Double
api.callFlutterEchoDouble(aDoubleArg) { result: Result<Double> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aStringArg = args[0] as String
api.callFlutterEchoString(aStringArg) { result: Result<String> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoUint8List",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aListArg = args[0] as ByteArray
api.callFlutterEchoUint8List(aListArg) { result: Result<ByteArray> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoList",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aListArg = args[0] as List<Any?>
api.callFlutterEchoList(aListArg) { result: Result<List<Any?>> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoMap",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aMapArg = args[0] as Map<String?, Any?>
api.callFlutterEchoMap(aMapArg) { result: Result<Map<String?, Any?>> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoEnum",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anEnumArg = AnEnum.ofRaw(args[0] as Int)!!
api.callFlutterEchoEnum(anEnumArg) { result: Result<AnEnum> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data!!.raw))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableBool",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aBoolArg = args[0] as Boolean?
api.callFlutterEchoNullableBool(aBoolArg) { result: Result<Boolean?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableInt",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anIntArg = args[0].let { if (it is Int) it.toLong() else it as Long? }
api.callFlutterEchoNullableInt(anIntArg) { result: Result<Long?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableDouble",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aDoubleArg = args[0] as Double?
api.callFlutterEchoNullableDouble(aDoubleArg) { result: Result<Double?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableString",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aStringArg = args[0] as String?
api.callFlutterEchoNullableString(aStringArg) { result: Result<String?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableUint8List",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aListArg = args[0] as ByteArray?
api.callFlutterEchoNullableUint8List(aListArg) { result: Result<ByteArray?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableList",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aListArg = args[0] as List<Any?>?
api.callFlutterEchoNullableList(aListArg) { result: Result<List<Any?>?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableMap",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aMapArg = args[0] as Map<String?, Any?>?
api.callFlutterEchoNullableMap(aMapArg) { result: Result<Map<String?, Any?>?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostIntegrationCoreApi.callFlutterEchoNullableEnum",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val anEnumArg = if (args[0] == null) null else AnEnum.ofRaw(args[0] as Int)
api.callFlutterEchoNullableEnum(anEnumArg) { result: Result<AnEnum?> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data?.raw))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
}
}
}
@Suppress("UNCHECKED_CAST")
private object FlutterIntegrationCoreApiCodec : StandardMessageCodec() {
override fun readValueOfType(type: Byte, buffer: ByteBuffer): Any? {
return when (type) {
128.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { AllClassesWrapper.fromList(it) }
}
129.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { AllNullableTypes.fromList(it) }
}
130.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { AllTypes.fromList(it) }
}
131.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { TestMessage.fromList(it) }
}
else -> super.readValueOfType(type, buffer)
}
}
override fun writeValue(stream: ByteArrayOutputStream, value: Any?) {
when (value) {
is AllClassesWrapper -> {
stream.write(128)
writeValue(stream, value.toList())
}
is AllNullableTypes -> {
stream.write(129)
writeValue(stream, value.toList())
}
is AllTypes -> {
stream.write(130)
writeValue(stream, value.toList())
}
is TestMessage -> {
stream.write(131)
writeValue(stream, value.toList())
}
else -> super.writeValue(stream, value)
}
}
}
/**
* The core interface that the Dart platform_test code implements for host integration tests to call
* into.
*
* Generated class from Pigeon that represents Flutter messages that can be called from Kotlin.
*/
@Suppress("UNCHECKED_CAST")
class FlutterIntegrationCoreApi(private val binaryMessenger: BinaryMessenger) {
companion object {
/** The codec used by FlutterIntegrationCoreApi. */
val codec: MessageCodec<Any?> by lazy { FlutterIntegrationCoreApiCodec }
}
/** A no-op function taking no arguments and returning no value, to sanity test basic calling. */
fun noop(callback: (Result<Unit>) -> Unit) {
val channelName = "dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.noop"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(null) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
callback(Result.success(Unit))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Responds with an error from an async function returning a value. */
fun throwError(callback: (Result<Any?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.throwError"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(null) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0]
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Responds with an error from an async void function. */
fun throwErrorFromVoid(callback: (Result<Unit>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.throwErrorFromVoid"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(null) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
callback(Result.success(Unit))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed object, to test serialization and deserialization. */
fun echoAllTypes(everythingArg: AllTypes, callback: (Result<AllTypes>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAllTypes"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(everythingArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as AllTypes
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed object, to test serialization and deserialization. */
fun echoAllNullableTypes(
everythingArg: AllNullableTypes?,
callback: (Result<AllNullableTypes?>) -> Unit
) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAllNullableTypes"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(everythingArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0] as AllNullableTypes?
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/**
* Returns passed in arguments of multiple types.
*
* Tests multiple-arity FlutterApi handling.
*/
fun sendMultipleNullableTypes(
aNullableBoolArg: Boolean?,
aNullableIntArg: Long?,
aNullableStringArg: String?,
callback: (Result<AllNullableTypes>) -> Unit
) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.sendMultipleNullableTypes"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aNullableBoolArg, aNullableIntArg, aNullableStringArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as AllNullableTypes
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed boolean, to test serialization and deserialization. */
fun echoBool(aBoolArg: Boolean, callback: (Result<Boolean>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoBool"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aBoolArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as Boolean
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed int, to test serialization and deserialization. */
fun echoInt(anIntArg: Long, callback: (Result<Long>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoInt"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(anIntArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0].let { if (it is Int) it.toLong() else it as Long }
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed double, to test serialization and deserialization. */
fun echoDouble(aDoubleArg: Double, callback: (Result<Double>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoDouble"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aDoubleArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as Double
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed string, to test serialization and deserialization. */
fun echoString(aStringArg: String, callback: (Result<String>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoString"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aStringArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as String
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed byte list, to test serialization and deserialization. */
fun echoUint8List(aListArg: ByteArray, callback: (Result<ByteArray>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoUint8List"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aListArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as ByteArray
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed list, to test serialization and deserialization. */
fun echoList(aListArg: List<Any?>, callback: (Result<List<Any?>>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoList"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aListArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as List<Any?>
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed map, to test serialization and deserialization. */
fun echoMap(aMapArg: Map<String?, Any?>, callback: (Result<Map<String?, Any?>>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoMap"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aMapArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as Map<String?, Any?>
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed enum to test serialization and deserialization. */
fun echoEnum(anEnumArg: AnEnum, callback: (Result<AnEnum>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoEnum"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(anEnumArg.raw)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = AnEnum.ofRaw(it[0] as Int)!!
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed boolean, to test serialization and deserialization. */
fun echoNullableBool(aBoolArg: Boolean?, callback: (Result<Boolean?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableBool"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aBoolArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0] as Boolean?
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed int, to test serialization and deserialization. */
fun echoNullableInt(anIntArg: Long?, callback: (Result<Long?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableInt"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(anIntArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0].let { if (it is Int) it.toLong() else it as Long? }
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed double, to test serialization and deserialization. */
fun echoNullableDouble(aDoubleArg: Double?, callback: (Result<Double?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableDouble"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aDoubleArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0] as Double?
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed string, to test serialization and deserialization. */
fun echoNullableString(aStringArg: String?, callback: (Result<String?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableString"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aStringArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0] as String?
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed byte list, to test serialization and deserialization. */
fun echoNullableUint8List(aListArg: ByteArray?, callback: (Result<ByteArray?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableUint8List"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aListArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0] as ByteArray?
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed list, to test serialization and deserialization. */
fun echoNullableList(aListArg: List<Any?>?, callback: (Result<List<Any?>?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableList"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aListArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0] as List<Any?>?
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed map, to test serialization and deserialization. */
fun echoNullableMap(
aMapArg: Map<String?, Any?>?,
callback: (Result<Map<String?, Any?>?>) -> Unit
) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableMap"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aMapArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = it[0] as Map<String?, Any?>?
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed enum to test serialization and deserialization. */
fun echoNullableEnum(anEnumArg: AnEnum?, callback: (Result<AnEnum?>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoNullableEnum"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(anEnumArg?.raw)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
val output = (it[0] as Int?)?.let { AnEnum.ofRaw(it) }
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/**
* A no-op function taking no arguments and returning no value, to sanity test basic asynchronous
* calling.
*/
fun noopAsync(callback: (Result<Unit>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.noopAsync"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(null) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else {
callback(Result.success(Unit))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
/** Returns the passed in generic Object asynchronously. */
fun echoAsyncString(aStringArg: String, callback: (Result<String>) -> Unit) {
val channelName =
"dev.flutter.pigeon.pigeon_integration_tests.FlutterIntegrationCoreApi.echoAsyncString"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aStringArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as String
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
}
/**
* An API that can be implemented for minimal, compile-only tests.
*
* Generated interface from Pigeon that represents a handler of messages from Flutter.
*/
interface HostTrivialApi {
fun noop()
companion object {
/** The codec used by HostTrivialApi. */
val codec: MessageCodec<Any?> by lazy { StandardMessageCodec() }
/** Sets up an instance of `HostTrivialApi` to handle messages through the `binaryMessenger`. */
@Suppress("UNCHECKED_CAST")
fun setUp(binaryMessenger: BinaryMessenger, api: HostTrivialApi?) {
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostTrivialApi.noop",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
var wrapped: List<Any?>
try {
api.noop()
wrapped = listOf<Any?>(null)
} catch (exception: Throwable) {
wrapped = wrapError(exception)
}
reply.reply(wrapped)
}
} else {
channel.setMessageHandler(null)
}
}
}
}
}
/**
* A simple API implemented in some unit tests.
*
* Generated interface from Pigeon that represents a handler of messages from Flutter.
*/
interface HostSmallApi {
fun echo(aString: String, callback: (Result<String>) -> Unit)
fun voidVoid(callback: (Result<Unit>) -> Unit)
companion object {
/** The codec used by HostSmallApi. */
val codec: MessageCodec<Any?> by lazy { StandardMessageCodec() }
/** Sets up an instance of `HostSmallApi` to handle messages through the `binaryMessenger`. */
@Suppress("UNCHECKED_CAST")
fun setUp(binaryMessenger: BinaryMessenger, api: HostSmallApi?) {
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostSmallApi.echo",
codec)
if (api != null) {
channel.setMessageHandler { message, reply ->
val args = message as List<Any?>
val aStringArg = args[0] as String
api.echo(aStringArg) { result: Result<String> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
val data = result.getOrNull()
reply.reply(wrapResult(data))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
run {
val channel =
BasicMessageChannel<Any?>(
binaryMessenger,
"dev.flutter.pigeon.pigeon_integration_tests.HostSmallApi.voidVoid",
codec)
if (api != null) {
channel.setMessageHandler { _, reply ->
api.voidVoid() { result: Result<Unit> ->
val error = result.exceptionOrNull()
if (error != null) {
reply.reply(wrapError(error))
} else {
reply.reply(wrapResult(null))
}
}
}
} else {
channel.setMessageHandler(null)
}
}
}
}
}
@Suppress("UNCHECKED_CAST")
private object FlutterSmallApiCodec : StandardMessageCodec() {
override fun readValueOfType(type: Byte, buffer: ByteBuffer): Any? {
return when (type) {
128.toByte() -> {
return (readValue(buffer) as? List<Any?>)?.let { TestMessage.fromList(it) }
}
else -> super.readValueOfType(type, buffer)
}
}
override fun writeValue(stream: ByteArrayOutputStream, value: Any?) {
when (value) {
is TestMessage -> {
stream.write(128)
writeValue(stream, value.toList())
}
else -> super.writeValue(stream, value)
}
}
}
/**
* A simple API called in some unit tests.
*
* Generated class from Pigeon that represents Flutter messages that can be called from Kotlin.
*/
@Suppress("UNCHECKED_CAST")
class FlutterSmallApi(private val binaryMessenger: BinaryMessenger) {
companion object {
/** The codec used by FlutterSmallApi. */
val codec: MessageCodec<Any?> by lazy { FlutterSmallApiCodec }
}
fun echoWrappedList(msgArg: TestMessage, callback: (Result<TestMessage>) -> Unit) {
val channelName = "dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoWrappedList"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(msgArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as TestMessage
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
fun echoString(aStringArg: String, callback: (Result<String>) -> Unit) {
val channelName = "dev.flutter.pigeon.pigeon_integration_tests.FlutterSmallApi.echoString"
val channel = BasicMessageChannel<Any?>(binaryMessenger, channelName, codec)
channel.send(listOf(aStringArg)) {
if (it is List<*>) {
if (it.size > 1) {
callback(Result.failure(FlutterError(it[0] as String, it[1] as String, it[2] as String?)))
} else if (it[0] == null) {
callback(
Result.failure(
FlutterError(
"null-error",
"Flutter api returned null value for non-null return value.",
"")))
} else {
val output = it[0] as String
callback(Result.success(output))
}
} else {
callback(Result.failure(createConnectionError(channelName)))
}
}
}
}
| packages/packages/pigeon/platform_tests/test_plugin/android/src/main/kotlin/com/example/test_plugin/CoreTests.gen.kt/0 | {
"file_path": "packages/packages/pigeon/platform_tests/test_plugin/android/src/main/kotlin/com/example/test_plugin/CoreTests.gen.kt",
"repo_id": "packages",
"token_count": 54249
} | 1,112 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import XCTest
@testable import test_plugin
class NonNullFieldsTests: XCTestCase {
func testMake() {
let request = NonNullFieldSearchRequest(query: "hello")
XCTAssertEqual("hello", request.query)
}
}
| packages/packages/pigeon/platform_tests/test_plugin/example/ios/RunnerTests/NonNullFieldsTest.swift/0 | {
"file_path": "packages/packages/pigeon/platform_tests/test_plugin/example/ios/RunnerTests/NonNullFieldsTest.swift",
"repo_id": "packages",
"token_count": 118
} | 1,113 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "test_plugin.h"
// This must be included before many other Windows headers.
#include <flutter/plugin_registrar_windows.h>
#include <windows.h>
#include <memory>
#include <optional>
#include <string>
#include "pigeon/core_tests.gen.h"
namespace test_plugin {
using core_tests_pigeontest::AllClassesWrapper;
using core_tests_pigeontest::AllNullableTypes;
using core_tests_pigeontest::AllTypes;
using core_tests_pigeontest::AnEnum;
using core_tests_pigeontest::ErrorOr;
using core_tests_pigeontest::FlutterError;
using core_tests_pigeontest::FlutterIntegrationCoreApi;
using core_tests_pigeontest::HostIntegrationCoreApi;
using flutter::EncodableList;
using flutter::EncodableMap;
using flutter::EncodableValue;
// static
void TestPlugin::RegisterWithRegistrar(
flutter::PluginRegistrarWindows* registrar) {
auto plugin = std::make_unique<TestPlugin>(registrar->messenger());
HostIntegrationCoreApi::SetUp(registrar->messenger(), plugin.get());
registrar->AddPlugin(std::move(plugin));
}
TestPlugin::TestPlugin(flutter::BinaryMessenger* binary_messenger)
: flutter_api_(
std::make_unique<FlutterIntegrationCoreApi>(binary_messenger)) {}
TestPlugin::~TestPlugin() {}
std::optional<FlutterError> TestPlugin::Noop() { return std::nullopt; }
ErrorOr<AllTypes> TestPlugin::EchoAllTypes(const AllTypes& everything) {
return everything;
}
ErrorOr<std::optional<AllNullableTypes>> TestPlugin::EchoAllNullableTypes(
const AllNullableTypes* everything) {
if (!everything) {
return std::nullopt;
}
return *everything;
}
ErrorOr<std::optional<flutter::EncodableValue>> TestPlugin::ThrowError() {
return FlutterError("An error");
}
std::optional<FlutterError> TestPlugin::ThrowErrorFromVoid() {
return FlutterError("An error");
}
ErrorOr<std::optional<flutter::EncodableValue>>
TestPlugin::ThrowFlutterError() {
return FlutterError("code", "message", EncodableValue("details"));
}
ErrorOr<int64_t> TestPlugin::EchoInt(int64_t an_int) { return an_int; }
ErrorOr<double> TestPlugin::EchoDouble(double a_double) { return a_double; }
ErrorOr<bool> TestPlugin::EchoBool(bool a_bool) { return a_bool; }
ErrorOr<std::string> TestPlugin::EchoString(const std::string& a_string) {
return a_string;
}
ErrorOr<std::vector<uint8_t>> TestPlugin::EchoUint8List(
const std::vector<uint8_t>& a_uint8_list) {
return a_uint8_list;
}
ErrorOr<EncodableValue> TestPlugin::EchoObject(
const EncodableValue& an_object) {
return an_object;
}
ErrorOr<EncodableList> TestPlugin::EchoList(const EncodableList& a_list) {
return a_list;
}
ErrorOr<EncodableMap> TestPlugin::EchoMap(const EncodableMap& a_map) {
return a_map;
}
ErrorOr<AllClassesWrapper> TestPlugin::EchoClassWrapper(
const AllClassesWrapper& wrapper) {
return wrapper;
}
ErrorOr<AnEnum> TestPlugin::EchoEnum(const AnEnum& an_enum) { return an_enum; }
ErrorOr<std::string> TestPlugin::EchoNamedDefaultString(
const std::string& a_string) {
return a_string;
}
ErrorOr<double> TestPlugin::EchoOptionalDefaultDouble(double a_double) {
return a_double;
}
ErrorOr<int64_t> TestPlugin::EchoRequiredInt(int64_t an_int) { return an_int; }
ErrorOr<std::optional<std::string>> TestPlugin::ExtractNestedNullableString(
const AllClassesWrapper& wrapper) {
const std::string* inner_string =
wrapper.all_nullable_types().a_nullable_string();
return inner_string ? std::optional<std::string>(*inner_string)
: std::nullopt;
}
ErrorOr<AllClassesWrapper> TestPlugin::CreateNestedNullableString(
const std::string* nullable_string) {
AllNullableTypes inner_object;
// The string pointer can't be passed through directly since the setter for
// a string takes a std::string_view rather than std::string so the pointer
// types don't match.
if (nullable_string) {
inner_object.set_a_nullable_string(*nullable_string);
} else {
inner_object.set_a_nullable_string(nullptr);
}
AllClassesWrapper wrapper(inner_object);
return wrapper;
}
ErrorOr<AllNullableTypes> TestPlugin::SendMultipleNullableTypes(
const bool* a_nullable_bool, const int64_t* a_nullable_int,
const std::string* a_nullable_string) {
AllNullableTypes someTypes;
someTypes.set_a_nullable_bool(a_nullable_bool);
someTypes.set_a_nullable_int(a_nullable_int);
// The string pointer can't be passed through directly since the setter for
// a string takes a std::string_view rather than std::string so the pointer
// types don't match.
if (a_nullable_string) {
someTypes.set_a_nullable_string(*a_nullable_string);
} else {
someTypes.set_a_nullable_string(nullptr);
}
return someTypes;
};
ErrorOr<std::optional<int64_t>> TestPlugin::EchoNullableInt(
const int64_t* a_nullable_int) {
if (!a_nullable_int) {
return std::nullopt;
}
return *a_nullable_int;
};
ErrorOr<std::optional<double>> TestPlugin::EchoNullableDouble(
const double* a_nullable_double) {
if (!a_nullable_double) {
return std::nullopt;
}
return *a_nullable_double;
};
ErrorOr<std::optional<bool>> TestPlugin::EchoNullableBool(
const bool* a_nullable_bool) {
if (!a_nullable_bool) {
return std::nullopt;
}
return *a_nullable_bool;
};
ErrorOr<std::optional<std::string>> TestPlugin::EchoNullableString(
const std::string* a_nullable_string) {
if (!a_nullable_string) {
return std::nullopt;
}
return *a_nullable_string;
};
ErrorOr<std::optional<std::vector<uint8_t>>> TestPlugin::EchoNullableUint8List(
const std::vector<uint8_t>* a_nullable_uint8_list) {
if (!a_nullable_uint8_list) {
return std::nullopt;
}
return *a_nullable_uint8_list;
};
ErrorOr<std::optional<EncodableValue>> TestPlugin::EchoNullableObject(
const EncodableValue* a_nullable_object) {
if (!a_nullable_object) {
return std::nullopt;
}
return *a_nullable_object;
};
ErrorOr<std::optional<EncodableList>> TestPlugin::EchoNullableList(
const EncodableList* a_nullable_list) {
if (!a_nullable_list) {
return std::nullopt;
}
return *a_nullable_list;
};
ErrorOr<std::optional<EncodableMap>> TestPlugin::EchoNullableMap(
const EncodableMap* a_nullable_map) {
if (!a_nullable_map) {
return std::nullopt;
}
return *a_nullable_map;
};
ErrorOr<std::optional<AnEnum>> TestPlugin::EchoNullableEnum(
const AnEnum* an_enum) {
if (!an_enum) {
return std::nullopt;
}
return *an_enum;
}
ErrorOr<std::optional<int64_t>> TestPlugin::EchoOptionalNullableInt(
const int64_t* a_nullable_int) {
if (!a_nullable_int) {
return std::nullopt;
}
return *a_nullable_int;
}
ErrorOr<std::optional<std::string>> TestPlugin::EchoNamedNullableString(
const std::string* a_nullable_string) {
if (!a_nullable_string) {
return std::nullopt;
}
return *a_nullable_string;
}
void TestPlugin::NoopAsync(
std::function<void(std::optional<FlutterError> reply)> result) {
result(std::nullopt);
}
void TestPlugin::ThrowAsyncError(
std::function<void(ErrorOr<std::optional<EncodableValue>> reply)> result) {
result(FlutterError("code", "message", EncodableValue("details")));
}
void TestPlugin::ThrowAsyncErrorFromVoid(
std::function<void(std::optional<FlutterError> reply)> result) {
result(FlutterError("code", "message", EncodableValue("details")));
}
void TestPlugin::ThrowAsyncFlutterError(
std::function<void(ErrorOr<std::optional<EncodableValue>> reply)> result) {
result(FlutterError("code", "message", EncodableValue("details")));
}
void TestPlugin::EchoAsyncAllTypes(
const AllTypes& everything,
std::function<void(ErrorOr<AllTypes> reply)> result) {
result(everything);
}
void TestPlugin::EchoAsyncInt(
int64_t an_int, std::function<void(ErrorOr<int64_t> reply)> result) {
result(an_int);
}
void TestPlugin::EchoAsyncDouble(
double a_double, std::function<void(ErrorOr<double> reply)> result) {
result(a_double);
}
void TestPlugin::EchoAsyncBool(
bool a_bool, std::function<void(ErrorOr<bool> reply)> result) {
result(a_bool);
}
void TestPlugin::EchoAsyncString(
const std::string& a_string,
std::function<void(ErrorOr<std::string> reply)> result) {
result(a_string);
}
void TestPlugin::EchoAsyncUint8List(
const std::vector<uint8_t>& a_uint8_list,
std::function<void(ErrorOr<std::vector<uint8_t>> reply)> result) {
result(a_uint8_list);
}
void TestPlugin::EchoAsyncObject(
const EncodableValue& an_object,
std::function<void(ErrorOr<EncodableValue> reply)> result) {
result(an_object);
}
void TestPlugin::EchoAsyncList(
const EncodableList& a_list,
std::function<void(ErrorOr<EncodableList> reply)> result) {
result(a_list);
}
void TestPlugin::EchoAsyncMap(
const EncodableMap& a_map,
std::function<void(ErrorOr<EncodableMap> reply)> result) {
result(a_map);
}
void TestPlugin::EchoAsyncEnum(
const AnEnum& an_enum, std::function<void(ErrorOr<AnEnum> reply)> result) {
result(an_enum);
}
void TestPlugin::EchoAsyncNullableAllNullableTypes(
const AllNullableTypes* everything,
std::function<void(ErrorOr<std::optional<AllNullableTypes>> reply)>
result) {
result(everything ? std::optional<AllNullableTypes>(*everything)
: std::nullopt);
}
void TestPlugin::EchoAsyncNullableInt(
const int64_t* an_int,
std::function<void(ErrorOr<std::optional<int64_t>> reply)> result) {
result(an_int ? std::optional<int64_t>(*an_int) : std::nullopt);
}
void TestPlugin::EchoAsyncNullableDouble(
const double* a_double,
std::function<void(ErrorOr<std::optional<double>> reply)> result) {
result(a_double ? std::optional<double>(*a_double) : std::nullopt);
}
void TestPlugin::EchoAsyncNullableBool(
const bool* a_bool,
std::function<void(ErrorOr<std::optional<bool>> reply)> result) {
result(a_bool ? std::optional<bool>(*a_bool) : std::nullopt);
}
void TestPlugin::EchoAsyncNullableString(
const std::string* a_string,
std::function<void(ErrorOr<std::optional<std::string>> reply)> result) {
result(a_string ? std::optional<std::string>(*a_string) : std::nullopt);
}
void TestPlugin::EchoAsyncNullableUint8List(
const std::vector<uint8_t>* a_uint8_list,
std::function<void(ErrorOr<std::optional<std::vector<uint8_t>>> reply)>
result) {
result(a_uint8_list ? std::optional<std::vector<uint8_t>>(*a_uint8_list)
: std::nullopt);
}
void TestPlugin::EchoAsyncNullableObject(
const EncodableValue* an_object,
std::function<void(ErrorOr<std::optional<EncodableValue>> reply)> result) {
result(an_object ? std::optional<EncodableValue>(*an_object) : std::nullopt);
}
void TestPlugin::EchoAsyncNullableList(
const EncodableList* a_list,
std::function<void(ErrorOr<std::optional<EncodableList>> reply)> result) {
result(a_list ? std::optional<EncodableList>(*a_list) : std::nullopt);
}
void TestPlugin::EchoAsyncNullableMap(
const EncodableMap* a_map,
std::function<void(ErrorOr<std::optional<EncodableMap>> reply)> result) {
result(a_map ? std::optional<EncodableMap>(*a_map) : std::nullopt);
}
void TestPlugin::EchoAsyncNullableEnum(
const AnEnum* an_enum,
std::function<void(ErrorOr<std::optional<AnEnum>> reply)> result) {
result(an_enum ? std::optional<AnEnum>(*an_enum) : std::nullopt);
}
void TestPlugin::CallFlutterNoop(
std::function<void(std::optional<FlutterError> reply)> result) {
flutter_api_->Noop([result]() { result(std::nullopt); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterThrowError(
std::function<void(ErrorOr<std::optional<flutter::EncodableValue>> reply)>
result) {
flutter_api_->ThrowError(
[result](const std::optional<flutter::EncodableValue>& echo) {
result(echo);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterThrowErrorFromVoid(
std::function<void(std::optional<FlutterError> reply)> result) {
flutter_api_->ThrowErrorFromVoid(
[result]() { result(std::nullopt); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoAllTypes(
const AllTypes& everything,
std::function<void(ErrorOr<AllTypes> reply)> result) {
flutter_api_->EchoAllTypes(
everything, [result](const AllTypes& echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoAllNullableTypes(
const AllNullableTypes* everything,
std::function<void(ErrorOr<std::optional<AllNullableTypes>> reply)>
result) {
flutter_api_->EchoAllNullableTypes(
everything,
[result](const AllNullableTypes* echo) {
result(echo ? std::optional<AllNullableTypes>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterSendMultipleNullableTypes(
const bool* a_nullable_bool, const int64_t* a_nullable_int,
const std::string* a_nullable_string,
std::function<void(ErrorOr<AllNullableTypes> reply)> result) {
flutter_api_->SendMultipleNullableTypes(
a_nullable_bool, a_nullable_int, a_nullable_string,
[result](const AllNullableTypes& echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoBool(
bool a_bool, std::function<void(ErrorOr<bool> reply)> result) {
flutter_api_->EchoBool(
a_bool, [result](bool echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoInt(
int64_t an_int, std::function<void(ErrorOr<int64_t> reply)> result) {
flutter_api_->EchoInt(
an_int, [result](int64_t echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoDouble(
double a_double, std::function<void(ErrorOr<double> reply)> result) {
flutter_api_->EchoDouble(
a_double, [result](double echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoString(
const std::string& a_string,
std::function<void(ErrorOr<std::string> reply)> result) {
flutter_api_->EchoString(
a_string, [result](const std::string& echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoUint8List(
const std::vector<uint8_t>& a_list,
std::function<void(ErrorOr<std::vector<uint8_t>> reply)> result) {
flutter_api_->EchoUint8List(
a_list, [result](const std::vector<uint8_t>& echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoList(
const EncodableList& a_list,
std::function<void(ErrorOr<EncodableList> reply)> result) {
flutter_api_->EchoList(
a_list, [result](const EncodableList& echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoMap(
const EncodableMap& a_map,
std::function<void(ErrorOr<EncodableMap> reply)> result) {
flutter_api_->EchoMap(
a_map, [result](const EncodableMap& echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoEnum(
const AnEnum& an_enum, std::function<void(ErrorOr<AnEnum> reply)> result) {
flutter_api_->EchoEnum(
an_enum, [result](const AnEnum& echo) { result(echo); },
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableBool(
const bool* a_bool,
std::function<void(ErrorOr<std::optional<bool>> reply)> result) {
flutter_api_->EchoNullableBool(
a_bool,
[result](const bool* echo) {
result(echo ? std::optional<bool>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableInt(
const int64_t* an_int,
std::function<void(ErrorOr<std::optional<int64_t>> reply)> result) {
flutter_api_->EchoNullableInt(
an_int,
[result](const int64_t* echo) {
result(echo ? std::optional<int64_t>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableDouble(
const double* a_double,
std::function<void(ErrorOr<std::optional<double>> reply)> result) {
flutter_api_->EchoNullableDouble(
a_double,
[result](const double* echo) {
result(echo ? std::optional<double>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableString(
const std::string* a_string,
std::function<void(ErrorOr<std::optional<std::string>> reply)> result) {
flutter_api_->EchoNullableString(
a_string,
[result](const std::string* echo) {
result(echo ? std::optional<std::string>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableUint8List(
const std::vector<uint8_t>* a_list,
std::function<void(ErrorOr<std::optional<std::vector<uint8_t>>> reply)>
result) {
flutter_api_->EchoNullableUint8List(
a_list,
[result](const std::vector<uint8_t>* echo) {
result(echo ? std::optional<std::vector<uint8_t>>(*echo)
: std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableList(
const EncodableList* a_list,
std::function<void(ErrorOr<std::optional<EncodableList>> reply)> result) {
flutter_api_->EchoNullableList(
a_list,
[result](const EncodableList* echo) {
result(echo ? std::optional<EncodableList>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableMap(
const EncodableMap* a_map,
std::function<void(ErrorOr<std::optional<EncodableMap>> reply)> result) {
flutter_api_->EchoNullableMap(
a_map,
[result](const EncodableMap* echo) {
result(echo ? std::optional<EncodableMap>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
void TestPlugin::CallFlutterEchoNullableEnum(
const AnEnum* an_enum,
std::function<void(ErrorOr<std::optional<AnEnum>> reply)> result) {
flutter_api_->EchoNullableEnum(
an_enum,
[result](const AnEnum* echo) {
result(echo ? std::optional<AnEnum>(*echo) : std::nullopt);
},
[result](const FlutterError& error) { result(error); });
}
} // namespace test_plugin
| packages/packages/pigeon/platform_tests/test_plugin/windows/test_plugin.cpp/0 | {
"file_path": "packages/packages/pigeon/platform_tests/test_plugin/windows/test_plugin.cpp",
"repo_id": "packages",
"token_count": 7220
} | 1,114 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:pigeon/generator_tools.dart';
import 'package:test/test.dart';
void main() {
test('pigeon version matches pubspec', () {
final String pubspecPath = '${Directory.current.path}/pubspec.yaml';
final String pubspec = File(pubspecPath).readAsStringSync();
final RegExp regex = RegExp(r'version:\s*(.*?) #');
final RegExpMatch? match = regex.firstMatch(pubspec);
expect(match, isNotNull);
expect(pigeonVersion, match?.group(1)?.trim());
});
}
| packages/packages/pigeon/test/version_test.dart/0 | {
"file_path": "packages/packages/pigeon/test/version_test.dart",
"repo_id": "packages",
"token_count": 227
} | 1,115 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io' as io show Platform, stdin, stdout;
import 'platform.dart';
/// `Platform` implementation that delegates directly to `dart:io`.
class LocalPlatform extends Platform {
/// Creates a new [LocalPlatform].
const LocalPlatform();
@override
int get numberOfProcessors => io.Platform.numberOfProcessors;
@override
String get pathSeparator => io.Platform.pathSeparator;
@override
String get operatingSystem => io.Platform.operatingSystem;
@override
String get operatingSystemVersion => io.Platform.operatingSystemVersion;
@override
String get localHostname => io.Platform.localHostname;
@override
Map<String, String> get environment => io.Platform.environment;
@override
String get executable => io.Platform.executable;
@override
String get resolvedExecutable => io.Platform.resolvedExecutable;
@override
Uri get script => io.Platform.script;
@override
List<String> get executableArguments => io.Platform.executableArguments;
@override
String? get packageConfig => io.Platform.packageConfig;
@override
String get version => io.Platform.version;
@override
bool get stdinSupportsAnsi => io.stdin.supportsAnsiEscapes;
@override
bool get stdoutSupportsAnsi => io.stdout.supportsAnsiEscapes;
@override
String get localeName => io.Platform.localeName;
}
| packages/packages/platform/lib/src/interface/local_platform.dart/0 | {
"file_path": "packages/packages/platform/lib/src/interface/local_platform.dart",
"repo_id": "packages",
"token_count": 439
} | 1,116 |
## test
This package uses integration tests for testing.
See `example/README.md` for more info.
| packages/packages/pointer_interceptor/pointer_interceptor/test/README.md/0 | {
"file_path": "packages/packages/pointer_interceptor/pointer_interceptor/test/README.md",
"repo_id": "packages",
"token_count": 28
} | 1,117 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pointer_interceptor_ios/pointer_interceptor_ios.dart';
class TestApp extends StatefulWidget {
const TestApp({super.key});
@override
State<StatefulWidget> createState() {
return TestAppState();
}
}
class TestAppState extends State<TestApp> {
String _buttonText = 'Test Button';
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: const Text('Body'),
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: PointerInterceptorIOS().buildWidget(
child: TextButton(
onPressed: () => setState(() {
_buttonText = 'Clicked';
}),
child: Text(_buttonText),
))),
));
}
}
void main() {
testWidgets(
'Button remains clickable and is added to '
'hierarchy after being wrapped in pointer interceptor',
(WidgetTester tester) async {
await tester.pumpWidget(const TestApp());
await tester.tap(find.text('Test Button'));
await tester.pump();
expect(find.text('Clicked'), findsOneWidget);
});
}
| packages/packages/pointer_interceptor/pointer_interceptor_ios/test/pointer_interceptor_ios_test.dart/0 | {
"file_path": "packages/packages/pointer_interceptor/pointer_interceptor_ios/test/pointer_interceptor_ios_test.dart",
"repo_id": "packages",
"token_count": 522
} | 1,118 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: avoid_print
// Imports the Flutter Driver API.
import 'package:flutter/src/widgets/framework.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
import 'package:pointer_interceptor_web_example/main.dart' as app;
import 'package:web/web.dart' as web;
final Finder nonClickableButtonFinder =
find.byKey(const Key('transparent-button'));
final Finder clickableWrappedButtonFinder =
find.byKey(const Key('wrapped-transparent-button'));
final Finder clickableButtonFinder = find.byKey(const Key('clickable-button'));
final Finder backgroundFinder = find.byKey(const Key('background-widget'));
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
group('Without semantics', () {
testWidgets(
'on wrapped elements, the browser does not hit the background-html-view',
(WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAtCenter(clickableButtonFinder, tester);
expect(element.id, isNot('background-html-view'));
}, semanticsEnabled: false);
testWidgets(
'on wrapped elements with intercepting set to false, the browser hits the background-html-view',
(WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAtCenter(clickableWrappedButtonFinder, tester);
expect(element.id, 'background-html-view');
}, semanticsEnabled: false);
testWidgets(
'on unwrapped elements, the browser hits the background-html-view',
(WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAtCenter(nonClickableButtonFinder, tester);
expect(element.id, 'background-html-view');
}, semanticsEnabled: false);
testWidgets('on background directly', (WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAt(tester.getTopLeft(backgroundFinder));
expect(element.id, 'background-html-view');
}, semanticsEnabled: false);
});
group('With semantics', () {
testWidgets('finds semantics of wrapped widgets',
(WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAtCenter(clickableButtonFinder, tester);
expect(element.tagName.toLowerCase(), 'flt-semantics');
expect(element.getAttribute('aria-label'), 'Works As Expected');
},
// TODO(bparrishMines): The semantics label is returning null.
// See https://github.com/flutter/flutter/issues/145238
skip: true);
testWidgets(
'finds semantics of wrapped widgets with intercepting set to false',
(WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAtCenter(clickableWrappedButtonFinder, tester);
expect(element.tagName.toLowerCase(), 'flt-semantics');
expect(element.getAttribute('aria-label'),
'Never calls onPressed transparent');
},
// TODO(bparrishMines): The semantics label is returning null.
// See https://github.com/flutter/flutter/issues/145238
skip: true);
testWidgets('finds semantics of unwrapped elements',
(WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAtCenter(nonClickableButtonFinder, tester);
expect(element.tagName.toLowerCase(), 'flt-semantics');
expect(element.getAttribute('aria-label'), 'Never calls onPressed');
},
// TODO(bparrishMines): The semantics label is returning null.
// See https://github.com/flutter/flutter/issues/145238
skip: true);
// Notice that, when hit-testing the background platform view, instead of
// finding a semantics node, the platform view itself is found. This is
// because the platform view does not add interactive semantics nodes into
// the framework's semantics tree. Instead, its semantics is determined by
// the HTML content of the platform view itself. Flutter's semantics tree
// simply allows the hit test to land on the platform view by making itself
// hit test transparent.
testWidgets('on background directly', (WidgetTester tester) async {
await _fullyRenderApp(tester);
final web.Element element =
_getHtmlElementAt(tester.getTopLeft(backgroundFinder));
expect(element.id, 'background-html-view');
});
});
}
Future<void> _fullyRenderApp(WidgetTester tester) async {
await tester.pumpWidget(const app.MyApp());
// Pump 2 frames so the framework injects the platform view into the DOM.
await tester.pump();
await tester.pump();
}
// Calls [_getHtmlElementAt] passing it the center of the widget identified by
// the `finder`.
web.Element _getHtmlElementAtCenter(Finder finder, WidgetTester tester) {
final Offset point = tester.getCenter(finder);
return _getHtmlElementAt(point);
}
// Locates the DOM element at the given `point` using `elementFromPoint`.
//
// `elementFromPoint` is an approximate proxy for a hit test, although it's
// sensitive to the presence of shadow roots and browser quirks (not all
// browsers agree on what it should return in all situations). Since this test
// runs only in Chromium, it relies on Chromium's behavior.
web.Element _getHtmlElementAt(Offset point) {
// Probe at the shadow so the browser reports semantics nodes in addition to
// platform view elements. If probed from `html.document` the browser hides
// the contents of <flt-glass-name> as an implementation detail.
final web.ShadowRoot glassPaneShadow =
web.document.querySelector('flt-glass-pane')!.shadowRoot!;
// Use `round` below to ensure clicks always fall *inside* the located
// element, rather than truncating the decimals.
// Truncating decimals makes some tests fail when a centered element (in high
// DPI) is not exactly aligned to the pixel grid (because the browser *rounds*)
return glassPaneShadow.elementFromPoint(point.dx.round(), point.dy.round());
}
/// Shady API: https://github.com/w3c/csswg-drafts/issues/556
extension ElementFromPointInShadowRoot on web.ShadowRoot {
external web.Element elementFromPoint(int x, int y);
}
| packages/packages/pointer_interceptor/pointer_interceptor_web/example/integration_test/widget_test.dart/0 | {
"file_path": "packages/packages/pointer_interceptor/pointer_interceptor_web/example/integration_test/widget_test.dart",
"repo_id": "packages",
"token_count": 2194
} | 1,119 |
# quick_actions
This Flutter plugin allows you to manage and interact with the application's
home screen quick actions.
Quick actions refer to the [eponymous
concept](https://developer.apple.com/design/human-interface-guidelines/home-screen-quick-actions)
on iOS and to the [App
Shortcuts](https://developer.android.com/guide/topics/ui/shortcuts.html) APIs on
Android.
| | Android | iOS |
|-------------|-----------|------|
| **Support** | SDK 16+\* | 9.0+ |
## Usage
Initialize the library early in your application's lifecycle by providing a
callback, which will then be called whenever the user launches the app via a
quick action.
```dart
final QuickActions quickActions = const QuickActions();
quickActions.initialize((shortcutType) {
if (shortcutType == 'action_main') {
print('The user tapped on the "Main view" action.');
}
// More handling code...
});
```
Finally, manage the app's quick actions, for instance:
```dart
quickActions.setShortcutItems(<ShortcutItem>[
const ShortcutItem(type: 'action_main', localizedTitle: 'Main view', icon: 'icon_main'),
const ShortcutItem(type: 'action_help', localizedTitle: 'Help', icon: 'icon_help')
]);
```
Please note, that the `type` argument should be unique within your application
(among all the registered shortcut items). The optional `icon` should be the
name of the native resource (xcassets on iOS or drawable on Android) that the app will display for the
quick action.
### Android
\* The plugin will compile and run on SDK 16+, but will be a no-op below SDK 25
(Android 7.1).
If the drawables used as icons are not referenced other than in your Dart code,
you may need to
[explicitly mark them to be kept](https://developer.android.com/studio/build/shrink-code#keep-resources)
to ensure that they will be available for use in release builds.
| packages/packages/quick_actions/quick_actions/README.md/0 | {
"file_path": "packages/packages/quick_actions/quick_actions/README.md",
"repo_id": "packages",
"token_count": 536
} | 1,120 |
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style
name="LaunchTheme" parent="@android:style/Theme.Black.NoTitleBar">
<item name="android:windowBackground">@drawable/ic_launcher_background</item>
</style>
</resources> | packages/packages/quick_actions/quick_actions/example/android/app/src/main/res/values/styles.xml/0 | {
"file_path": "packages/packages/quick_actions/quick_actions/example/android/app/src/main/res/values/styles.xml",
"repo_id": "packages",
"token_count": 84
} | 1,121 |